I'm working on a top down game and I was experimenting with ways to stop the cobblestoning/staircasing effect when moving in diagonals, you know, that jitter that occurs when you're not moving at exactly 1 pixel increments diagonally.
I tested a couple of great solutions already out of there (for instance the one lazydevs showed on his shmup tutorial series), but I felt that something different would work better for me.
Basically, it works by making sure you're always moving at whole pixel increments. There's two buffers used to change the x and y coordinates on the screen. The buffers increment towards the integer nearest to the character speed, and movement only happens when that whole number target is reached.
This a simple program to test the buttons from your keyboard and gamepad. I built it as a reference, so I can know where each button (especially the action buttons) is on the gamepad. The other programs I tested weren't aligned to my xbox styled gamepad.
Since it's a windowed program, it can be installed as a widget.
Picotron always registers keyboard as player 0, and gamepad as player 1.
Even when I boot picotron with the gamepad already plugged in, it gets assigned as if it were a second player (player 1), instead of mirroring the input of the first player (player 0).
Is that intended?
To work around this, I've been using this to check for both players when handling my input checks:
if btnp(0, 0) or btnp(0, 1) then -- do whatever I need end |
I'm working on a desktop app for Picotron and I need to detect when the AltGr key is being pressed. I tried
if keyp("altgr") then
but it doesn't work. I've also tried
if key("alt") and key("ctrl") then
and its variants (like "lctrl" or "rctrl") but it also doesn't detect when I press altgr on my keyboard.
Thanks for any help!

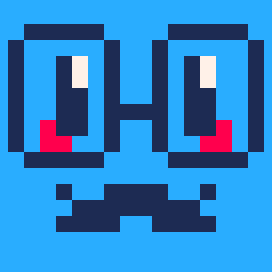
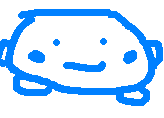
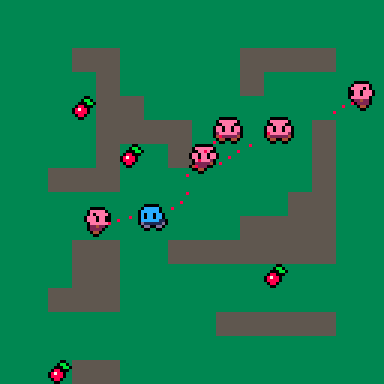
This is an example of how to do enemies that somewhat follow and try to seek the player.
It works like this:
- each enemy shoots sensors towards the player.
- if a sensor hits a wall, it gets deleted.
- if a sensor exceeds a set amount of time, it gets deleted.
- if a sensor hits the player, a position gets marked as a target for the enemy that emitted that sensor.
- enemies will move to their target positions.
- enemies will roam randomly when the player hasn't been detected.
- enemies have a fov: sensors are emitted only if they are inside the range of this fov.
- if the player gets too close to enemies, they will detect the player, regardless of their fov.
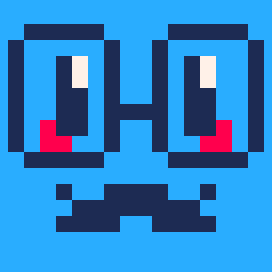

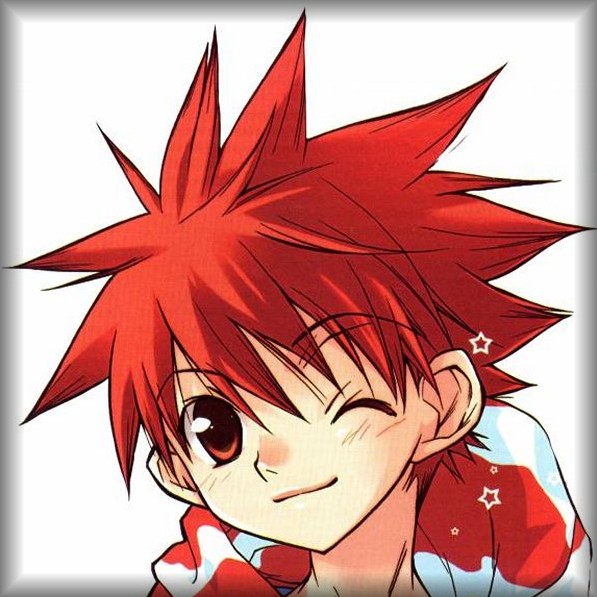
Controls!
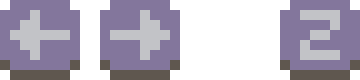
Arrows to move, Z to confirm, Enter for menu.
How to play!
Don't crash into turtles and avoid starfish and sea urchins!
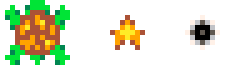
Collect hearts for extra lives!

To score points, stay behind turtles for as long as you can, but don't forget to dodge them at the last moment!
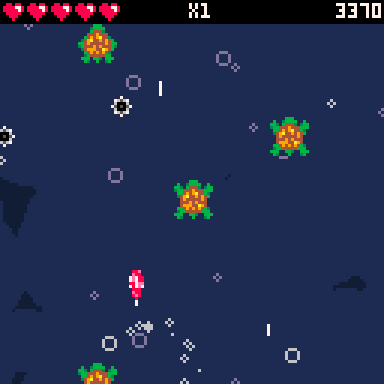
The score multiplier decreases if you are too far away from the next turtle. Keep the multiplier high to score more points!
Are you fast enough to keep up?
Tips and Tricks
You don't need to hold the arrow keys. Just tap the direction of the lane you want to switch to and you'll be moved there automatically.
If your fingers are fast enough, it is possible to stay in the space between lanes for a short while. That will help you avoid seemingly impossible obstacles:
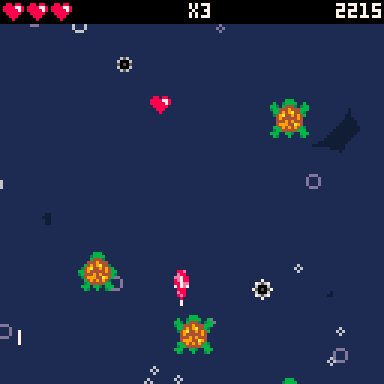
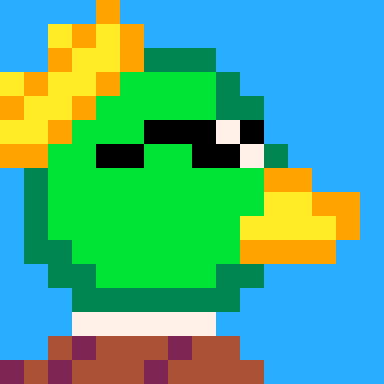
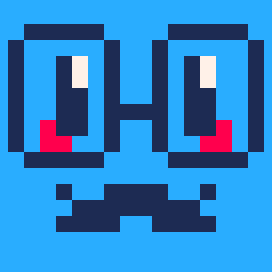

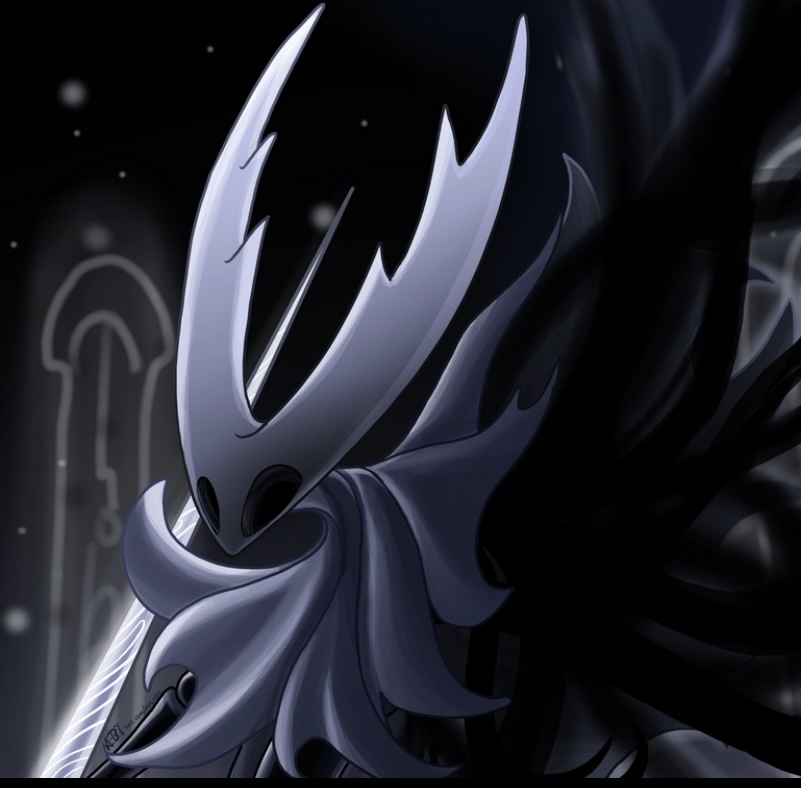
In TIC-80, you can type on the command prompt "help" followed by the name of any built in function and the console will then output a short explanation about how to use that function. It would be nice to have something like this in Pico-8 as well. For example, you type in the command prompt:
help spr
and then Pico-8 outputs a short explanation of how spr works, followed by the parameters it takes.
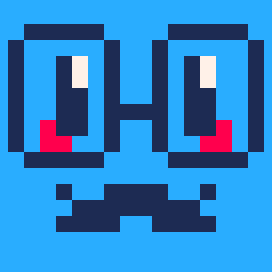
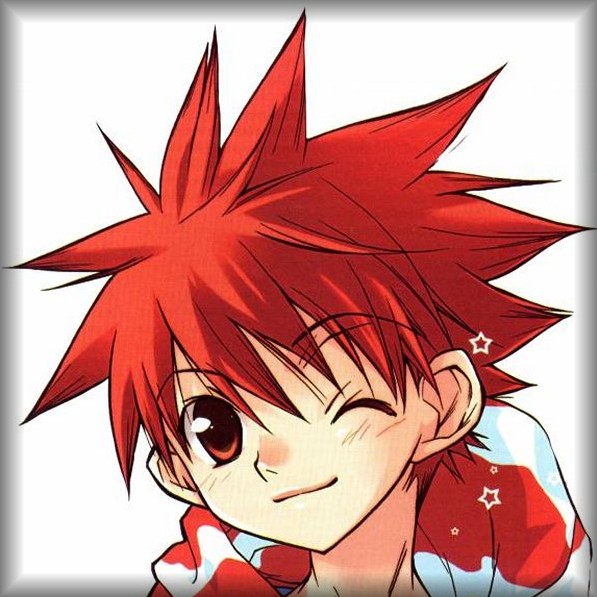
I release this under the CC0 1.0 license: https://creativecommons.org/publicdomain/zero/1.0/
This a simple collision system I wrote for fun. It can handle hit boxes with varying sizes and with different amounts of reference/sample points. It can handle collisions using tiles or used x/y positions from objects.
Also, in this example there's code for an 8 way movement system with acceleration and sliding along walls.
The code is VERY commented, so you can follow along and see what each part of it is doing/why it's there.
Feel free to use this in any sort of project.
If this was useful to you, consider buying me a coffee: https://ko-fi.com/profpatonildo70266
I'm running the Linux version of Pico-8
The load command works with some carts but doesn't work with others. The only output from the pico-8 console is "could not load". If I launch splore, then I can launch all carts from splore.
I just recently reinstalled my system and the problem started after that.
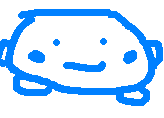
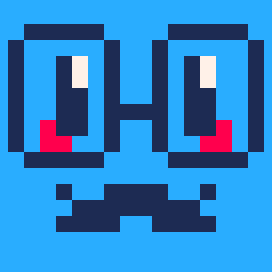
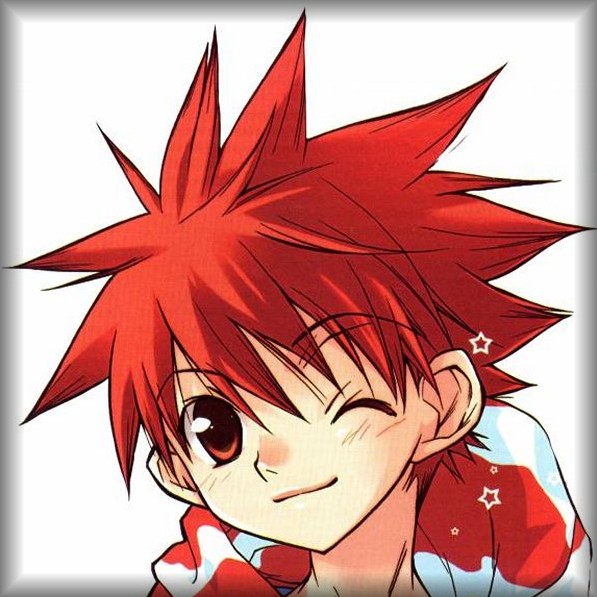
Simple input system, to manage key/button presses.
Registers pressed and release buttons.
Horizontal axis, vertical axis, O and X buttons are all independent of each other.
The code is commented, so you can follow along what it is doing (and possibly improve it, if you wish).
As is, it is good enough and does the job, but I wouldn't be surprised if there is a more efficient way of doing this.
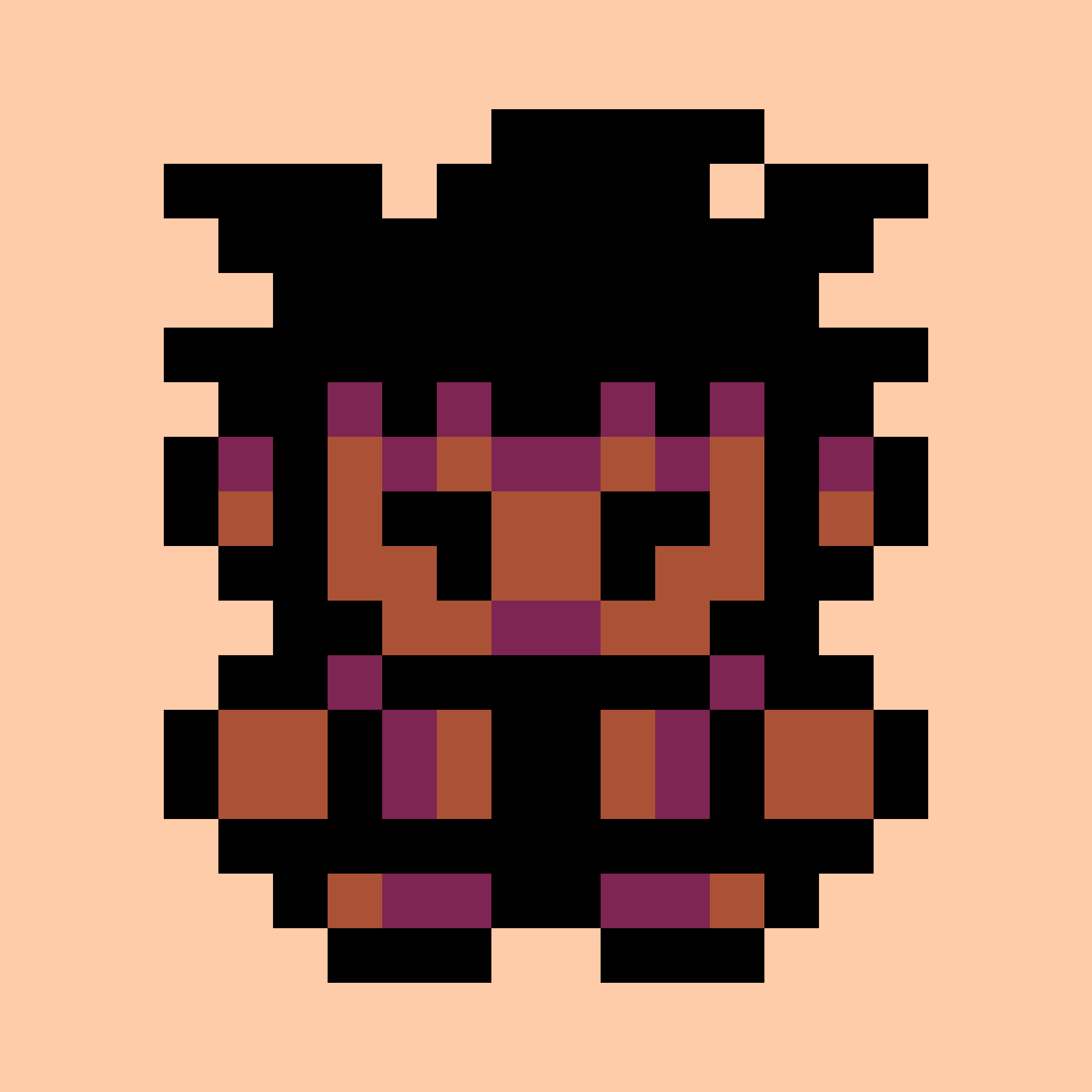


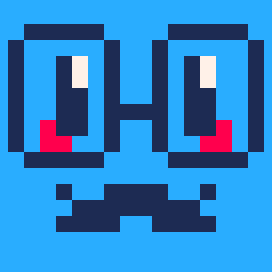
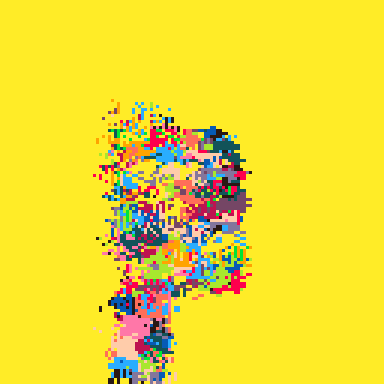
Working in Pico-8 is pure delight. I think it would be even nicer if we had the option to set Pico-8 to play a brief sound analogous to a floppy disk drive when loading a program/cart.
Completely superfluous, but I would be very glad to have this option built in (even if it weren't the default and I had to enable it through a config file or menu).
What do you think?

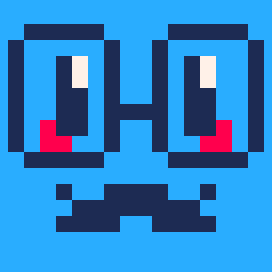
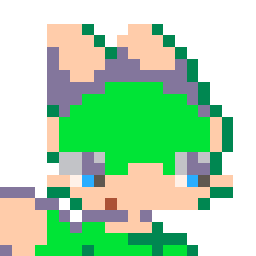
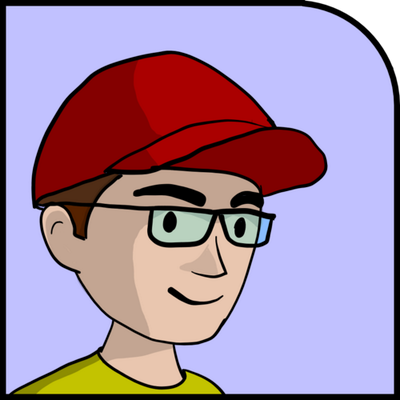


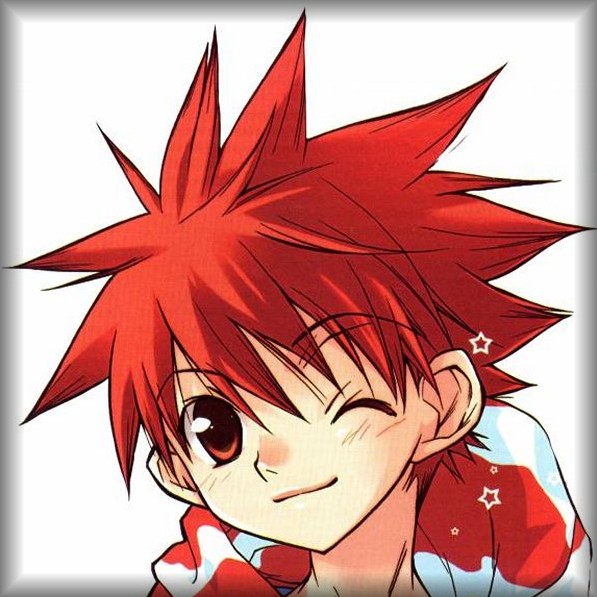

Just a simple vignette fade effect (like on loony tunes cartoons, that circular fade in/out).
Use X to open the vignette, Z to close it.
Arrow keys move the smiley face.
Can also be used to create a fake flashlight effect.
The code is very simple and commented, so it can be easily modified/repurposed.
The vignette works with a sprite that you can customize (to create other shapes).
Mine uses 4 sprite slots on the sprite sheet, but you could easily make it work with only one if you really need to save sprites. Just remember to also customize the draw_vignette function to make it work with different sized sprites.

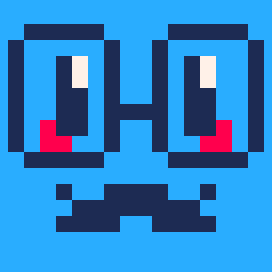
.jpg)
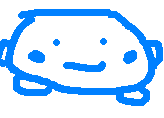
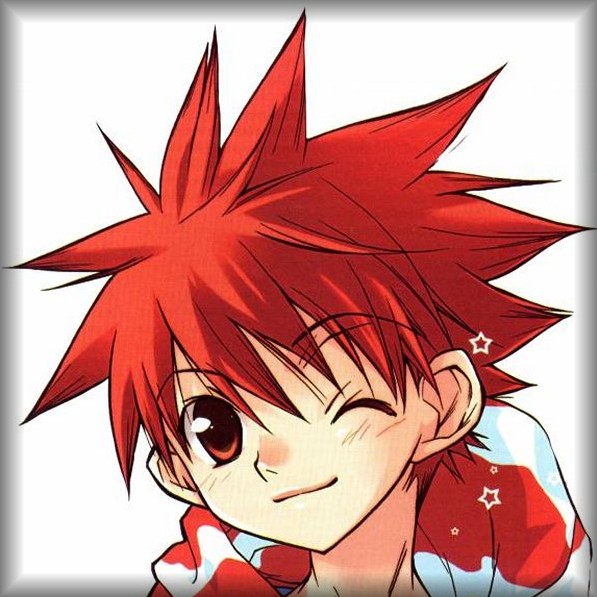
"It's a gory version of the Mario Paint game with a chill sound track. Very hip."
u/TheRedBeed from r/pico8
Smash bugs with Z, move with arrow keys.
To start the game, smash the bug!
Player 2 can join and play at anytime. The game gets slightly more unforgiving with a second player, to compensate.
You can hold down the buttons to move faster and to smash repeatedly or you can tap them to be more precise.
Smashing multiple bugs in a streak without missing gives you bonus points, but it takes more time.
Be it button mashing or attacking with precision, get rid of all the bugs!
Coins give you some points.
Clocks give you some points and more time.

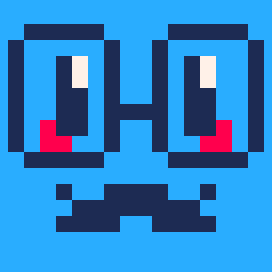
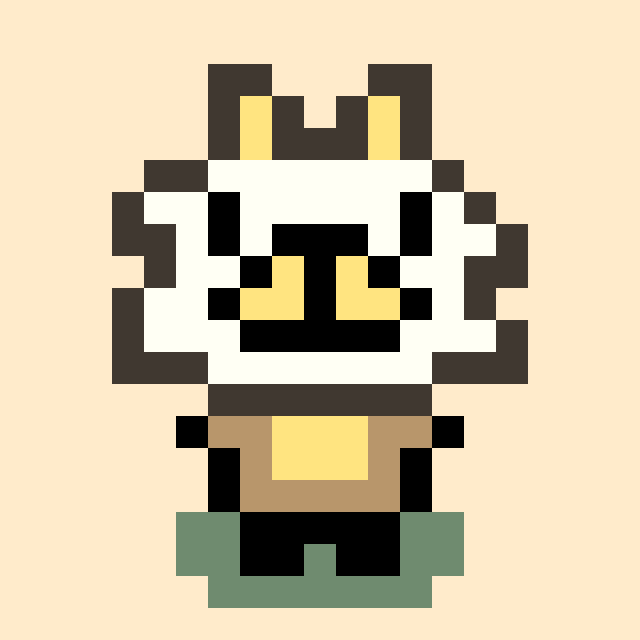