OK, I know we can use ternary conditions in variable assignments. But - as far as I can tell - they can't be used in calculations. Having logical statements be used as a value operator in a calculation can be used in many circumstances, would be readable and possibly pretty token efficient.
How might this look?
(a > b) would result in a 1 if true, 0 if false.
something like "pset(100+100*(a > b), 200, 7)" would prevent the use of a bunch of ternary assignments, local variables or if statements.
So what do you think? Useful? Doable? Worth it?
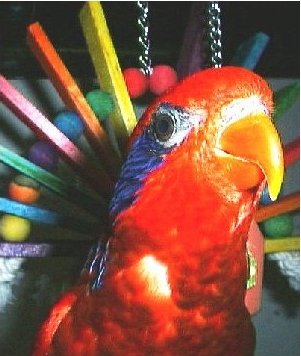
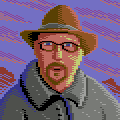
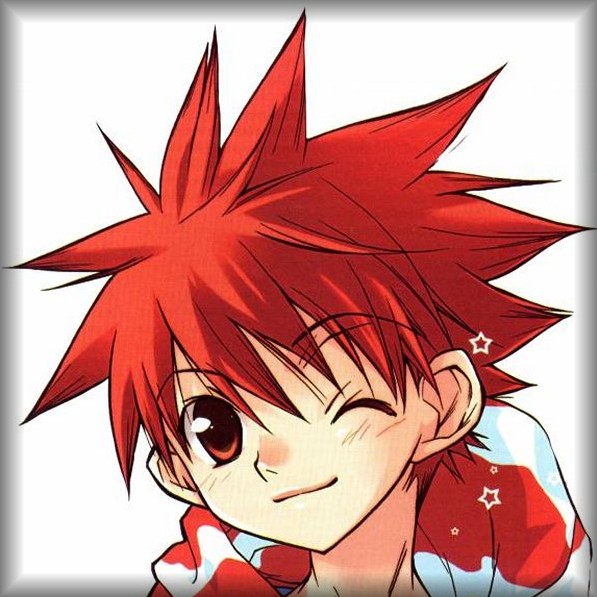


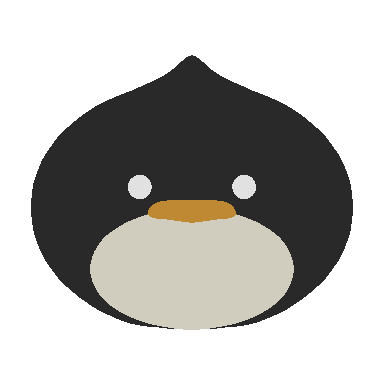

Probably a silly question, but I can't seem to work it out.
I have a slow function - it takes more than 1/30 of second to execute but needs to be done in the _draw() function. This makes my code slow and unresponsive and it's clearly not the way to do it.
So what I want to do is the following:
- run my slow function once in the _init() (drawing a bunch of stuff to the screen)
- capture the screen to extended memory
- in my _draw() function do repeated copies back to the screen
This works ... BUT ... when I run my slow function it shows on the screen (briefly, during _init() ). Probably because it overruns the 1/30 second _draw update time. I would rather suspend draw updates during _init().
So is there a way to suspend screen refreshing for a bit and then switch it back on?

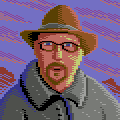

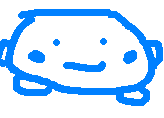

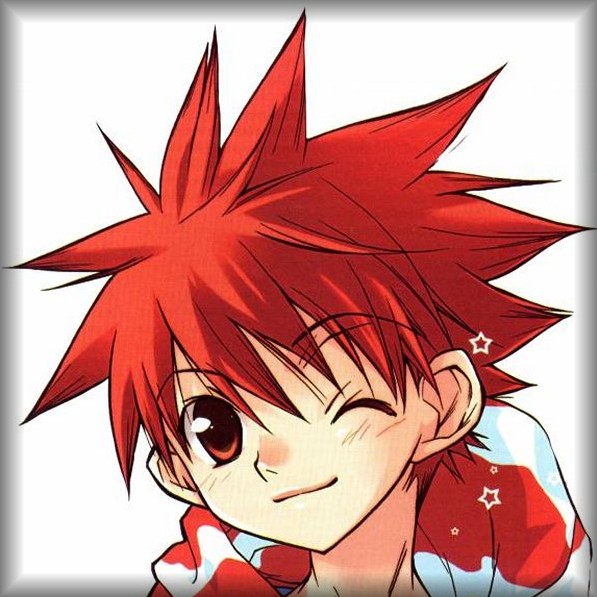
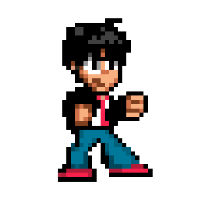
The code below creates a random "word" that's 2 to 12 characters long. Some results are better than others and absolutely no guarantee it won't generate rude words (in whatever language you'd like to be offended in). This snippet might come in handy somewhere and can very easily be tweaked.
Great for all your randomly generated NPC's, Legendary Weapons, Chthonic demons and faraway planet systems.
Very loosely based on Jabbalaci's Python version (https://github.com/jabbalaci/Elite) of Ian Bell's txtelite.c script for Elite.
str_namefrags=split("AB,OU,AA,IT,ION,YL,IF,ON,ETO,AE,ERA,US,EON,ES,AR,UME,IN,AH,ER,AL,UR,AV,ET,IUS,IE,UJ,EY,YS,ON","SE,NYA,HY,SU,LO,NU,GA,LON,TH,NA,HO,LE,XA,DIA,GE,ZA,VAN,CE,HE,BI,SO,MA,DI,VE,RE,SEN,RA,TE,HI,NY,WEN,DO,QU,KI") function random_word() -- creates a random word -- words are anything from 2 to 12 characters long -- _len = length of the word in fragments local _len,_res,_hyphen,_c,_frag = 2+flr(rnd(3)),"",0,0,"" [ [size=16][color=#ffaabb] [ Continue Reading.. ] [/color][/size] ](/bbs/?pid=105580#p) |
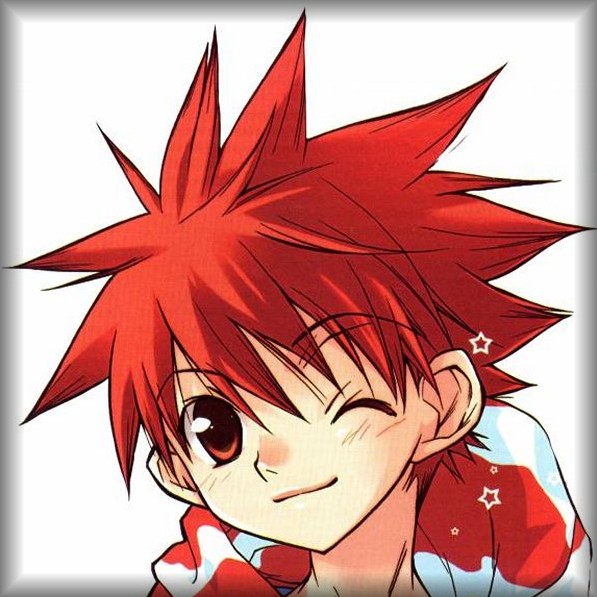
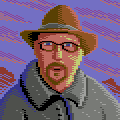
Tiny function that takes a number (float or int) and returns a string with (maximum) two decimals. For instance:
10 > "10"
20.03 > "20.03"
17.46134 > "17.46"
So not mathematically accurate, but useful under some circumstances...
function twodecimal(_n) -- will accept a number (float or int) -- turn it into a string and return the string with two decimals local _v=split(tostring(_n),".") if #_v==1 then return _v[1] else return (_v[1].."."..sub(_v[2],1,2)) end end |
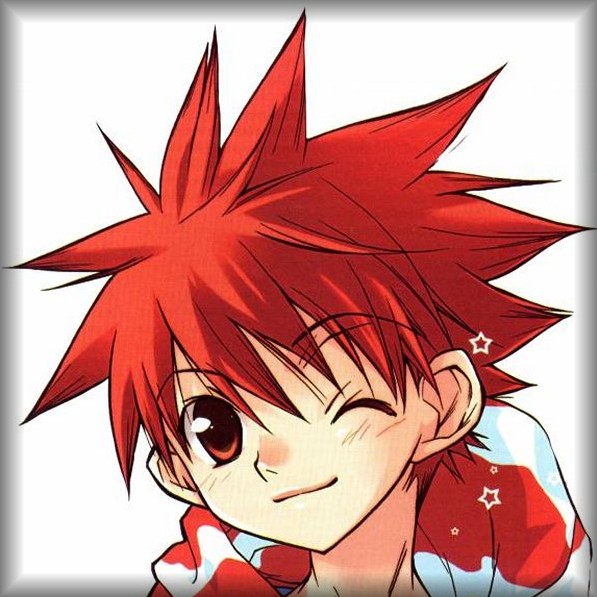
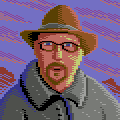