OK, I know we can use ternary conditions in variable assignments. But - as far as I can tell - they can't be used in calculations. Having logical statements be used as a value operator in a calculation can be used in many circumstances, would be readable and possibly pretty token efficient.
How might this look?
(a > b) would result in a 1 if true, 0 if false.
something like "pset(100+100*(a > b), 200, 7)" would prevent the use of a bunch of ternary assignments, local variables or if statements.
So what do you think? Useful? Doable? Worth it?


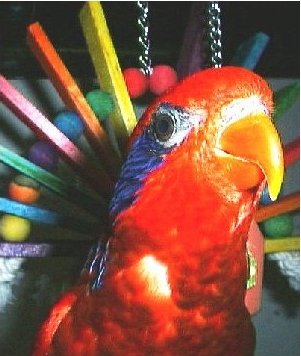
I believe pset(100+100*(a > b and 1 or 0), 200, 7)
should give the result you are looking for. You could also use pset(100+(a > b and 100 or 0), 200, 7)
.


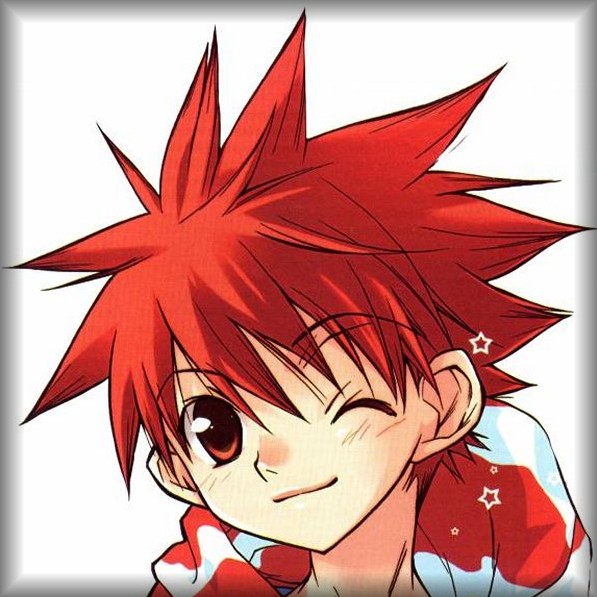
Hi @Miez. This is one of the first suggestions I made years ago and at the time I made it I was told it would not happen because, "That's just the way LUA is."
https://www.lexaloffle.com/bbs/?pid=57384
So if @zep likes your idea now, which is the same, then I will totally support you, gold star, as long as it gets made !
One possible way to invoke it:
poke(0xnnnn,nn) Change TRUE and FALSE nn=0: TRUE=TRUE FALSE=FALSE (Reset) nn=1: TRUE=FALSE FALSE=TRUE (Reverse) nn=2: TRUE=1 FALSE=0 (Standard) nn=3: FALSE=1 TRUE=0 (Reverse of Standard) nn=4: TRUE=-1 FALSE=0 (QBasic) nn=5: FALSE=-1 TRUE=0 (Reverse of QBasic) |
Just recently 11-17-22 I had to write a function for this.
function _(logic) if (logic==true) return 1 return 0 end |



_={[true]=1,[false]=0} ?_[a>10] |
would be an alternate.
In lua true/false are real booleans and not numbers like in qbasic or c.
It is also not so simple to change the result of an logical statement.
At the moment only two things will fail a logical test: false, nil
everything else - a string, a table, a number (even 0!) will result in a true.
To many things would not work any more.
And an other problem is, when you want to share your code. You must seek through the complete code to find the poke. And it is possible to switch "on the fly".
I think, one of the "main pillars" of pico-8 is: change!
Download a pico-8-game, look in the code and try what happend, when you change something in it.


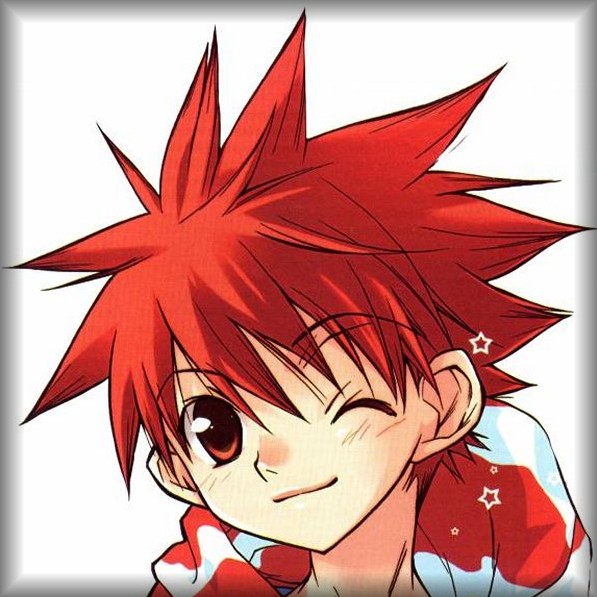
That's quite interesting what you did up there, @GPI !
I did not know you could use TRUE and FALSE as a value inside an array.
For those who are confused, and I was. He is saying:
_={} _[true]=1 _[false]=0 |
And before you think you can top this, if you try: true=1
it does not work, so this is indeed a super-small way of doing it.
Nicely done !
. . .
Wait. I'm running into an interesting error.
How do I do this ?
_[nil]=0
I want anything that is not TRUE to return 0 (zero), so I need NIL to also return 0.
For instance in BASIC, you can do this:
dim a[10] for i=0 to 9 print a[i] next |
And you would get back 10 zeroes, not [NIL].



It's a personal preference but I'm not generally a fan of this kind of thing. If something should evaluate to a boolean then it should evaluate to a boolean and not an integer. Evaluating non-booleans as truthy-falsy values just makes a language semantically messy and leads to all sorts of bugs....
Of course, having said that, I take advantage of it all the time so I'm basically a giant hypocrite and in a highly restrictive environment like Pico-8, a token saved is a token saved so more power to you.
You can use a function instead of a table:
function _(k) return k and 1 or 0 end |
It uses one less token than @GPI's (10 vs. 11) and will work for any truthy or falsy value including nil. It will be slightly less efficient though since function calls are relatively more expensive than table look-ups.
@dw817, you can't use nil as a key in a table which is what's causing your error. The function version above does what you want if I'm understanding your example correctly. This prints 10 zeros.
a = {} for i=1,10 do ?_(a[i]) end |


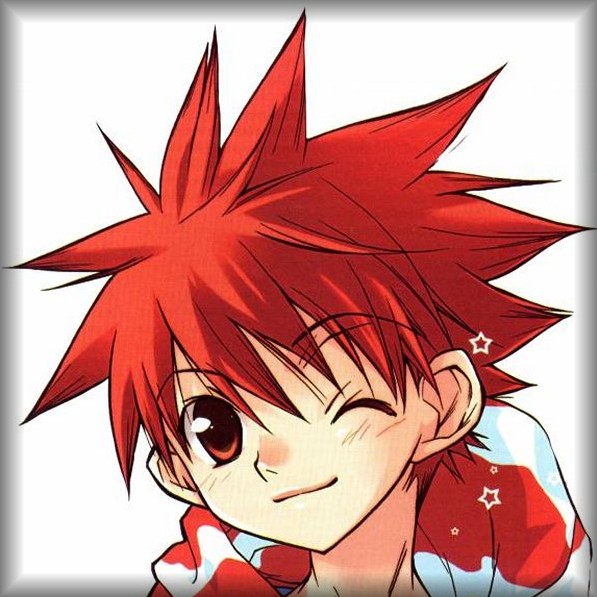
Yeah, that's it, @jasondelaat ! Sweet relief. Finally free of that infernal [NIL], at least in a function.
Now from what you did, how could this be accomplished in a single line ?
function _(a) if (a==true) return 1 if (a==nil or a==false) return 0 return a end |
So that the value is not automatically 1 if it is a normal number or string, but the same value fed into it as input.



@dw817
As a single line you could do this:
function _(k) return (not k and 0) or (k == true and 1 or k) end |
That's 19 tokens but the parentheses aren't actually needed so you can get rid of them and save 2 tokens. I put them in just to make it a little more clear what's actually happening. Not sure it needs to be a single line though.
Your version is 24 tokens but you can bring it down to 19 by simplifying the second if which is arguably more readable:
function _(a) if (a==true) return 1 if (not a) return 0 return a end |


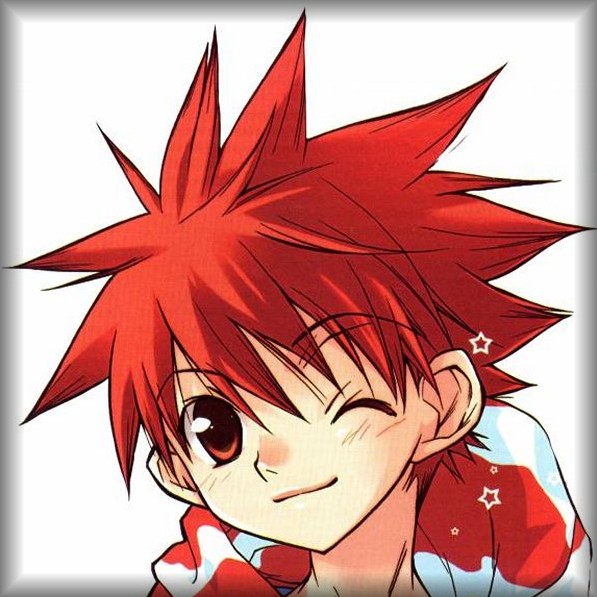
Once again that is quite good, @jasondelaat. I wish my brain could work like that. Less token hopefully means faster code. :)


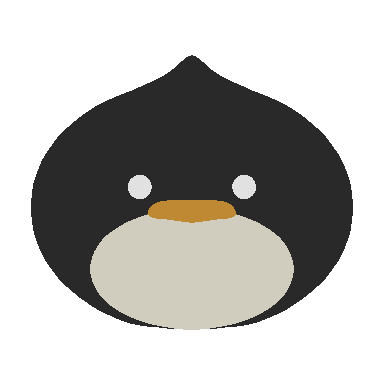
If I understand correctly, PICO-8's tonum
does what you want- tonum(true)
returns 1 and tonum(false)
returns 0.


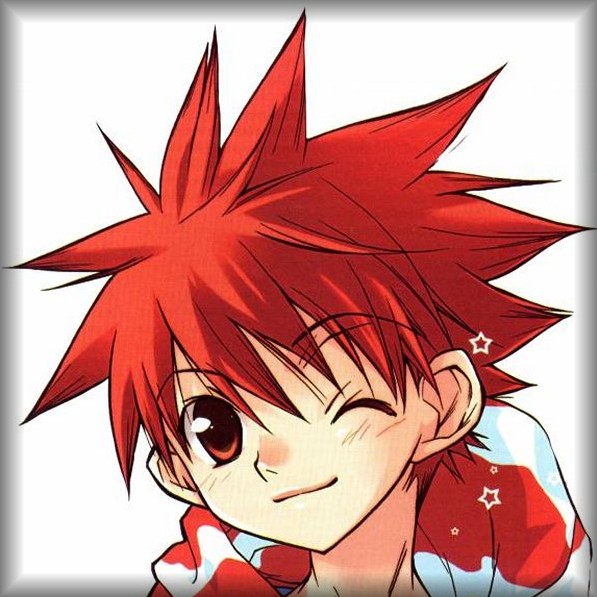
Hi @Meep. Indeed it does. However I want it to return a string in case a string is sent through it as well. IE:
23 > 47 = 0 abcd = 0 (unknown variable) apple = "sauce" (contents of this known variable) 18 = 18 47 > 23 = 1 FALSE = 0 TRUE = 1 NIL = 0 |
So this code:
function _(k) return (not k and 0) or (k == true and 1 or k) end |
cls() apple="sauce" ?_(23>47) ?_(abcd) ?_(apple) ?_(18) ?_(47>23) ?_(false) ?_(true) ?_(nil) |
0 0 sauce 18 1 0 1 0 |
Definitely gets it !
Marvelously done, @jasondelaat ! ⭐⭐⭐⭐⭐
However, to be back on topic. YES to be able to input and output 1 and 0 for TRUE and FALSE.
I completely support and agree with this request - and it shouldn't interfere with anyone else's code that is already making use of TRUE, FALSE, and NIL as it would be purely optional and either an obscure POKE() or EXTCMD().
So far I'm the only one that's given a star to this post. Yet it does seem like other people would be interested in this.



Honestly im gonna have to disagree- this would be (afaik i only have the barest knowledge of the lua c api) really messy on the backend, and wouldn't really make sense with the rest of lua- pico 8 isn't hardline manual lua, sure, but most of the major changes are api restrictions or shorthand. Like, this isn't some pre-processor step, or some system you can interact with through function calls, it'd be a change to the way boolean values are.
[Please log in to post a comment]