Description
Fill with sand is sand/dust puzzle game. In each level you have to create a mechanism that solves the puzzle.
Each level can be solved usually in multiple ways (tested).
Technical notes
Simulation is deterministic - same inputs yield same results (if not let me know and I have to fix it). Framerate should be stable no matter what you do.
The game is unfinished but it's already playable. Feedback is welcome. Have fun!
Changelog
Version 3
- +2 levels
Version 2
- new feature: prohibited materials
- +1 level
Version 1
- added small delay to cursor movement for more precise painting
- added tutorials/showcases to levels 2-5
Version 0
- initial commit
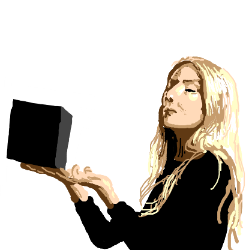
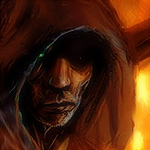
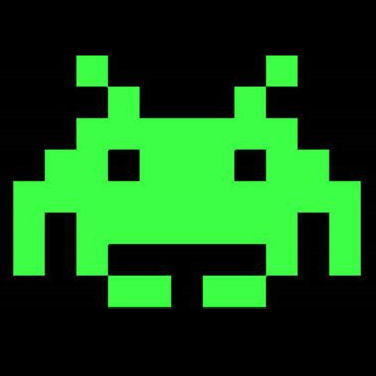
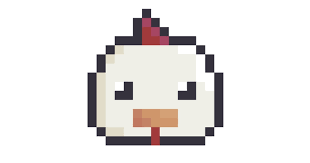

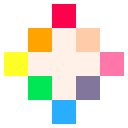
Hello,
as any of you guys I need some decent picture compression for the Pico Off Road game I'm making. PX-9 is very good choice but one of my passions always were compression algorithms. So I've decided to implement one myself. Sometimes is better and sometimes is worse than other available algorithms here. Consider it as my addition to the community and use it wherever you need.
Algorithm
LZ77 is very simple compression method that works similar to RLE with the exception: while RLE copies the same character N times LZ77 copies chunk of already decoded data of length N. I've used 3 different blocks: direct color output, offset + length block and just length for coherent rows (sequence that starts on the same X position but in previous row). Compression allows self-overlapping (for example offset 3 and length 20 is valid, it just copies itself with offset).
For more information check https://en.wikipedia.org/wiki/LZ77_and_LZ78#LZ77
Limits
- designed for PICO-8 pictures only (or any arrays of values in interval 0..15), don't try it on map or other binary data

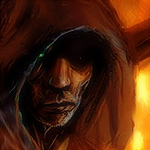
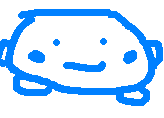
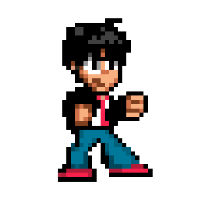
About
Pico Off Road is a racing game inspired by the classic Ironman Super Off Road. There are 5 car colors to choose from (equal by parameters), three tracks that can be played in both directions. Player can choose from two modes - single race or tournament. There is one "hidden" mode: single race with 0 opponents can be considered as a practice.
Menu controls
- Z = confirm
- X = back
Driving controls
- arrows = turning, throttle, reverse
- Z = nitro
- X = throttle (for controllers if you can't press left/right + forward at the same time)
Tech
Pico Off Road uses combination of 2D sprites, 3D height map and real-time rendered meshes (cars).


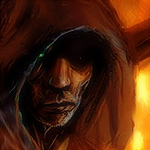
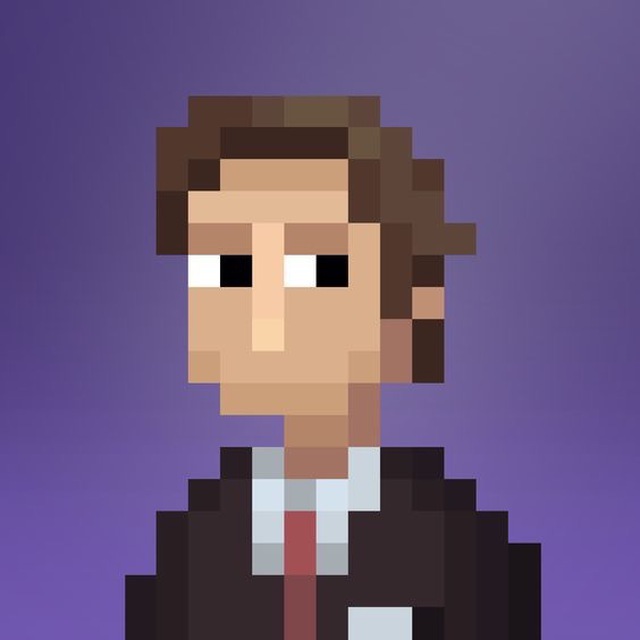
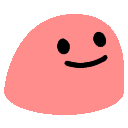


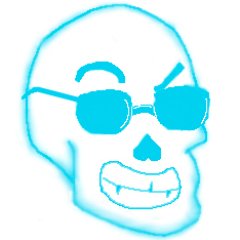

