UPDATE: Now with mouse support. Enable from the pause menu.
A cozy flight sim lite for PICO-8.
Become a pilot for Pico Air, a small air company operating between the islands of Pico Isles.
Perform deliveries, charter flights, crop dusting and other jobs to unlock new planes.
Features:
- A 16km x 16km map to explore
- 3 different planes
- External camera views
- Photo mode
Controls
- Arrows - Ailerons and elevator (roll and pitch)
- O + Up/Down - Throttle
- O + Left/Right - Rudder
- X + Up/Down - Adjust elevator trim
- X + Left/Right - Flaps
Or use player 2 controls for throttle and rudder.
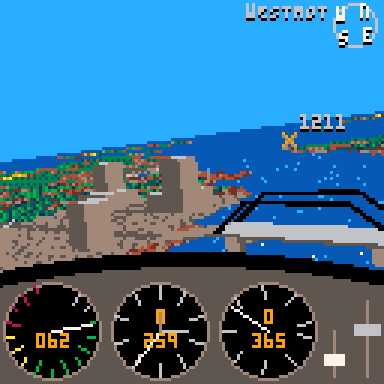


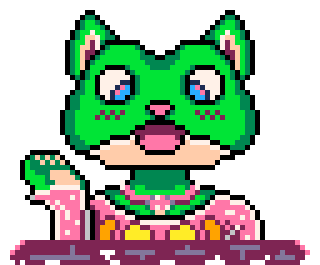

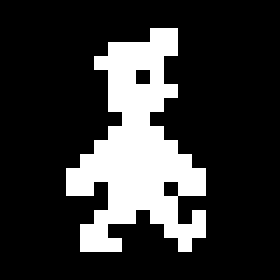

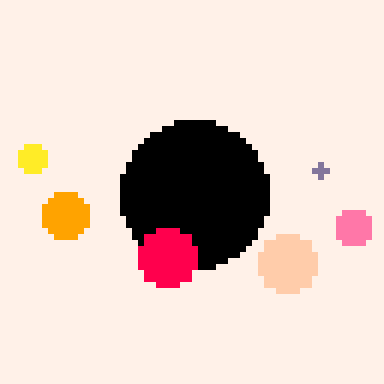

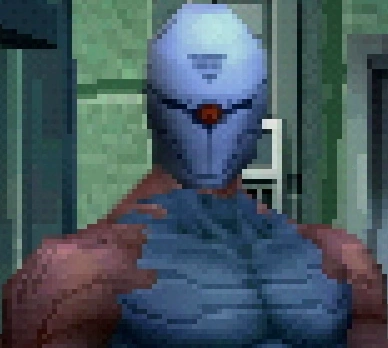

Just a little snippet I use when looking sfx that haven't been used in the pattern editor.
p,s={},"" for a=0x3100,0x31ff do p[@a]=true end for i=0,63 do if not p[i] then s..=i.." " end end print(s) |
Paste into the terminal and hit enter.
WARNING: This only shows sfx that are unused in any pattern. You are responsible for checking whether they are:
- Used as sfx in the program
- Used as instruments by another sfx (i.e. for sfx 0 to 7)
- Used in some other way I haven't thought of

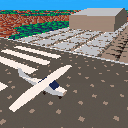
This is a work-in-progress flight simulator I've been working on.
The flight model is mostly implemented, but may need some tweaking. And I'm still to implement crashing into buildings and some sort of map or navigation aid.
I've never flown a plane, and only dabbled in flight sims, so I would really appreciate some feedback from anyone with more experience than me, so I can try to tweak the flight model parameters to be a bit more realistic.
The plane is based on the Cessna 172.
Controls
- Arrows - ailerons/elevators
- Player 2 up/down (E,D) - throttle
- Player 2 left/right (S,F) - rudder
While holding down X
- Up/down - adjust trim
- Left/right - adjust flaps
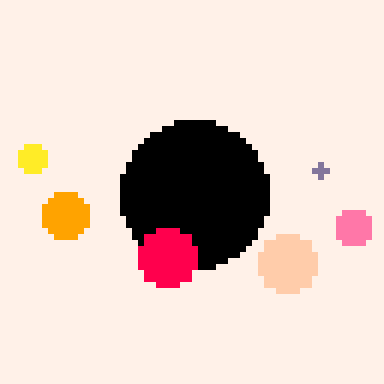
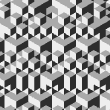


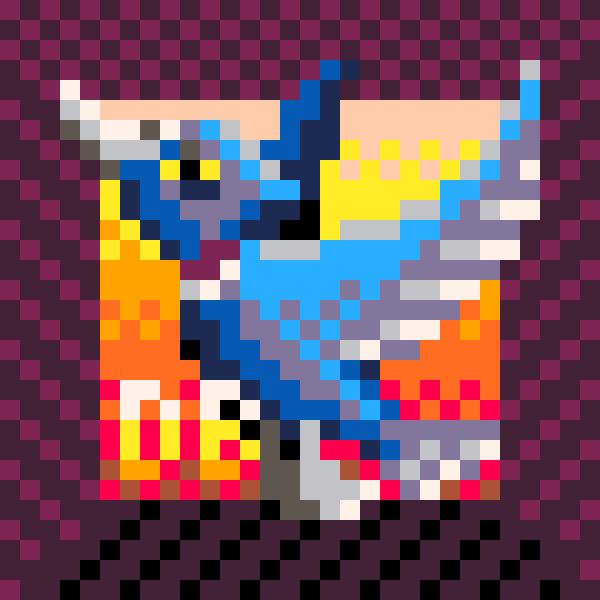
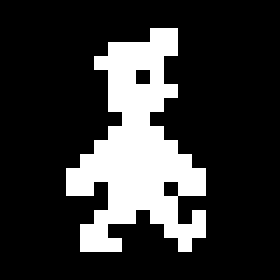

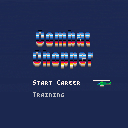
This is an update of Combat Chopper to improve the resolution of the ground, at least close to the camera, using an enhanced version of the Whiplash Taxi Co ground drawing code.
See the original game for instructions on how to play.
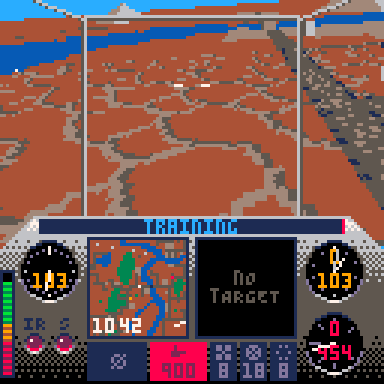
I had to sacrifice a few things in order to squeeze the ground drawing code in, particularly the heads-up artificial horizon, and the targeting camera effects, and the menu is now in a separate cart. Also it drops down to 15 fps a bit more than I'd like, but it feels pretty playable regardless.
Update: Bug fixes
Here's a little line drawing routine I've used for Whiplash Taxi and some other work-in-progress carts.
I wanted to package it up like a drop in tline
replacement.
It's equivalent to regular tline
, but instead of drawing each tile it uses it to lookup a higher resolution 8x8 tile region and draws that instead. The result is a map with 8 times the detail (or 8 times the size, depending on how you look at it).
The demo is a simple go-cart example. You can press X to toggle between the new routine and the regular tline
function.
The actual routine is in tab 1 and consumes 547 tokens.
To use it, you first create some 8x8 "textures" at the top left of your map region:


Here's a medieval procedural world generator I'm working on. It has a few different biomes, buildings and roads.
I'm thinking of turning it into some sort of Trial of the Sorcerer sequel.
Instructions
Give it a minute to generate the world, then open the pause menu and choose "explore world" to explore it in ray cast 3D.
There's no gameplay yet, just walk around and explore.
In the generator you can also use the direction pad to scroll around, and zoom in/out with X and O.

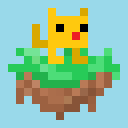
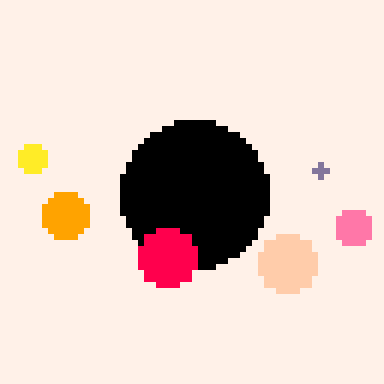

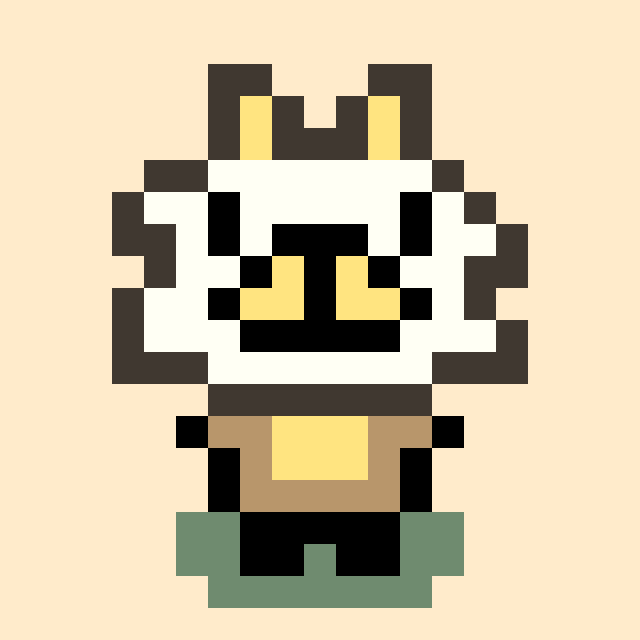


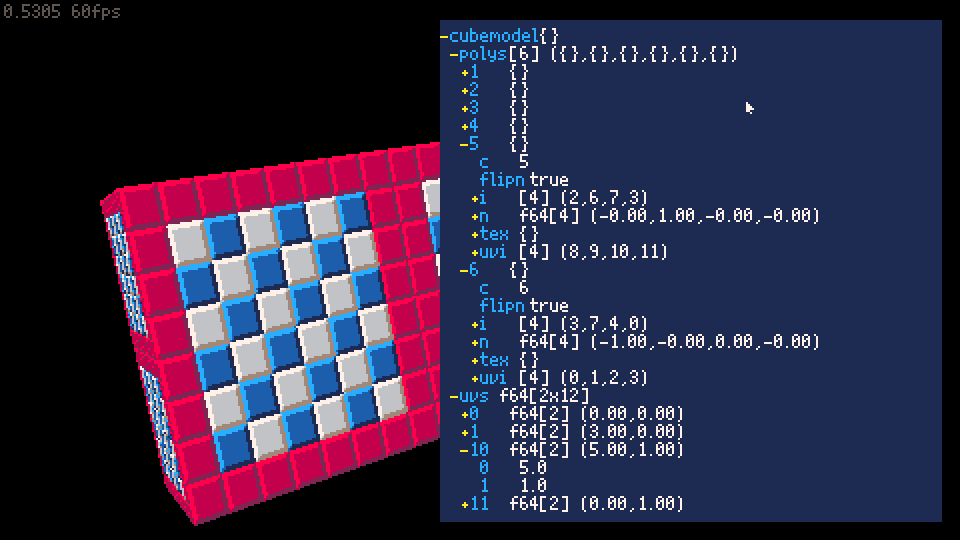
A quick port of my pico-8 variable inspector, I find useful for debugging.
It has a few more colours for readability, and can display userdata.
To use, hit Esc to break out of your program and enter:
dbg(v) |
Where v
is the value to inspect.
You can drill into values by clicking the "+" (or collapse them again by clicking "-").
Mouse wheel to scroll up and down.
Press Esc when done.
-- debug inspector -- lines to display and scroll offset local dbg_lines,dbg_s={},0,false -- add item to display function dadd(n,v,i,p) -- insertion sort in name order while p>1 and dbg_lines[p-1].i==i and type(dbg_lines[p-1].n)==type(n) and dbg_lines[p-1].n>n do p-=1 end add(dbg_lines,{n=n,v=v,x=(type(v)=="table" or type(v)=="userdata") and "+" or " ",i=i},p) [ [size=16][color=#ffaabb] [ Continue Reading.. ] [/color][/size] ](/bbs/?pid=156711#p) |


I've noticed the min
and max
functions have a surprisingly high CPU cost.
For example
a=stat(1) for i=1,1000 do b=min(1,2) end print(stat(1)-a) |
Outputs:0.103
Which is almost twice the CPU cost for sqrt()
a=stat(1) for i=1,1000 do b=sqrt(2) end print(stat(1)-a) |
Outputs:0.054
And even if I define my own "min" function in lua, it's still a lot faster.
function mymin(a,b) return a<b and a or b end a=stat(1) for i=1,1000 do b=mymin(1,2) end print(stat(1)-a) |
Outputs:0.057
Is this a mistake?
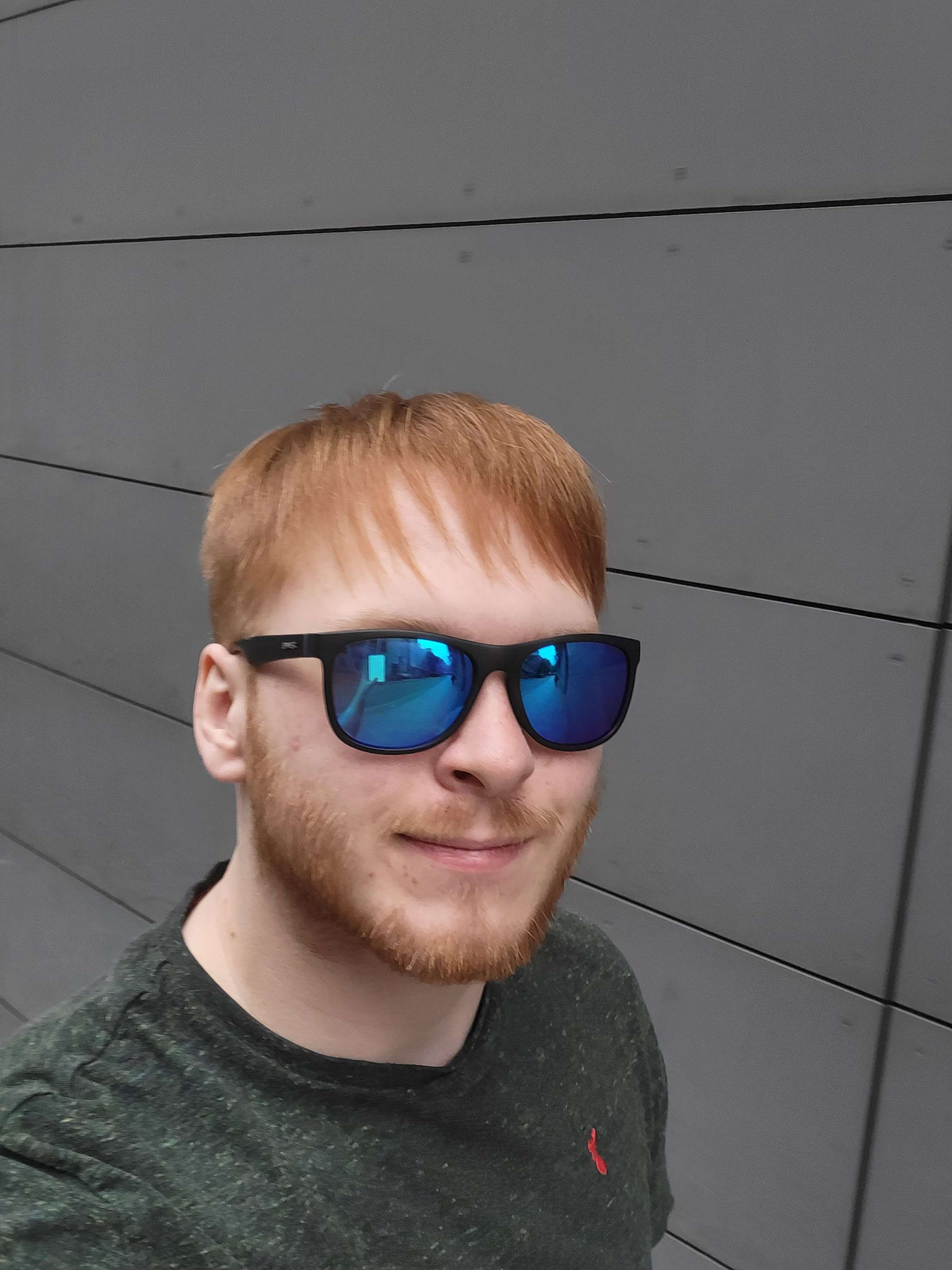

Here's a basic CSV parser I use to save tokens in my games. It uses split
for the heavy lifting.
Basic version
The most basic version is 66 tokens.
function data(str) local lines=split(str,"\n") local props,d=split(deli(lines,1)),{} for l in all(lines) do local o,v={},split(l) for i=1,#v do o[props[i]]=v[i] end if(o.name)d[o.name]=o add(d,o) end return d end |
It can be used like this (4 tokens):
spritetypes=data [[name,sx,sy,sw,sh alien,0,0,2,2 boss,4,0,4,4 player,0,2,4,2 bomb1,3,0,1,1 bomb2,2,1,1,1 bullet,2,0,1,1]] |
The data
function returns an array of objects, with properties that map to the CSV columns.
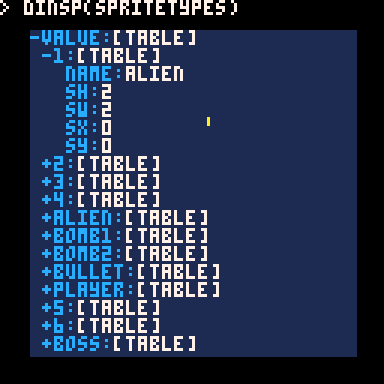
If objects have a name
property, then they will be added as a property to the main object with that name.
Which means we can reference the "player" sprite type as:
print(spritetypes.player.sx) |
References
This version allows you to reference objects defined in other CSV tables. It's 117 tokens.
glob={ ["true"]=true, [ [size=16][color=#ffaabb] [ Continue Reading.. ] [/color][/size] ](/bbs/?pid=155181#p) |
I thought I'd upload this because I doubt I'll be able to finish it - at least as a Pico-8 game. Basically it was too ambitious and I ran out of space. Maybe I'll port it to Picotron someday, but for now it's basically a tech demo.
You wake up in an android body in a factory in a mining town on Saturn's largest moon, Titan. You have to solve the mystery of who you are and what happened, and maybe how you can put things right.
Or at least you would if the game was finished. For now there's a little puzzle about exiting the factory, and some dialog sequences. After that there's a car to drive, a slightly glitchy plane to fly and some empty buildings you can look inside.
It's intended to be a game about exploration and dialog.
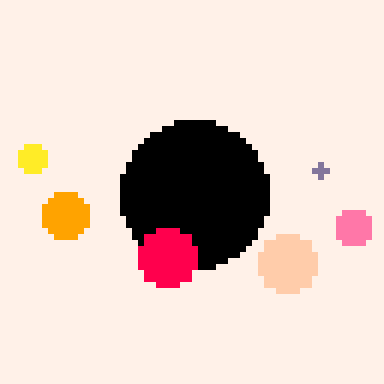


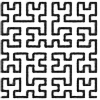
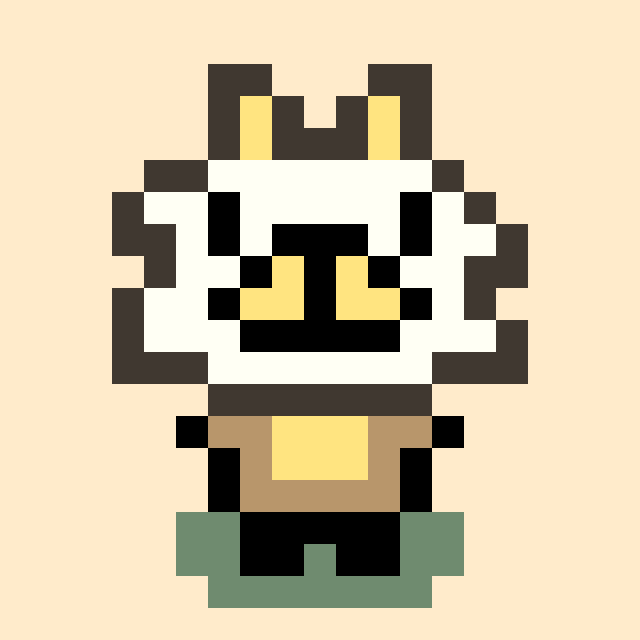

ChatGPT 4o seems pretty good at writing Pico-8 code. (At least for simple games).
I asked it:
"Can we try something different? Like a simple Space Invaders or Galaxians style game?
I have 4 sprites in slots 1,2,3,4 with a player ship, alien invader, player bullet and enemy bullet respectively. Could you make a simple game where the aliens move left and right in formation, and the player moves left to right along the bottom of the screen?"
I got this.
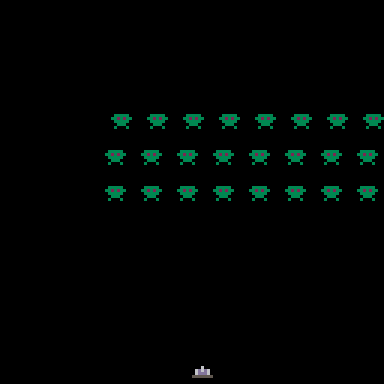
I pasted the gif into the chat window, and it said:
"Awesome! It looks like the basic mechanics are working. Let's add a few more features to make the game more interesting:
Enemy Bullets: Add bullets fired by the aliens.
Player Lives: Track the player's lives and end the game when they run out.
Score: Add a scoring system for each alien destroyed."
Then gave me an updated version that looks like this:
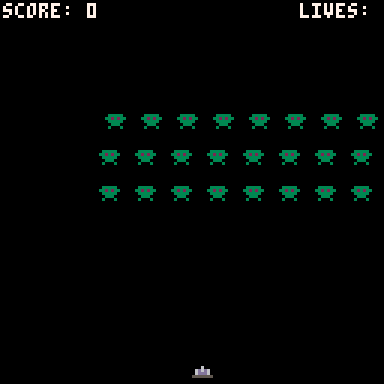
Then I got worried that AI is doing all the work and I'm going to turn into the spaceship inhabitants in WAL-E. So I asked it to quiz me on the code and check my answers.

.jpg)


Here's a little profiler you can drop into an existing program - if you have 313 tokens to spare.
It instruments the global functions to count the number of calls and measure the CPU time spent inside them. Then it dumps it to a CSV file you can open in a spreadsheet viewer, and hopefully get some insight into where your program is spending the most time.
Instructions are in the comments at the top.
EDIT:
- Count fn calls in a single 32bit integer (thanks @freds72).
- Use "..." arguments syntax to support more than 9 arguments (thanks @Siapran)
- Save uninstrumented fns to avoid calling the instrumented ones when dumping stats.
-->8 -- profiler -- usage: -- run your program -- press esc to break -- > _prof.begin() -- > resume -- run your program some more (to gather data) -- press esc to break -- > _prof.dump() -- this will dump a profile.csv file to the cart's folder -- notes: -- profiling for more than 2-3 minutes could cause cpu measurements [ [size=16][color=#ffaabb] [ Continue Reading.. ] [/color][/size] ](/bbs/?pid=142589#p) |

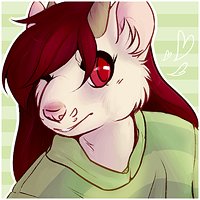
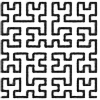

This has been sitting on my drive for a year or so. I was meaning to write up some documentation for it, but I'm throwing it up as is for now. (Until I can remember how to use it.)
Basically it's a sequel to Mot's Animation System built on top of the 2D transforms library. The main improvement being that it supports rotation.
The basic idea is you create "sprite" animations based on frames on your tilemap. You can then combine them into "timeline" animations where position, rotation etc can be set at different points by creating keyframes.
There's also a "storyboard" animation type that simply plays other animations in order.
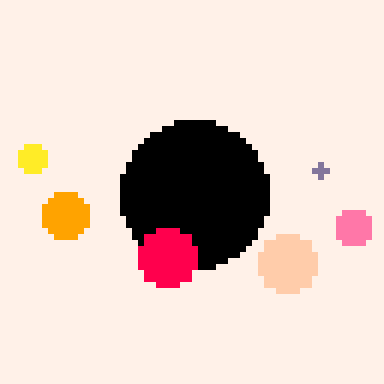
UPDATE: Fixed suspension to lean towards the outside of turns, not the inside.
Welcome to the Whiplash Taxi Company, where our motto is "The slower you're there, the lower the fare!"
As a valued employee and taxi driver, your job is to pick up passengers and deliver them to their destination as fast as possible, by any means necessary.
Passengers are easy to spot, just look for the big red arrows over their heads.
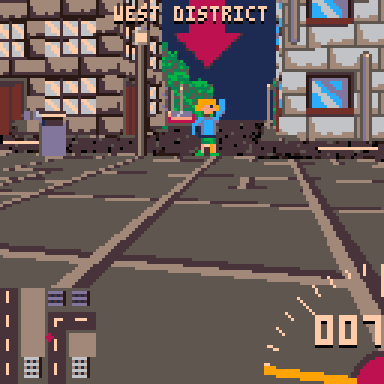
Simply stop next to them so they can jump in!
Then follow the red destination indicator to the destination and stop when it says "stop!".
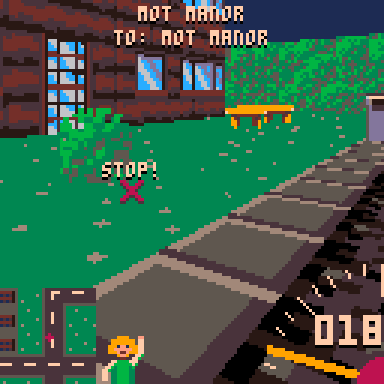


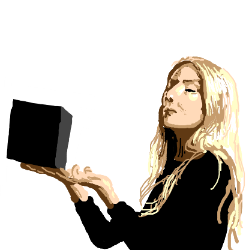


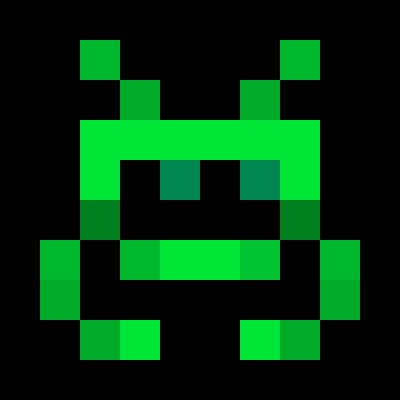
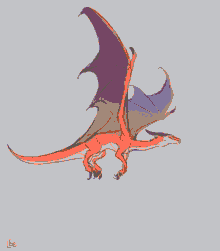

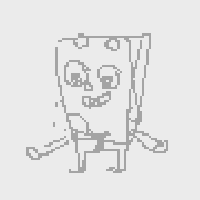

This is basically a Wolfenstein-3D style ray-caster with floor tiles.
There's no gameplay or even collision detection so far.
The top right of the Pico-8 map region is used to layout the game, using the sprites in tab 3.
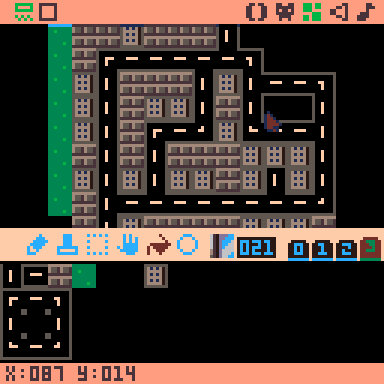
The sprites correspond to 8x8 regions in the top left of the map area, which represent the "texture" to draw.
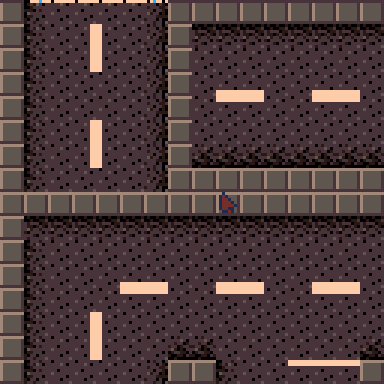
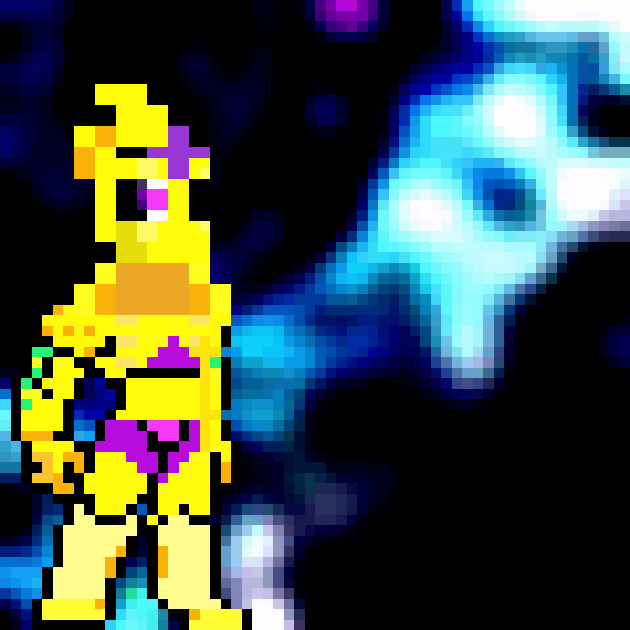

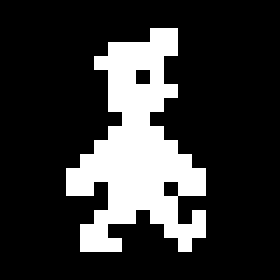
WARNING: This game attempts to emulate old 8-bit flight simulators from the 80s and 90s. The flight model leans towards realism rather than easy-to-pickup arcade gameplay (even if it's somewhat primitive compared to serious modern-day flight simulators). As such it's not an easy to pickup game - controlling the helicopter is challenging and takes practice. In combat, even more so.
Welcome to Combat Chopper, an 80s helicopter combat flight simulator for Pico-8!
Pilot your P8 64k Apachi armored attack helicopter on a series of missions destroying key targets in enemy territory.
Getting started
Training mode
Use the "Training" mode to practice controlling the helicopter before trying to take on any missions. You will need to be comfortable with keeping low, stopping and hovering, and landing, before attempting to engage in combat.




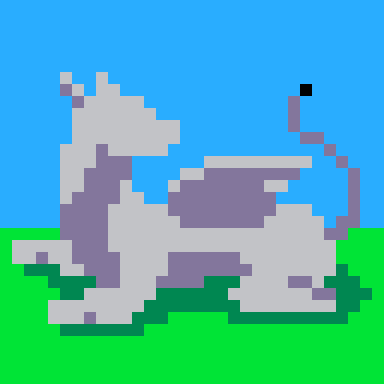

Update: Updated cart to latest version. This is probably close to what will be released.
A work-in-progress helicopter combat flight simulation. Loosely based on my memories of playing Gunship on C64 a few decades ago.
The heli can be controlled with player 1 input:
- By default the arrow keys control the cyclic stick.
- Hold down circle (z) to switch to collective (up/down) and tail rotor (left/right).
- Hold down cross (x) for weapons mode, where up/down cycles between targets, and left/right selects the weapon.
- Tap cross (x) quickly to fire.
So to take off, hold down circle (z) and up.
Alternatively you can use player 2 input (E,S,D,F in keyboard) for collective and tail rotor.
