I'm not sure if I should post this here or in the "Tutorials"-Section, but I think it better fits here:
I wanted to show a way on how to do Map-Collision, using the Flag-System in PICO8. This Function also takes care if your Sprite is wider or taller than 8 Pixels.
Basically you give a Function a few Parameters (like Position, Width & Height from the Player) and this Function checks the Flags from the Tile-Map on certain Pixels, depending on the Player-Position and it's Width/Height. You can use the Return-Value from this Function to allow or deny Player-Movement.
Before I show the "How-To use the essential Function", there's some preparation that you need to do.



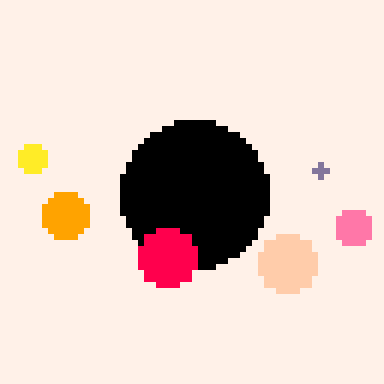

Hey there,
A Feature Request for the PICO8-Users out there which use a Handheld (like Anbernic RG351P/M/V) to play PICO8-Games:
Normally, you can start every Cartridge directly using "-run" in the Command Line:
pico8 -run some_cartridge.p8 |
On Handheld-Devices, that's great, because you can Pause and Shutdown PICO8 with the given Controls.
HOWEVER: I was able to run a Cartridge that "crashed" PICO8 (means it shows some Lua-Errors and the Program stops running). But after that Crash, PICO8 doesn't quit, instead it throws me into the Command line, like:
(example crash)
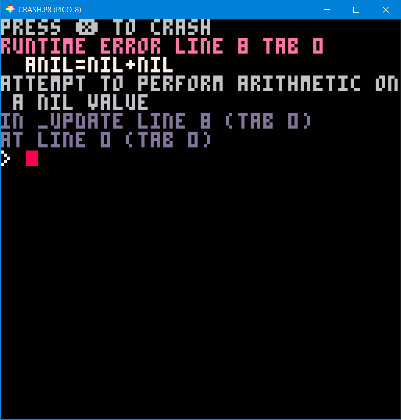
But that's not good if that happens on a Handheld, because you have no opportunity now to close PICO8 without a Keyboard (or something hacky like SSH etc.). After that, I have no other choice than Poweroff that Handheld and hoping that the Filesystem on the SD-Card don't get corrupted.

Hello there,
Krystman made an excellent Game called Porklike, you can find it here: https://www.lexaloffle.com/bbs/?pid=94445
I made a mod to this game which adds a german translation to it. Right after the Game starts, you'll be asked on which language you want to play.
I would've liked to add some more Functionality (like, a menuitem), but there wasn't enough tokens left to do so^^.
Here's the Mod:
Changelog:
Hey there,
In the 0.2.2 release, zep made a small tool for creating custom fonts:
https://www.lexaloffle.com/bbs/?tid=41544
I'd like to just slightly modify the Original-Font that provides PICO-8 by itself. I thought I could find an easy way to implement the Original-Font into the Spritesheet... But it seems there's no easy way to do so? And nobody did this before? So I decided to modify the Spritesheet, include the Original-Chars with it.
It SHOULD be the Original-Chars; if you find an error (misplaced Pixel or something like that), feel free to tell me so I can fix this :) .
--
While running PICO-8, type
import #font_orig |
to load the newest Version of the Cartridge.
EDIT: Of course, feel free to use it as you like^^.
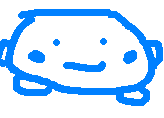
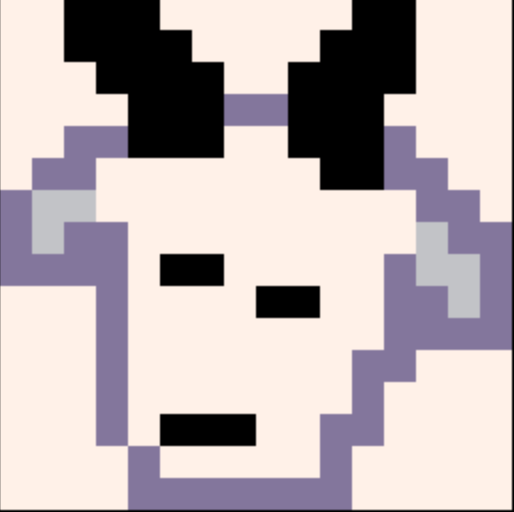
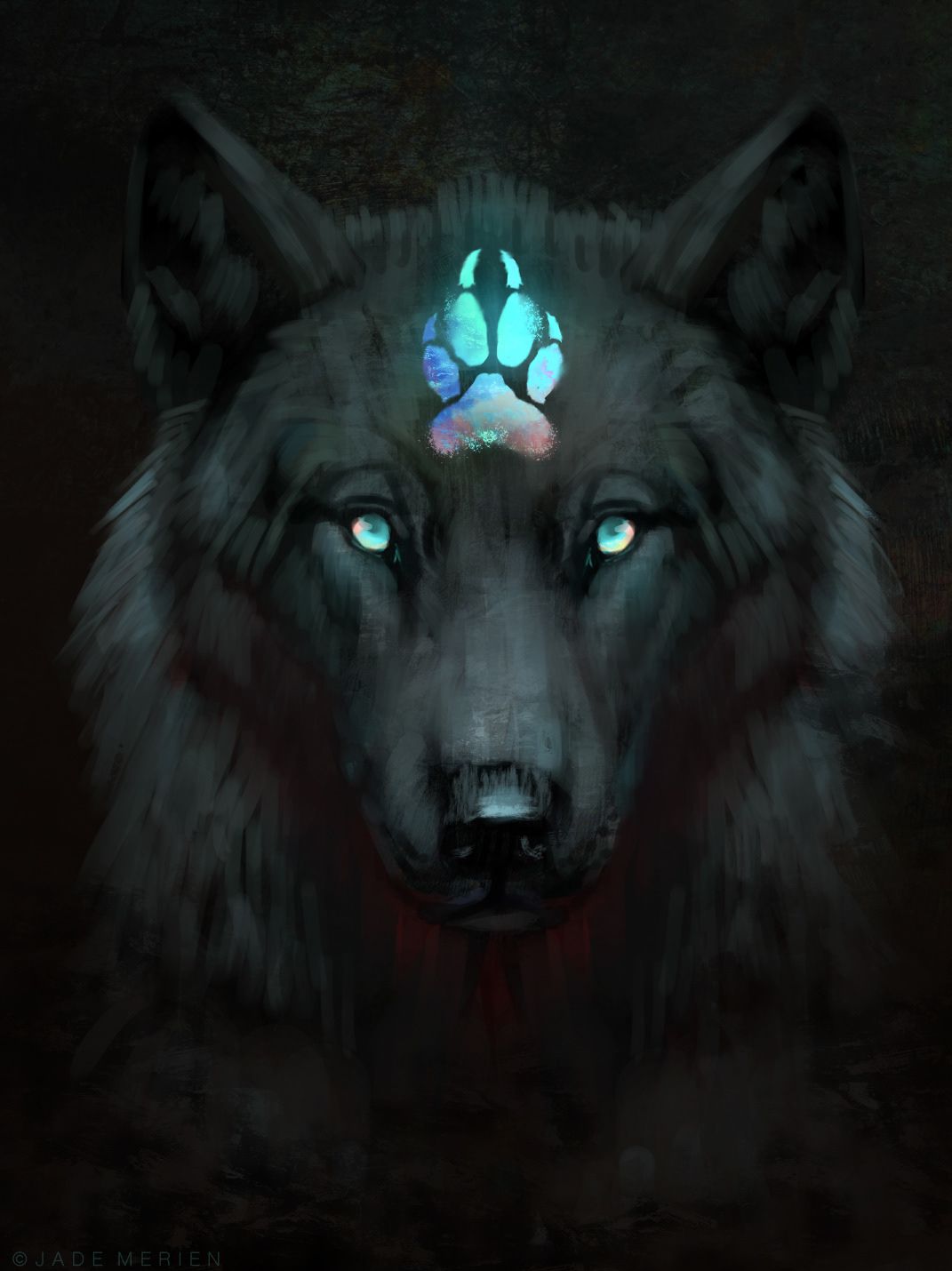
Hi @ all,
I like those old "Oddworld"-Games ("Abe's Oddyssey" and "Abe's Exoddus"). Just experimenting with a "Gamespeak"-like Control:
Absolutely nothing is finished, just the Gamespeak, Collision and the Animation works. No Gameplay so far.
For Gamespeak, hold Z and
UP: "Hello"
RIGHT: "Follow Me"
DOWN: "Wait"
LEFT: "Everyone"
I really want to make an Oddworld-like Demake, but I can't promise anything^^. I'll try to work on that Project^^...
Entry for the One Hour Game Jam 297th, Theme: Follow.
A Shadow is following you, and you need to reach the Goal. Your Shadow(s) also need to reach a Goal.
Note:
It took me four Hours instead of only one to make this Cart. It would be dishonest to other Jammers if I would hide that fact^^.
I like the Idea, maybe I'll work on some more Levels and a better presentation, but I really don't know atm^^.
Have Fun!
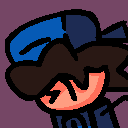
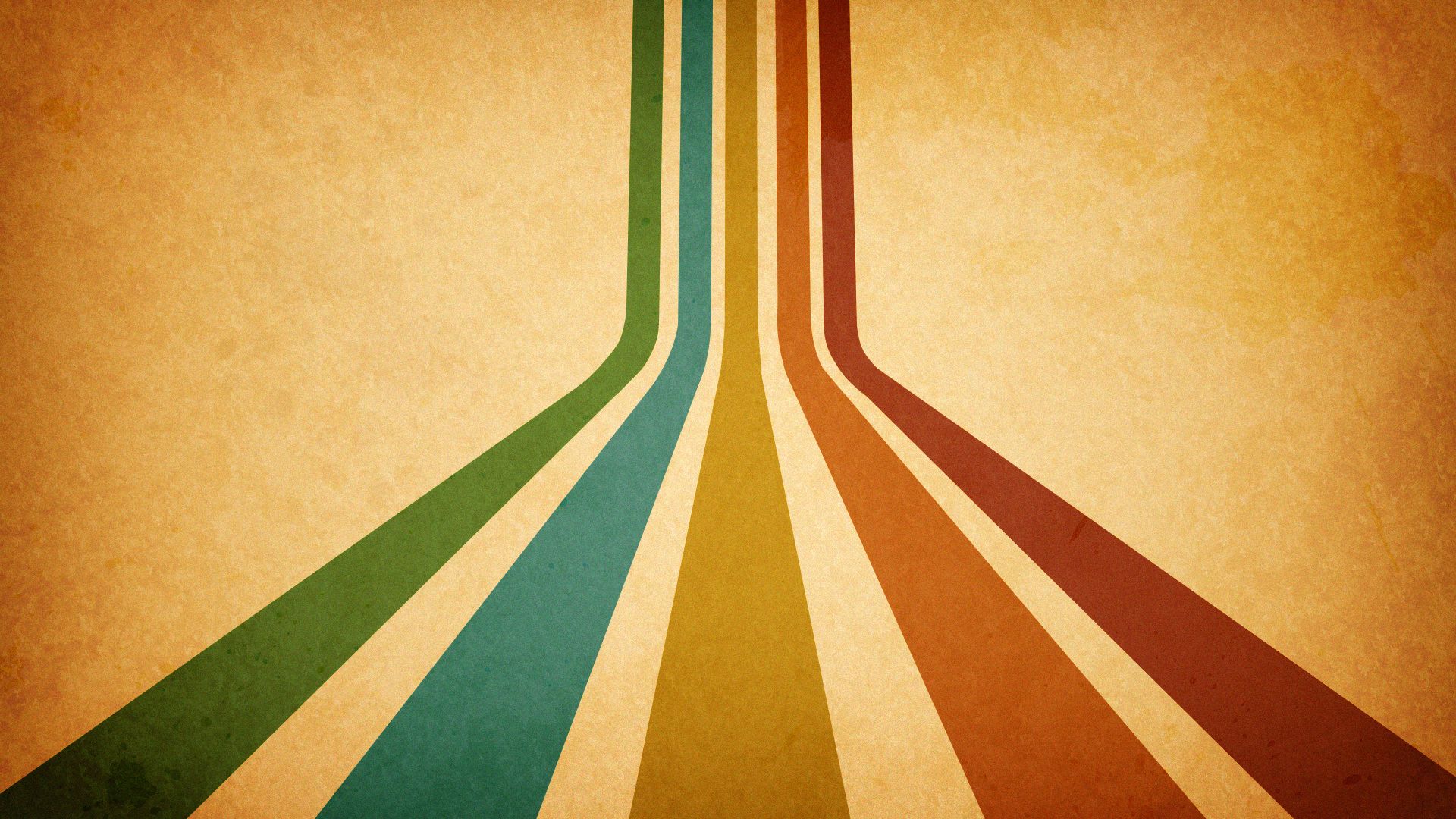
Mea's Castle
Mea's Castle is a Metroidvania-like Game: You're a little Adventurer and your Goal is to find the "Cup of Hope". You can run and jump, and soon you will find some Artifacts that'll give you more Abilities.
🠔🠖 - Move
🠗 + 🠔🠖 - Crouch
(Z) - Jump
(X) + 🠗 - Use the last Powerup
Warning
This Game is difficult. If you're not a Fan of Jump'n Run-like Games, I think it will be too frustrating^^. There are still Checkpoints basically everywhere, but it's still not easy to beat that Game. Expect to die a lot while playing this^^.
Credits
Thanks to
- @Gruber for the Music "Eyes in the Dark" and "Flight of Icarus" (both with slight Remixes) from Pico-8 Tunes Volume 2 .






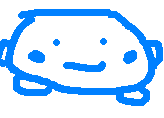
.jpg)
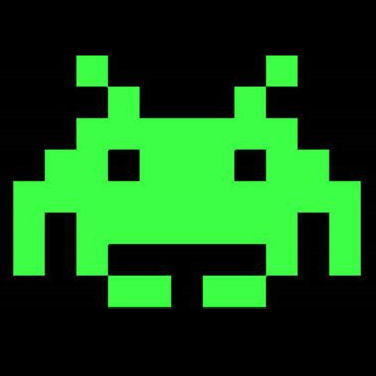

I doubt that's an intended behaviour: After running a Cart that's using the "FILLP"-Command, every Draw in the Sprite-Editor that includes Pencil or Shape (the last one; not shown in the GIF) also uses this pattern:
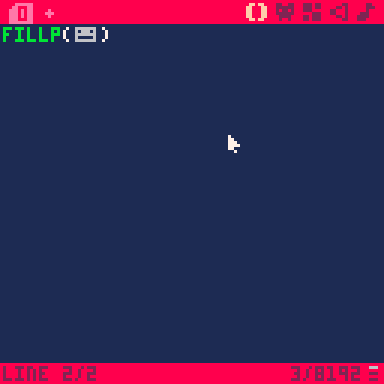
On 0.2.1B, it's possible to use "fillp(â–ˆ)" (or simply reboot) to solve that Problem...
(I was working on a Game and tried to change a Sprite, and I wondered that I suddenly couldn't overwrite some Pixels in the Sprite anymore. I was like "what the Hell is going on there?" until I discovered that^^)

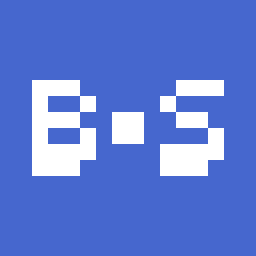
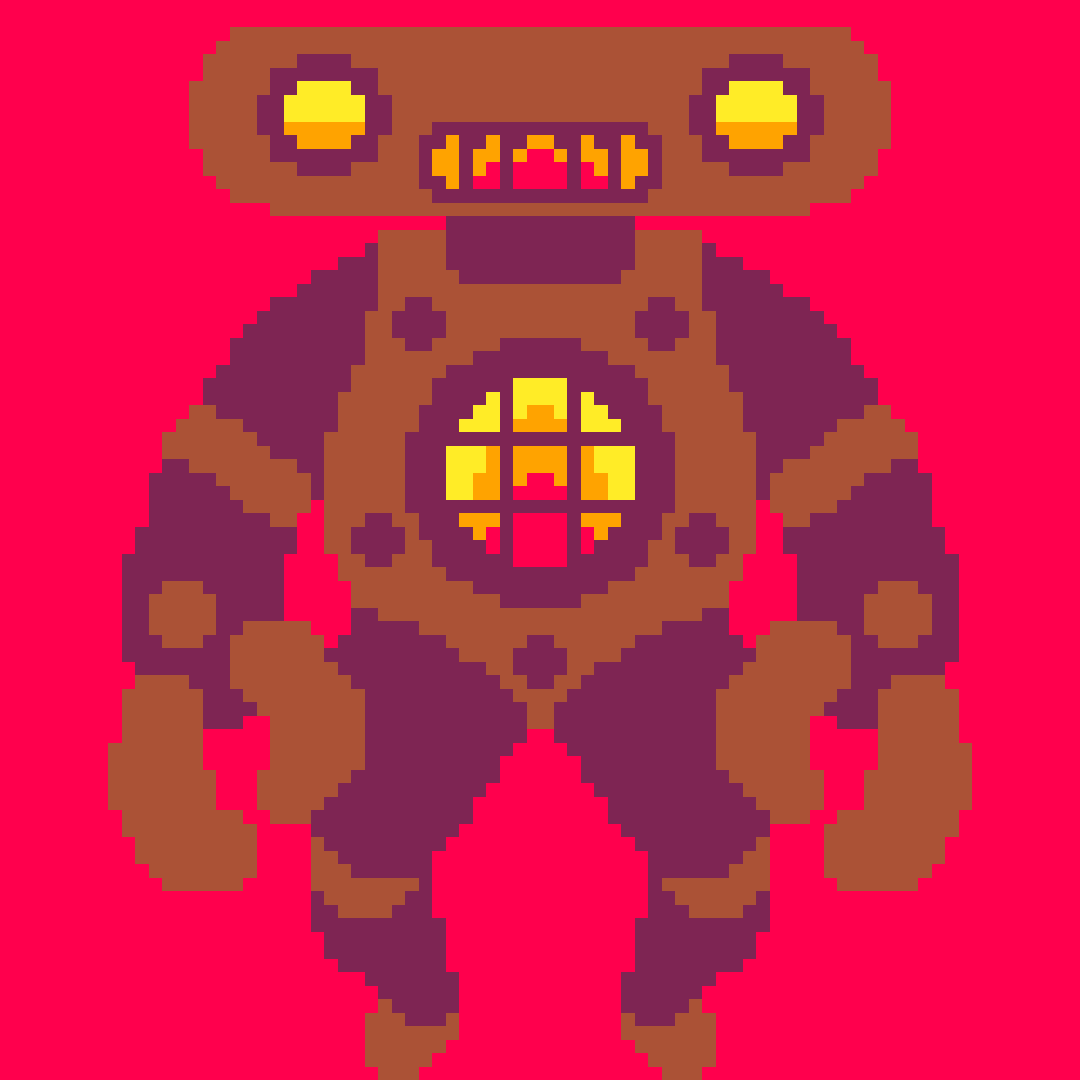

Hey there,
according from the Wiki-Page about Memory, it is unclear what a Poke to address 0x5f5e does, right?
0x5f5e-0x5f7f / 24414-24447 The remaining registers up to 0x5f7f are undocumented. |
I was playing around a bit and found something out: It seems to "reduce" the Colors that can be used. For example, poking a "1" reduce the Palette to only 2 Colors, Black and Dark-Blue.
A simple Description: It seems that poking around 0x5f5e is some sort of "pal" all over the Color-Palette, but also prevents you to "pal" another Color. For Example: If you poke a "1", "pal" is limited to set a Color to blue or black only.
I just uploaded a Cartridge where you could play around with that:





Hello there,
(English isn't my first Language, I hope it doesn't sound too bad, Sorry^^)
I was looking for a easy Solution to "modify" the Linux in my GPi Case to boot directly into PICO8. I don't want to use something like Retropie/Recalbox/Lakka, just a PICO8-Machine.
There was already a Project called PICOPI, which already assembled that. But it seems this Project doesn't exist anymore (Homepage is down; the Files I could find on archive.org doesn't seem to work anymore).
So I thought I write my own Script for this, which builds upon Raspbian, the Default-Linux-Distribution for Raspberry Pi (which builds upon GPi Case). I thought it could be useful for others, so I uploaded the Script^^.
While using this Script, you will be able to put your .p8-Files directly on the "boot"-Partition on the SD Card.
Script can be found on Github, including a Manual for using it:
https://github.com/Astorek86/Pico8-Script-for-GPi-Case
EDIT: I also uploaded a Video on Youtube to show the Process:
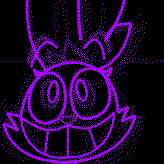
Hey everybody! Just sharing a little Minesweeper-Clone that I've programmed^^.
New Version:
Mouse is not required anymore, but you can use the Cartridge below if you wanna use the Mouse.
Controls for "Non-Mouse-Version":
- Arrow-Keys to move Cursor.
- Press and release X to open a Tile.
- Press and release O to mark a Tile (red or blue Flag).
- Hold X or O + Arrow-Keys to scroll.
And here the "Mouse-Version" of the Game:
IMPORTANT: Keep in mind that the "Mouse-Version" can be quite unplayable on Browsers. This Version of the Game runs best directly on PC, started by PICO8.
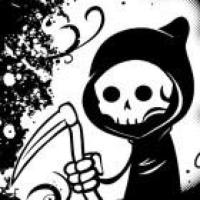
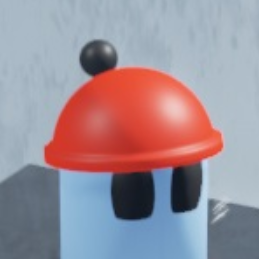
A little bit easier with less enemies:
Submit for the One Hour Game Jam 167,
http://onehourgamejam.com
Theme: That's not supposed to be a weapon.