This is a half-completed demake of the Sega Genesis title Shining Force. I played the game for the first time early this year, and I found myself fascinated with the turn-based battle system. My biggest annoyance with Shining Force was that characters take a movement turn on the map, then combat takes place in a battle screen for only one turn. As enjoyable as it is to see higher quality graphics of the characters and terrain on the battle screen, there's a lot of time wasted moving between the two views in larger battles. I decided to write a similar battle system, but have all combat take place on the map instead.
Here's how combat works: Characters on the map are sorted by their speed to determine turn order, then each character gets a movement and action turn. Playable characters have HP and MP bars that appear at the bottom of the screen during their turn. Depending on their class, a character may have ranged or melee attacks, as well as one or more magic spells. There are also potions hidden on the map, which characters can pick up by standing on them, and can drink as an action. There isn't a win condition implemented, but I found it fun to try and get my party to the castle in the center of the map while testing the mechanics.

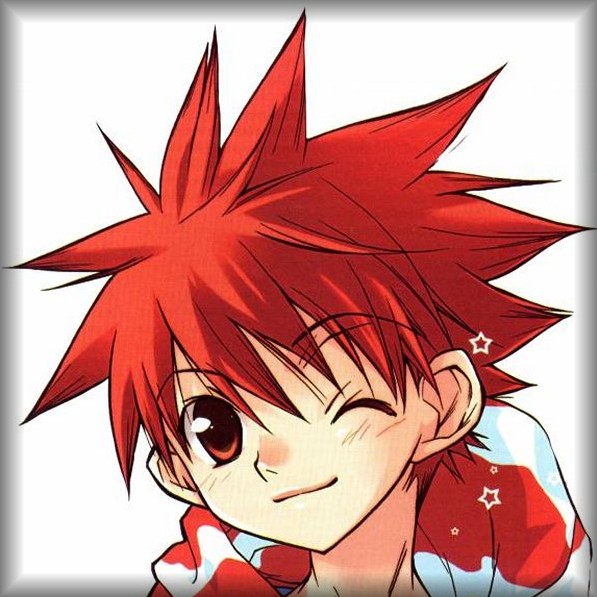
Hi all,
I'm writing a short graphical text adventure for the PICO-8, and a few weeks ago I determined that I needed a vector graphics editor capable of outputting PICO-8 code. I've created a web app called GMagic that lets you make vector drawings on a canvas, then export Lua functions for drawing them. I also provide the functions for drawing polygons and polylines:
ptstr() (needed for the functions):
Polygon:

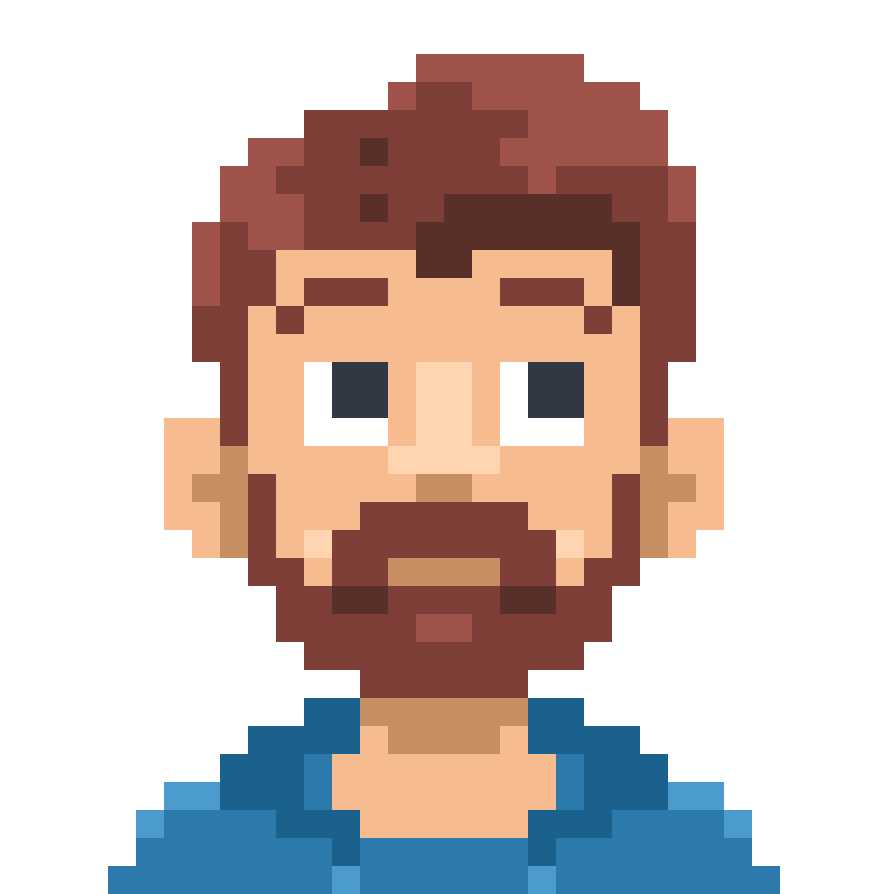
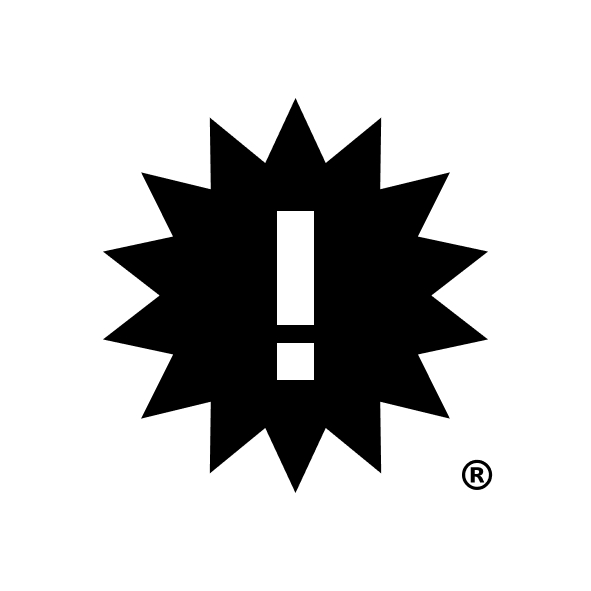

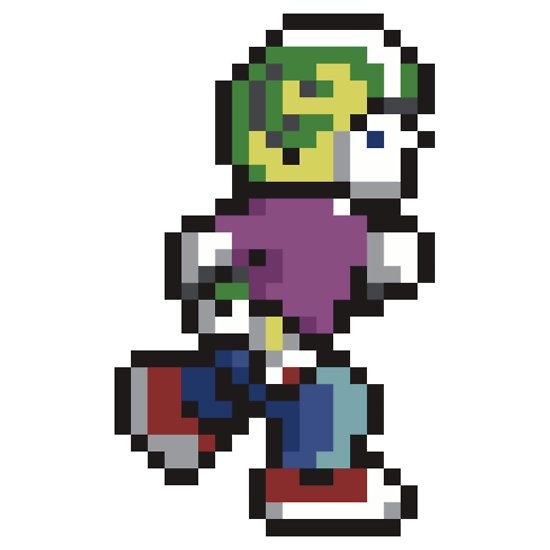
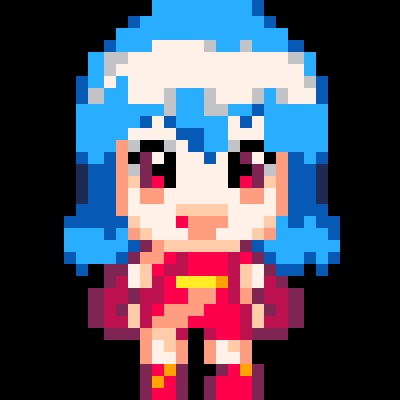
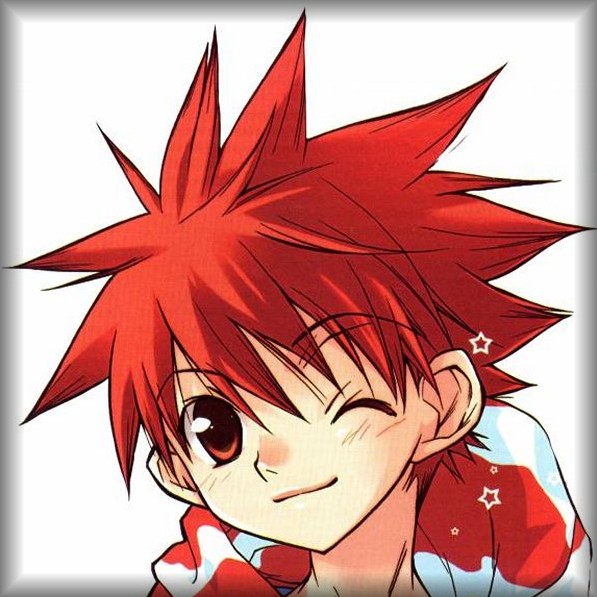


Hi all, I wanted to share with you an experiment I've been working on called World of Pico. It uses the GPIO pins to transfer map data from a JavaScript context to the cart, allowing for essentially unlimited maps for exploration when the cart is hosted on a webpage. The demo has 3 maps, but I can see this technique being used in combination with a map server, and eventually allowing for interactivity between the client and server.
The method of data transfer is very similar to how P8T by seleb works. Different values on pin 0 of the GPIO tell the JS app or the cart whose turn it is to read and update the following pin values. If you want to explore the source code or read more about the transfer technique, please check out the GitHub repo.
Thanks for taking a look!
This is a demo using the fill patterns introduced in 0.1.11 as a way of dithering between two or more colors. Use the buttons to adjust spread of the gradient, the number of sample circles drawn per frame, and the radius of the circles.
The way it works is I have a gradient table, a fill pattern table, and a spread value. I'm going to draw 500 circles randomly each screen refresh, and each circle is going to use two colors and a fill pattern as its fill. The circle's colors are determined by the nearest and next nearest colors on the gradient based on some measurement, in this case the proportion of the spread to the shortest distance from a circle to one of three bouncing points.
I set up a table of fill patterns that I generated from a 4x4 ordered dithering matrix. There's a good description of ordered dithering here. Here's the matrix I used:
Simple Voronoi diagram generator. I was trying to see if I could get a speed boost from writing pixel values directly to the video RAM, but regardless the fill walking through all the pixels is still very slow. Any ideas on how I could speed things up?
--voronoi diagram --by hypothete points={} function makepoints() for i=1,16 do e=rnd(128) f=rnd(128) add(points,{ x=e, y=f, c=rnd(255) }) end end function jitter() for i=1,#points do p=points[i] p.x+=rnd(2)-1 p.y+=rnd(2)-1 end end function near(pts,b) nt=nil --nearest pt nd=4096--dist to nt for i=1,#pts do a=pts[i] nl=(b.x-a.x)^2 + (b.y-a.y)^2 --dist leaving off sqrt if(nl<nd)then nd=nl nt=a end end return nt end function drawvoro() for i=0,63 do for j=0,127 do k=i+64*j --x+y*w = 1d position z={x=i*2,y=j} np=near(points,z) if(np) then memset(0x6000+k,np.c,0x1) end end end end function _init() makepoints() drawvoro() end function _update() if(btnp(5)) then jitter() drawvoro() end end |



Hi all, this is my unfinished Ludum Dare 36 submission (http://ludumdare.com/compo/ludum-dare-36/?uid=20847). I started building the game with the day/night system and text menus; by the time I was 40 hours in I realized that I hadn't found the game mechanic and decided to call it quits. Still learned a ton, though.
Quick shout out to geckojsc for the lua coroutine dialog system tutorial: https://www.lexaloffle.com/bbs/?tid=3833 I used a lot of the work here with a few tweaks to build the textboxes and in-game menu. One issue I'd like to address in the future is a more terse way of setting up actors and conditional scripts. I feel like when Pico-8 cartridges reach a length of ~1000 chars or so, things start to get really messy in terms of source code organization. Additionally, there were a few functions that I had trouble "hoisting" and I couldn't figure out why. If anyone has pointers on how to simplify the data structures I'm using or specifically better use of tables, please let me know. Thanks for taking a look!
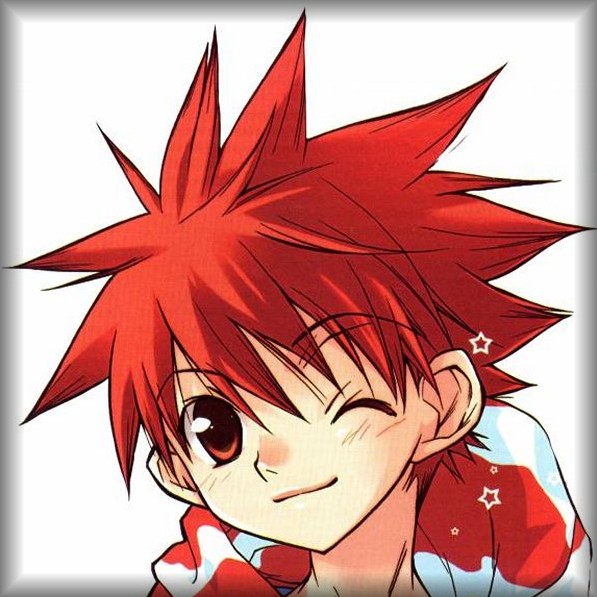