Hey everyone. I recently released Goober's in the Mix. It was my first bigger pico game that actually required me to optimize for tokens. I wrote a lot of pseudo object oriented code with classes and inheritance. I found myself with a lot of code that looked like this:
local thing = class{ update = function(self) -- cache a bunch of properties local x, y, dx, dy, health, frozen, invuln = self.x, self.y, self.dx, self.dy, self.health, self.frozen ... game logic in here -- resync all those properties on the instance self.x, self.y, self.dx, self.dy, self.health, self.frozen, self.invuln = x, y, dx, dy, health, frozen end } |
All that caching and syncing saves tokens instead of referencing self in the logic, but we can save more.
Lua has a feature called environments that lets you alter how global variables are looked up. So I altered my initial class declaration to look something like:

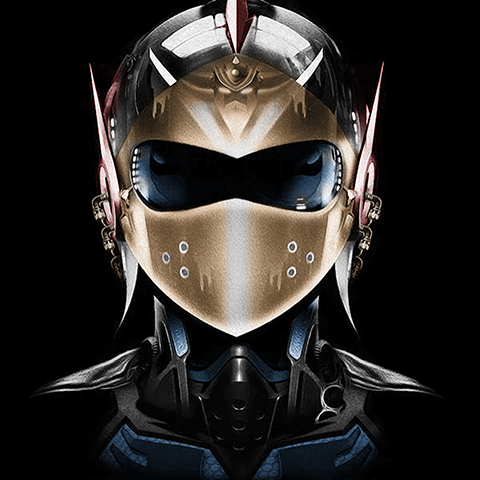

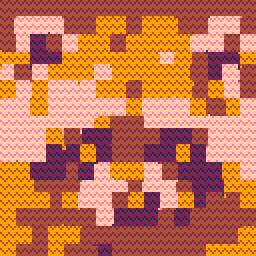
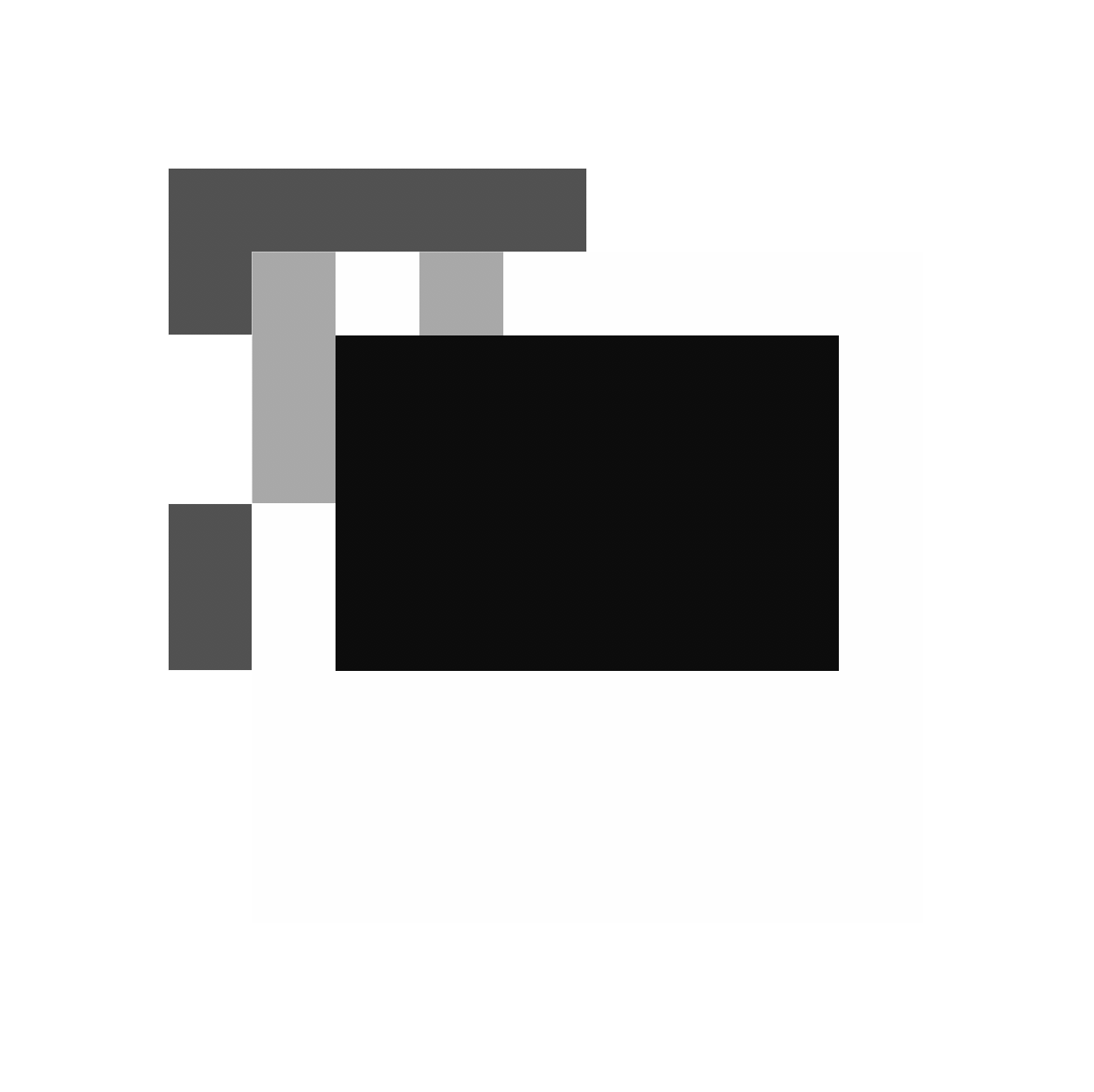
edit: fixed the sluggish jumps
edit: tightened up camera, added some tips about switching items, shrunk spike hitboxes, added some helpful tiles in the firepit
edit: widened the rat/bat safety window, added a chest near the star door which gives you an extra heart and serves as a 1 time savepoint
edit: healing salve only replenishes a heart, cap is still 4 hearts
edit: fix collisions at trigger points
edit: add skull to door guarded by boss & add visual feedback when boss is hit
edit: changed the healing salve to a full checkpoint, rat doesn't spawn under the double spikes until after you get the first potion, added a in-game tip about using potions, added another checkpoint in the firepit, took the corner off the annoying ledge over the firepit spike jump, changed boss dialog to make it more clear about what he does or doesn't have in his possession.
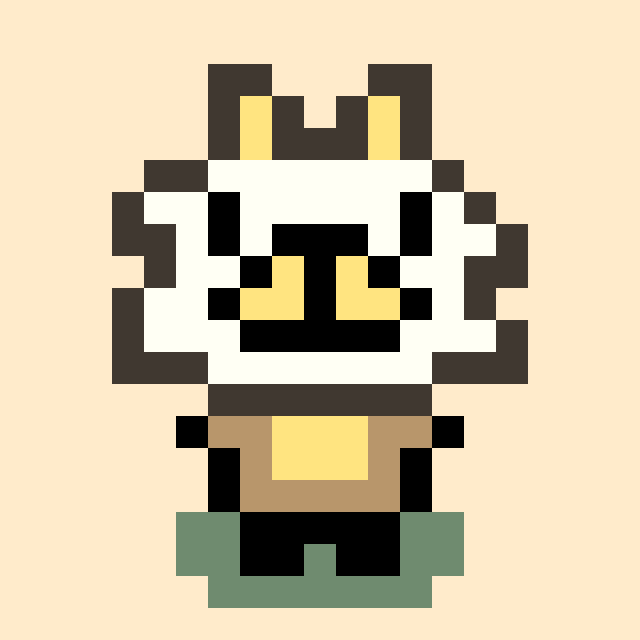



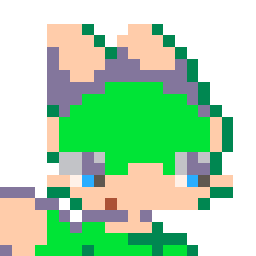

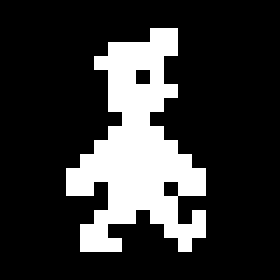
Hey guys, don't know how many people follow Zep on Twitter, but, thought I'd share some news:
"100% Lua scripted" - from 2:50 am - 17 May 2018

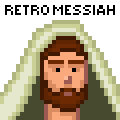
Rhythm Game made in about 50 hours for Music Game Jam. Team of codyloyd and myself. First jam for both of us.
Hit the buttons to the beat while avoiding the asteroids.
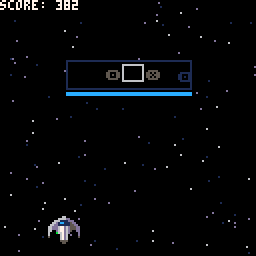
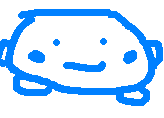
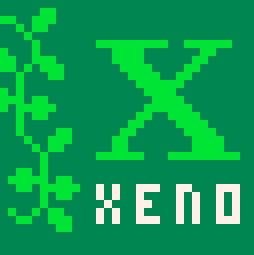
I've been seeing a lot of version spam in Splore while browsing, and I'd like to avoid doing that myself if I can avoid it. But I'm not entirely sure how Splore works. Is there some sort of wiki info about it? Or a guide some where? Are there best practices for stuff like versioning/cart data/naming?



