Here's a small code snippet that allows you to enter "debug mode" and then go frame by frame through your game.
The idea is, that _update()
wraps the actual update()
function, and _draw()
wraps the actual draw()
function.
So you can have extra output only drawn in debug mode, and go frame by frame through the game to do some bug hunting.
It would also allow to append to a debug file only if debug mode is enabled.
It's pretty simple code, but quite helpful.
To switch to release mode, all you need to do is to swap the names of _draw() and draw() and _update() and update().
function _init() poke(0x5f2d, 0x01) debug=false waitframe=false t=0 end function _update() if stat(30) then local ch=stat(31) if ch == "d" then if debug then waitframe = false debug = false else debug=true end end if debug and ch==" " then waitframe = false [ [size=16][color=#ffaabb] [ Continue Reading.. ] [/color][/size] ](/bbs/?pid=113573#p) |
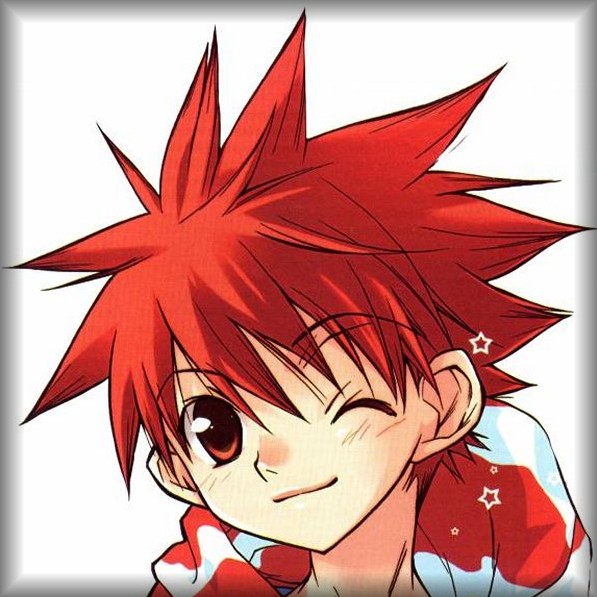
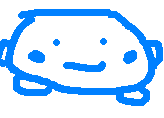
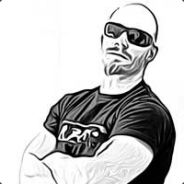
This is a very small arcade game for a game jam.
Theme was "paranormal Activity"
In this game you're a wraith. Get close to those pesky humans to suck out their life force.
Move to the magic circle to feed the portal the life force you gathered.
Avoid those priests...
Spent roughly a day, so don't expect too much :)

Inspired by @slainte 's post, I wanted to code a simple isometric map renderer with height information.
This is my first try - feedback on tiles and general how to approach this stuff is very welcome.
Right now the height is bruteforced rendered by overlaying the tile sprites height times. This could be optimized by checking the surrounding height and not drawing the area that will be painted over anyway.
Feedback very welcome :)
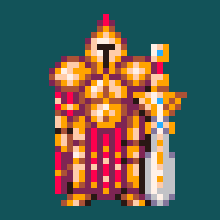
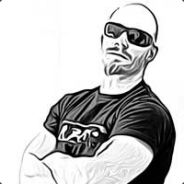


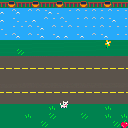
I took the wander demo and changed it a bit by making it slightly more "frogger"-y. I thought it might be fun if somebody else would grab the cart and add something else to it, until we have a pico8-kitty frogger.
:)
New version below, this is the first version
This is a remake of a game I coded mumble years ago with allegro.
It's still very much WIP, I started today and just got the basics working and some rough sprites drawn.
You can switch from joypad to mouse using a flag in the the code - which I would recommend if you can.
I enabled the mouse emulation in this cart, since the manual mentioned problems with mouse support on the bbs.
Issues:
- There is a bug when picking up an atom (it doesn't connect the free electrons to nearby other free electrons)
- in mouse emulation there is no bounds checking
Todo:
- an actual goal for the game
- removing molecules when all electrons are bound

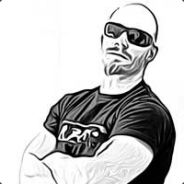
I needed a way to display graphics without using sprites/ or tiles.
So I wrote a small python script that takes an 128x128 image, finds the best matching colors from either the normal or secret palette (or the best 16 colors of both) and stores that in a data string.
If there's interest I can share the python code - main reason I haven't done so is, that I haven't made he python script user friendly :)
No sprites used in this demo.
You can download the image -> string conversion tool and some example code here:
https://github.com/iSpellcaster/pico8rle
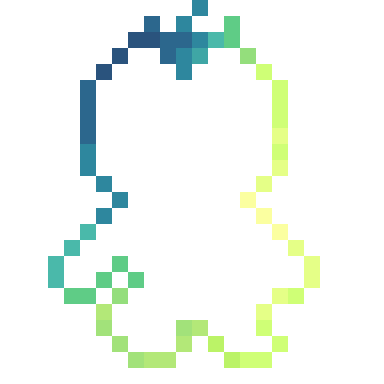
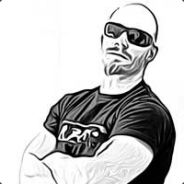
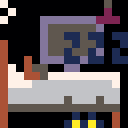


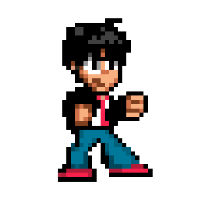

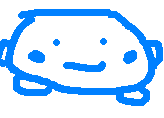
I wanted to create background images w/o having to use tiles/sprites for that.
So I'm wroting a small python script, that takes a 128x128 png, reduces it to the most commonly 16 used colors from the pico8 palette, does RLE encoding on them and outputs the values as a string.
This string can then be imported into pic8 and used to render your background image.
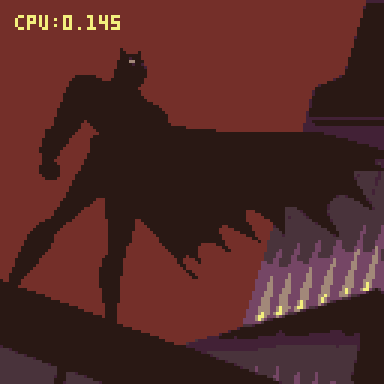
The python part is not yet perfect (I need to write the custom palette generation code), but if you limit it to one of the two pals, it kinda works.
End even when re-drawing the bg from the data every frame, it's kinda ok.
The encoder and the functions to use the result can now be found at: https://github.com/iSpellcaster/pico8rle
Here's the code of the latest version.
Includes base64 encoded of variable sized sprites than can be flipped. Should run after copy and paste, nothing else required.
To encode your own images, check the github link above.
function _init() state = "title" title = explode64(kanji_rle) batman= explode64(bat_rle) antiriad = explode64(antiriad_title_rle) ryu=explode64(ryu_rle) t=0 _draw = draw_title start_t = time() end function _update() t+=1 if btnp(❎) then if state == "title" then _draw= draw_batman setpal(bat_pal) state="batman" elseif state == "batman" then _draw= draw_antiriad state = "antiriad" start_t = time() pal2() else _draw= draw_title state = "title" start_t = time() pal() end end end function draw_title() local i,ofs,y ofs = flr((time()-start_t)*45) ofs2= flr((time()-start_t)*2) --rectfill(0,0,127,127,ofs2%4) cls() y = ofs%250-100 if y > -127 then spr_rle(title,(128-title [ [size=16][color=#ffaabb] [ Continue Reading.. ] [/color][/size] ](/bbs/?pid=79494#p) |

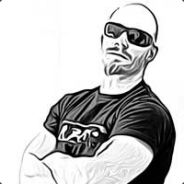

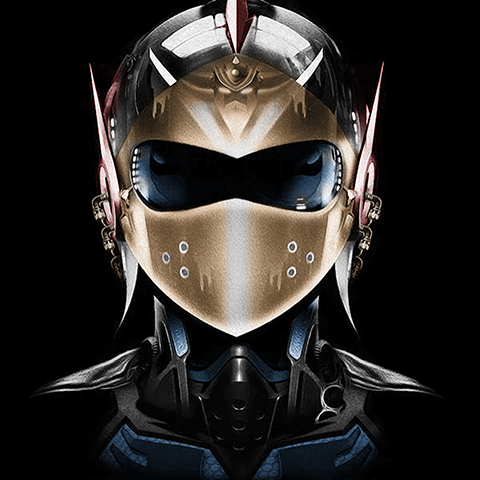
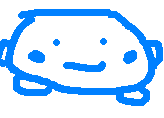
Hello!
just started with pico8, and played around with it.. this is (at the moment) just something to get used to the API. There's a function in there, that might be interesting for some of you, though. It prints a string with different colors per scanline.
function _draw() cls() cprint("colored text",nil,20, {7,11,3}) cprint("pass nil for x to center",nil,30, {12,6,13}) cprint("press ❎ to start",nil,80,{9,10,9}) end function cprint(txt,x,y,cols) local len,org=#txt*4+4,clip() local a x=x and x or 64-len/2 for a=1,3 do print(txt,x,y,cols[a]) clip(x,y+a*2,len,2) end clip(org) end [ [size=16][color=#ffaabb] [ Continue Reading.. ] [/color][/size] ](/bbs/?pid=78976#p) |