After a long journey, I'm proud to present to you...
parens-8 v3!
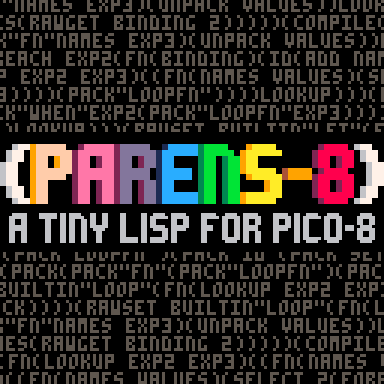
...Along with the obligatory demo, a cart that stores its entire game code in ROM:
Parens-8 is a tool for bypassing the pico-8 token limit. It takes up 5% of the allowed 8192 tokens, and gives you practically infinite code space in return: store extra code in strings or cart ROM, load it during init, run it like regular Lua code.
Parens-8 is designed for maximum interoperability with Lua. Functions, tables, values and coroutines can be passed and used seamlessly between Lua and parens-8. Think of parens-8 as Lua semantics with Lisp syntax.
[ [size=16][color=#ffaabb] [ Continue Reading.. ] [/color][/size] ](/bbs/?pid=142700#p) |

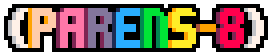
first off, a demo. here's a cart that stores the entirety of its game logic in its sprite sheet:
this is a parens-8 port of an old cart of mine. it has the lua code for the parens-8 language, and then parens-8 code for loading parens-8 game logic from ROM. you can find the full source for the game logic here
parens-8 is a lisp interpreter/compiler designed specifically to bypass the lua token limit.
the idea: use a portion of your cart (between 330 and 900 tokens, depending on your use case) to store the parens-8 interpreter, offload performance noncritical code into strings, and then optionally into ROM.
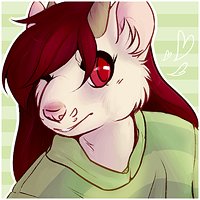

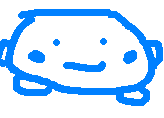
Here's a small cart I made to teach programming to a friend.
It's far from complete, and I'll post more updates as they make progress.
I restrained myself from using lua tricks like coroutines, metatables, and abusive oop, so the code could be kept as much approachable as possible.
Direction keys to move, C/Z to cycle lifes.



Organ is a simple sfx organizer.
When I make music with the pico8 tracker, I usually end up with an unreadable mess of samples that have nothing in common.
This utility lets you switch sfx indexes and update the song data accordingly.
You can either specify a filename in the code (still waiting for a usable ls()), or paste the song data of another cart manually.
The data is saved on the editor itself (even when a filename is specified). To save it to your music cart, you'll need to edit it manually (I'll make a better UI in the future).
I may or may not extend this to make a music compression utility :T
A veeery old thing I did in 2013 when I didn't really know how to program yet.
I was bored in math class so I did this thingy on my calculator.
I pretty much copy-pasted the whole thing and applied a few fixes for pico8, but it's really the same old code (ewww...)
At the time, the thing blurted out a frame every 28 seconds. Needless to say I was REALLY bored.
nostalgia link to the original code
A friend of mine already adapted it for Love2D a while ago, with much better results (video).
For fun times, try doing what I first did when I wrote this code: setting elasticity above 1/2.
EDIT: oh, yeah, you can control the camera with the arrow keys and reset the cloth with x
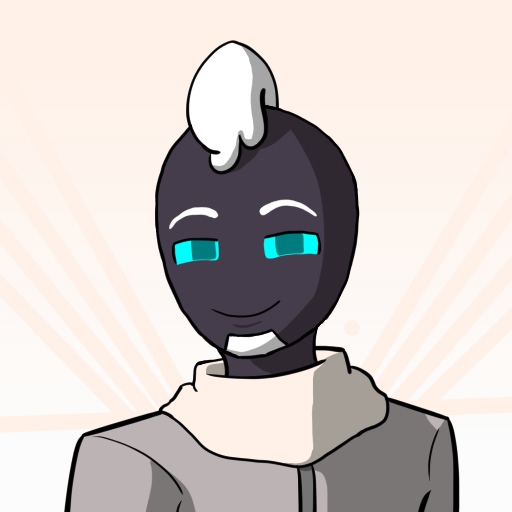

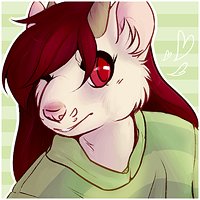
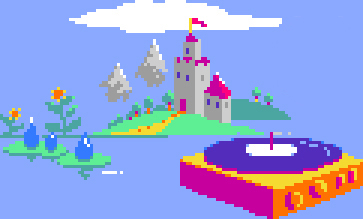


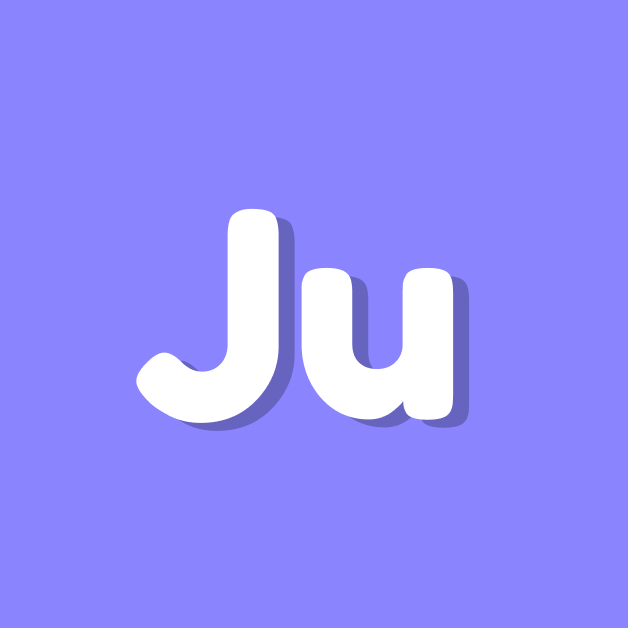
Update: tweaked the cooldown
just a simple demonstration of the undocumented feature of stat(x)
using the demo musics of course
feel free to use it in your music carts :D

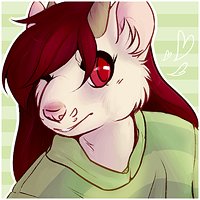
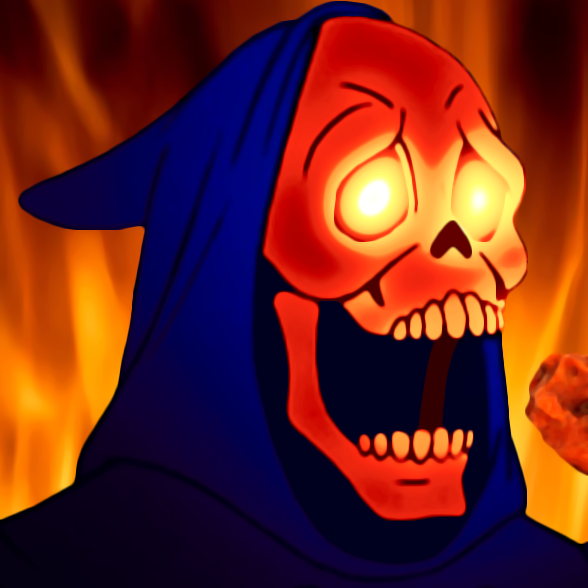