can someone tell me why this always fails?
it isn't garenteed to always reach 63,63 even through i floor it.
function main() domain=true while domain do cls() spr(16,4,0,15,2) for i=0,8 do star() end yield() end end function star() dostar=true inst = { sx=rnd(127), sy=127, ex=63, ey=63, } while dostar do local dx=flr(inst.sx)==flr(inst.ex) local dy=flr(inst.sy)==flr(inst.ey) rectfill(0,0,127,24,0) print(tostr(dx)..","..tostr(dy),0,0,7) if dx and dy then dostar=false else pset (inst.sx,inst.sy,7) print(flr(inst.sx).."/"..flr(inst.ex),0,8,7) print(flr(inst.sy).."/"..flr(inst.ey),0,16,7) inst.sx+=(inst.ex-inst.sx)*mainstarspd inst.sy+=(inst.ey-inst.sy)*mainstarspd end yield() end end |


is it possible to create a await function?
for example..
if await(60,"my identifer") then --done end |
here is my code
function await(t,id) if not cofun[id] then --don't know how to return true here add(cofun,cocreate(function() while t>0 do t-=1 yield() end end)) else return false end end function coupdate() for c in all(cofun) do if costatus(c) then coresume(c) else del(cofun,c) end end end |
EDIT: idk if this is possible but maybe something like this..
--id is determined by function execution index if await(60) then --done end |
for example..
await(60)--await id 0 await(60)--await id 1 await(60)--await id 2 --nextframe await(60)--await id 0 await(60)--await id 1 await(60)--await id 2 |



can someone tell me how to fix this bug?
function range(low,high,step) local t={} for i=low,high,step do add(t,flr(i/step)*step)-- end return t end |
for example I am doing this for my menus..
function run_menu1() scene.upd = upd_menu1 scene.drw = drw_menu1 --options selectors optiony=1 --optiony options= { --optionx and options {1, {false , true }}, --powerups {1, {"grow", "infinite" }}, --tail type {42,range(-4.0, 4.0, 0.1)}, --player speed {42,range(-4.0, 4.0, 0.1)}, --player accel on any deaths {1, range( 0.0,32.0, 1.0)}, --border size {42,range(-4.0, 4.0, 0.1)}, --border speed {42,range(-4.0, 4.0, 0.1)} -- border accel on any deaths } end |
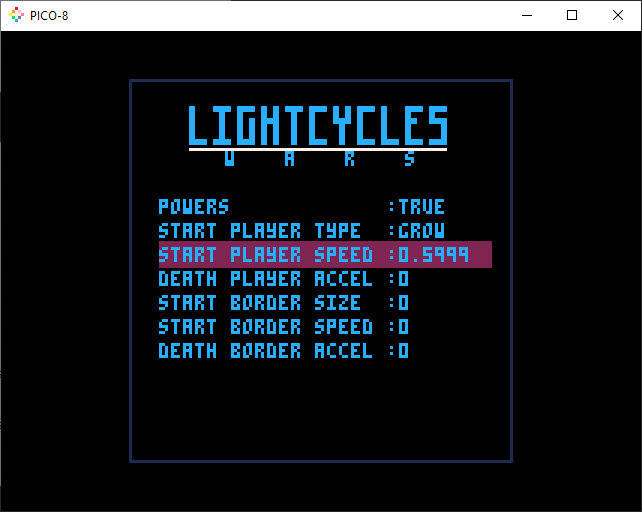
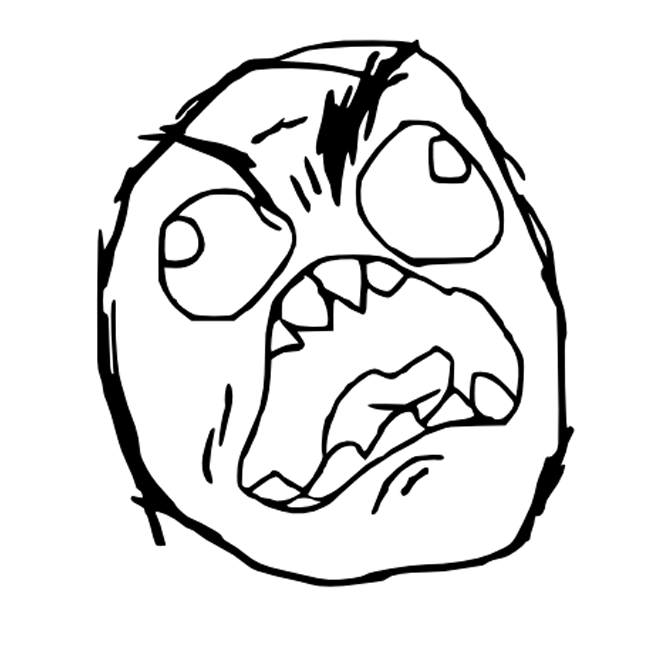



can someone explain to me how to get a sprites bounding box?
I have this code which reads from the center of a sprite and keeps checking above it until the pixel is 0 or its at the top of the sprite.
function boxt(s,x,y,w,h) local sx1=flr(s%16)*(w*8) local sy1=flr(s/16)*(h*8) local sx2=sx1+(w*8) local sy2=sy1+(h*8) local cx =4 local cy =4 //find 0 pixel or top while sget(sx1+cx,sy1+cy) != 0 or sy1+cy > sy1 do cy-=1 end sspr(sx1,sy1,sx2-sx1,sy2-sy1,x+cx,y+cy) return cy+y end |
however it doesn't seem to work
local s,x,y=22,63,63 function _draw() cls(1) if(boxt(s,x,y,1,1) > 0) then y-=1 end //spr(s,x-4,y-4) end |
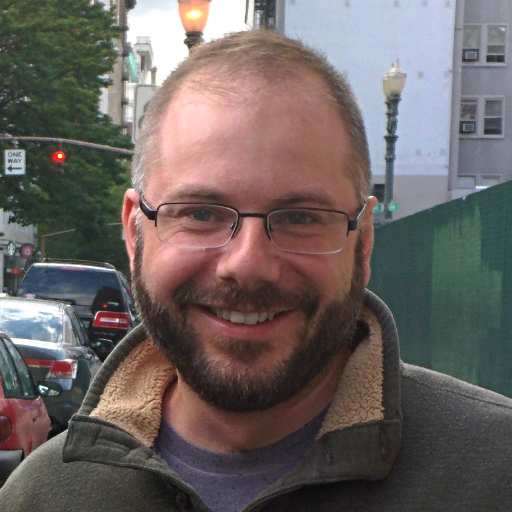

can someone tell me why I am running out of memory?
@Catatafish I completely changed the question I realized i do not have an infinite loop. I just assumed since I was running out of memory.
this line seems to be doing it and idk why..
o:procx() |

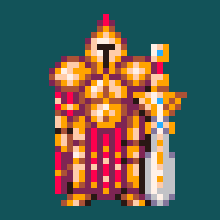
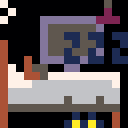

can someone tell me why this throws the error "attempt to call global vec a table value"?
vec clearly has its metatable set with a call metaevent
vec= { x=0, y=0, mag=function(o) return sqrt (o.x^2+o.y^2) end, ang=function(o) return atan2(o.x, o.y ) end, nrm=function(o) return vec(o:ang()/360) end, } vecmeta= { __call=function(o,x,y) o =o or {} o.x=y and x or cos((x+90)/360) o.y=y and y or-sin((x+90)/360) return setmetatable(o,vec) end, __eq=function(a,b) a=type(a) == "number" and vec(a,a) or a b=type(b) == "number" and vec(b,b) or b return (a.x==b.x and a.y==b.y) end, __add=function(a,b) a=type(a) == "number" and vec(a,a) or a b=type(b) == "number" and vec(b,b) or b return vec(a.x+b.x,a.y+b.y) end, __sub=function(a,b) a=type(a) == "number" and vec(a,a) or a b=type(b) == "number" and vec(b,b) or b return vec(a.x-b.x,a.y-b.y) end, __mul=function(a,b) a=type(a) == "number" and vec(a,a) or a b=type(b) == "number" and vec(b,b) or b return vec(a.x*b.x,a.y*b.y) end, __div=function(a,b) a=type(a) == "number" and vec(a,a) or a b=type(b) == "number" and vec(b,b) or b return vec(a.x/b.x,a.y/b.y) end, } setmetatable(vec, vecmeta) local test = { pos=vec(0,0), vel=vec(0,0), } |
can someone tell me why I am getting nil when printing out the stars px and py values?
local stars={} for i=0, 16 do add(stars,{x=rnd(128),y=rnd(128),px=x,py=y,c=7}) end --px and py are equal to x and y drwmenu=function() if btn(2) then menuind-=1 end if btn(3) then menuind+=1 end menuind=mid(0,4) for v in all(stars) do print(v.px) --prints nil print(v.py) --prints nil line(v.x,v.y,v.px,v.py,v.c) --line(v.x,v.y-128,v.px,v.py,v.c) --v.py=v.y --v.y+=8 if v.y > 127 then v.y-=128 end end if (time()-last > 1) then rectfill(0,112,127,120,1) print("press start!",0,114,7) if (time()-last > 2) then last=time() end end end |


does yield() work outside of coroutines and inside functions?
I feel coroutines are a pain to setup and update when it would just be easier to call yield inside a function
not to mention the tokens required to setup coroutines and call/update them
function wait(t) for x=1,t do yield() end end function test() wait(2) print("test") wait(2) end function _update() test() end |


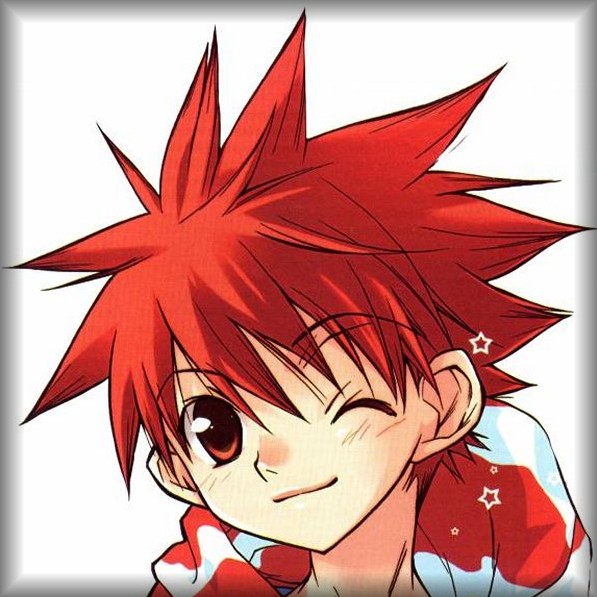

EDITED 2/14/2020
I came up with several functions that I think would go good in pico8 and would reduce file size for larger games
--math
function ldx(d,l) --stands for lengthdirx return cos(d)* l or 1 end |
function ldy(d,l) --stands for lengthdiry return sin(d)* l or 1 end |
function ptl(x1,y1,x2,y2) --stands for point length return sqrt(((y2-y1)^2)+((x2-x1)^2)) end |
function ptd(x1,y1,x2,y2) --stands for point direction return atan2(y2-y1,x2-x1) end |
function wrp(v,l,h) -- stands for wrap local o=v while(o < l) o += (h-l) while(o > h) o -= (h-l) return o end |
function rng(v, l1, h1, l2, h2) -- stands for range return l2 + (v - l1) * (h2 - l2) / (h1 - l1) end |
--vectors
function vec(vx,vy) --stands vector local m= [ [size=16][color=#ffaabb] [ Continue Reading.. ] [/color][/size] ](/bbs/?pid=55218#p) |
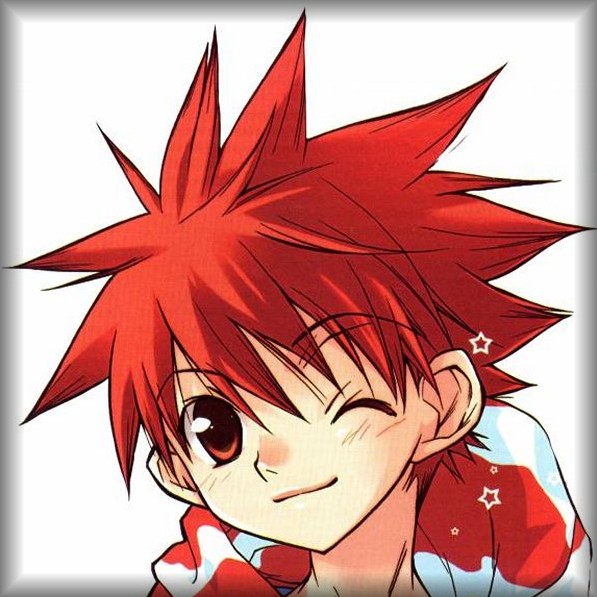
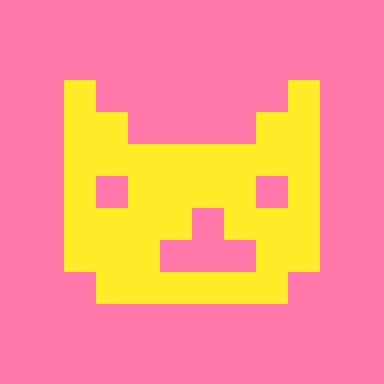

is there a plan to add built in pico8 multiplayer using synced inputs?
basically it would be handled in pico8 rather than the cart.
it would allow players to connect to each other via ip or game uid and official lexaloffle connecting servers through the pause menu.
this might be the easiest way to build a multiplayer game since there would be no packets being sent besides the hard coded inputs.
networked inputs would be accessible the same way normal inputs are with btn(btn, player).
this would also make multiplayer games have not just networked players but local ones as well.
players count would be limited to the number of inputs pico8 has.
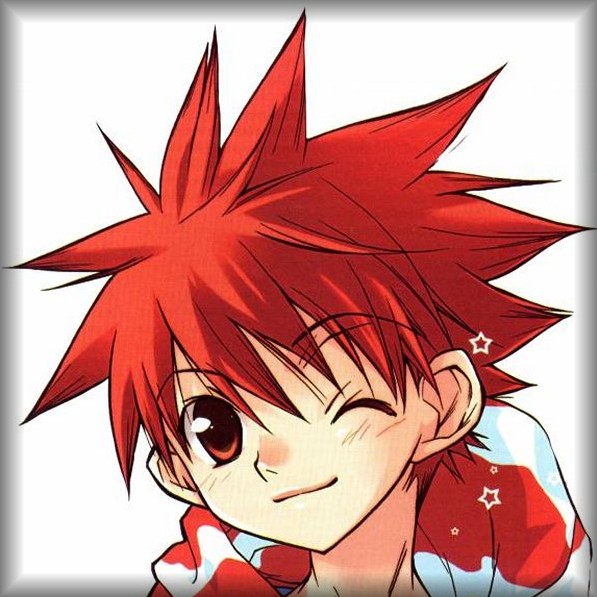


ok so I think I get how people like pico 8 for its limitations. but will pico 8 ever support 4 players simultaneously?
this would be good for things like raspberry pi where 4 players can sit on a couch and play small 4 player games together.
what do you guys think?
I know older hardware only supported up to 2 players but I think this would be a good idea for the compactness of pico8.


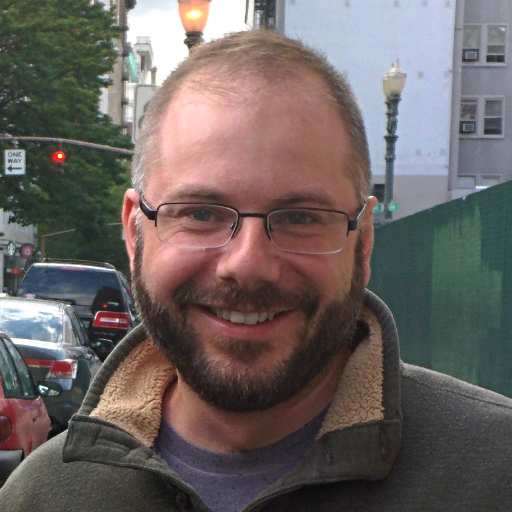


I was just wondering everybody's opinion on the limitations of pico8?
right now there isn't really much we can do with it besides make very simple 1 level games.
pico8 could do much better if code limitations weren't so harsh.
also it seems people smash all there code together to preserve tokens which is not good
for example..
this takes up more space
local var = 0 var = var + 1 |
then this
local var=0 var=var+1 |
which makes no sense
if code limitations were removed and cart loading time was reduced significantly
then pico8 could make much larger games while still saving to a small cart size.
what would even be better if pico 8 carts were separated into 2 parts
- the compiled game
- and the dev files
so what do you guys think?
keeping the games small maybe be fun but you can only do so much with it especially with the severe limitations of pico8.
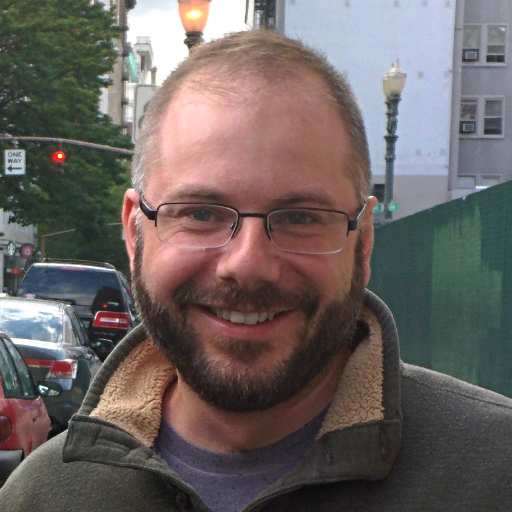








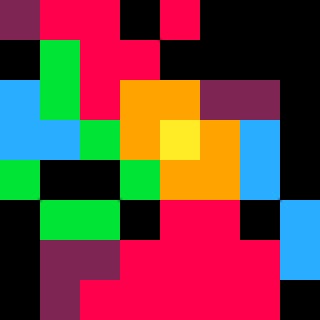