So I recently rediscovered the magic that is Puyo Puyo! However, I'm absolutely terrible at it. I'm a pretty slow thinker and can never analyse the situation quickly enough to predict the fall of blocks and construct large chains.
So, I wrote this little training tool that allows you to play Puyo in single-player as slowly as you like! You can spend an infinite amount of time deliberating each move, and even go upwards.
Threw in some sfx and autotiling for fun :)
[update 2017-02-22]
- configurable rotation controls, mainly for those using the web player on mobile devices
[update 2016-08-13]
- the 13th row (outside the top of the board) is now treated correctly
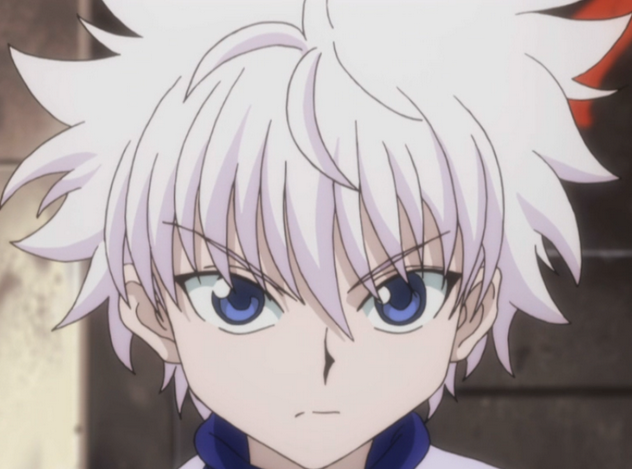

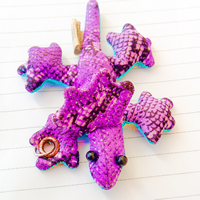



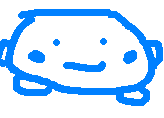
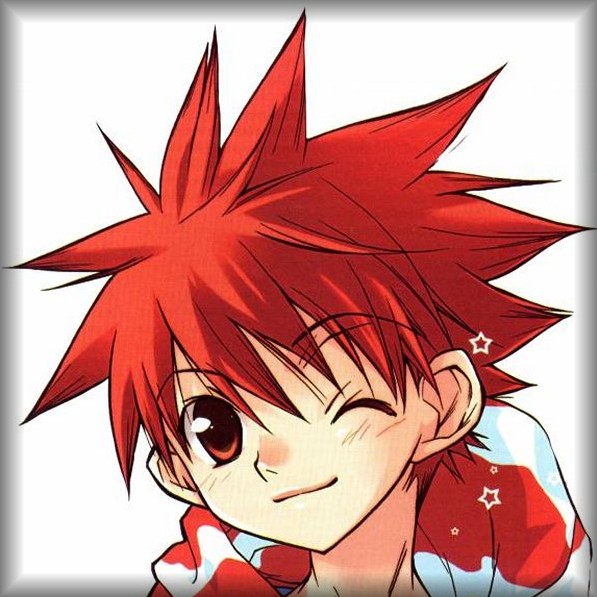
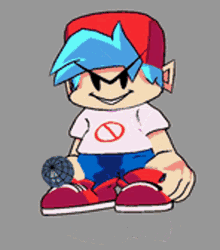
Hey folks! This is a demo cart to showcase use of coroutines to create animations and dialogue.
By having a single active 'script' coroutine we can do the following:
- reveal text frame by frame
- do nothing until player presses [x]
- give the player a choice of answers [up/down + x] to select
- do nothing until npc has finished walking
- do several of the above simultaneously, and only continue once all of them are finished.
all this without blocking the _update() function
For more info on coroutines, check out the forum post. Also keep an eye out for Fanzine #5, where Dan Sanderson will be exploring these topics!


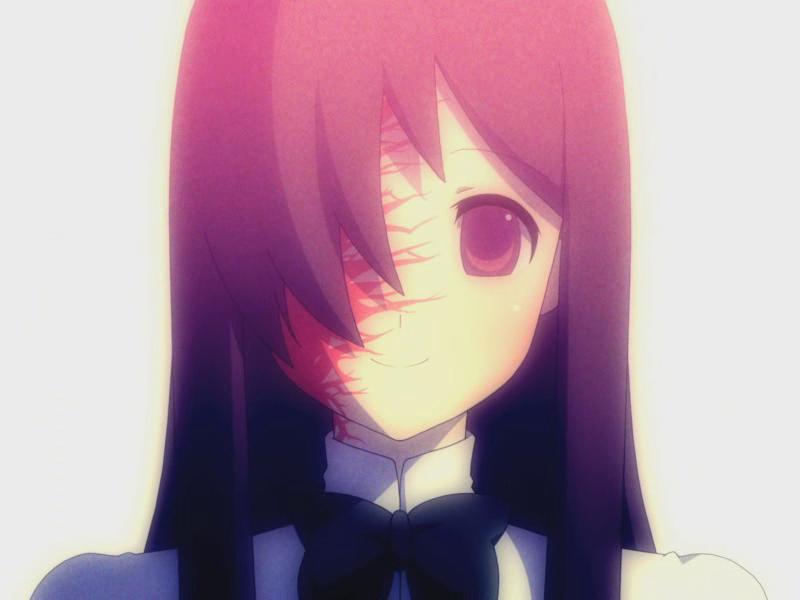


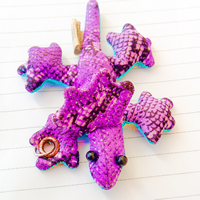
Hi! Some folks on the IRC were struggling with metatables, and Ivoah suggested I post an explanation here to help more people get to grips with them. Here goes nothing:
A table is a mapping of keys to values. They're explained quite well in the PICO-8 manual so I won't go into more detail. In particular you should know that t.foo is just a nicer way of writing t["foo"] and also that t:foo() is a nicer way of calling the function t.foo(t)
A metatable is a table with some specially named properties defined inside. You apply a metatable to any other table to change the way that table behaves. This can be used to:
- define custom operations for your table (+, -, etc.)
- define what should happen when somebody tries to look up a key that doesn't exist
- specify how your table should be converted to a string (e.g. for printing)
- change the way the garbage collector treats your table (e.g. tables with weak keys)

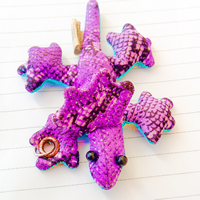




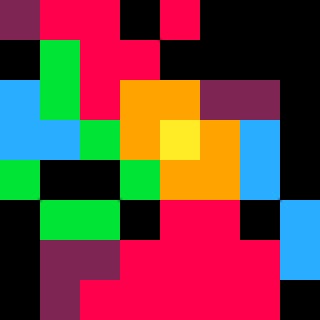
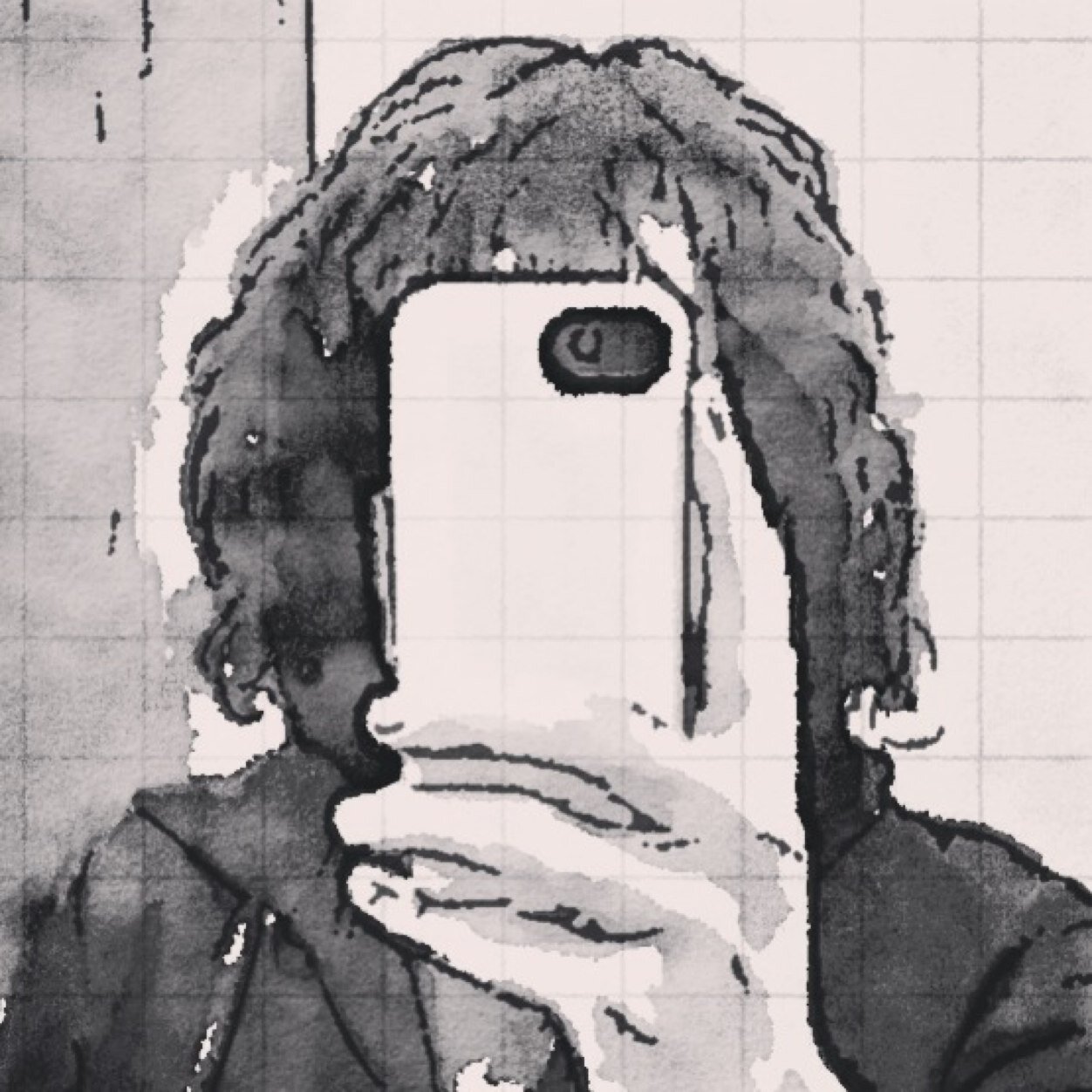


Updated version for UoB Game Dev Society halloween jam :)
- now has a fancy title screen and animations
- now has diagonal chains (like in normal reversi/othello)
- individual cats can be flipped (including white -> black)
Puzzle game concept for the 1 Hour Game Jam.
I didn't have time to design difficult puzzles, but the concept is there. :)
Follows the rules of Reversi (but only for horizontal+vertical chains)
To move: make a bridge from a white kitten to your cursor, over 1 or more non-white kittens.
Sleepy kittens will become normal (white) when flipped
Fire kittens will destroy their neighbours when flipped
Queasy kittens will become sleepy when flipped
To win the level, make all kittens normal (white)!
ARROWS: move
Z/C: flip
X: restart level

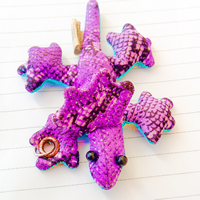
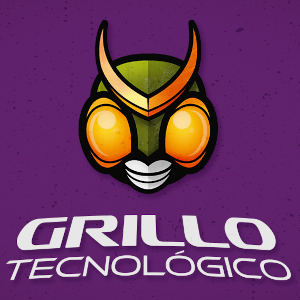
Hi zep! Just a small suggestion, but I'm finding the low-resolution cursor movement really quite awkward to use when I'm so comfortable with existing graphics software on the PC.
Would it be possible (as an optional setting) to either:
a) detach the in-app cursor from the 128x128 grid by creating some special rendering code independent from the console display?
b) hide the in-app cursor and show the system cursor instead?
What are others' opinions on this? I suppose it's not a major issue for most people, and I completely understand if you don't want to do it, to preserve the style/feel of the console. :)
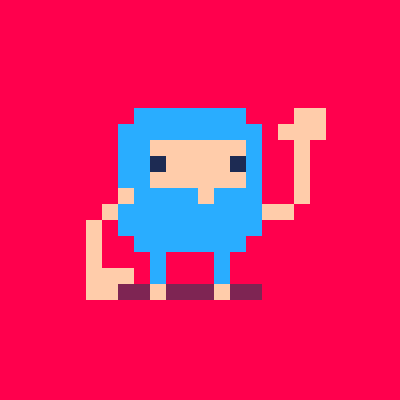
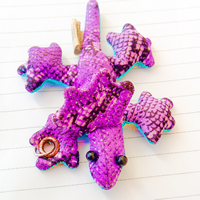