Hi all,
I'm writing a short graphical text adventure for the PICO-8, and a few weeks ago I determined that I needed a vector graphics editor capable of outputting PICO-8 code. I've created a web app called GMagic that lets you make vector drawings on a canvas, then export Lua functions for drawing them. I also provide the functions for drawing polygons and polylines:
ptstr() (needed for the functions):
Polygon:
Polyline:
If you want to read more about GMagic or check out some sample screenshots, you can view the Github repository here. If you end up using GMagic for a cart, I'd love to hear about it!
EDIT 6/24: I can't believe I forgot to include ptstr()! I've added it above, and to the Lua sample in the repo.



This looks way cool! I'm interested in using this because I'm annoyed with trying to do it all with the built in line() function, but when I ran it in Pico 8 the ptstr() function throws an error: I'm not sure where it's expecting that to come from.


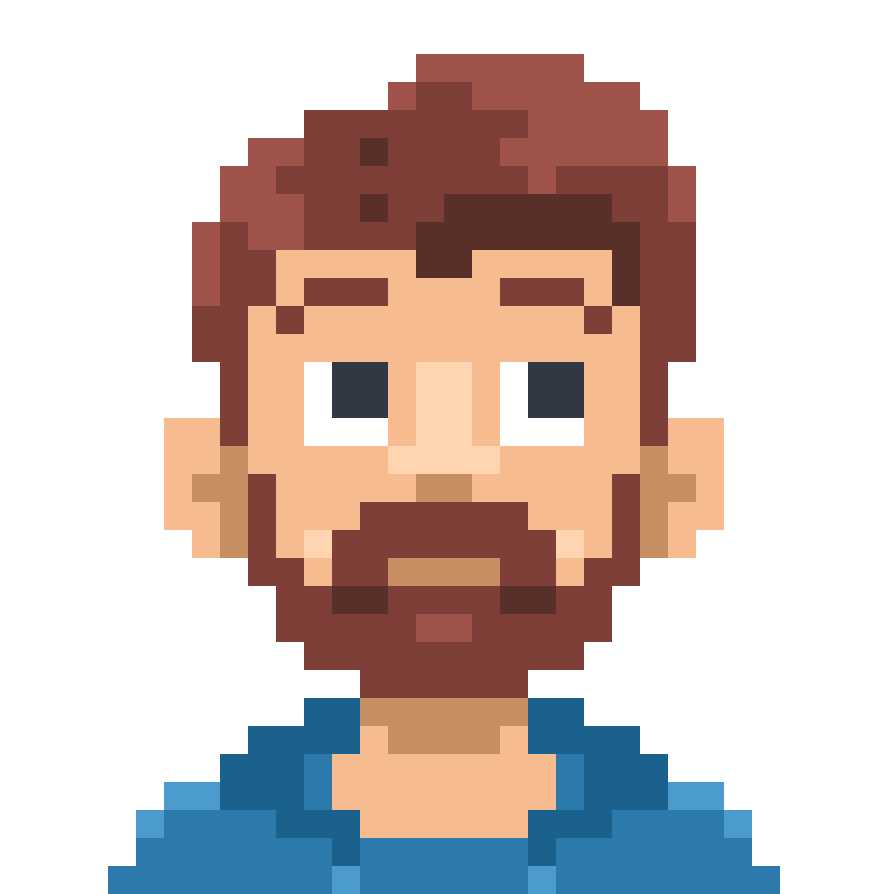
Very cool.
Was about to say this would be useful for an adventure game engine, then I see you're doing just that! 😁



Hey all, sorry I forgot about ptstr()! I've updated the main post, it's just a function that divvies up a comma-separated string of points. That way your shapes can count as one token each.


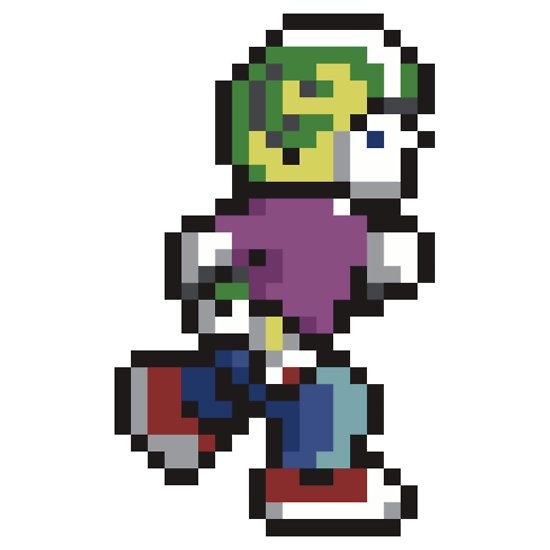
Wow this looks like the drawing style of early graphic adventure games! Cool idea!


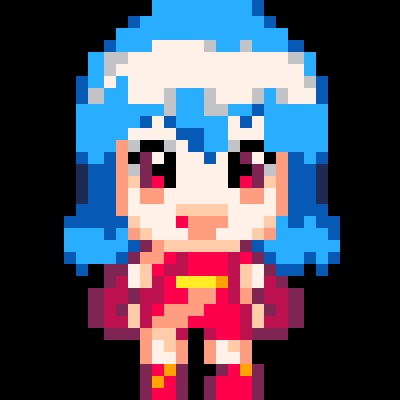
I LOVE THIS tool. Ago you think you could add RECT and CIRC to the web app? I could obviously see this tool ballooning out of control, but at least circles would add an art layer that's pretty important.



Just discovered this and think it's great!
Is it still being worked on?
Just a couple of things:
The points are big when trying to draw some little detail. It'd be great if they went to big pixels rather than boxes when not being edited.
It's be flippin' great if you could load a backdrop picture in to trace around.
Other than that. Love it.



Absolutely astounding stuff!
This community's incredible for how quickly you can think 'I wonder if such and such is possible' to discovering someone's built a solution for exactly what you had in mind!
[Please log in to post a comment]