This is a tiny (47 token!) Entity Component System library.
Entity Component Systems are design patterns where entities are generic collections of components and logic is written for specific component types. It's popular for game design as we often have many 'things' that don't always fall within clear cut types.
There's different approaches to ECS, here we'll consider a table to be an entity, and the key/value pairs to be component names and values.
This library is basically a single function, system, which creates a function that selects every entity with the specified components and calls the user provided function on each one.


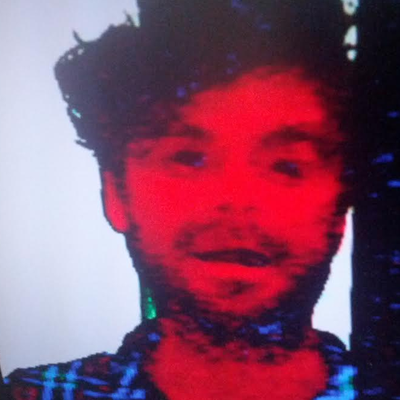


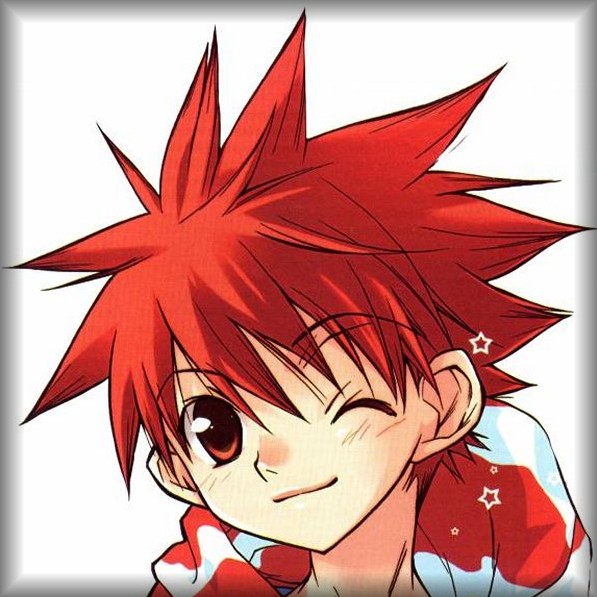

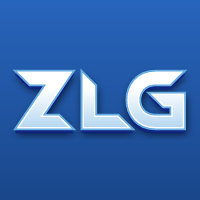
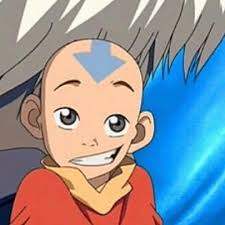
This is a small (259 token) library for finding entities close to a point.
A bucket hash (also called a spatial or grid hash) breaks up space by a grid size, and maintains a sparse table of "buckets" representing a grid location. When entity membership in these buckets is updated, you can quickly
retrieve entities near a given point.
-- usage -- create a data 'store' {size=30,prop="pos"} -- store.prop should match your entities' position property name, -- which should be a 'point' value like {x=0,y=0} -- store.size should be tuned to the max neighbor distance you'll be finding -- periodically call bstore(store, entity) to update their bucket membership -- bget(store, point) returns stored entities from a 3x3 square of buckets around -- the given point, filter these by a distance function if you need more precision -- bdel(store, entity) to remove an entity -- remember you can maintain multiple stores based on the needs of your game! |

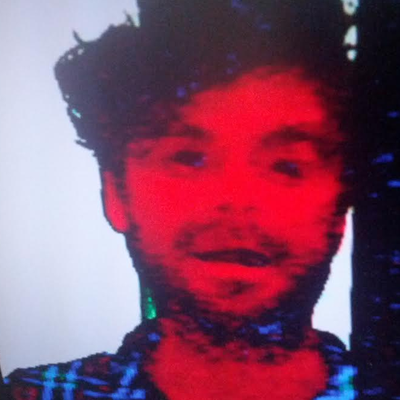

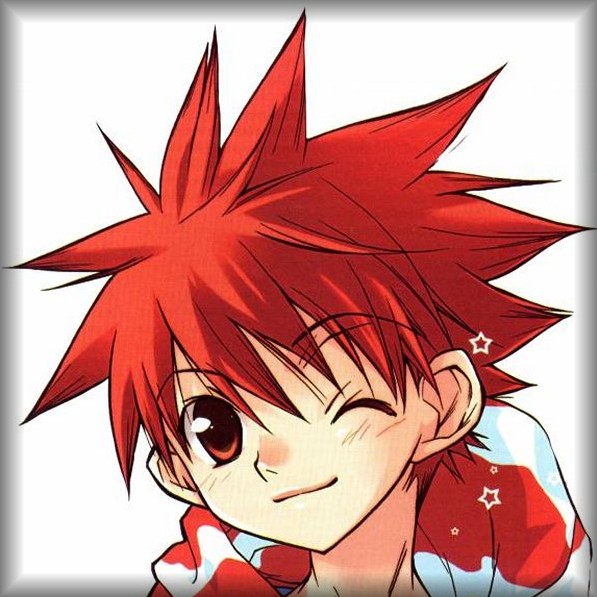

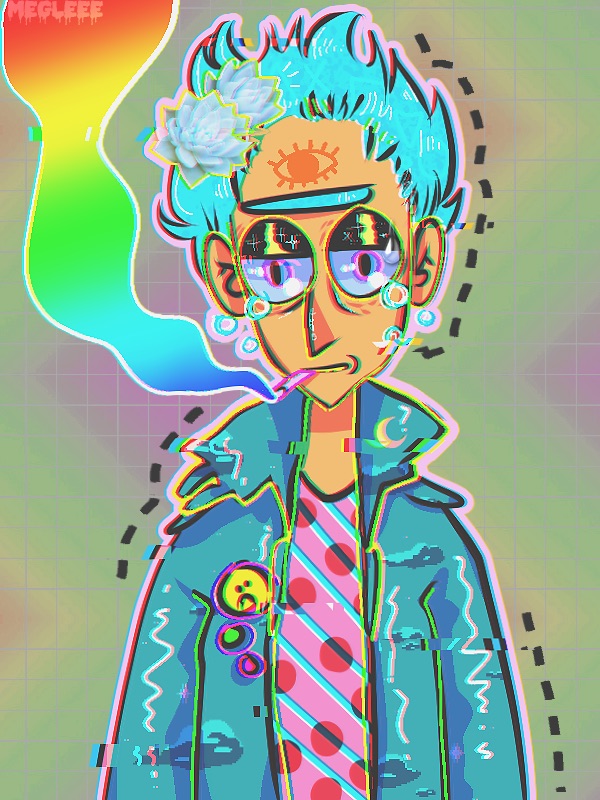
This is a small (257 token) tweening library. It allows you to tween any value over time, and can be extended
by supplying your own easing and lerping functions. It also allows callbacks, functions that are called when the tween has finished, letting you sequence animations and code.
quick start:
tween(player.position, "x", 64, 100, {e=pow(2)}) -- tween(object, prop_name, target_value, duration, options_table) -- options are -- "e" dual in+out easing fn -- "ei","eo" in,out easing fn -- "f" tween callback (takes 1 argument - the object) -- "l" lerping function, default lerp works with number values |