Orb Pondering Simulator is a silly little art experiment I made for a game jam. Read more about the motivations on the game's Itch page.
Press UP and DOWN to cycle through orb palettes. LEFT and RIGHT cycle through the available backgrounds. X pauses or resumes the background animations.
Happy pondering!
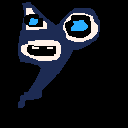

I'm working on a game that notes how high players climb. Right now I'm trying to put together a "ruler" that stays on the left side of the screen and scrolls with the player, letting them know at a glance how far they've traveled. Every 50 pixels, the ruler has a slightly longer line, and a printed numerical value indicating the corresponding height. Here's what I have so far.

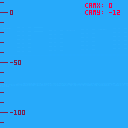
Right now, the ruler is powered by a for
loop in the function draw_elevation()
, which means that it has a definite start and end. Could anyone recommend a method for making the ruler extend in both directions indefinitely (without crashing PICO-8)? My efforts to try while
and repeat
loops have failed. (Bonus points for making the 0
line start at the bottom or center of the screen!)
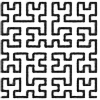
.png)
The Question
Hello everyone,
I am ludicrously close to finishing the engine for my game, but some physics equations have me stuck. Here is what I'm trying to achieve:
- The players are effectively nodes that can switch between being pendulum bobs and fulcrums. One can be anchored to serve as a fulcrum while the other swings. If both are anchored, neither moves. If both try to un-anchor themselves, they fall.
- In order to let the players navigate the playfield, I want the swing of a pendulum bob to increase bit by bit. (This will let players climb the playfield by swinging upward instead of just descending.)
- Once a bob makes a full loop around the fulcrum, I want it to continue in a circle at a more-or-less consistent speed.
With the help of this math writeup, I managed to assemble a simplified set of physics variables and equations that do an okay job of simulating momentum and creating a non-degrading swing (i.e., the swing distance never shrinks due to friction). But I haven't figured out how to make the desired increase in swing distance happen. I'm sure it's a single value or sign that I haven't identified.

.png)
I am working on a little shooter where players can move up and down a fixed track, and can aim a cannon to shoot a projectile at an angle. Ideally, this angle can run parallel to the track, but shouldn't intersect it.
I thought I had the math down, but I am running into an issue where the players' projectiles are able to intersect the track. It appears that, even though I constrain the angles that player cannons can point and fire, players seem to be able to extend and shoot a tiny bit beyond it. Strangely, a simple debug test that prints the angles indicates that they should be parallel to the track in these situations.
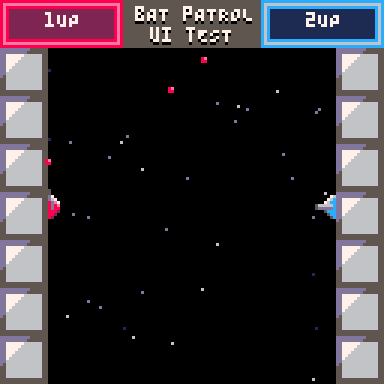
Angle constraint formulas:
--player 1 is on the left side, and aims right if p1.a<270 and p1.a>100 then p1.a=270 end if p1.a>90 and p1.a<270 then p1.a=90 end --player 2 is on the right side, and aims left if p2.a<90 then p2.a=90 end if p2.a>270 then [ [size=16][color=#ffaabb] [ Continue Reading.. ] [/color][/size] ](/bbs/?pid=116799#p) |
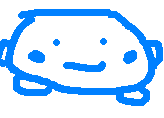
.png)


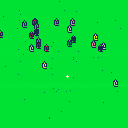
A silly little toy I threw together. Click your mouse to generate a bunch of randomly-colored snails that flee at varying speeds to the left and right of the screen.
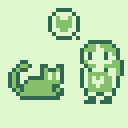

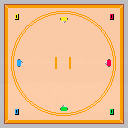
I'm pleased to unveil my finished weekend project: Robosumo! Inspired by this entertaining video a friend showed me this week.
Special thanks to:
freds72, for helping me in the forums.
huulong, for an invaluable sprite rotation function.
NuSan, for a number of useful physics functions in Combo Pool.
Daisyfield's archive of public domain Japanese music.
Introduction
It's sumo, but with robots! Ram your opponent and send them flying from the dohyō. Speed and finesse are your only weapons – but take care they're not turned against you.
.png)
Hi everyone,
I'm working on a little robotic sumo game for a friend after seeing an IRL video about it. I decided to include a 4-player mode, because I like multiplayer games.
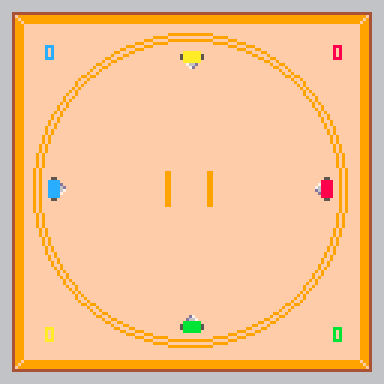
I want players to score only if they're the ones who knocked the player out of the arena when play is halted (basically like in Super Smash Bros), but I'm having trouble recording that information.
Here's the relevant code:
--main game loop for p in all(players) do check_hit(p) --other stuff end function check_hit(p) for o in all(players) do if circlehit(p,o) and --Basic collision function. p.col~=o.col then --This line is meant to keep players from hitting themselves. p.lasthit=o.col --Here, "col" is the player's color, and "lasthit" is who last hit them. end --In another function, I award points to the player whose color [ [size=16][color=#ffaabb] [ Continue Reading.. ] [/color][/size] ](/bbs/?pid=87548#p) |

.png)
Hi everyone!
I am making a little game where you scare off crows to protect your vegetable garden:
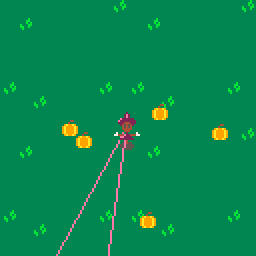
I am trying to make the crows pick a vegetable to target when they spawn. I have a function that will make them chase down a point by entering its X and Y value. My problem is that I don't know how to extract the vegetables' X and Y values because of how I've set things up.
Every vegetable is decided at random at the game's outset, and stored inside another table, like so:
[note: dif is an integer, adding more vegetables at higher difficulties] function setup() for i=1,dif do add(vegetables, { x=originx+(rnd(40)+10)*cos(rnd(360)/360), y=originy+(rnd(40)+10)*sin(rnd(360)/360), w=6, h=6, [more variables and stuff you don't need to care about...] }) end end |
My question is, how would I go about accessing the X and Y values for these? I have been trying something like this...

.png)

It's a long way from finished, but I now have something approximating a playable demo of a 1- to 4-player cart I've been working on.
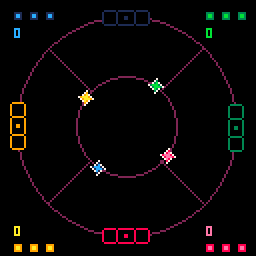
Here is Star Shield for your... Uh... Enjoyment? Or whatever you want to do with it.
Premise
Defend your base against the stars' relentless assault by activating your left or right shield at the correct moment. Rack up a high score in single player, or be the last base standing in multiplayer!
Stars speed up after several deflections, and new stars appear at high point thresholds. Extra lives are awarded to particularly beleaguered players.
Controls
- LEFT or (o): Activate left shield.
- RIGHT or (x): Activate right shield.
For proper orientation, imagine yourself seated behind your station, looking into the circle.
- Player 1: Red (South)
- Player 2: Blue (North)
- Player 3: Yellow (West)
- Player 4: Green (East)

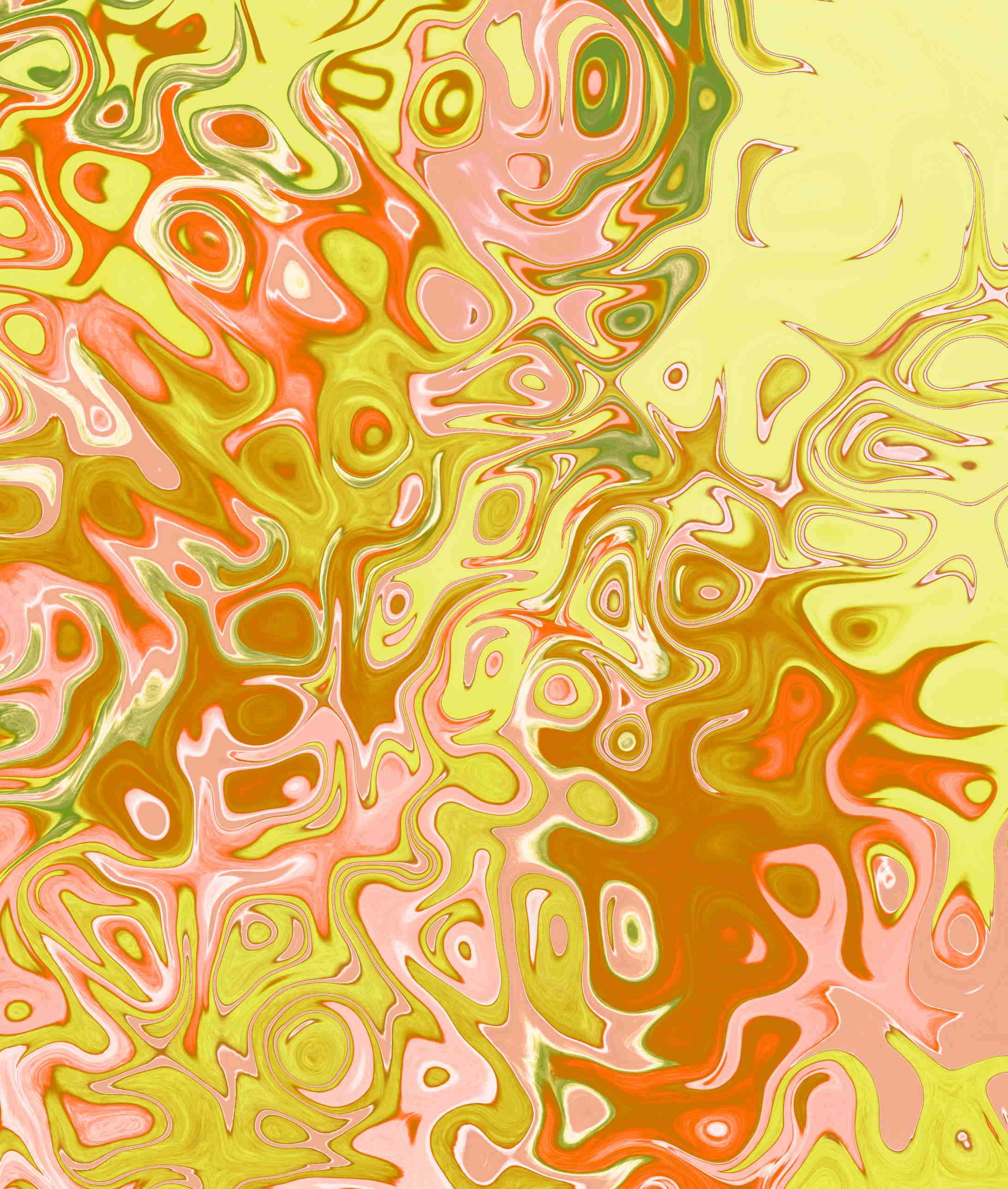
Hi everybody!
So, for a jam I'm far too unskilled to have considered, I'm putting together a little game where up to four players can pilot tiny ships and defend a fort. The ships can move about, and (eventually) shoot in the direction of a target that can be rotated around the ship by holding a button. It looks like this in practice:
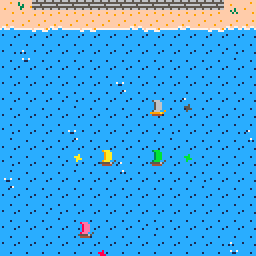
(Enemies and animations forthcoming.)
Right now, I am attempting to optimize the code, because the player inputs eat a LOT of tokens in their current form. I currently have four different sets of inputs, one for each player, and each also moves a target that is specified as its own object (target1 with .x and .y, target2 with .x and .y, etc.). This is obviously no good, given the immense redundancies.
I think one could probably code a generic input function by making each target a property of its ship, using something like this:
function input(p) if btn(0,p.c) then p.x=p.x-1 p.targetx=p.targetx-1 --repositions player and target [ [size=16][color=#ffaabb] [ Continue Reading.. ] [/color][/size] ](/bbs/?pid=73758#p) |
.png)
I'm having an issue where it seems like I absolutely cannot pass an argument to a function, no matter what I do.
For starters, I am using doc_robs' famous hitbox function:
function player_hit(x1,y1,w1,h1, x2,y2,w2,h2) local hit=false local xs=w1/2+w2/2 local ys=h1/2+h2/2 local xd=abs((x1+(w1/2))-(x2+(w2/2))) local yd=abs((y1+(h1/2))-(y2+(h2/2))) if xd<xs and yd<ys then hit=true end return hit end |
So far, so good, right? Then I pass the following arguments along to it from another function:
if player_hit(p1.x,p1.y,pw,ph,star1.x,star1.y,sw,sh) then --stuff happens in here end |
All of the values in the preceding if statement are defined at _init(). The values pw, ph, sw, and sh are all 2, defined as global variables. The p1 and star1 values come from tables.
But every time I run my program, this error occurs:
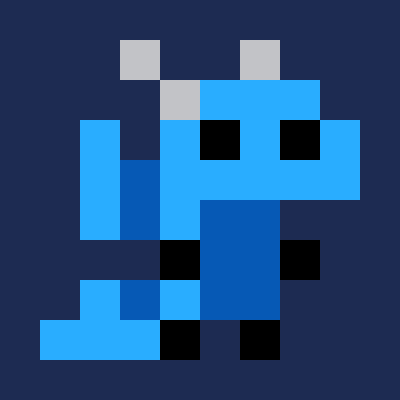
If you grew up with the NES, you remember that their basslines had a certain "snappiness" to them (for lack of a better word). Here are two of my favorite examples:
DAT BASS!
Anyway, I've been trying to mimic that type of bass as closely as I can in my PICO-8 carts, but I haven't quite hit the mark yet.
Does anyone have instrument/effect suggestions for achieving this kind of sound?
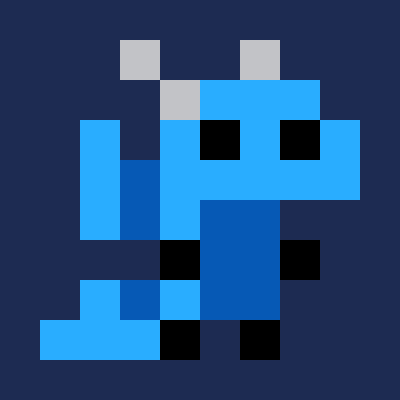
.png)
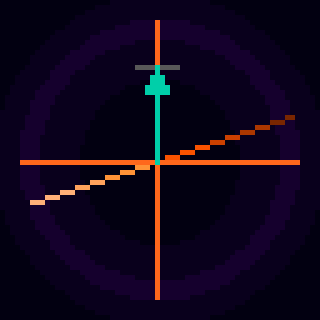
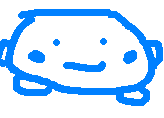
This is probably a ludicrously simple thing that I never learned, so please forgive me in advance.
I'm trying to write a function that checks whether a thing (star1) moving in a circle intersects with any of four lines (four_p_lineX) that connect to the circle. The best way I can think to do this is to make the function check whether the X and Y coordinates of star1 match the X and Y coordinates of any of the line endpoints.
The thing is, PICO-8 doesn't like what I've written:
function check_direction() local s1switch=flr(rnd(1)) --You wonderful forum people can ignore this part. if (star1.x,star1.y)==(four_p_line1.x1,four_p_line1.y1) or (four_p_line1.x2,four_p_line1.y2) or (four_p_line2.x1,four_p_line2.y1) or (four_p_line2.x2,four_p_line2.y2) or (four_p_line3.x1,four_p_line3.y1) or (four_p_line3.x2,four_p_line3.y2) or (four_p_line4.x1,four_p_line4.y1) or [ [size=16][color=#ffaabb] [ Continue Reading.. ] [/color][/size] ](/bbs/?pid=74024#p) |

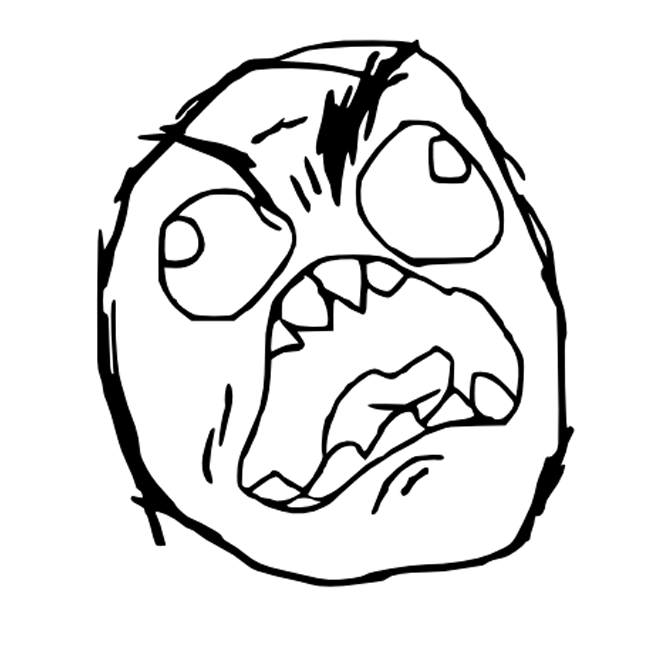
.png)
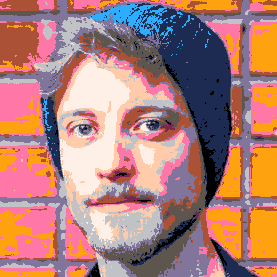
Hi everyone!
I'm hard at work on a 1-4 player cart, but I've run into a snag when it comes to moving a sprite around in a circle.
I more or less have the movement down (thanks to tonechild), but it looks like the wrong part of the sprite is tracing the circle I want:
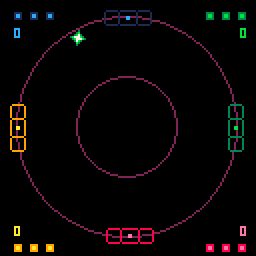
(Please excuse the incomplete graphics and such.)
It appears that the upper-left corner of the sprite is tracing the circle here, when I'd really prefer something closer to the center of the sprite. Is there a way to make this happen? Or, if not, is there a workaround any of you might recommend?
Here are the relevant points of my (messy) code. You'll notice the star is able to "trace" the track because it uses the same variables I used to draw the circle.
--variables scene="logo" last=time() game_over=false --determines that a solo player has lost player_win=false --determines that a player won in multiplayer [ [size=16][color=#ffaabb] [ Continue Reading.. ] [/color][/size] ](/bbs/?pid=73965#p) |

.png)

With many thanks to packbat for helping me in the forum, I can finally say my first real cart is complete!
I give you: BASTARD SQUASH.
I don't have any training in programming or math, so this isn't as impressive as most debut carts. Still, we all have to start somewhere! I hope you find something to enjoy in it. Also, don't mind the unused sound samples, and general code inelegance...
Description
Have you ever thought your game of squash needed to hate you a little more? Then do I have a cart for you!
Your paddle shrinks with each successive hit. Past certain point thresholds, it also begins to creep toward the ceiling. Extra lives are granted every 300 points, because I love you, even if my game doesn't.
I'm looking for some advice on how to grant the player extra lives after the score crosses a certain point interval.
I've checked a few carts that do this, but I'm not able to make sense of the functions they use, and I was wondering whether anybody had a simple method handy.
At the moment, I only have a brute-force method. My cart uses two straightforward variables: "lives" counts the player's lives, and "score" counts the score. Let's say I want to give the player an extra life for every 300 points they score. Right now, I only have this:
function gainlife() if score=300 then lives+=1 elseif score=600 then lives+=1 elseif score=900 then lives+=1 end end |
It kinda works (assuming the player loses interest in the game after around 1,000 points). But I figure there HAS to be a way to capture the interval in a neater function. Unfortunately, my stupid brain can't figure it out!
Thank you for whatever wisdom you have to share.
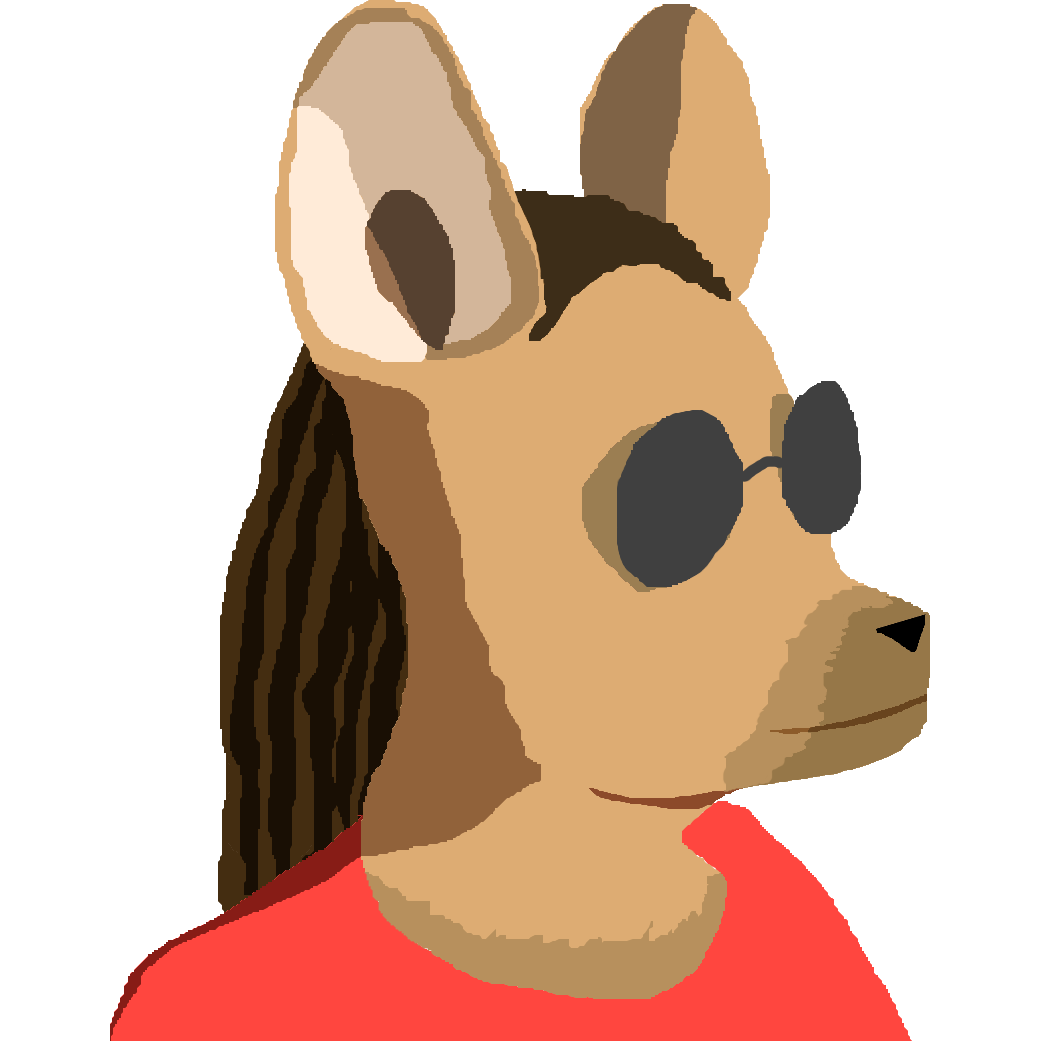
.png)


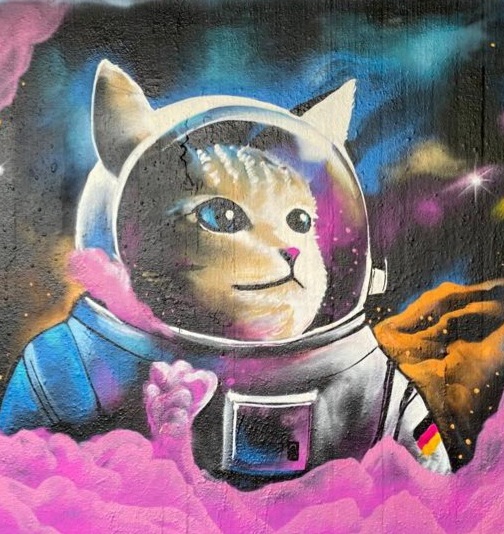
Once again using Dylan Bennett's Game Development with PICO-8 as the backbone, I've rolled out a new project based on his "Lander" recipe. Presenting Red Planet Mission:

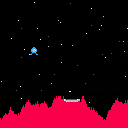
I've tweaked the sprites, added a start screen (with art!), and managed to put together menu and gameplay themes.
I couldn't figure out how to randomize the star background each time you start up the game, but I'll keep trying.
It's quite exciting to have put all the features of PICO-8 to use in a cart! The next step is to do something using my own code next time.
Hi everybody,
I'm having some difficulty figuring out how to solve a problem with how PICO-8 handles music, and I was wondering whether any of you out there could help me.
Let's say I have a few SFX files that I intend to collate into a music composition for my game, like in the following image:
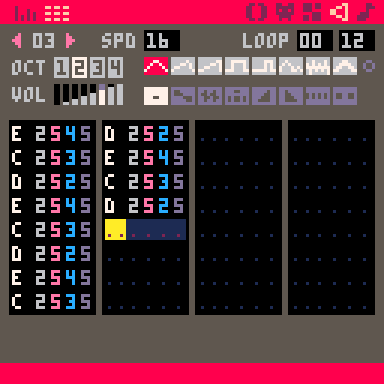
Notice that there are a few unused measures for this clip. Let's assume that I have several other clips that are similarly structured.
When I try to string these together in the music editor, the system plays all those empty measures like they are rests. But what I would really like to happen is for the music to "jump" directly from the end of the clip to the beginning of the next one.
Is there a way to make this happen?
Thank you for any and all input!
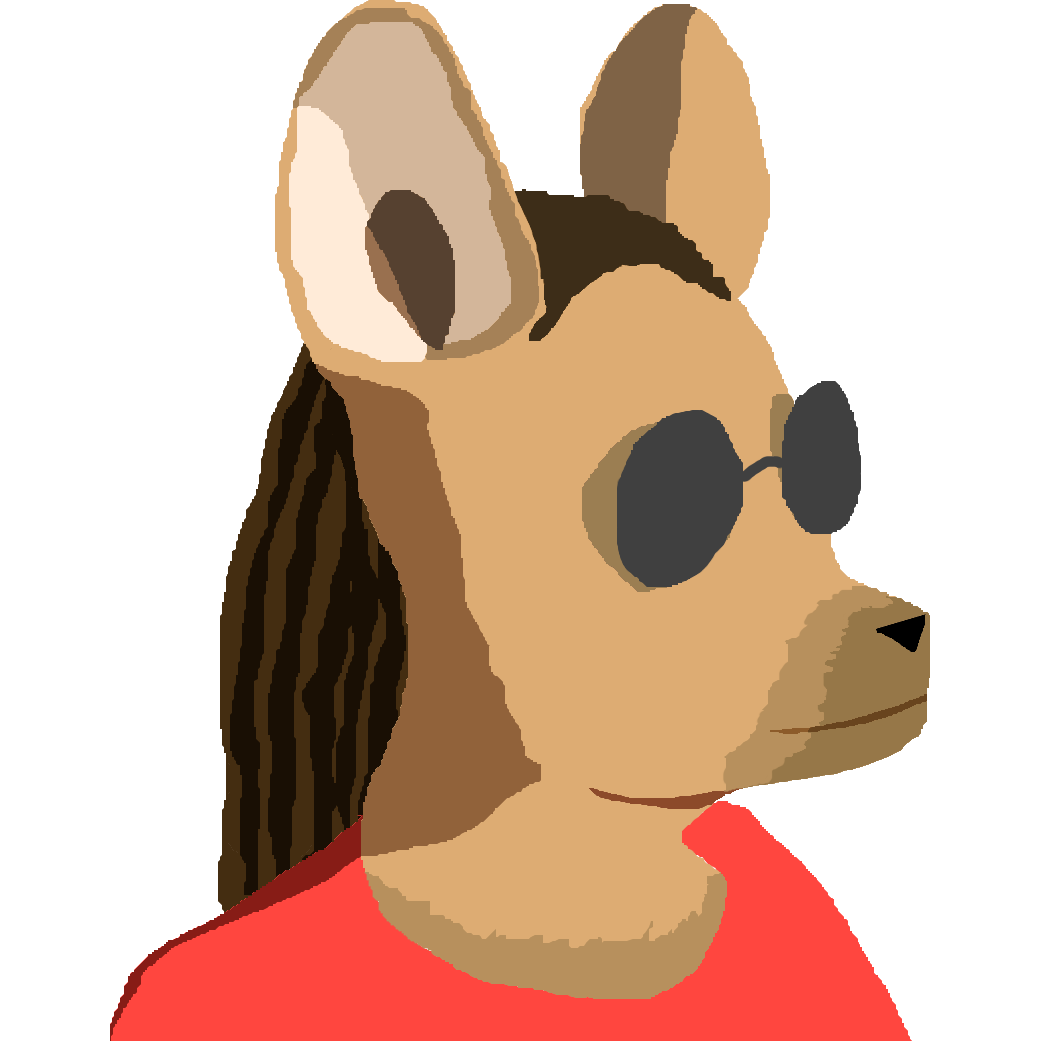
.png)
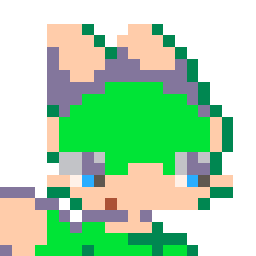
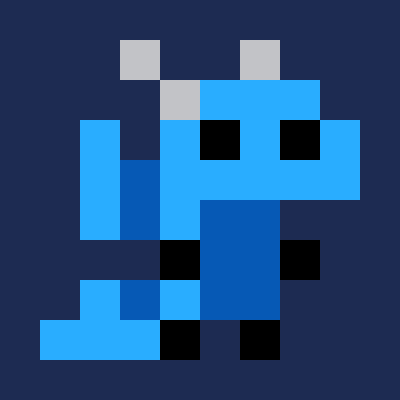
I sampled PICO-8 for the first time this weekend. Being a rank novice at programming, I have little idea of what I'm doing. Luckily, given the abundance of PICO-8 resources here and elsewhere, I'm learning quickly!
Here is my first attempt at a game: a barely modified version of Cave Diver from Dylan Bennett's Game Development with PICO-8. I give you: Bat Cave!
It leaves a bit to be desired as a "game," but in terms of newbie accomplishments, I'm pretty proud of figuring out how to insert a title screen. That will certainly come in handy later.
Here's to producing better games in the future!
