
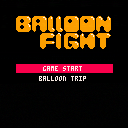
Hey,
I worked on this a few years ago, but lost steam when I realized that I wasn't going to be able to do the music. If anyone has any interest (or if you like the work-in-progress) let me know. The fish doesn't really work, and the bonus stage is just balloons (no player) but it's a reasonable approximation of what I was going for: a fairly faithful demake.
Thanks.
This is a remake of an old Flash game I used to enjoy.
Here's an URL to the Author's Boomshine page: https://k2xl.com/#/games/boomshine
Anyway, one of the best things about the game was the music, but I struggle with the sound/music part. So, if anyone wants to create the music that would be great! Here's a working version of the game online (I'm assuming it's some kind of Flash emulator): https://www.addictinggames.com/strategy/boomshine
Also, my sound effects are pretty crappy too.
Another thing that bothers me is how much the game slows down once we reach 40 balls at the screen at a time. I've gone over everything and I've narrowed it down to the following function.

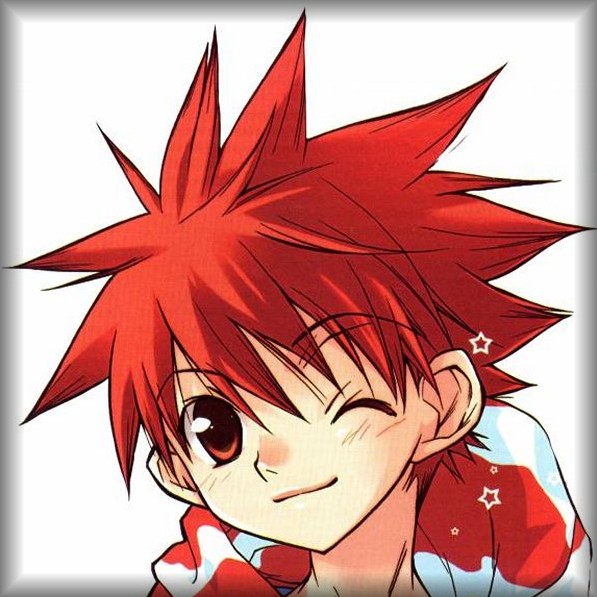

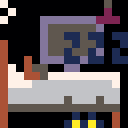

I'm trying to use a breadth-first search on a grid for path finding. My code works for a grid of 7x7, and just barley for 8x8, but it runs out of memory at 9x9. I was just wondering if there was a way I could do things differently to keep the memory usage down.
function grid:findpath(src,dest) function buildpath(node) local path={} local current=node while current do add(path,current,1) current=current.parent end return path end local dirs={ {x=-1,y=0}, {x=1,y=0}, {x=0,y=-1}, {x=0,y=1} } src.parent=nil local queue={} local visited={} for y=1,grid_size do local row={} for x=1,grid_size do add(row,false) end add(visited,row) end add(queue,src) while #queue>0 do local node=deli(queue,1) visited[node.y][node.x]=true if node.x==dest.x and node.y==dest.y then return buildpath(node) end for dir in all(dirs) do local new_node={ parent=node, x=node.x+dir.x, [ [size=16][color=#ffaabb] [ Continue Reading.. ] [/color][/size] ](/bbs/?pid=100526#p) |



Just the old Mastermind board game redone in PICO-8.
I got the music from Robby Duguay (Thanks Robby!): https://www.lexaloffle.com/bbs/?tid=2619
Feel free to let me know about bugs and such.
Edit: New version with bug fix and changes as per comments.

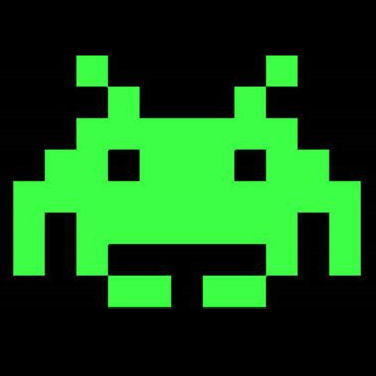

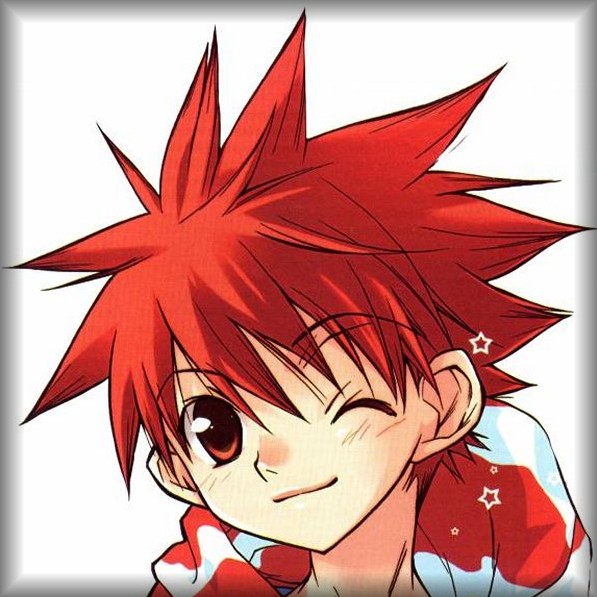


I've run into the same thing on a couple of projects, so I'm wondering if I'm missing something in regards to map().
- sprites have flags marked 0-7
- the map command has the optional argument 'layer' that will display only sprites of a certain flag
- the default argument for layer is 0, which mean to show everything
- if I mark a sprite with flag 0, then using 0 as the argument will just show everything (which isn't what I want)
I think in the past I just didn't make use of flag 0 and it looks like that's what Zep did with Jelpi, but it seems odd that I can't (I think) use flag 0 as a layer argument.
Thanks


So, I was in love with PICO-8 while making a game, but then I ran out of tokens. So, I tried a few techniques to optimize my code but it became clear that I wasn't going to be able to fit everything I had planned. So, I just posted the game with some significant content removed.
Some people have reported bugs here and there, but when I go to fix them I end up running out of tokens just to do my debugging (i.e.adding extra keyboard input for testing purposes).
I get, and enjoyed, the idea of having strict limitations, but hitting the token limit just ruined the experience for me. Even if I could just go outside of its limitations just for the testing process, that would be great. I know it's part of the charm of PICO-8, but the token limit (at least) has kinda killed the fun for me.
Does anyone else know of any tricks for this? Does anyone else feel the same way?
Thanks.
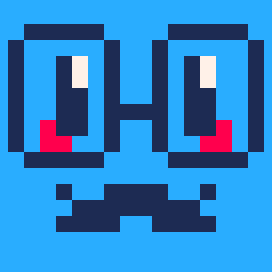

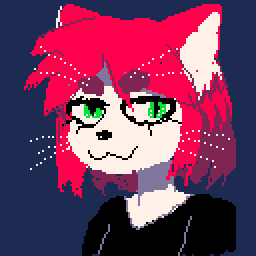
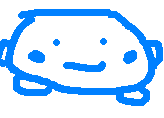
I'm loving PICO-8 with one exception: I'm not crazy about Lua. I've read the Lua documentation for OOP, but the table thing is so different and I can't find examples for what I'm trying to do.
I'm almost finished something I'm working on, but the larger the program gets the more I miss being able to separate the logic into objects (the way I'm used to anyway).
Long story short, I wanted to do the following:
Entity Class:
- x, y, width, height, speed, etc
- function update()
- function draw()
Player Class(that inherits from the entity class):
- score, weapon, power, etc
- function update(overrides but calls super function from entity)
- function draw(same)
Enemy Class(that inherits from the entity class):
- extra variables
- overridden functions calling super functions Entity class
SpecialEnemy Class(that inherits from Enemy/and then from Entity obviously)
- extra variables
- overridden functions calling super functions from Enemy class



