I'm looking for some advice on how to grant the player extra lives after the score crosses a certain point interval.
I've checked a few carts that do this, but I'm not able to make sense of the functions they use, and I was wondering whether anybody had a simple method handy.
At the moment, I only have a brute-force method. My cart uses two straightforward variables: "lives" counts the player's lives, and "score" counts the score. Let's say I want to give the player an extra life for every 300 points they score. Right now, I only have this:
function gainlife() if score=300 then lives+=1 elseif score=600 then lives+=1 elseif score=900 then lives+=1 end end |
It kinda works (assuming the player loses interest in the game after around 1,000 points). But I figure there HAS to be a way to capture the interval in a neater function. Unfortunately, my stupid brain can't figure it out!
Thank you for whatever wisdom you have to share.


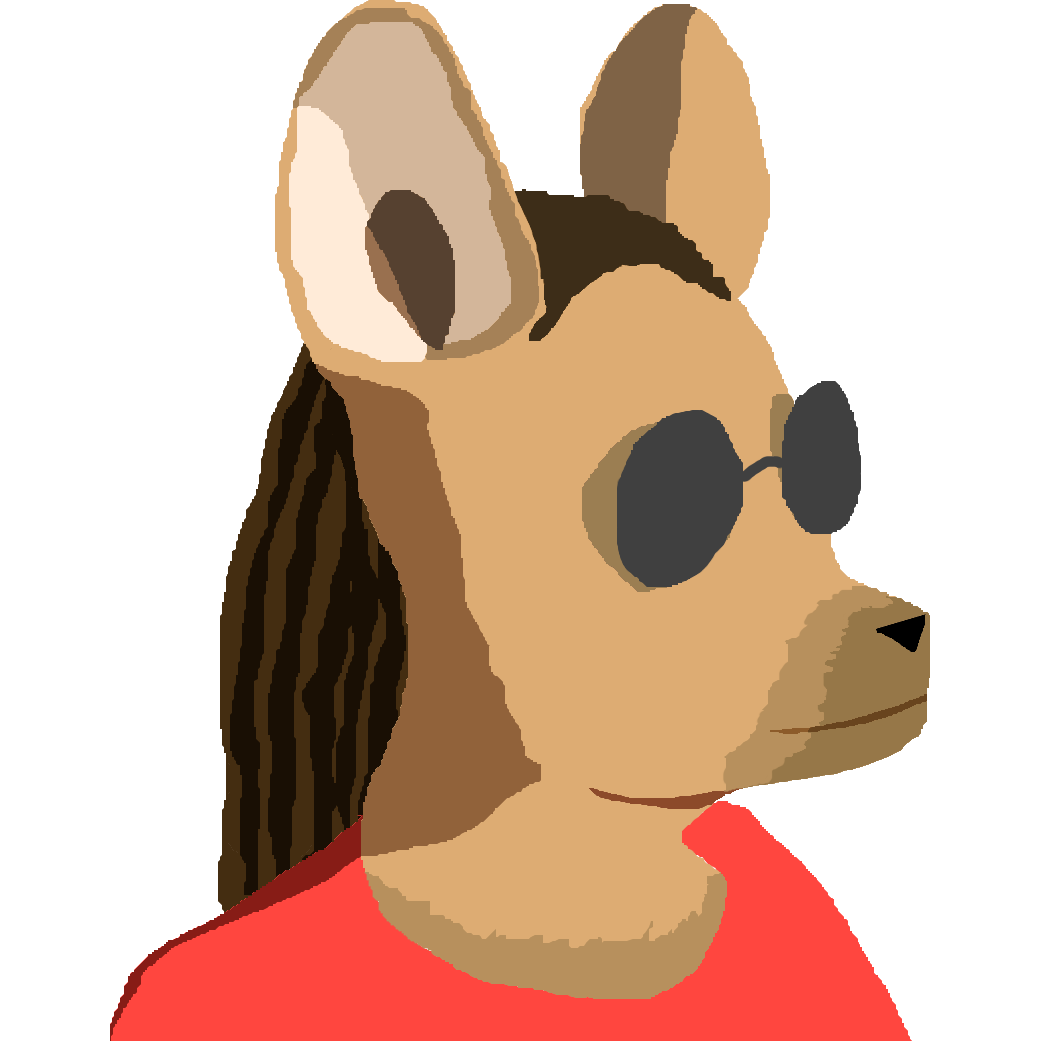
Might be able to do it with the % (modulo) function - score%300 would be the remainder after dividing by 300, and equal to zero at 300,600,900,&c.
So the equivalent of your example might be:
function gainlife() if score%300==0 then lives+=1 end end |
It might not work right if the player's score skipped over 300/600/900/whatever exactly - say, they were at 890 points and got 20 in one go. But with that caveat, I think modulo would work.


.png)
Thanks for your quick response! I appreciate the support.
This seems like it works! You've brought me that much closer to issuing my first real (albeit humble) cart. Thank you so much.



Another possible Solution is: Storing the Values that gains Life in a Table, and iter through it using "for":
function gainlife() local gainlives_tbl={300,600,900} for gainlive in all(gainlives_tbl) do if score == gainlive then lives+=1 end end end |
May be useful if you want something "hard-coded" (not every single +300 Score) but also want to reduce the amount of Space and Tokens...



The method I use is something like this ..
score = 0 bonus = 300 . . . score+=15 -- or whatever . . . if score>= bonus then lives += 1 bonus += 300 -- or bonus += bonus to make getting lives a little harder end |
Allows the score to increment at any value then :)


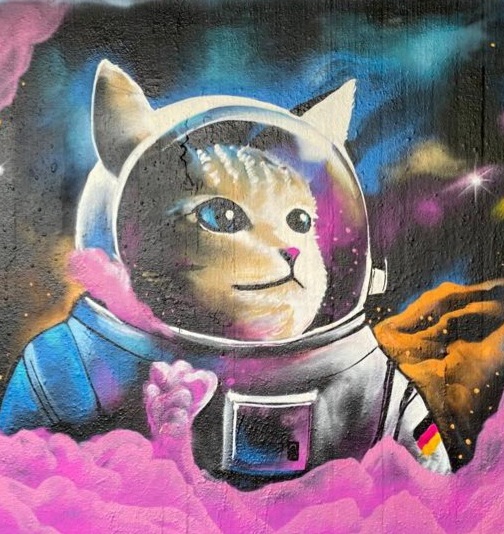
If you dont increase the score in every frame make sure it won
t add / check for extra lives until the score changed again!
[Please log in to post a comment]