controls:
This is a niche tool to help you save characters when writing carts that are codesize-constrained.
- type in a number; press enter
- then press up/down and ctrl-c/enter to copy a code
- navigate back up (or press backspace) to start typing a new number
Example: 0x6000 can be written as 0x6000 (6 chars), 24576 (5 chars), 6^13 (4 chars) or ⌂-🐱 (3 chars!)
The tool sorts the results by character count (on twitter), so the top results are your best bet.
Update: The latest versions of pico8 have special P8SCII codes for poking which are often fewer characters than calling poke directly (even after using this tool). e.g. ?"\^!5f10249?"
instead of poke(0x5f10,50,52,57,63)
. Consider using that instead! But this tool might still be useful sometimes, and at the very least, it's an interesting artifact.
motivation:
While I was making Free Cell 1K (itch | twitter | bbs) for the #Pico1K jam, I realized I could save characters by taking advantage of the built-in constants. I needed to poke a few things to various addresses (for setting the palette, drawing dynamic shadows with bitplanes, and enabling the mouse) so I had code that looked like this:
-- 94 chars (not including comments or newlines) poke(0x5F10,8,1,251,7,4,0,3,15) --palette poke(0x5F2D,3) --mouse poke(0x5F5C,-1) --disable btnp repeat poke(0x5F5E,0xF4) --shadows on poke(0x5F5E,0xFF) --shadows off |
Each of those poke addresses uses 6 characters; we can do better! The first thing I did to save characters was this:
-- 84 chars (not including comments or newlines) A=24365 poke(A-78,8,1,251,7,4,0,3,15) poke(A-49,3) poke(A-2,-1) poke(A,0xF4) poke(A,0xFF) |
But then I saw @zep tweet a trick for writing sqrt(x) as x^█ instead (because █ (shift+A) is defined to have a value of 0.5), and I realized I could do even better: the 😐 (shift+M) character is defined to have a value of -24351.5, so I did this:
-- 95 chars (not including comments or newlines) poke(-15.5-😐,8,1,251,7,4,0,3,15) poke(13.5-😐,3) poke(60.5-😐,-1) poke(62.5-😐,0xF4) poke(62.5-😐,0xFF) |
95 chars is not an improvement... yet! However, poke() ignores fractional addresses, so those .5s aren't necessary. Also, I was able to combine the 0x5F5C and 0x5F5E pokes into a single poke, which nullifies some of the relative advantage of the A=24365 technique. In the end, this was the shortest code I could find:
-- 64 chars (not including comments or newlines) q=poke2 q(-15-😐,264,2043,4,3843) q(14-😐,3) q(63-😐,244) q(61-😐,-1,-1) |
This saves 2 characters over the equivalent version that uses A=24365 instead of the moon face. (or maybe just 1 character, if the newline after q=poke2 can't be removed)
how did you know moon face was the one to use?!
I didn't! I wrote a program to brute-force try all the built-in symbols and see if any were useful for my needs. Check out tab 5 of the cart ("analysis") to see the brute-force algorithm I used.
I've cleaned that program up and posted it here for you. It might be less useful for tweetcarts (because stuff like 😐 takes up 2 characters on twitter) but it saved me 1~2 entire characters (genuinely very helpful!) during the Pico1K jam, and I hope it helps you too.
Leave a message here or tag me on twitter if you found it useful; I'd like to see what you make!
how does it work?
- build a list of all 150 "symbols" under consideration:
█▒🐱░✽●♥☉웃⌂😐♪◆…★⧗ˇ∧▤▥
(most symbols)- the numbers 0-9
- and the negated version of everything above
- the numbers 10-99 (but not their negated versions)
- try combining every pair of symbols a,b with these operations:
a a*b a+b a&b a|b a~b a-b a/b a^b
- if any result is between
target
andtarget+1
, display the result. this was chosen because poke/memset/etc round their inputs down to the nearest integer. custom checks are easy to hack in yourself; search for "function near" in the code
changelog
- v8:
- add support for new pico-8 syntax
a~b
for bitwise not (1 char shorter than the olda^^b
) - sort results by twitter character count instead of naive character count. thanks to jadelombax for the handy reference table here: https://www.lexaloffle.com/bbs/?tid=44375
- add support for new pico-8 syntax
- v7: fix typo in v6
- v6:
- fix exponentiation parse order (
-a^b
gets parsed by pico8 as-(a^b)
, unlike any other operation on the list) - increase list scroll speed
- clean up output list results a bit (e.g. no more
-a+b
, sinceb-a
is shorter) - remove
~x
from consideration; it's very similar mathematically to-x
, so calculating it was making all searches slower for hardly any benefit. (the code is commented out, so you can re-add it yourself pretty easily if you download the cart and search for~
) - make it easier to do custom "is close enough" checking; search for "function near" in the code
- fix exponentiation parse order (
- v5: show options (and allow them to be copied) as soon as they're found. (no longer need to wait for the entire search to complete)


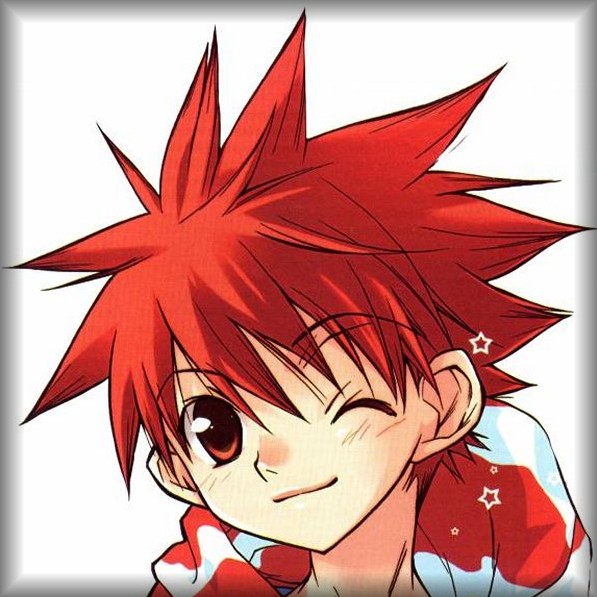
I am just now seeing this for the first time. It reminds me of the way I compress some data tables. I check to see which is smaller, a compression block or decompressed block.
Your Constant Companion @pancelor will definitely aid Twitter coders. Nicely done ! Gold star.
I am a little confused though. Shouldn't 5
return as ❎ ?


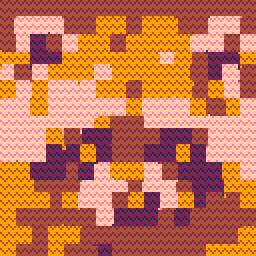
thanks! the tool has a big list of possible inputs including "5", "😐", and other single-character symbols, but it doesn't include "❎" -- because you can just type "5" instead ;)


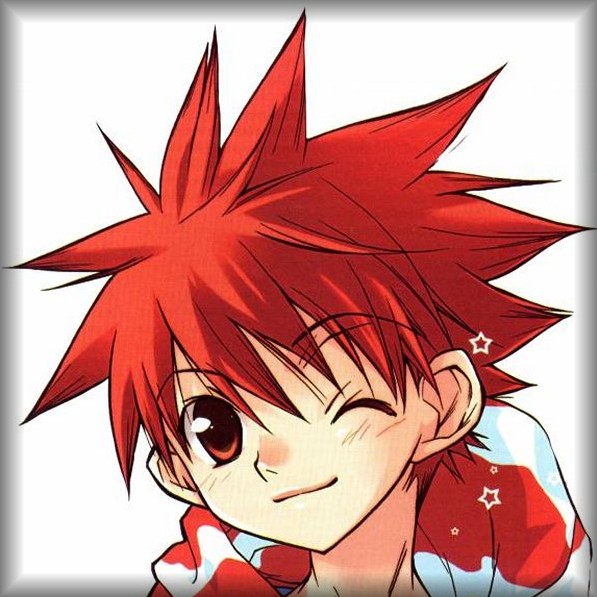
Well it wasn't so much the fact it matched the same character size, @pancelor. I was wondering if you were checking every possible combination and permutation of creating an alternative method of either calculating or replacing the value with matched glyphs.


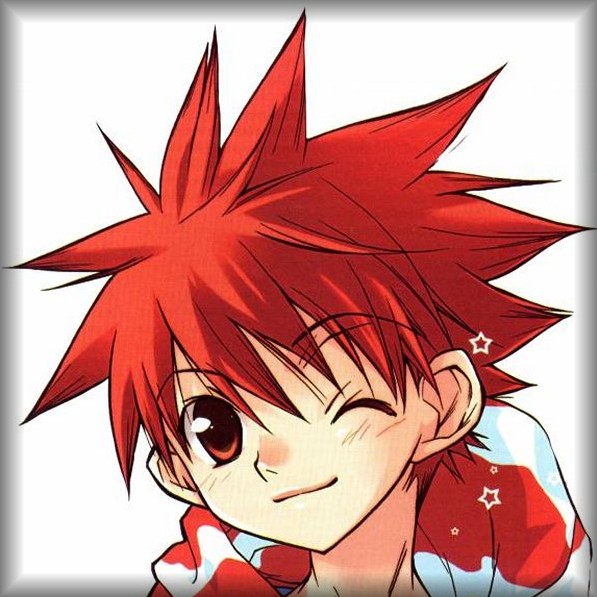
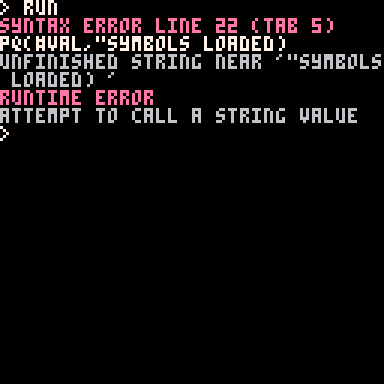
Wow. I just tried running your code, @pancelor, and it crashes straight out in immediate mode. Online too. I ran it in immediate mode though so I could F1 the screenshot to show you.


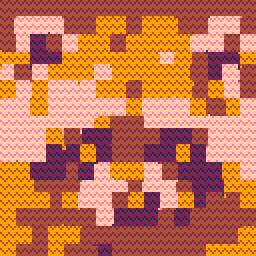
whoops, silly mistake on my part. thanks for the heads up -- fixed!
[Please log in to post a comment]