This is probably a ludicrously simple thing that I never learned, so please forgive me in advance.
I'm trying to write a function that checks whether a thing (star1) moving in a circle intersects with any of four lines (four_p_lineX) that connect to the circle. The best way I can think to do this is to make the function check whether the X and Y coordinates of star1 match the X and Y coordinates of any of the line endpoints.
The thing is, PICO-8 doesn't like what I've written:
function check_direction() local s1switch=flr(rnd(1)) --You wonderful forum people can ignore this part. if (star1.x,star1.y)==(four_p_line1.x1,four_p_line1.y1) or (four_p_line1.x2,four_p_line1.y2) or (four_p_line2.x1,four_p_line2.y1) or (four_p_line2.x2,four_p_line2.y2) or (four_p_line3.x1,four_p_line3.y1) or (four_p_line3.x2,four_p_line3.y2) or (four_p_line4.x1,four_p_line4.y1) or (four_p_line4.x2,four_p_line4.y2) then if s1switch==1 then --Ignore this, too. star1.line=not(star1.line) end end end |
The above code produces the following syntax error:
SYNTAX ERROR LINE 187 (TAB 2) if (star1.x,star1.y)==(four_p_line1.x1,four_p_line1.y1) or ')' expected near ',' |
I can't really figure out what the error is telling me. Is it as simple as me forgetting some punctuation, or can I not make the comparison I'm trying to make?



suggest to read the lua manual to learn about lua syntax: https://www.lua.org/manual/5.2/
you cannot test equality with multiple values.
if a==b or a==c or a==d then... end |
is the correct syntax.


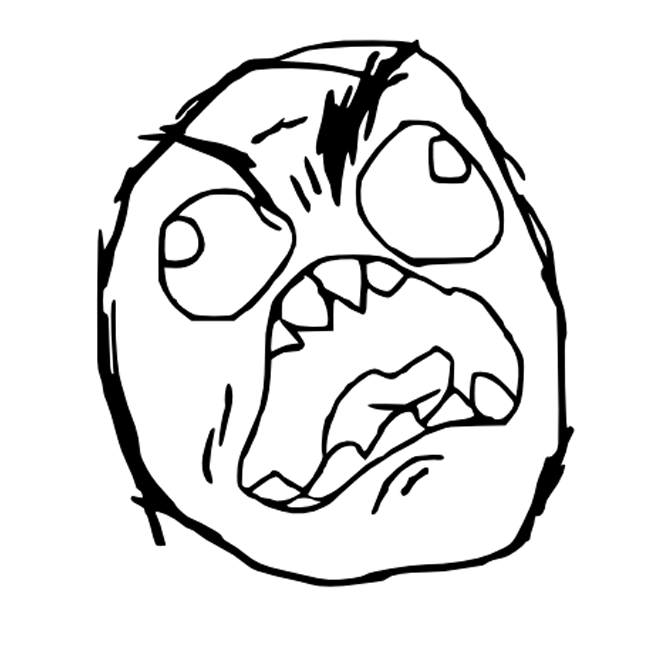
Here is one possible way to do it:
function check_direction() local s1switch=flr(rnd(1)) --You wonderful forum people can ignore this part. local intersect = false for p in all({four_p_line1,four_p_line2,four_p_line3,four_p_line4}) do if (star1.x==p.x1 and star1.y==p.y1) or (star1.x==p.x2 and star1.y==p.y2) then intersect = true break end end if intersect then if s1switch==1 then --Ignore this, too. star1.line=not(star1.line) end end end |


.png)
Thanks for your inputs! The link to the Lua manual has been helpful, and this code snippet seems like it should work.
samhocevar, if you don't mind my asking, how does the "p" in "for p" work? It seems like it's one of the following:
- A random variable that for loop outputs (i.e., do this thing, and call the result "p").
- A thing to identify each of the lines, since they all contain a single "p."
Thanks for your help! I am grateful.


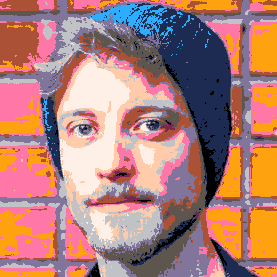
The "p" is a loop variable. It is set to each value that is returned from "all()" in turn.
So when you see "for p in all(table)" you can think of it as "for each element in table, call that element p and do the following".
See also the Lua manual and Programming in Lua (but note that regular Lua doesn't have "all()", it has "ipairs()" instead).
[Please log in to post a comment]