I just found a weird bug where pushing play in the waveform editor seems to corrupt the first note of sfx 63
Steps to reproduce
- click the "waveform" button in any sfx 0-7 and push spacebar to play
- view sfx 63 and see that the first note has now changed to a C2 using the waveform instrument that you were just editing
using version 0.2.6b
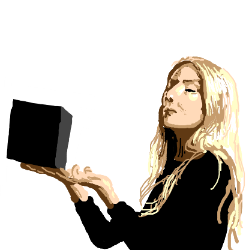
This software product was made for https://itch.io/jam/picostevemo and is based on the 2001 science fiction horror novel Dreamcatcher by American writer Stephen King.
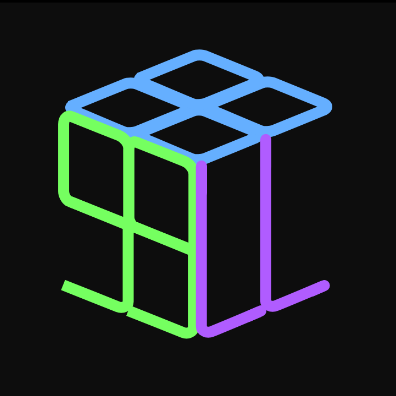
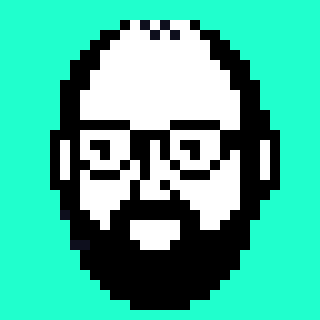

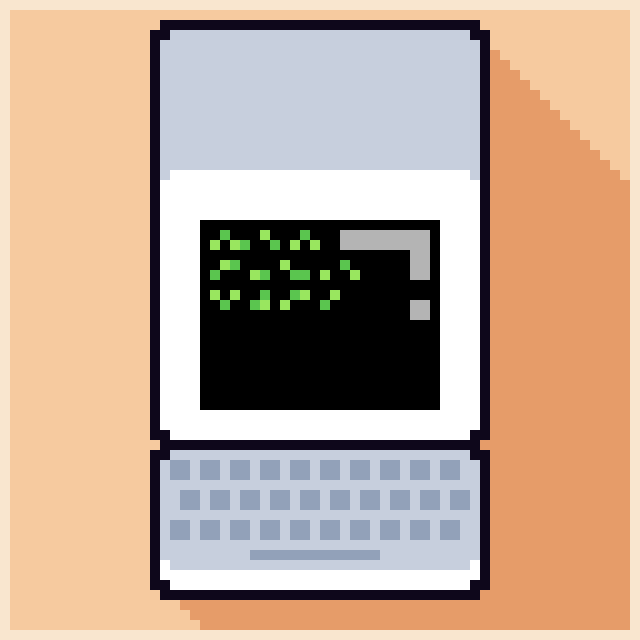
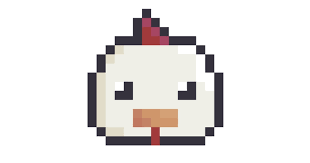
I think table serialization is a pretty well-known token-saving technique but I hadn't seen anyone post one like this that just operates via peek
and poke
rather than using strings, so I thought I'd share my table serializer and deserializer here (originally written for PIZZA PANDA) in case this is useful to anyone.
The serializer writes binary data directly to memory so you can use the output however you want, e.g. you can use the "storing binary data as strings" technique to store it as a string, but you could also just store it anywhere in the cart's data.
The deserializer (the part you need to include in your final cart) is 170 tokens and it similarly reads bytes directly from memory.
The serialized format is pretty efficient and uses data types which are more specific than Lua itself, in order to save storage space; each one of the following is a separate "type":
- 8-bit integer
Straight out of the Bamboo Forest!
Meet PIZZA PANDA, a fluffy little fast-driving bear, as he beeps, bounces, and barrels his way through 20+ levels in search of the lost pizza slices. He'll have to zigzag through city streets to beat the clock, delivering the freshly reconstructed pizzas straight into the mouths of hungry citizens.
But that's not all! Puzzling hats, helpless rats, and meddlesome cats also await PIZZA PANDA and his trusty red automobile. It's non-stop action all the way to the top of the winner's podium!
Controls
- Jump: 🅾️
- Honk/Start Level: ❎
- Move Left/Right: ⬅️/➡️
(open the pause menu to restart a level or return to the overworld)
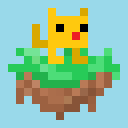

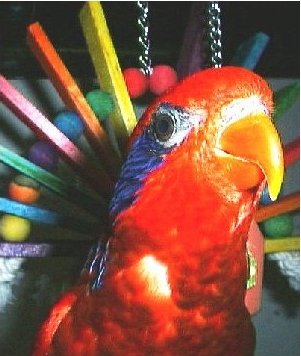
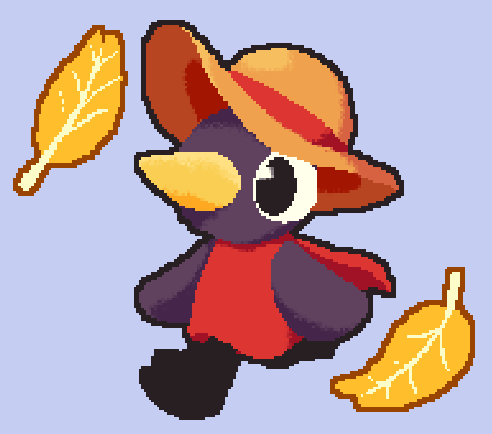

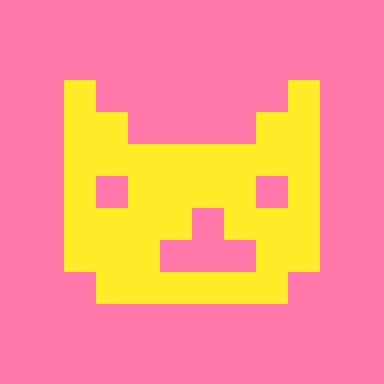

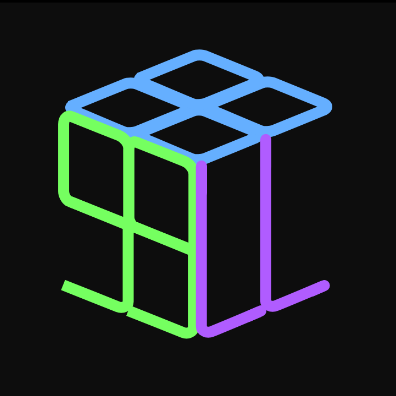
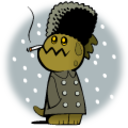
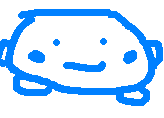

I'm pretty sure I remember this working correctly in a previous version but as of version 0.2.5g if you navigate left/right through patterns in the music editor using the -/= keys on the keyboard, if you get to an empty pattern, the keys no longer work and I haven't been able to find any way to regain control of moving through patterns except by using the mouse.
as of 0.2.2, all menuitems now have their functions called when left/right are pushed while the menuitem is selected?? It took me an embarrassingly long time to realize this... (probably due largely to a bug where the menu doesn't actually close but the items are still triggered, even if the function does not return true which helped to obscure this behavior)
@zep it seems like this change to menuitem's functionality was intended to be extra functionality and only improve the capabilities of menuitems, but the problem is that the new functionality is always activated, even it's not needed or wanted, so if the user accidentally presses left or right (which is very easy to do with a joystick or with the mobile D-pad UI, and this is how I noticed it!) the menuitem's function gets triggered, and the only way to prevent that (i.e. in order to maintain the old behavior where left/right do not trigger the function) is to use up more tokens in the menuitem's function to check which button was pressed!
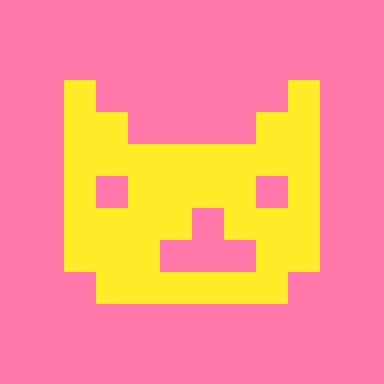
solo un piccolo cart per aiutarmi a imparare
aprire il menu per attivare la modalità "quiz"
bird sprite adapted from @SirTimofFrost's picobirds :>

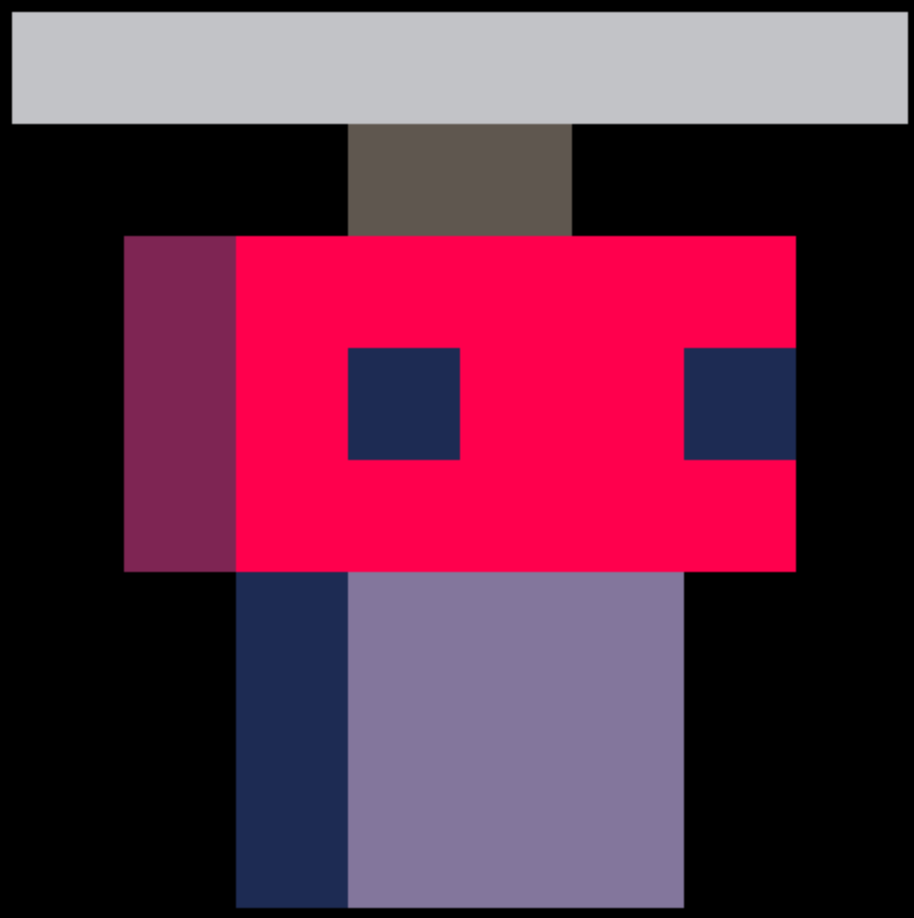
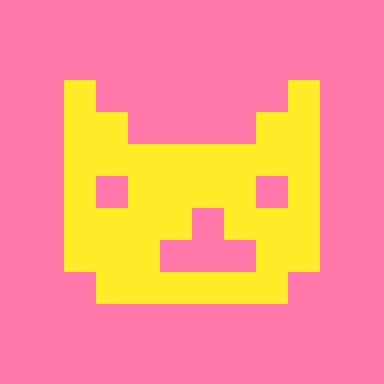
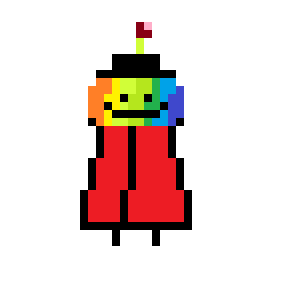
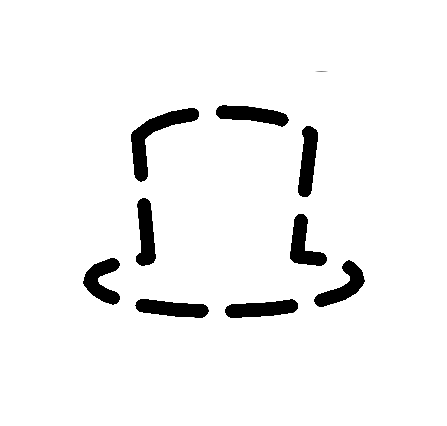
Does anyone know if there is a way to exit early when a print command is running with the \^d
p8scii special command that adds delay frames in between each character?
I'm in token crunch mode and realizing this new special command could be extremely handy for easy RPG-style printing delay with zero code in certain situations, but if there was a way to exit early, it would be even more useful!
And if this is not currently possible, consider it a feature request :p


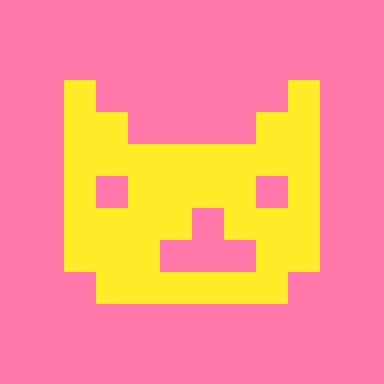
I've been playing a bunch of Cassino lately in real life, so just for fun I thought I'd try making a simple PICO-8 version of it where you play against an AI.
The suits are all just built-in PICO-8 glyphs so note that the stars are spades.
If you've never played Cassino before, it's a really fun two-player card game (it can technically be played with more than 2 players but it doesn't work nearly as well) with an uncommon "fishing" mechanic and I highly recommend trying it with a real human!
TODO:
- multiple rounds with alternating deals until someone reaches 21 points
- SFX
- title screen/better cart label?
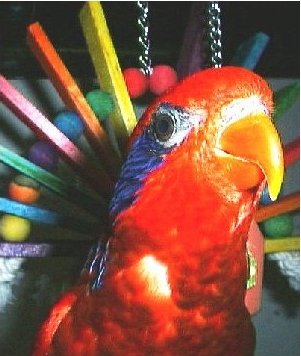

Feature Request:
a config.txt option to allow PICO-8 to respond to gamepad input even when the window is not in focus
I imagine this option would be disabled by default, but it would come in handy for a workflow which is very common for me, wherein both PICO-8 and an external text editor are open side by side (and sometimes also a terminal window for viewing printh output) and I want to be able to control something in the game with my gamepad, but then also be able to type in a different window using my keyboard, without having to switch windows as frequently. Obviously in this out-of-focus situation PICO-8 would have to ignore keyboard input, but still respond to gamepad input.
love,
kittenm4ster
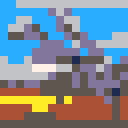
- Run the cart at this link: https://www.lexaloffle.com/bbs/?tid=41062
- Pick up the hen with the web
- Slam into the right side of the screen repeatedly
observe that part of the lines which make up the web are visible on the left (opposite) side of the screen
This happens in v0.2.2 of the BBS player, the native version, and the HTML export from the native version. It does not happen in the previous version.
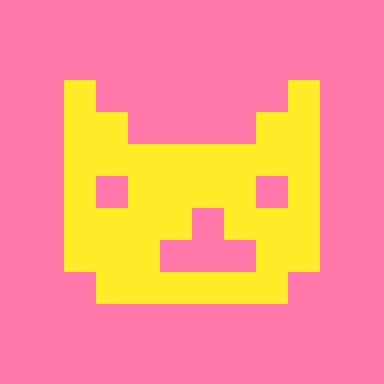
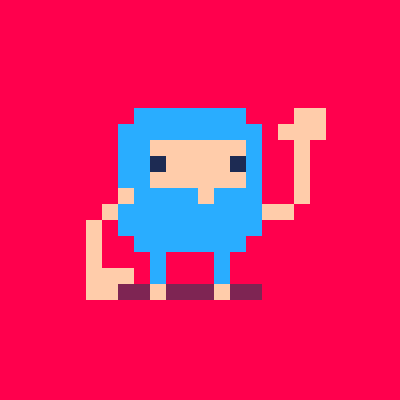
This was made in two weeks for Toy Box Jam 2020, a jam in which we had to make something using only premade graphics/sfx/music assets.

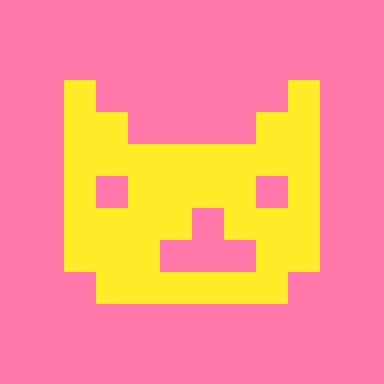

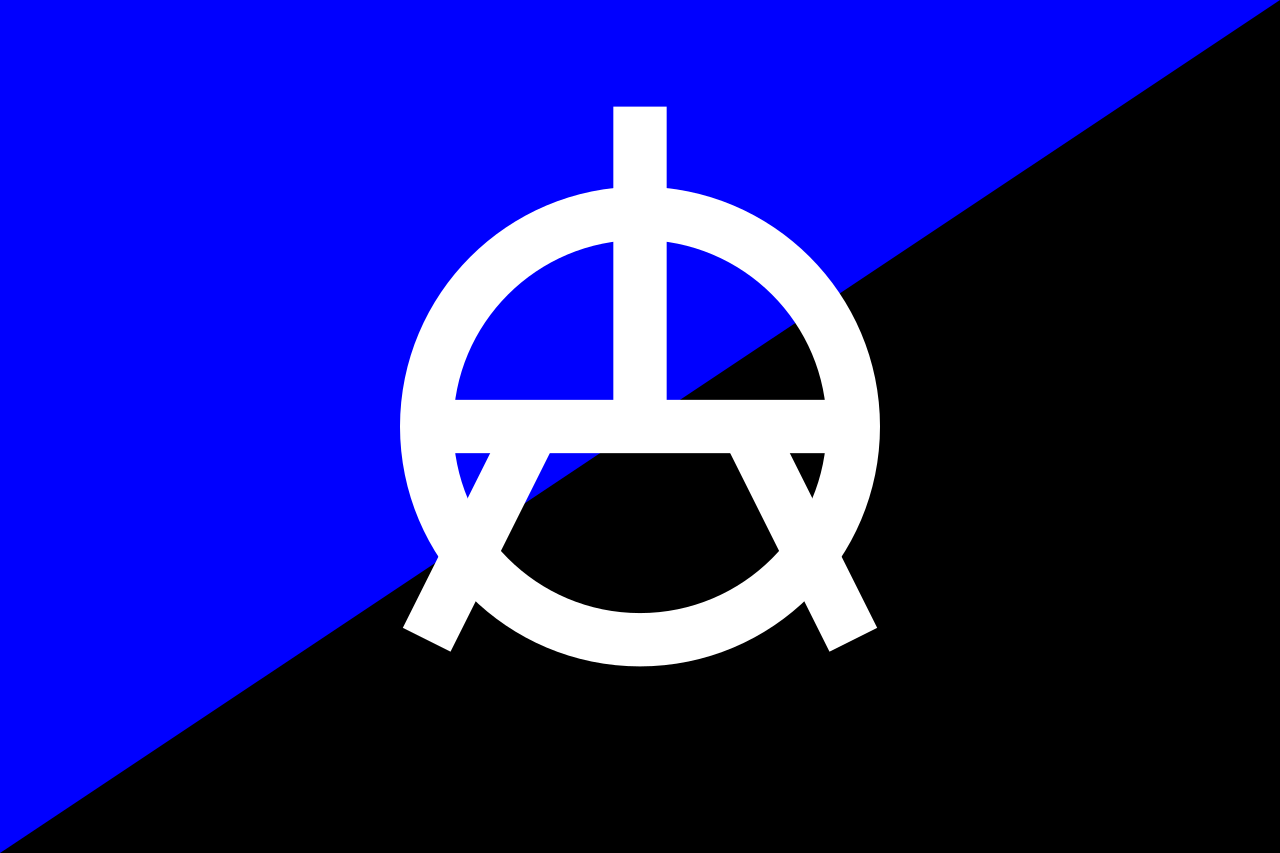

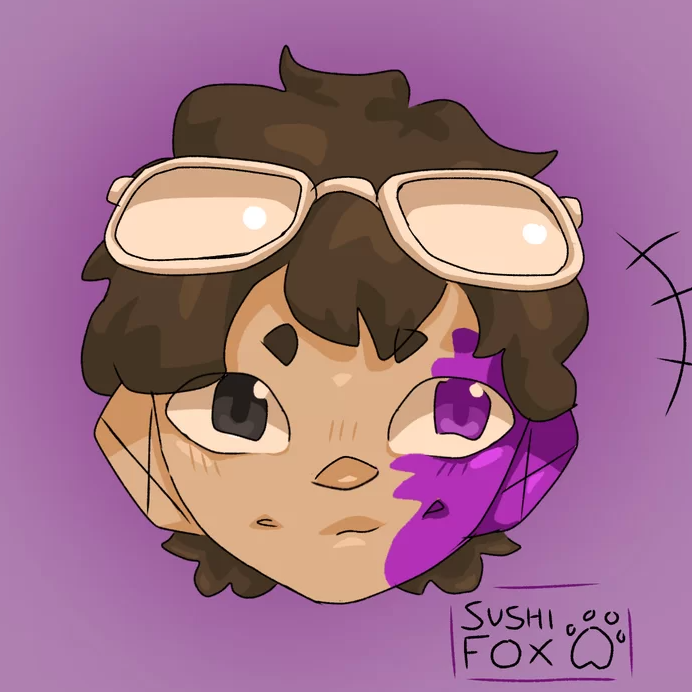
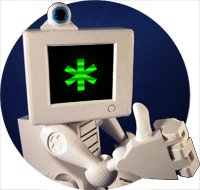
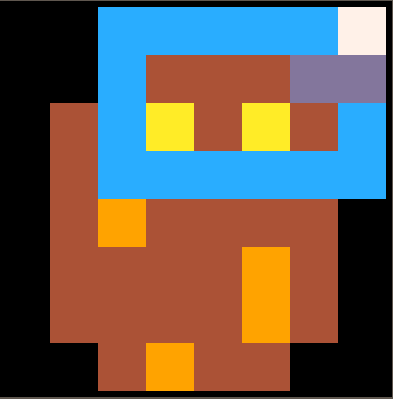
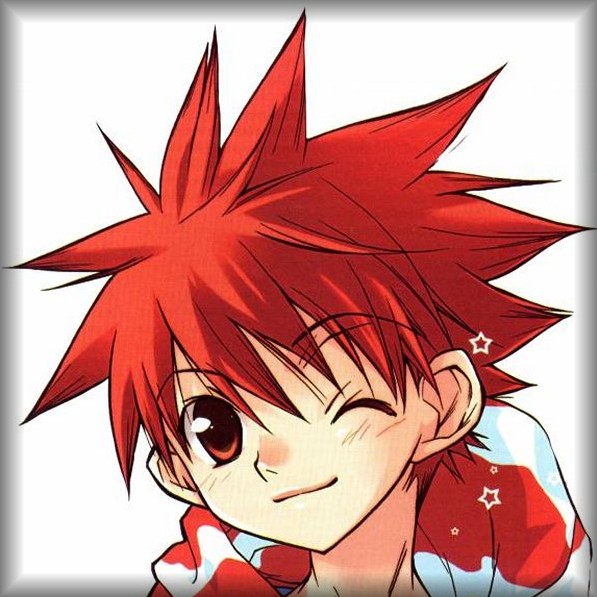


@zep it looks like if you do a cstore from a cart in splore or an exported binary, what it saves to the .lexaloffle/pico-8/cstore folder is only those regions of cart ROM that were cstored, and then next time you launch it from splore (or next time you run the exported binary), only those regions of the ROM are copied to base RAM; everything else is blank
I'm guessing the cstore file is intended be overlayed on top of the original ROM contents when copying from cart ROM to cart RAM (and that's why it only saves the changed parts?), but it looks like there was a mistake in implementation and the cstore file contents actually are the only thing copied to base RAM?
I can't reproduce the behavior when using a p8 or p8.png file; this happens only with splore or from an exported binary
To reproduce:
- load a cart from the splore or launch a binary export of a cart that contains data and calls cstore specifying a partial section of cart memory, e.g. cstore(0, 0, 0x2000) (which is what my jigsaw puzzle cart does but I'm about to change it to write the entire ROM as a workaround ;p)
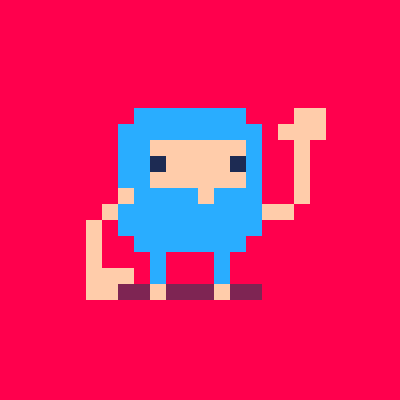
~ ~ ~
The classic holiday pastime of jigsaw puzzles, now in the cozy confines of your PICO-8 fantasy console!
~ ~ ~
Like all "Pro" versions of Jigsaw Puzzle Pack sold by KITTENM4STERSOFT, this version allows any PNG image (up to 128x128 px) to be transformed into a custom puzzle via convenient drag-and-drop!
Features
- 4 Pixel Art Puzzles (+1 custom) In 1 Cartridge!
- Drag-and-drop Custom Puzzles!
- Auto-Save!
- Devkit Mouse support!
- Traditional Background Music!
- RandoCut technology for random cuts every time!
Controls
D-Pad: move cursor
[O]: Pick up/drop
[X]: (Hold) move faster
When Mouse Is Enabled (in pause menu)
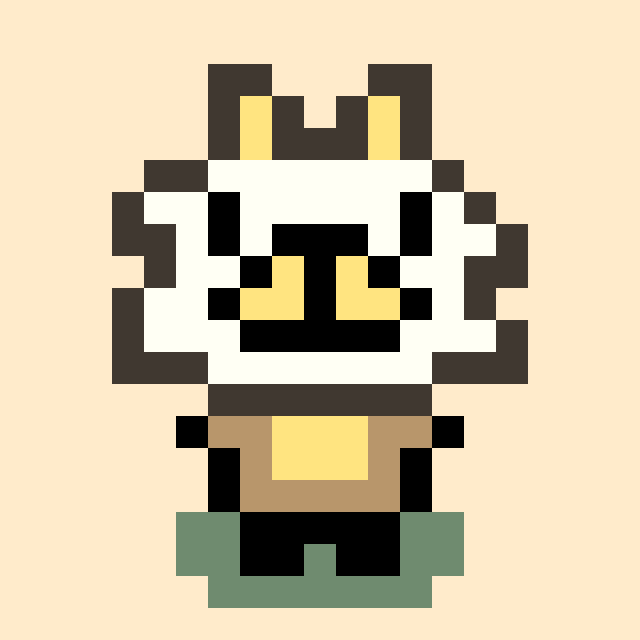
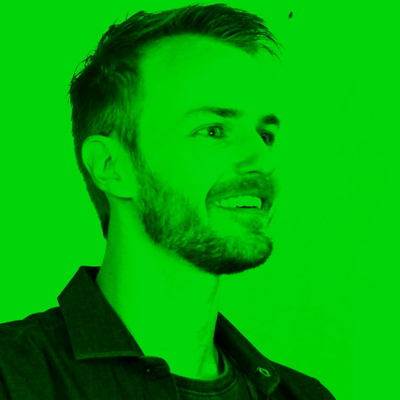
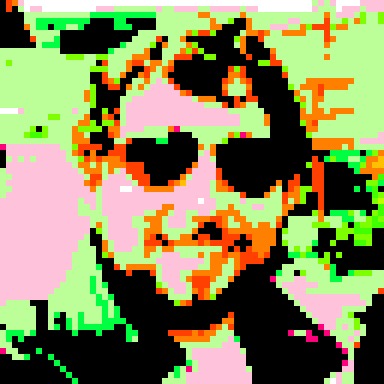



I seem to have found a weird bug. Normally sfx(-1, -2) will stop sfx on all channels, but if it is triggered by a menuitem callback, it doesn't work; in that case only explicitly stopping sfx on each channel works
to reproduce, enter some notes on sfx 8 (so you can hear when playback stops), then use this code and compare the behavior of the two menu items:
function stop_all_sfx_short() sfx(-1, -2) end function stop_all_sfx_long() for i = 0, 3 do sfx(-1, i) end end function _init() menuitem(1, 'stop sfx (-2)', stop_all_sfx_short) menuitem(2, 'stop sfx (long)', stop_all_sfx_long) sfx(8) end function _update() end function _draw() end |