If you feel like you don't understand Lua's tables, I hope this post helps. I use tables all the time when I'm programming; they are super useful. Let's study them so you can use them too!
Tables can do several different things, which makes them a bit tricky. We'll start by exploring a common use: using tables to store a list of things, such as:
- A hand of cards: 2♠, 3♥, Q♦, K♣, J♣
- A list of monsters: Ogre, goblin, slime mold
- The positions of all the black checkers: 12, 24, 20, 27
Making lists
This makes an empty list:
items={} |
This makes a list with the names of some things found in a forest:
items={'tree', 'rock', 'stream'} |
Now items
contains 3 things; you can think of it like 3 boxes:
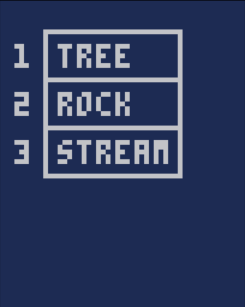
The first box contains 'tree', the second contains 'rock', the third contains 'stream'.
Getting items out of a list
Each box has a number, called its "index". To get the value from the first box, we do items[1]
like this:
.jpg)
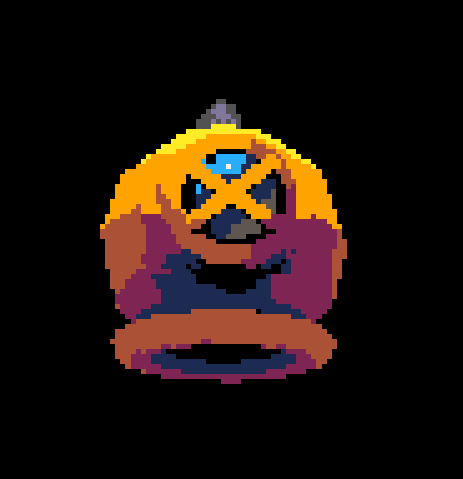

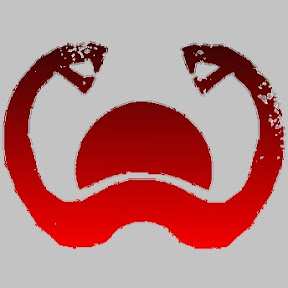
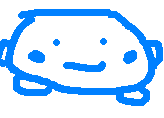

I feel like making a set of tutorial carts. What topic would help people the most?
I was thinking of a simple introduction to math for games, since that topic comes up frequently on the forums. Or maybe how to do collision detection? I see people asking about that fairly often too.
Any thoughts? Or suggestions of a topic? Would you find this useful?
.jpg)

3D texture mapping with tline (plus some other stuff, like wireframe rendering, and solid color polygon rendering). Did this as a learning project, to study old-school pre-GPU 3D graphics.
Thanks to freds72 and johanp for inspiration & example code.
References if you want to learn.
http://www.multi.fi/~mbc/sources/fatmap2.txt
https://chrishecker.com/miscellaneous_technical_articles#perspective_texture_mapping
https://www.cs.cmu.edu/~fp/courses/graphics/pdf-color/14-raster.pdf
https://en.wikipedia.org/wiki/digital_differential_analyzer_(graphics_algorithm)
https://en.wikipedia.org/wiki/selection_sort
https://en.wikipedia.org/wiki/sutherland%e2%80%93hodgman_algorithm



I'm trying to use anonymous inline functions and I'm finding some weird behavior when they are on tabs.
Putting everything in tab #0 works:
x=1 function _draw() cls(1) print('x is '..x) end (function() x=2 end)() |
And moving the inline function call to tab #1 works:
x=1 function _draw() cls(1) print('x is '..x) end -->8 (function() x=3 end)() |
But putting an inline function call on both tab #0 and tab #1 does not work:
x=1 function _draw() cls(1) print('x is '..x) end (function() x=2 end)() -->8 (function() [ [size=16][color=#ffaabb] [ Continue Reading.. ] [/color][/size] ](/bbs/?pid=83769#p) |
I'm trying to figure out why this cart only runs at 10 FPS on a Raspberry Pi Zero. stat(1) returns 0.49 and stat(7) returns 30. However, the screen is only updating at 10-11 FPS. Other carts with higher stat(1) values don't show this problem, so it seems specific to something in this cart.
I can optimize to get the CPU usage down to 17%, and then the real framerate becomes acceptable. However, I'd actually like to understand why it is slow so I can work around it. (Also, I'd prefer to be able to use the whole CPU budget instead of just 17% of it!)
I assume what's happening is that the pico-8 CPU costs are optimistic for some instructions, and the actual cost on the Pi Zero hardware is higher. But which instructions?
Are there specific instructions I should be avoiding?
Are there specific types of drawing (like large map areas, or off-screen drawing) I should avoid?
Can I hook up a profiler to see what it is spending its time on?
The original raspberry pi post (https://www.lexaloffle.com/bbs/?tid=3085) says math-heavy carts run slowly. Is that still true?

