I am working on a little shooter where players can move up and down a fixed track, and can aim a cannon to shoot a projectile at an angle. Ideally, this angle can run parallel to the track, but shouldn't intersect it.
I thought I had the math down, but I am running into an issue where the players' projectiles are able to intersect the track. It appears that, even though I constrain the angles that player cannons can point and fire, players seem to be able to extend and shoot a tiny bit beyond it. Strangely, a simple debug test that prints the angles indicates that they should be parallel to the track in these situations.
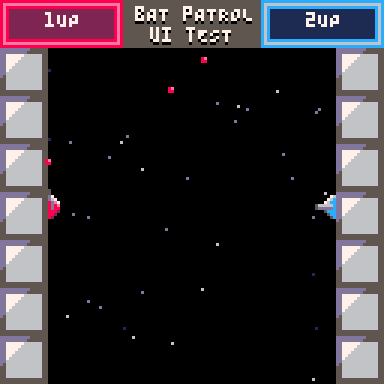
Angle constraint formulas:
--player 1 is on the left side, and aims right if p1.a<270 and p1.a>100 then p1.a=270 end if p1.a>90 and p1.a<270 then p1.a=90 end --player 2 is on the right side, and aims left if p2.a<90 then p2.a=90 end if p2.a>270 then p2.a=270 end |
Cannon aiming formulas:
if btn(⬅️, p.c) then p.a+=target_speed p.tx=p.x+radius*cos(p.a/360) p.ty=p.y+radius*sin(p.a/360) if p.a>360 then p.a=0 end end if btn(➡️, p.c) then p.a-=target_speed p.tx=p.x+radius*cos(p.a/360) p.ty=p.y+radius*sin(p.a/360) if p.a<0 then p.a=360 end end |
Have I found a bug with how PICO-8 does its circle math? Or have I erred somewhere? (It's probably the latter, but I can't see what I'm doing wrong.)

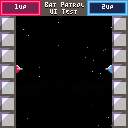


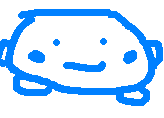
pico8 angles are 0 to 1, not 0 to 360.
see: https://www.lexaloffle.com/dl/docs/pico-8_manual.html#Quirks_of_PICO_8
https://demoman.net/?a=trig-for-games


.png)
Well, PICO-8 does angles as a percentage of 1, so you can still use the calculation functions as long as you express things as a percentage (which I do – all my trig functions use p.a/360). I don't think that's the root of the problem? But I could be wrong.



The tank positions (p.tx
, p.ty
) are not updated after the angle constraint! Therefore, in _draw()
and add_bullet(source)
, p.tx
and p.ty
are still not constrained, and can go behind player.
if p1.a<270 and p1.a>100 then p1.a=270 end if p1.a>90 and p1.a<270 then p1.a=90 end -- update the tank positions! p1.tx=p1.x+radius*cos(p1.a/360) p1.ty=p1.y+radius*sin(p1.a/360) |
Also, there is another problem with the current approach: when you hold left and press "x" to shoot bullet, due to the order of the program flow, the constraint will not be applied when calling add_bullet()
and can go behind player. You need to apply the constraint after "left/right" button processing but before "x" button processing.
if btn(right, p.c) then ... end -- Maybe do angle constraint here. if btn(x. p.c) then add_bullet() end |
Good luck!


.png)
Oh, this is a great fix! Thank you, bob1996w. You've helped me over a big hurdle.
And thank you, merwok, for your help! I appreciate you reaching out to assist.
[Please log in to post a comment]