My submission for code guessing round #43. It's a visualisation of a spirograph with adjustable parameters:
- R1: radius of the blue, centered circle
- R2: radius of the white, rolling circle
- D: length of the drawing rod coming from the rolling circle
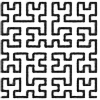
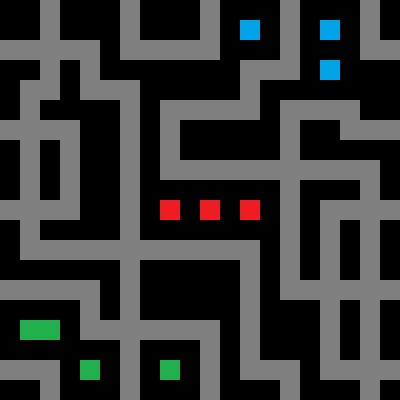
It's a very minor issue but may be important for some carts. In the web version of PICO-8 using menuitems in the pause menu causes _update
and _draw
to be called.
Version: 0.2.4c4 web applet
How to replicate
Make a cart that has an _update
function defined that will cause a visible change on screen. Define a menuitem with a callback that returns true
so that the menu stays open after the menu item is used. Run the cart, pause it and use the menuitem.
Expected behavior
The callback runs and the state of the program is only changed in the way the callback changed it. (This is waht happens in the full desktop app version 0.2.4)
Actual behavior
A frame progresses before the callback is resolved. The calling order seems to be: _update
, _draw
, callback.
Examples:
Simple example:
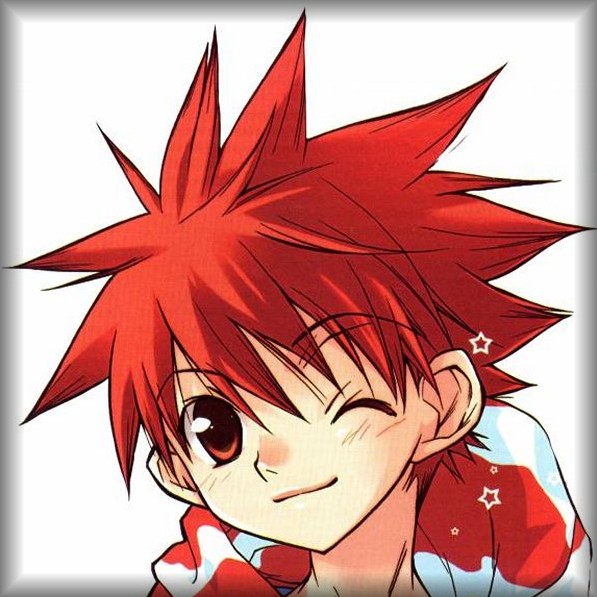
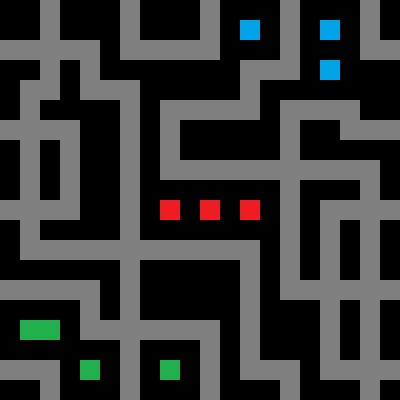
This is an implementation of an algorithm that plays snake very well. It's based on a path through all board cells and calculated shortcuts to get more efficiency.
Controls:
X - show/hide the path
Menu items:
ALG - controls which variant of the algorithm is used.
SPEED - Snake's speed, lower = faster.
SNAKE - Change snake visualisation.
The path was generated based on a Hilbert curve. It would work with any path that goes through all board cells, but the Hilbert curve has some nice properties and looks.
I have implemented two variants of this algorithm here, togglable in the menu. "Tail" algorithm tends to follow it's tail very closely when it can't get directly to food. "Blocky" algorithm curls on itself until it has a clear path to food resulting in big patches of snake body later in the game. I believe "Tail" is more efficient than "Blocky".

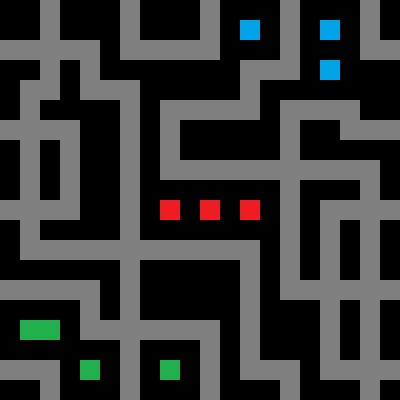
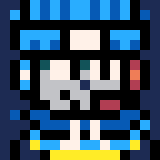
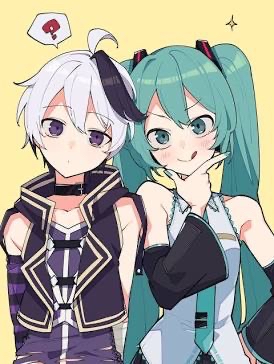
Instructions
Left/Right - change real part of the parameter
Up/Down - change imaginary part of the parameter
X - redraw with new parameter
Z - show/hide the parameter
Explanation
This is a visualisation of what is (wrongly*) called Julia sets after a French mathematician Gaston Julia who devised the formula governing them. They are drawn by assigning each pixel a complex value based on its coordinates and looking at a certain sequence based on that value. If the sequence shoots off to infinity in either direction the pixel is coloured based on how quickly it shoots off. If it stays bounded it is colored black.
The formula for the sequence is
[ [size=16][color=#ffaabb] [ Continue Reading.. ] [/color][/size] ](/bbs/?pid=99606#p) |


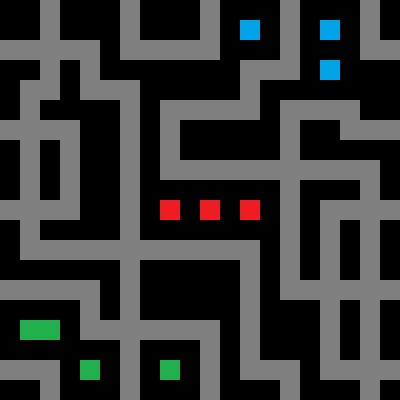
Dijkstra Map visualizer
While trying to follow a talk on map generation on youtube I created some demos. Here is one that is most functional and most pretty so far.
Controls:
- Arrows: move the cursor
- Z: Calculate a new map starting from the cursor
- X: Generate new cave
Explanation:
Dijkstra maps contain information about distance from each point on the graph to a set starting point. In this case the graph is a generated cave-like map and the point is picked at random, but can be changed with arrow keys + Z.
After the Dijkstra map is calculated the cave map is colored like this:
- Walls are black.
I just found that the Shift + Enter shortcut doesn't work after the 'else' keyword. I know it was supposed to be context aware, I see it properly adds 'until' after 'repeat' which is nice, but this case seems to have been omitted in the update which makes coding slightly annoying.
I'm not sure if this is a bug or a (not so useful) feature, because it's consistent with the changelog which states that as of 0.2.2b:
> shift+enter in code editor only auto-completes block for DO, THEN, REPEAT or FUNCTION
and not ELSE. If this is intended I'll move the post to the workshop section as a feature request.
Version: 0.2.2c
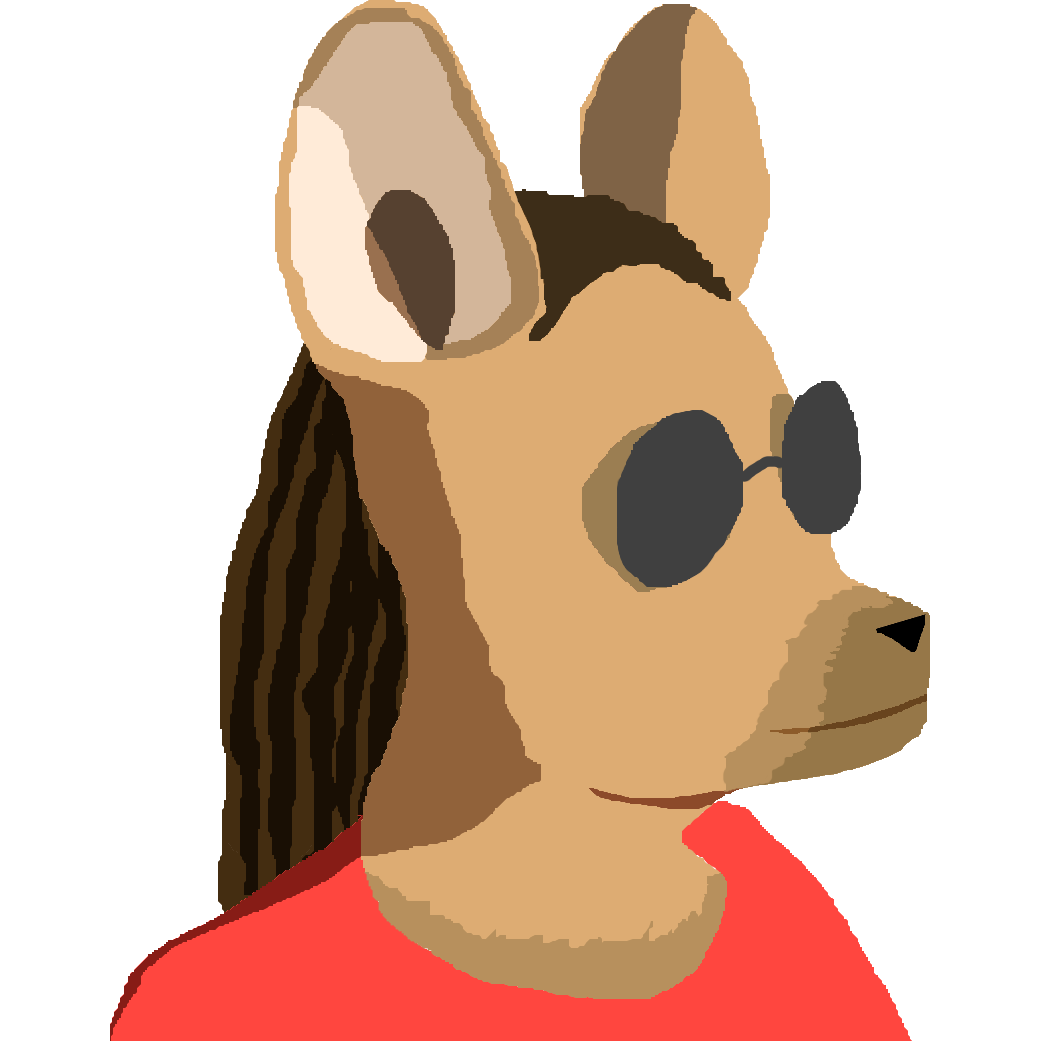
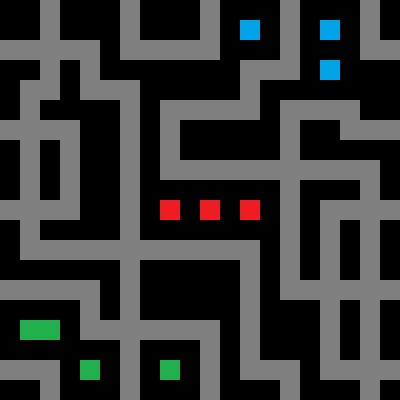
On my linux machine I use a window manager that swaps between tabs using win + tab number. PICO-8 treats win same as ctrl and if I have PICO on tabs 6 through 9 it makes screenshots, gifs and label images when I switch to (or sometimes from) it. In config there's an option to disable this functionality with function keys F6 through F9, but I would like it to work ONLY on function keys or just not recognise win as ctrl, though I might be the only one. Til then I'll keep PICO-8 on tab 5.
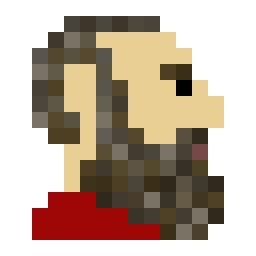
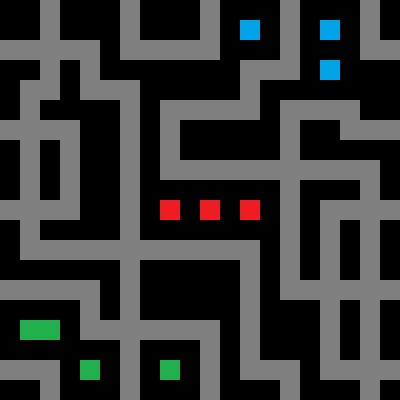
Example
Controls
Up/Down - change number of pixels drawn per frame by 20
Steps to reproduce
Write a program that runs nested coroutines when the frame end takes place.
Resulting and expected behavior
All coroutines except from the top one yield().
It's expected that coroutines resume normal work after frame end.
Example explanation
It's a program supposed to color the whole screen generating coordinates to color with a recursive coroutine (tab 2, "coroutine itself"). It is wrapped by a function that ensures all errors will be propagated and unwraps the output when no error is encountered (tab 1, "coroutine wrapper").
In pseudo code:
function coroutine(depth) if (depth == 0) yield{0,0} return c = cocreate(coroutine) x,y = wrap(c, depth - 1) -- a wrapper around coresume while x do -- this assumes that if x is nil then the coroutine yield{x,y} -- has reached a return or end. x,y = wrap(c) end c = cocreate(coroutine) x,y = wrap(c, depth - 1) while x do yield shifted {x,y} x,y = wrap(c) end end |
Info written on screen:
- Number of pixels coloured/number of pixels on screen
- Number of pixels coloured per frame (adjustable with Up/Down)
- Number of calls to subroutines of certain depth/expected number of calls
Failed workarounds
Adding a global table to hold recursive coroutines changes nothing.
Working workarounds
Limit the number of coroutine calls per frame with flip() outside the coroutines.
A wrapper that catches the nil yield and checks if the coroutine is dead. Doesn't work on coroutines that are supposed to yeld() or yield(nil)
WARNING! Skipps some coroutine outputs if flip() is called.
old_coresume = coresume function coresume(c, ...) flag, out = old_coresume(c,...) if not flag or out != nil or costatus(c) == 'dead' then return flag, out -- coroutine raised an error, yielded a non-nil value or finished else return coresume(c) -- resume from the unexpected yield() end end |
The example using an equivalent wrapper:
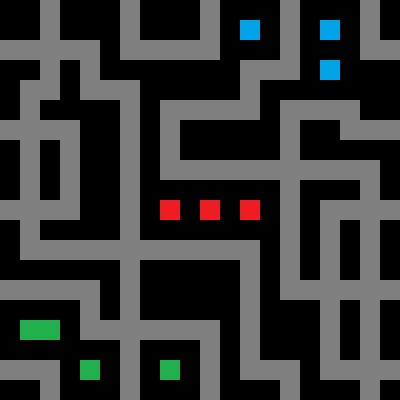


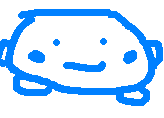
I tried making a 60 FPS game and noticed a weird behavior of my PICO-8: it's refresh rate stayed at 29-30 FPS even though the game was supposed to be running on 60 FPS according to stat(7) and CTRL-P widget. Movement looked choppy and the builtin FPS counter (stat(9) and show_fps config) shown 29-30 FPS. My monitor is capable of 60hz refresh rate and my CPU and GPU usage wasn't even remotely close to 100% (or even 8%, which is half of a processor core and can be a limit for single-threaded apps). It works properly on the web version.
Pls halp!
P.S.: It works just fine on Linux on the same machine with the same cart and default config.
Relevant config:
show_fps 1 foreground_sleep_ms 5 background_sleep_ms 10 host_framerate_control 0 |
Some stats and readings:
stat(7) and CTRL-P: 60/60 stat(9) and show_fps: ~30 stat(1) and CTRL-P: ~0.3 CPU usage: ~7% GPU usage (3D): ~30% |
Soft/hardware:
PICO-8 v.0.2.2 [ [size=16][color=#ffaabb] [ Continue Reading.. ] [/color][/size] ](/bbs/?pid=87885#p) |
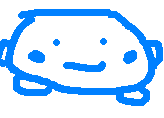
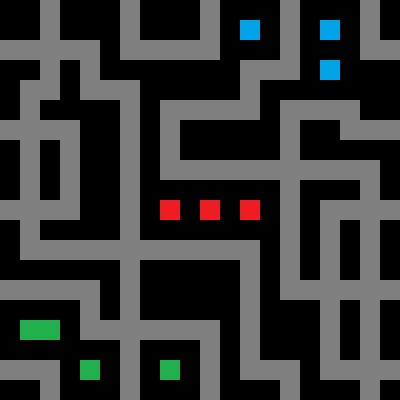

Controls
Arrows to move cursor
X to place mark/go level deeper
Hold Z for 1 second to restart game
Press Z to recreate demo in demo modes
Open pause menu (Enter/P) to:
- enable demo modes (auto - computer vs computer, fill - random pattern)
- switch between single and multiplayer
- open settings menu (this will restart the game!)
Settings in settings menu:
- meta level: ranges from 1 (normal tic-tac-toe) to 4 (81x81, four levels of nested boards)
- AI difficulty: how long in seconds will the AI think about the move. 0 is random moves, 3 never loses normal tic-tac-toe.
- game modes: singleplayer, multiplayer, automatic (AI vs AI) or randomly fill board.
Rules
Each board contains 9 a smaller boards. The lowest meta boards are just normal tic-tac-toe boards where you can place your signs. Where you move in the lowest board controls on which higher board your oponent can make their next move (indicated by the colored squares). Winning a lower board replaces it with a sign in a higher board. Win the highest board to win the game.
If you didn't understand that's my fault, I suck at explanations. Try it for yourself at small meta levels, it's not as complicated as it seems.
Changelog
Version 1.6:
- 4th meta level
- settings menu
- endgame sonuds
- changed AI
- bugfixes
Previous versions:
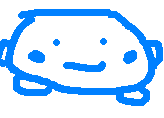
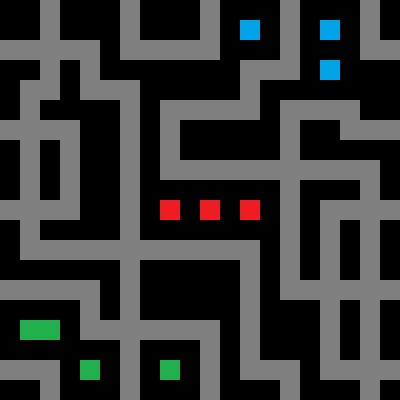
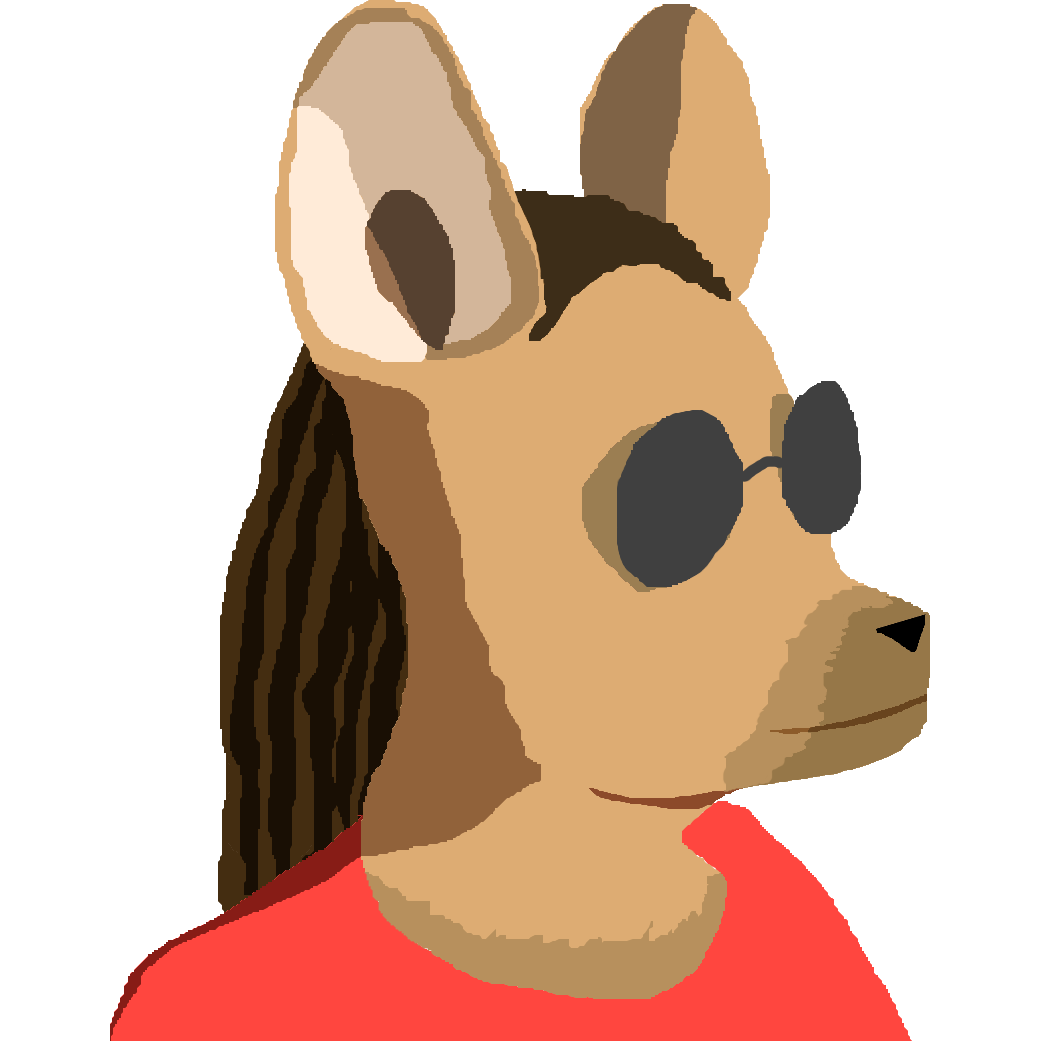

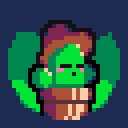
Expected behavior
When running any cart on BBS pressing CTRL-V makes stat(4) read pasted content.
Actual behavior
If the first cart that is run after loading the page is not the top cart then stat(4) fails to read pasted content regardless of which cart is run, even if the top cart is run later.
Environment
Firefox 83.0
Windows 10
Example
All three example carts are the same cart uploaded three times as three revisions to prevent another BBS quirk, which may or may not be a bug.
Run this first and it works:
Run this first and it breaks:
While trying to make a "class" (metatable + constructor function) for big integers I stumbled upon a weird error. It went off a couple of times before, but I decided to finish debugging the "class" before I report it in case it went away. It's not a memory error or a stack overflow, because they get reported properly. I don't use any actual coroutines, nor you will find "yield" anywhere in my code. The code itself is a bit computation and memory heavy, there's probably a better approach to this, but none of it justifies such an error. Another thing I noticed is that it breaks on seemingly random lines, and almost any change to the code makes it change the line it reports as the source of the error. It even breaks in a different spot on the web than on my computer! (look at the line shown on the thumbnail vs what actually happens when you press and hold X)
The showcase is a simple program showing more and more powers of 15 as you press X. An interesting fact is that it breaks on different values depending on wether you press-and-hold X or repeatedly press X. It's all very confusing.
Advent (of code) is here!
On 1st December every year since 2015 starts the best advent calendar: Advent of Code! It's a series of 50 small programming challenges that get progressively harder every day and can be solved in any programming language!
Quote from the creator, Eric Wastl:
Advent of Code is an Advent calendar of small programming puzzles for a variety of skill sets and skill levels that can be solved in any programming language you like. People use them as a speed contest, interview prep, company training, university coursework, practice problems, or to challenge each other.
I can't find any mentions about AoC on BBS which is a true shame, because PICO-8 is perfect for solving and visualising AoC puzzles! (At least the first ones.) I wanted to share this awesome contest with you and in this post I will be including carts with my solutions for as long as I can keep up. GL;HF!
If you want to compete here's my private leaderboard code: 979701-cf5ded3a
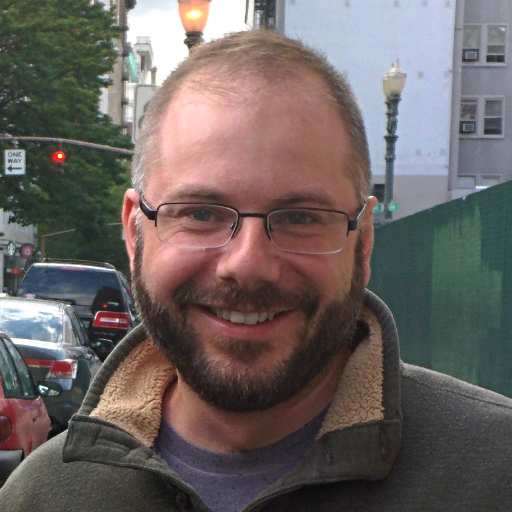

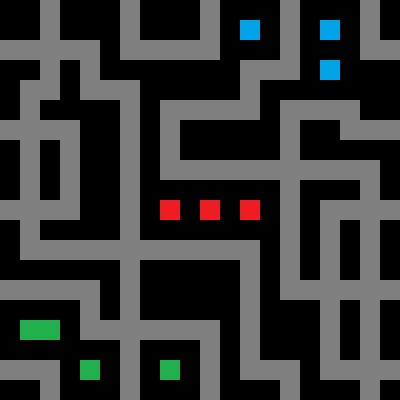
I found a cool cave generation algorithm and I'm building a game around it. Currently you can only explore a single random level with 100 bats that fly randomly.
Features:
- random 128x64 map that wraps around
- rendering y-sorted mobs
- Kate, who can run, dash and stand still
Todo:
- damage system
- attack for Kate
- better sprites for the level (pls help, I suck at esthetics)
- progression
- more enemies
- enemy AI
- collision detection between mobs
- more sounds
- and a LOT more
Any feedback or suggestion are appreciated.
Versioin history:
1.1 (latest)
- dash
- stamina
- less noisy walls
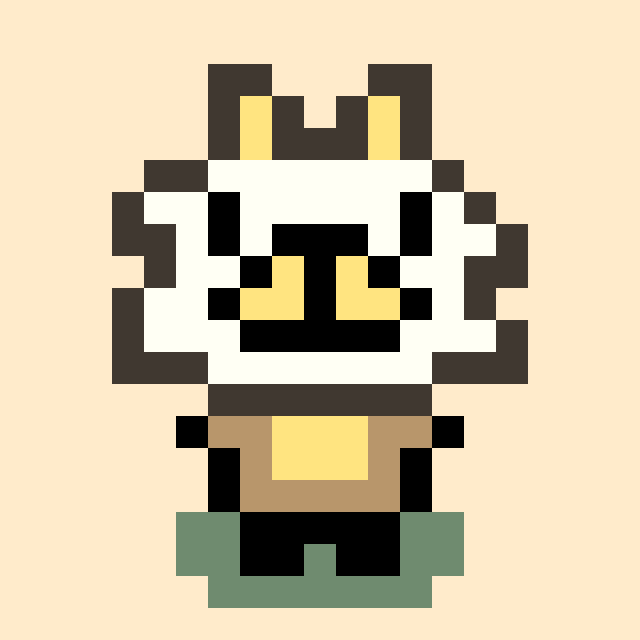

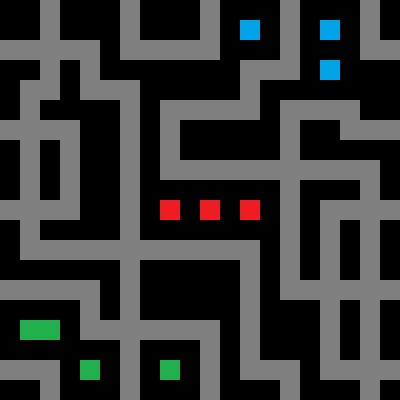
ladies and gentelmen, behold the thing no one asked for, the pinnacle of console and cart design alike, my opus magnum:
Brainf**k Fantasy Console!
Have you ever got lost in the many interface options your console provides? Have you ever felt overrun with the abundance of commands included in normal programming languages that everybody knows? Do you feel flabbergasted looking at the vibrant interface colors of the mainstream fantasy consoles? My newest addition to the market has you covered! It has:
- A full-featured memory editor optimised for the purpose of programming the BFC!
- A brand new programming language based on the popular Brainf**k!

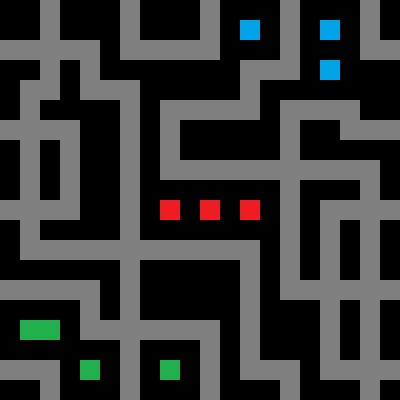
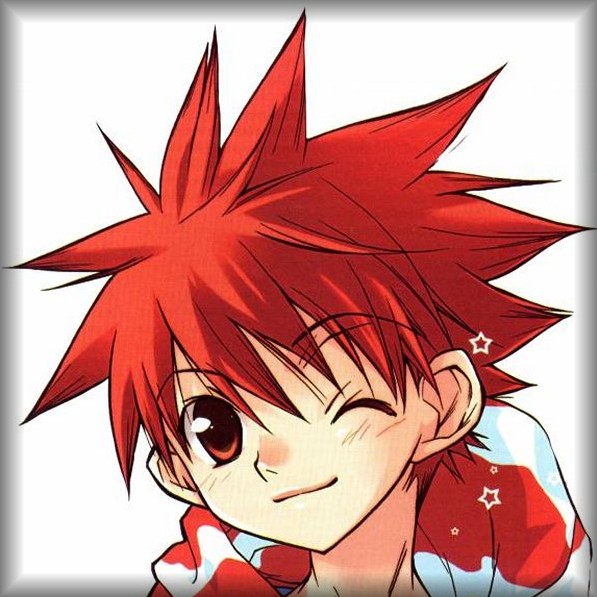

A small showcase of how to generate random 2D caves using a cellular automaton. Inside the code there are some parameters worth tweaking, maybe I'll add an interface for this in a later update.
The automaton is really simple: it changes based on the majority of it's neighbours and remains unchanged when tied.
Parameters:
- width and height - self explanatory, cave will be displayed in the upper-left corner
- live and text color - coloring of the cave and the "reset" message
- iterations - more iterations of the automaton mean less small noise and more smooth walls. Over 20 will not change much.
- density - chance of a cell being dead at the start. Very susceptible to change, more will create wider corridors.
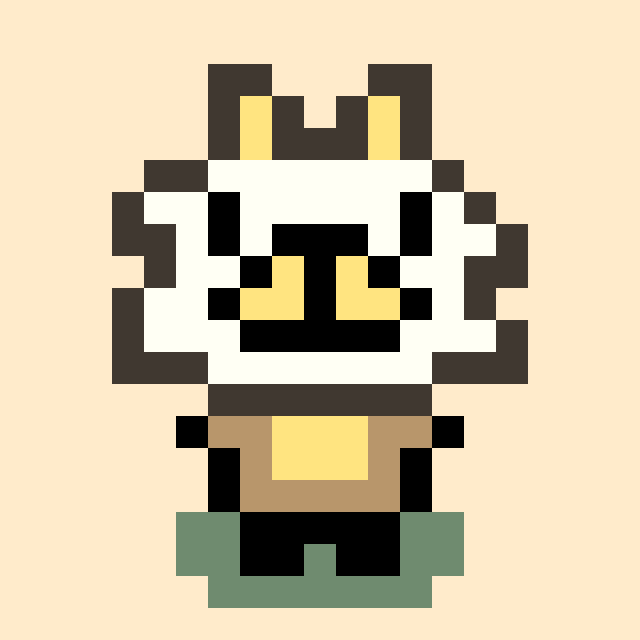
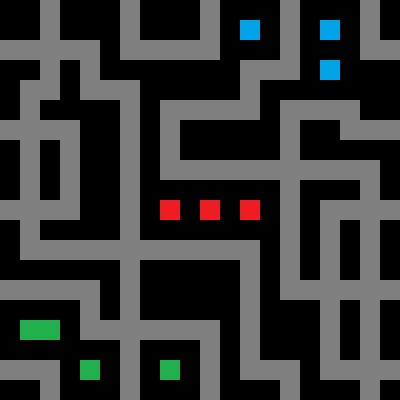