A complete remaster of my pico-8 game!
The controls are Z/C to Jump, and X/V = Shoot (or respawn if you become a slimepire)
Because picotron cartridges are much more spacious, I've been able to tidy up the code and comment ALL of it, so if there's anything you're curious about - take a look! It shouldn't be too hard to see how it works.
WARNING: your progress won't be saved playing in a browser! Best to get Picotron and load #slimepires
~ Extras ~
If you tipped $3 or more on the original game at itch, you can get access to new extras including a second video talking about this project and new maps made from in-game screenshots.
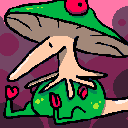
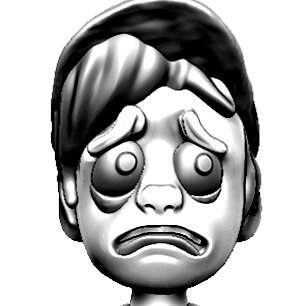
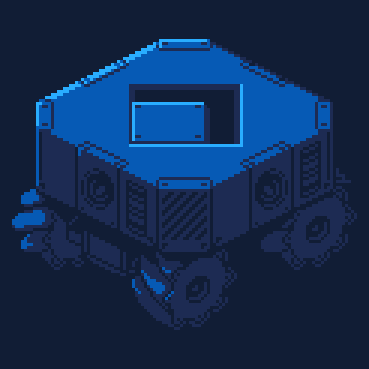


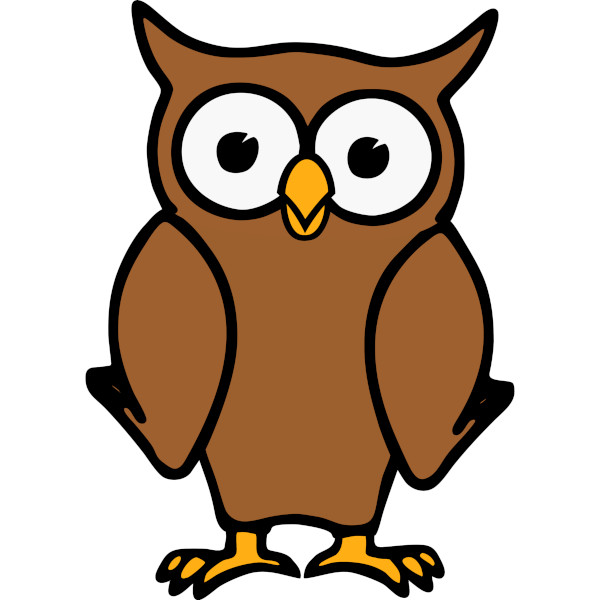


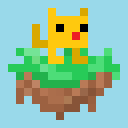
So I was curious if picotron would be capable of running the original Lemmings game as it was on Amiga, it turns out - yes!
Well, with the exception of no halfwidth pixels for the UI. But otherwise, you could make a 1:1 clone. Which I started doing, but then I thought it would be more fun if I pretended Psygnosis had time-travelled and asked me to make a picotron port. So I added extra in-between frames to the classic walk cycle for smoother animation, climbing gloves and umbrellas to indicate lemmings with permanent skills, fast forward (an invaluable feature added after the first games), and a nicer level select.
At the moment there are only a handful of levels and only one half-finished music track, I hope to work on more and have all the levels from the first four games (Lemmings, Oh No! More Lemmings, and the two holiday packs from '93 and '94), buuuuut I don't know that I'd be comfortable posting that here (or at all) - Lemmings is somebody else's IP after all. This is a fun hobby project for me, but if you want to play classic lemmings there's a few other options for you. I'm only really sharing this so that anyone that wants to see how I've done it can check the code, and also it's a way for me to call it a milestone I can step away from - I've got other projects with my own IP to finish!


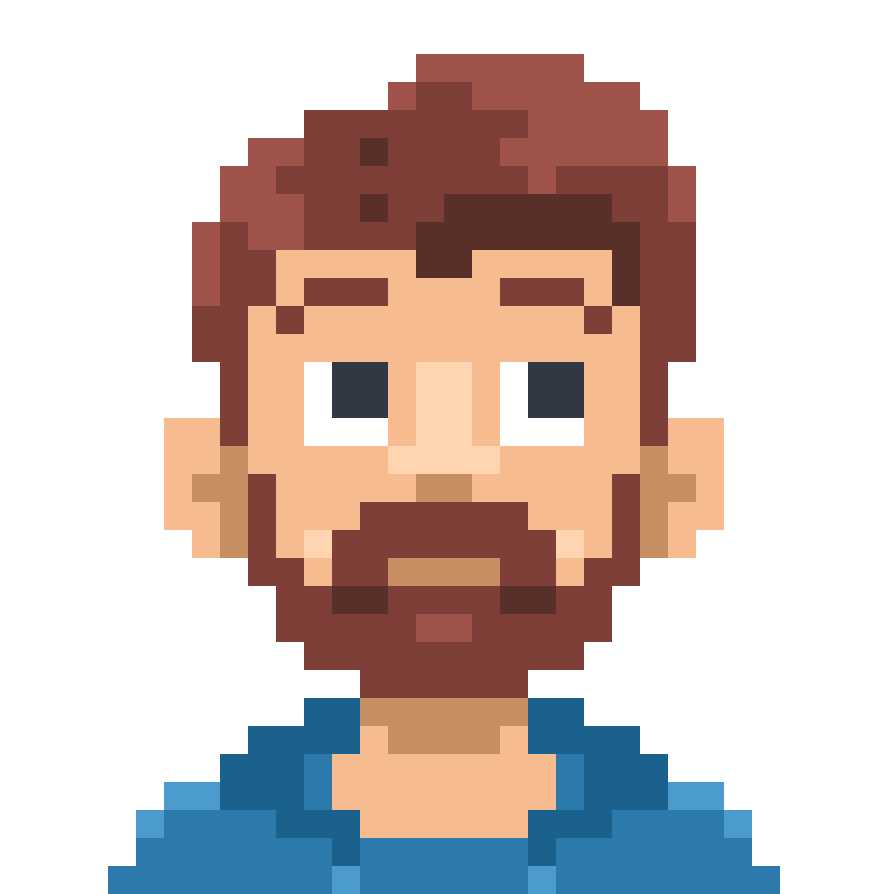

.jpg)

(click for different demos)
I want to make a game using vector animations so I got started on a way for drawing that.
Main tips for speedy drawing:
- Scaling/rotating is slower
- More vector sprites is slower
- Bigger vector sprites is slower
- More triangles is MUCH slower
code here:
[hidden]
--[[ vgfx.lua vector graphics drawing === todo: === -more triangulation functions -tween/interpolate between two vector sprites/polygons -animation playback -compression for vector data -maybe look into userdata for optimisations -maybe try writing directly to graphics memory if it's faster also: -make a vector sprite editor/animator app === docs: === "vector sprite": an array of polygons "polygon": an array with 3 elements: color 1, color 2, vertices "vertices": array of numbers (x0,y0, y1,y2, ...etc) vertices list should be triangulated, sprv() will NOT triangulate arbitrary polygons tri() and triraster() are decent triangle drawing functions, for lightweight vector drawing, skip the vector sprite stuff and just use these ]] vgfx_wire=false--enable for debug view of polygon triangles vgfx_precise=false--enable if you have issues with edge seams function make_polygon(col1,col2, verts) return {col1,col2,verts} end --vector sprite expectations: -- array of polygons -- each polygon is an array with: -- color1, color2, vertices --vertices expectations: -- array of numbers (x0,y0, y1,y2, ...etc) -- should be triangulated already - each three vertices will be drawn as a tri --draw a vector sprite function sprv(v, x,y, rot,scale, col) local s, cs = 0,0 if rot and rot!=0 then s = sin(rot) cs = cos(rot) end if (col) color(col) for p=1,#v do--loop through each polygon in the vector sprite --prep to draw this polygon's colours if not col then fillp(0b1010010110100101) color((v[p][1]<<8)+v[p][2]) end --third item in each polygon is a list of vertices (triangulated!) local t = v[p][3] --draw each tri now local b=0 local l=#t/6 local v0 = {} local v1 = {} local v2 = {} for i=1, l do if rot and rot!=0 then v0 = rotvert(t[1+b],t[2+b],s,cs) v1 = rotvert(t[3+b],t[4+b],s,cs) v2 =rotvert(t[5+b],t[6+b],s,cs) else v0 = {x=t[1+b],y=t[2+b]} v1 = {x=t[3+b],y=t[4+b]} v2 = {x=t[5+b],y=t[6+b]} end if scale and scale!=1 then tri(x+v0.x*scale,y+v0.y*scale, x+v1.x*scale,y+v1.y*scale, x+v2.x*scale,y+v2.y*scale) else tri(x+v0.x,y+v0.y, x+v1.x,y+v1.y, x+v2.x,y+v2.y) end b+=6 end end return tris end function rotvert(x,y,s,c) local v={} v.x=x*c-y*s v.y=x*s+y*c return v end function fan_triangulate(points) local v,i={},3 while i<=#points do add(v,points[i].x) add(v,points[i].y) add(v,points[i-1].x) add(v,points[i-1].y) add(v,points[1].x) add(v,points[1].y) i+=1 end return v end function strip_triangulate(points) if (#points<=3) return points local v,i={},4 add(v,points[1].x) add(v,points[1].y) add(v,points[2].x) add(v,points[2].y) add(v,points[3].x) add(v,points[3].y) while i<=#points do add(v,points[i].x) add(v,points[i].y) add(v,points[i-1].x) add(v,points[i-1].y) add(v,points[i-2].x) add(v,points[i-2].y) i+=1 end return v end vgfx_trisdrawn=0 function tri(x0,y0, x1,y1, x2,y2) vgfx_trisdrawn += 1 if (vgfx_precise) x0,y0,x1,y1,x2,y2 = flr(x0),flr(y0),flr(x1),flr(y1),flr(x2),flr(y2) --wireframe if vgfx_wire then fillp() line(x0,y0, x1,y1, 7) line(x1,y1, x2,y2, 7) line(x2,y2, x0,y0, 7) return end --order the vertices so they are descending from top to bottom --we need this since we are drawing it as two triangles: --one with a flat base, one with a flat top if (y1<y0) x0,x1=x1,x0; y0,y1=y1,y0 if (y2<y0) x0,x2=x2,x0; y0,y2=y2,y0 if (y2<y1) x1,x2=x2,x1; y1,y2=y2,y1 --draw the top half local hh=y1-y0--height of the half local x3=x0+hh*(x2-x0)/(y2-y0)--slicing the tri in two makes another vertex if (y0!=y1) triraster(y0,y1, (x3-x0)/hh,(x1-x0)/hh, x0,x0) --draw the bottom half hh=y2-y1 if (y1!=y2) triraster(y1,y2, (x2-x1)/hh,(x2-x3)/hh, x1,x3) end --draws a filled triangle line-by-line, top-to-bottom --args: top, bottom, step left, step right, left pixel, right pixel function triraster(t,b, sl,sr, pl,pr) for y=t,b do rectfill(pl,y,pr,y) pl+=sl pr+=sr end end |
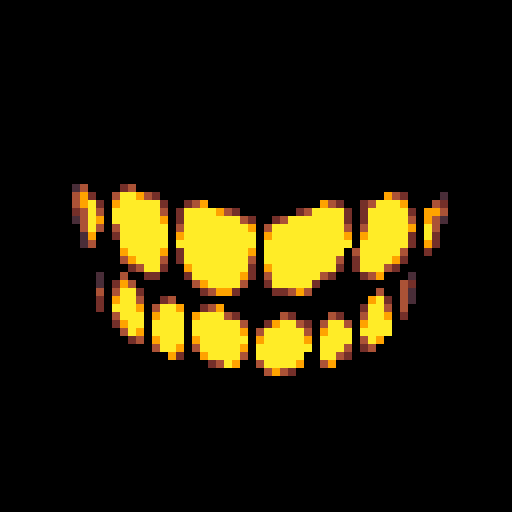
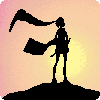
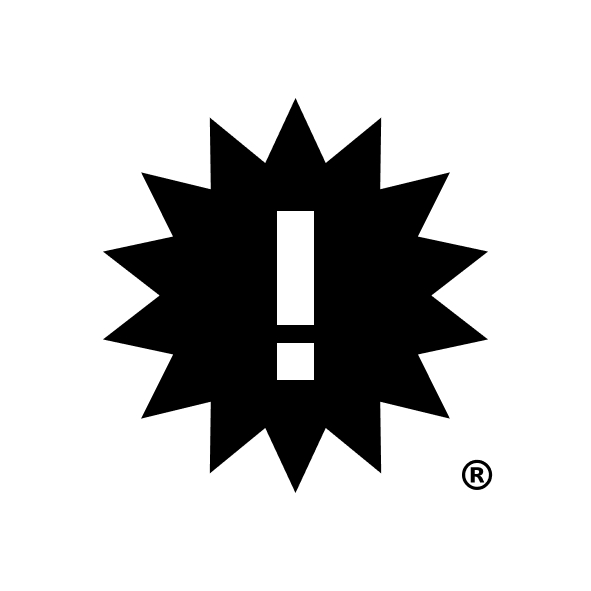
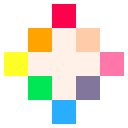
Update: The current version of ENView contains work by pancelor, NuSan, and myself. I'll try to keep it up to date so that load #enview
will always give you the best version, but do scroll this thread for more info as I'm often late to keep things updated!
The largest part of this work is based on pancelor's api explorer
It has some improvements like sub-tables being sorted, and not printing text above/below the screen.
Also I made some style changes to try and get it to gel with the OS as a windowed app.
I'd like to have it so clicking on a function shows you what parameters it expects, but honestly I have no idea if that's a thing you can even query in lua.


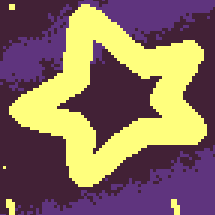
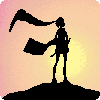
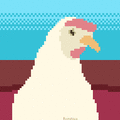

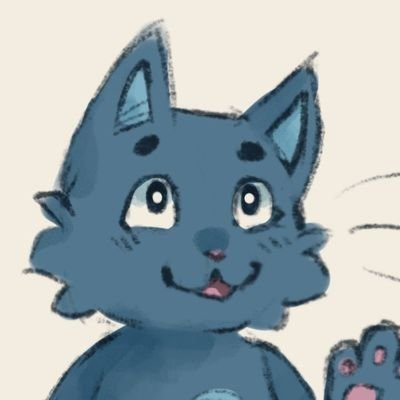
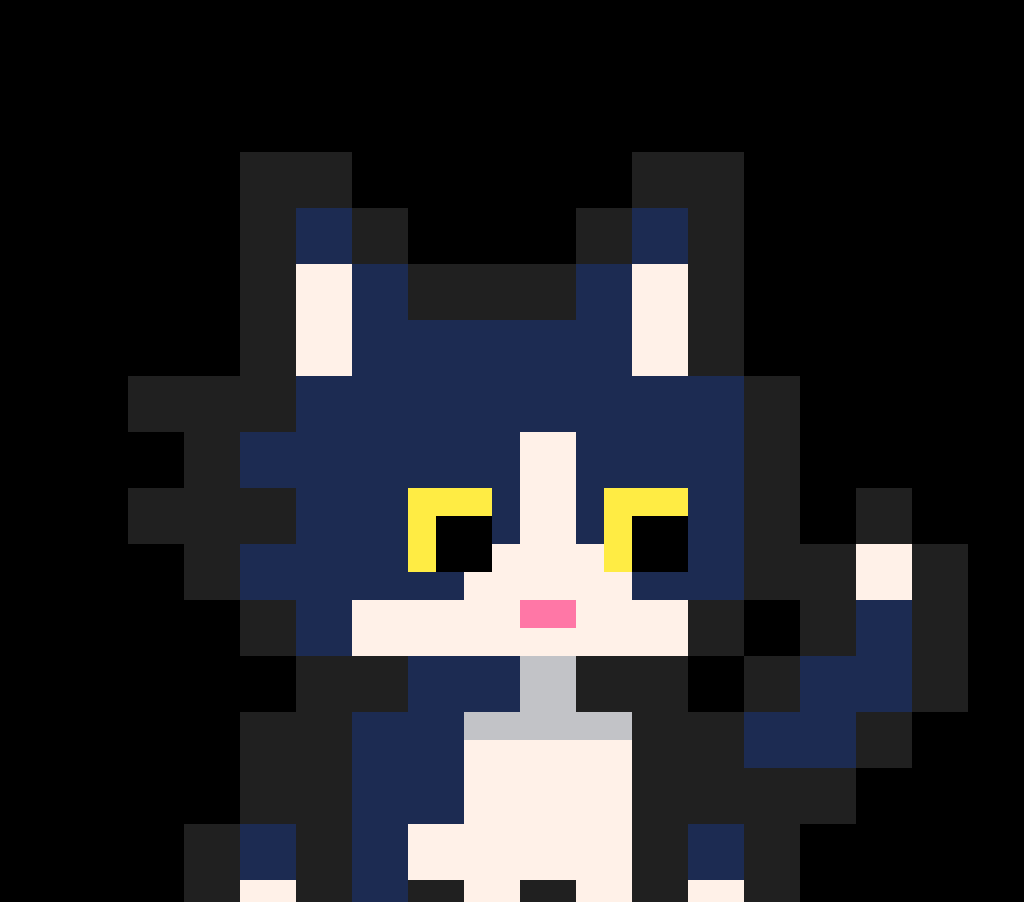

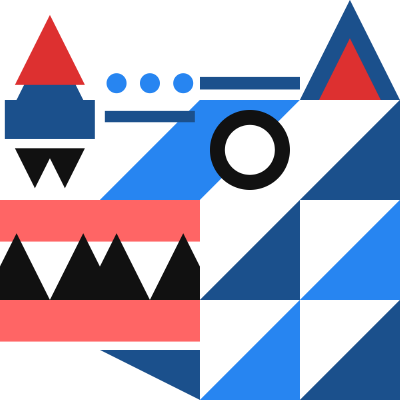
Hi! It's been a while since I have finished a pico-8 game to be able to share it here, but this is one!
The controls are Z/C to Jump, and X/V = Shoot (or respawn if you become a slimepire)
~ Story: ~
Years after the horde have claimed Earth's surface, they find their way into Bunker 4103 - the last bastion of humanity. You awake in a remote part of the underground shelter, and if you want to remain human you must reach the bunker's deepest point: the safe room.
~ Extras ~
You can tip $3 or more on itch.io for access to extra goodies: illustrated & pixel maps, a comprehensive manual, and also a 2 hour video of me talking through the game project and its code.
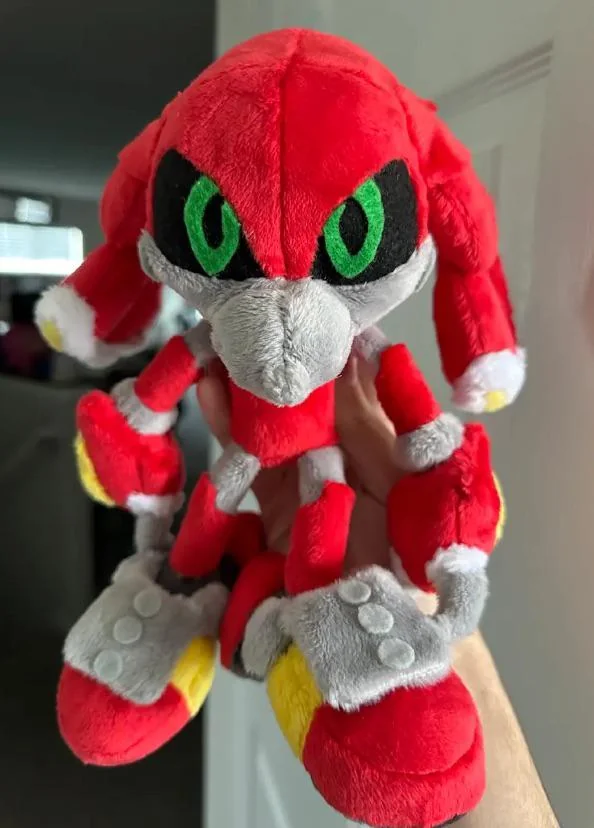

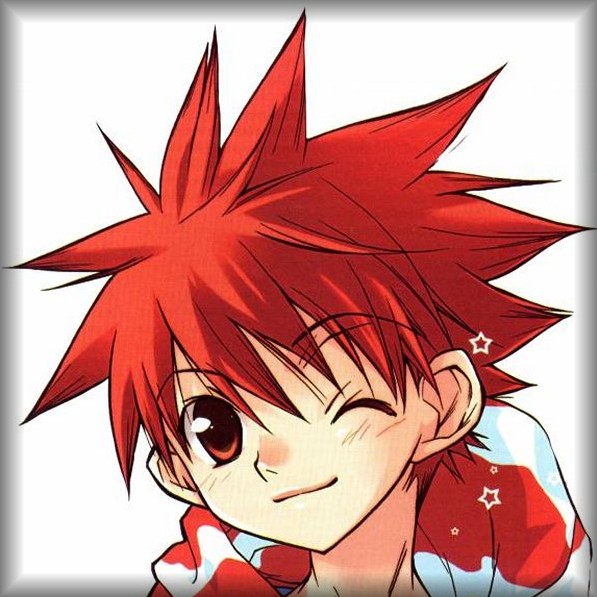

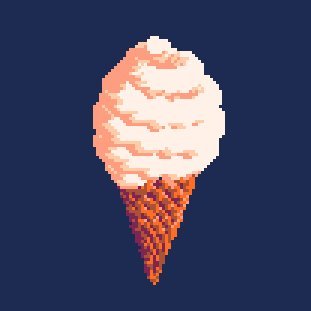
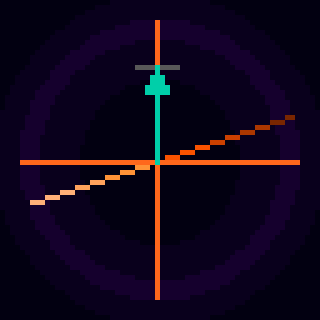


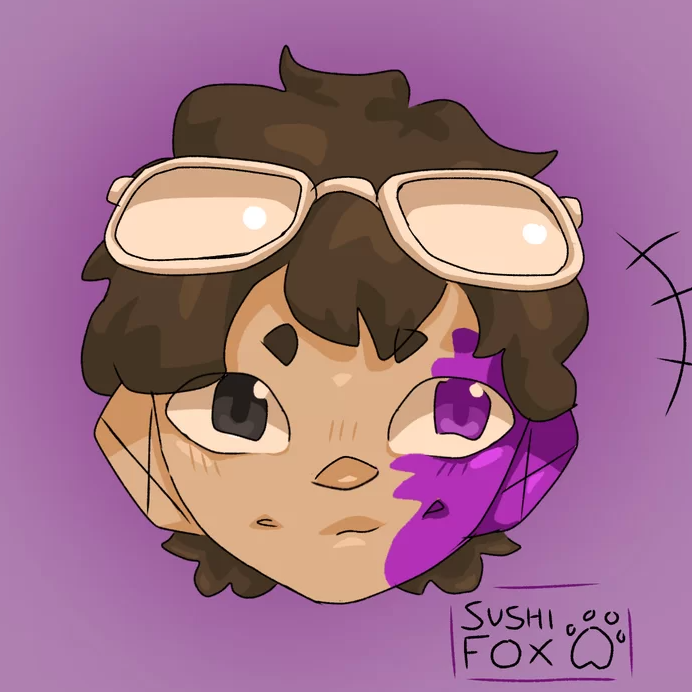
Originally made for Ludum Dare 40, then upgraded a bunch for my patrons, now available for everyone! <3
The vector drawing/animation on the title screen is based on Gabriel Crowe's systems found >here<.
~ Links: ~
Curse of Greed on itch.io
My Patreon - supports things like this ;)
~ Changelog: ~
Curse of Greed - Ludum Dare Release
Ultimate 1.0 - New levels, difficulties, fixes & improvements, title effects
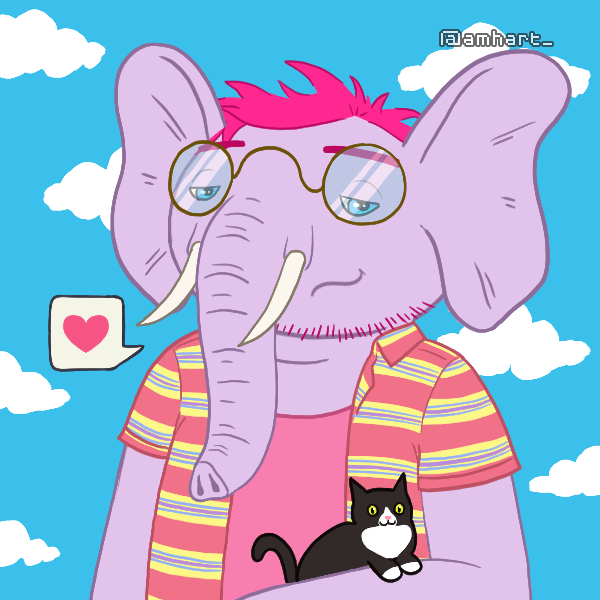

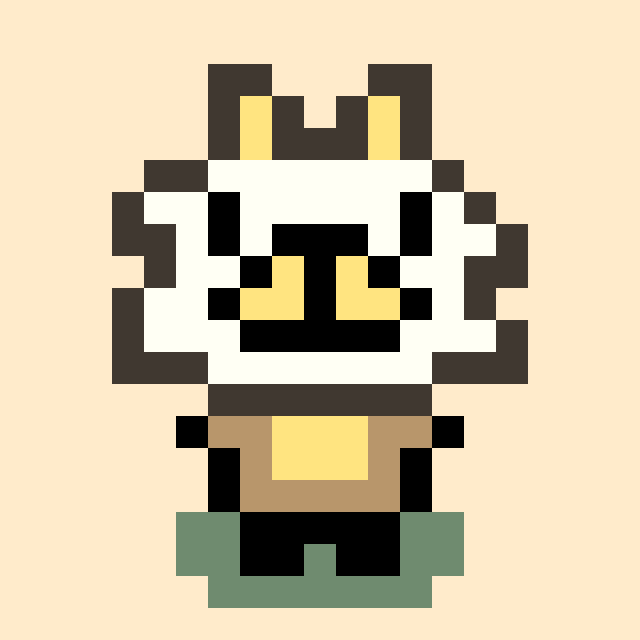

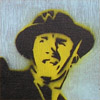
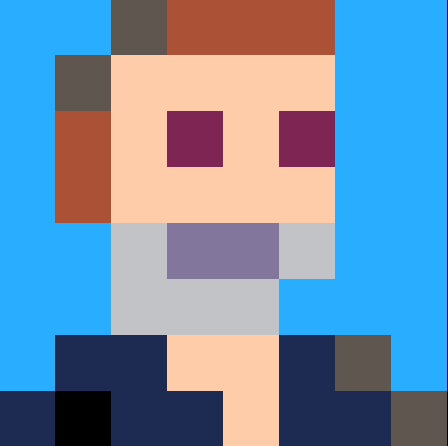
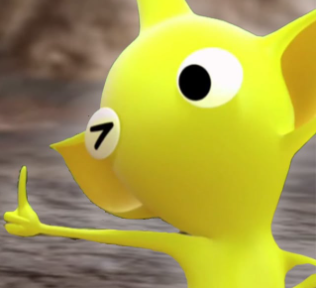
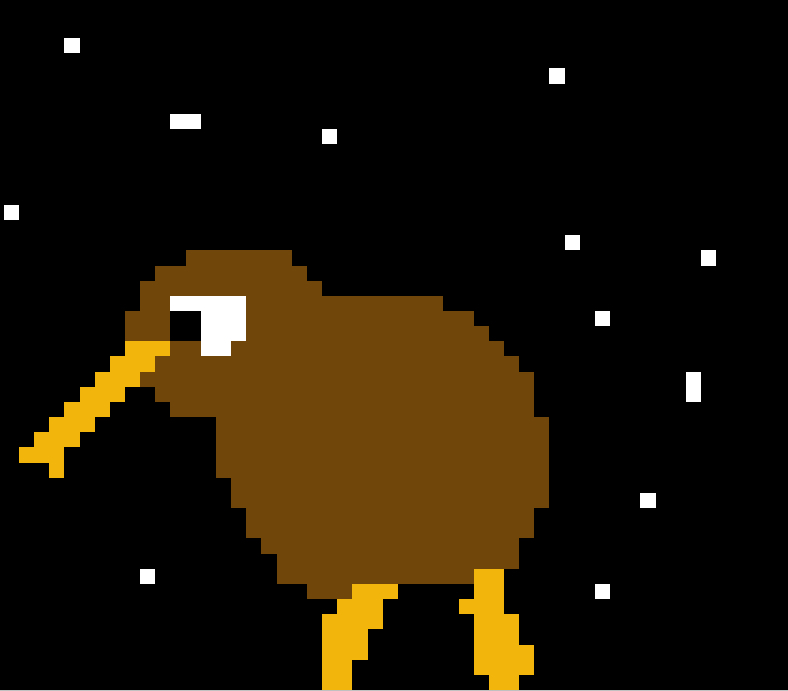
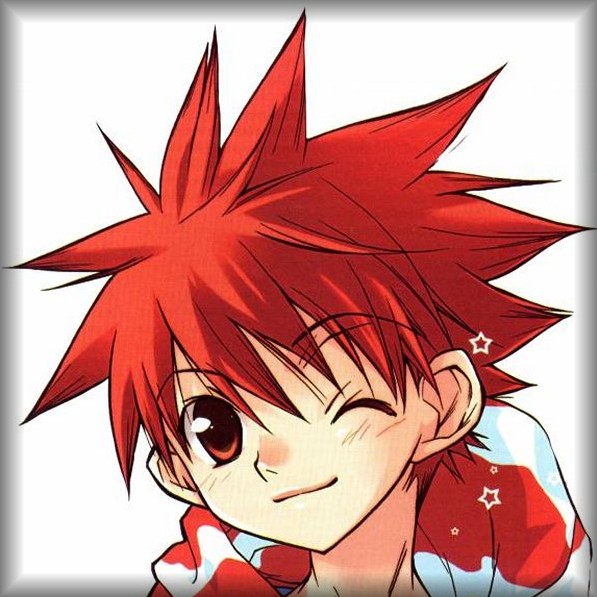
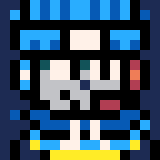
It's a platformer, a difficult one!
I made this in 3 days for my patrons as a Halloween gift, then took an extra day to polish it up and include some spooOOooky music by Tim Monks. Now it's available for everyone, hooray!
Good luck, little pumpkin!
~ Links: ~
Pumpking on itch.io
My Patreon - supports things like this ;)
~ Changelog: ~
1.0 Patreon release
1.1 first public release (Game improvements, added music by Tim Monks, extra boss stages)
1.1a Commented all code for learners, made small background improvements, better cartridge label.

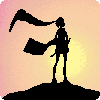

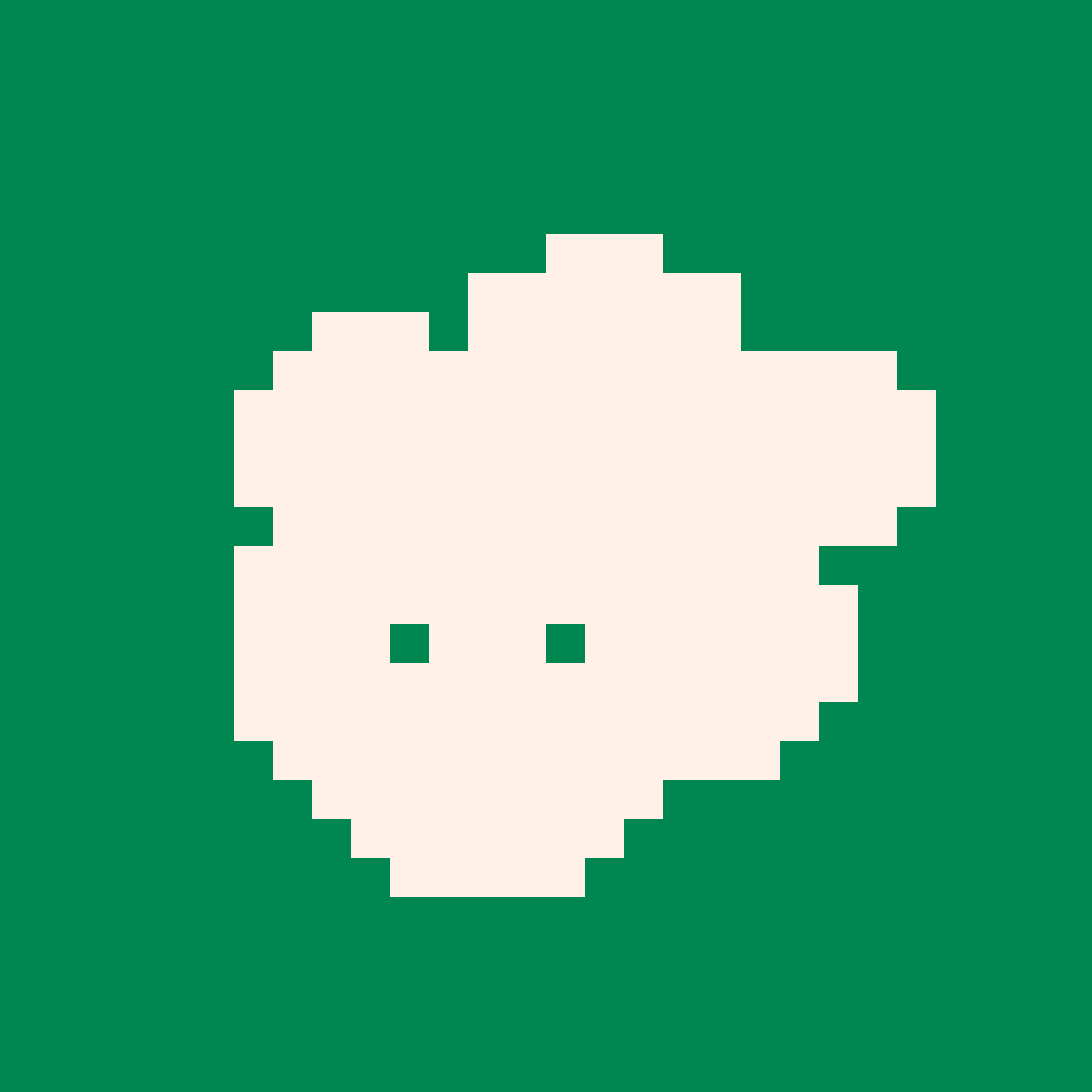

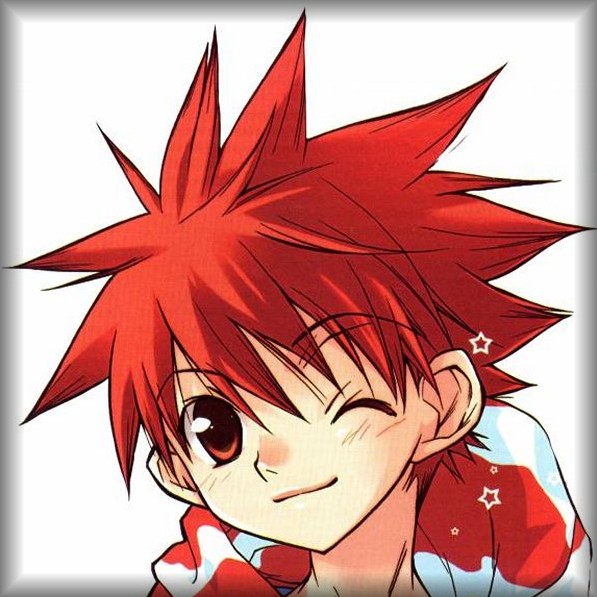
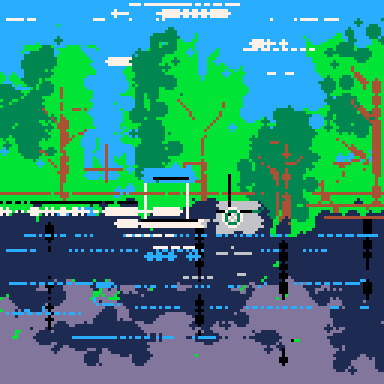
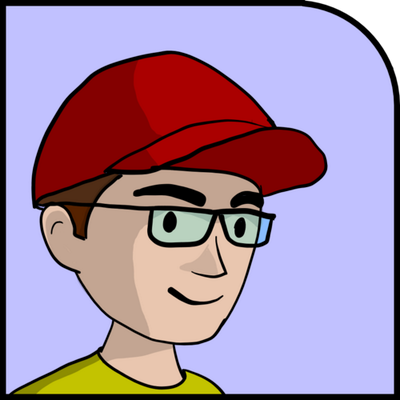

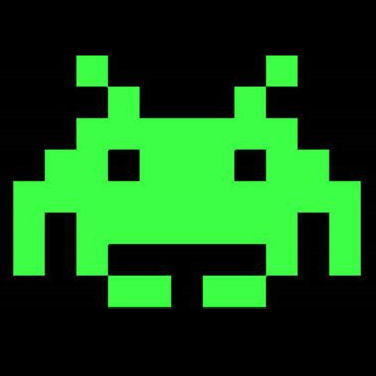
~ Story: ~
Oh no! Zaks the evil wizard has once again captured Dizzy's girlfriend, Daisy! And the other yolkfolk need help too, looks like another adventure for Dizzy!
~
~ Controls: ~
[ Left ]/[ Right ] - Move
[ Z ] - Jump
[ Down ] - Drop from ledge
[ Up ] - Cycle through inventory
[ X ] - Interact/pick up item/drop item
~
~ Music: ~
Huge thanks to @gruber_music for making a brilliant pico-8 version of the Amiga Magicland Dizzy theme :D
~
~ About: ~
I wanted there to be a Dizzy game for Pico-8, so now there is! Heads up though; I've tried to be faithful to the classic Dizzy games - that means limited lives, rubbish platforming, cheesy dialogue and a kidnapped damsel.

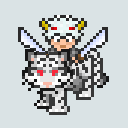

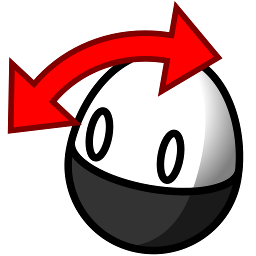
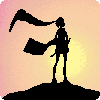
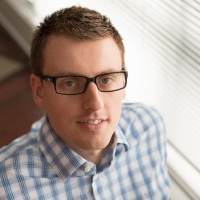
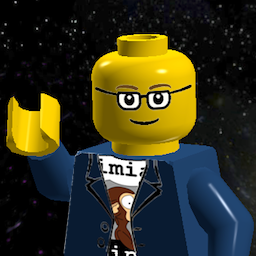

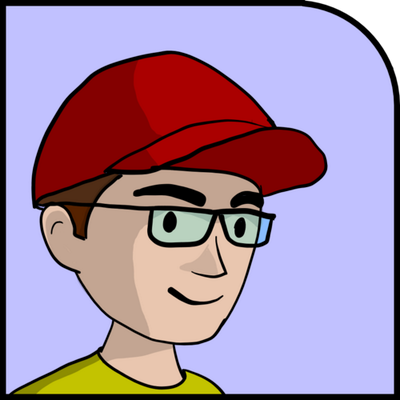

Controls:
Z - Examine/Pick-up/Drop
X - (not used until later in the game)
Up - Jump
Down - Duck/Crawl
Left/Right - Move
Story:
You have been drawn to a mysterious place, what secrets does it hold, and what will they mean for you?
Help:
When not carrying an item, you can examine things. If you're stuck, try examining signs and other objects.
When you have explored far enough into the western temple, you can use the 'X' button.
I'm calling this "done" though there are a few things I might touch up in the future. Right now though, I've spent too long working on this already and need to get back to work on things that stand a chance of making some money :P
1.4: fixed map initialisation corrupting some sprites
1.3: fixed a bug & added some minor text






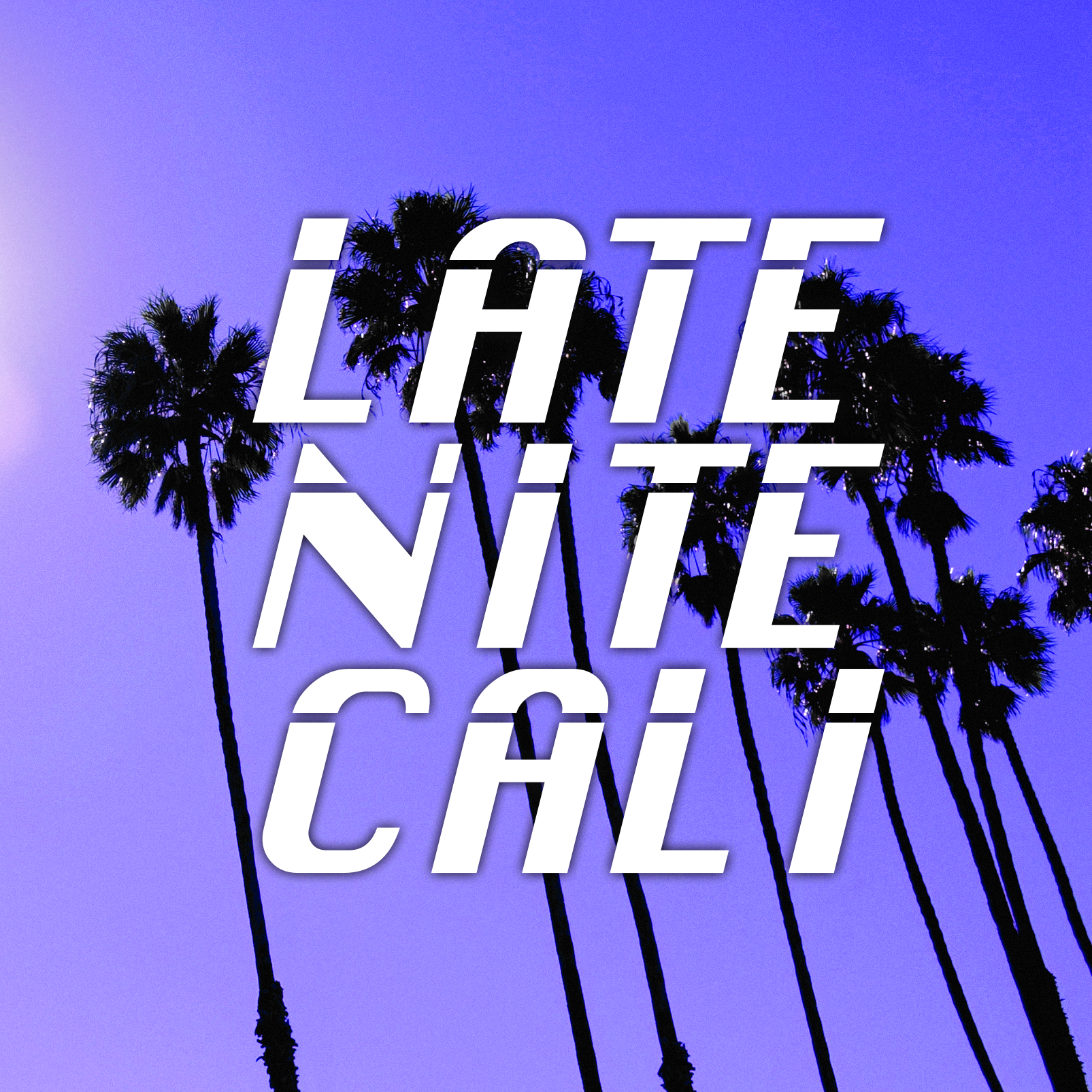


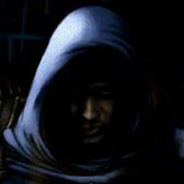