Intro
What if there was a fantasy console in a fantasy console? That's a question that I never got. Instead, I was curious to see if I could come up with a way to get some form of programming on Pico-8.
Well, here you go. The language resembles CPU instructions. The fantasy console allows up to 256 instructions in ROM and has 256 byte RAM which can be viewed at runtime.
The console itself comes with a 16x16 1-bit display and 6 buttons. All IO can be directly accessed and manipulated through RAM. For convenience, it's marked with green (display) and yellow (input) in the editor.
Also, I came up with this idea due to the Ludum Dare theme "The more you have, the worse it is". I actually did it during Ludum Dare and finished it in time. I guess it's an unofficial Ludum Dare entry :-)
[b]Usage



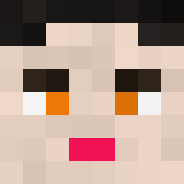


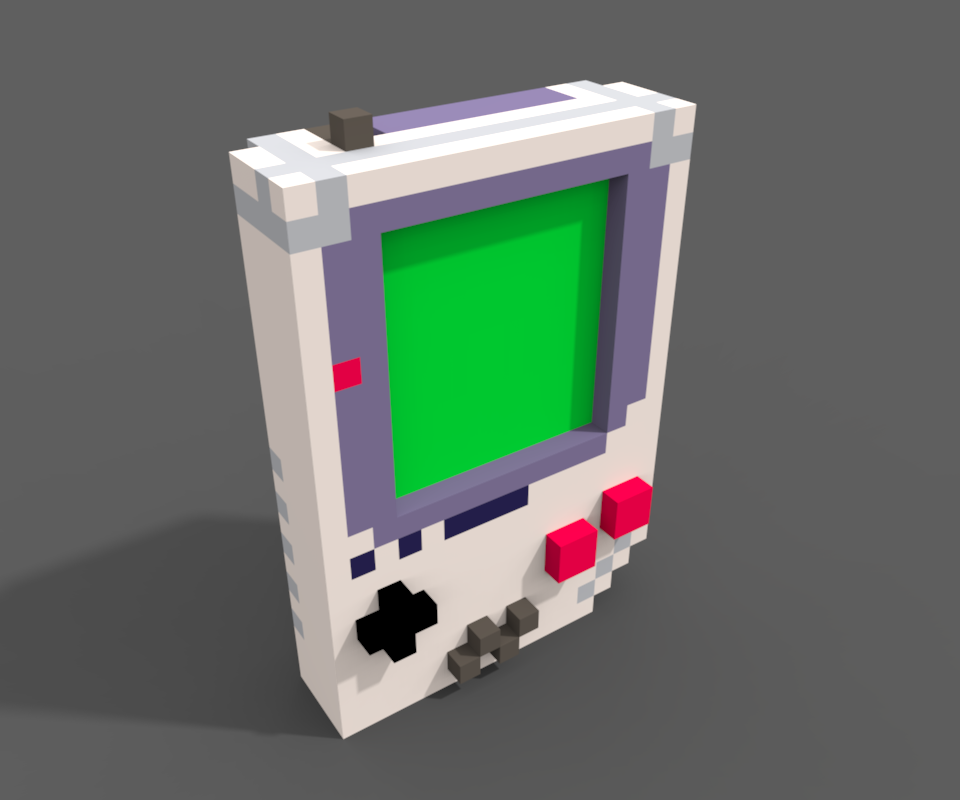
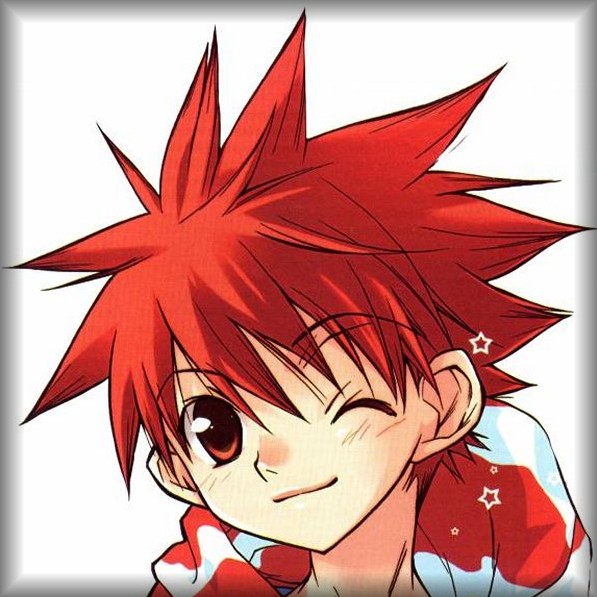
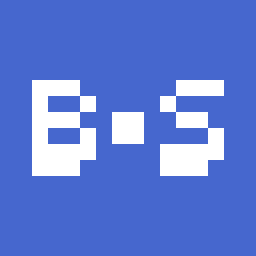
Recently I've worked on 2 little libraries. However, I'm not sure how I feel about the token count in these cases and I'd love to discuss that with the community.
Libraries can provide a piece of functionality that they would have otherwise programmed themselves. Someone creates a library and other people can use it in their games.
But unfortunately I feel like it's simply not worth it to ever use a library with Pico-8. Why? Because of the token count.
In pretty much every instance you're better off writing a piece of code yourself then using someone else's library. You may not need specific features from a library but using this library will still cost you those tokens.
What are your thoughts about this? Would you use a library? Or maybe you have a smart idea of dealing with this limitation.




Walk around with WASD. You can enter the house near the old lady.
Pico Crossing is (as the name implies) inspired by games like Animal Crossing and My Sims.
I've been working on this game for a few days now and a lot of the basic systems are in place now.
If everything goes to plan I have a pretty sweet never-seen-before feature high on my priority list which will allow players to interact with other players which should keep the game interesting to play for a longer period of time than a single play session.
More on this later in the future.
Planned Features
- Buy furniture for your very own home.
- Collect/harvest items to trade with other villagers.
- ??Super cool secret feature??

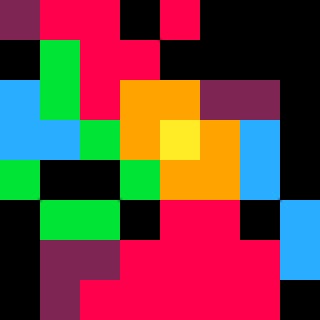
Dialogue Text Box Library (DTB)
DTB is a code snippet that you can use to implement a dialogue text box to any game in just seconds.
Setting it up goes as follows:
- Add the dtb code to the top of your own code.
- Call dtb_init in your initialization.
- Call dtb_update in your update.
- Call dtb_draw function in your draw.
And that all there is! You can now simply call "dtb_disp" wherever you want.
Usage
dtb_init(numlines) |
Call this to initialize all dtb's variables. Takes an optional parameter which defines the maximum number of lines that will be displayed. Defaults to 3.
dtb_update() |

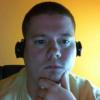

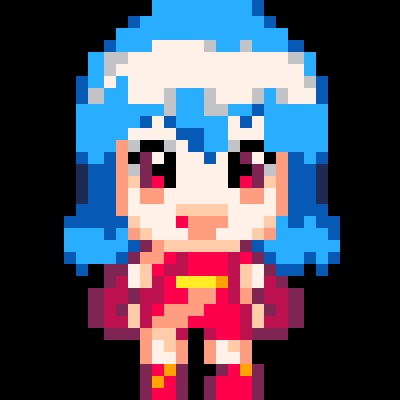
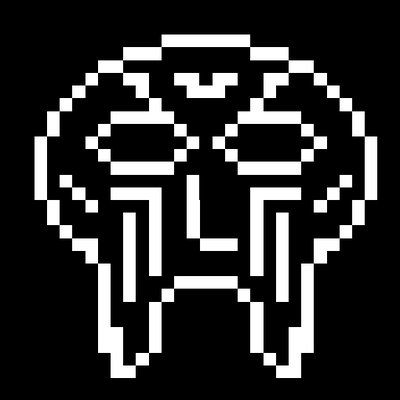




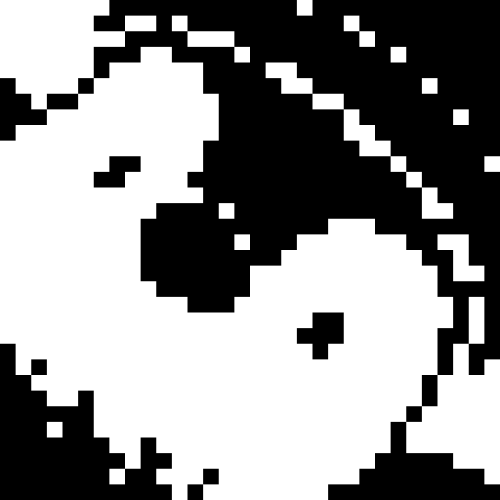
Demo Controls:
Position the pointer in one of the 4 quarters using the arrow keys to change the music.
The music will change depending on which quarter you're in. The check marks in these quarters show which channels are enabled for each quarter.
The changes will go into effect as soon as the pattern ended.
Background Story:
I came up with the idea of having the music chance depending on where you are while still keeping the same song. I quickly ran into an issue. I realized that there was no way to make this happen using just the "music" function.
Later that day I found out about the "peek" and "poke" functions: allowing you to directly read and write to ram. I found out where to find the music pattern section and started mapping it out to get more control over it.
[b]Music in RAM: