This is my code snippet for 8-dir movement in PICO-8. There are some in BBS like this but I think I have something to add here because:
1 - It doesn't move faster when going diagonals;
2 - It can move at speeds below 1 pixel/frame (sub-pixel movement);
3 - And most importantly: IT DOESN'T HAVE ANY ANNOYING JITTERING (the staircase effect)!!!
Feel free to scrutinize my code and give me any constructive tips. I am still getting started with PICO-8 and Lua.
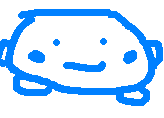
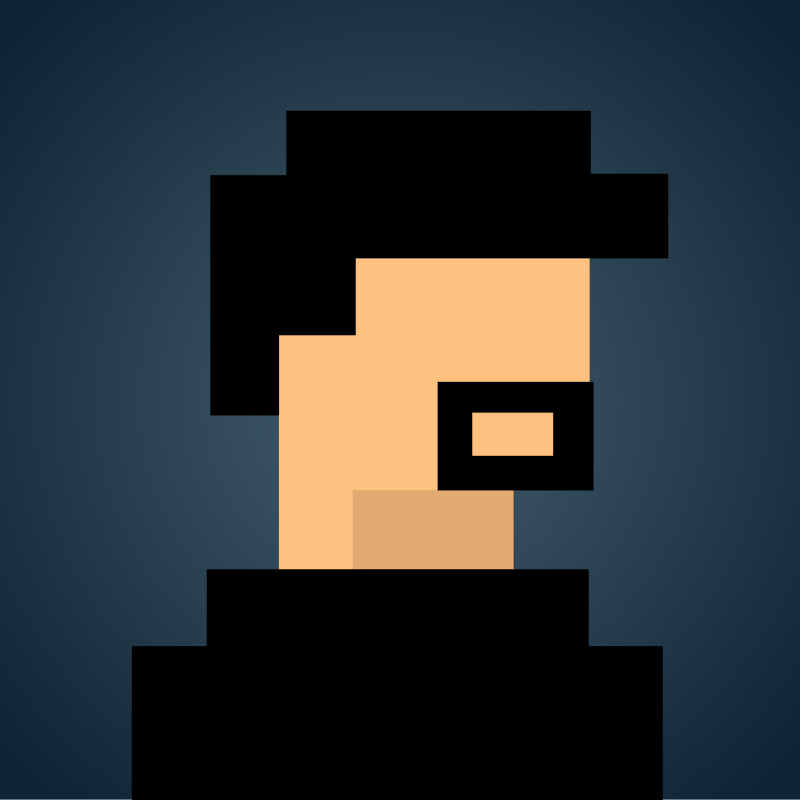

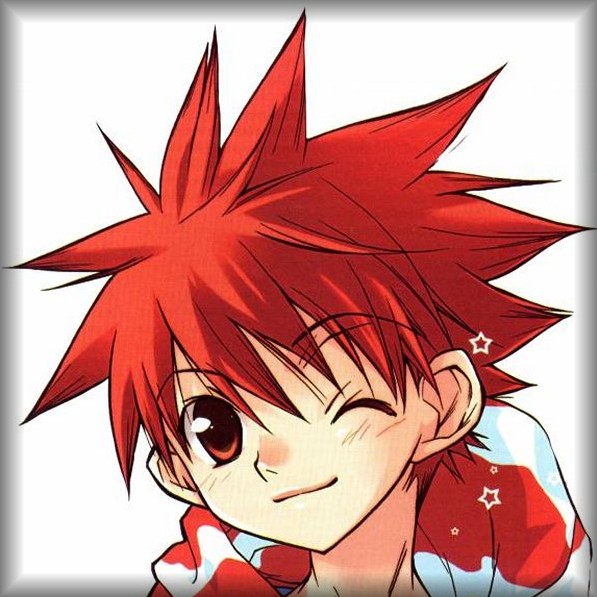

Do anyone know how constant is the duration of a frame in PICO-8?
I am considering making samething that will use rhythm and decided to use the frames as a time basis. First thing I implemented was a simple metronome.
Code below:
This function rounds a number n to the nearest integer that is multiple of m:
function round_mult(n, m) -- n is the number to be -- rounded. -- m is the integer -- significance. -- returns the nearest -- multiple of m. if (n % m) == 0 then return n else v_up = flr(n) + 1 v_up = n + (m - n % m) v_dn = flr(n) v_dn = n - (n % m) if (v_up - n) <= (n - v_dn) then return v_up else return v_dn end end end |
On the metronome itself, I want to put a bpm and use the nearest bpm that can fit inside a frame time.
function _init() bpm = 50 -- this is not necessarily the actual bpm ingame. btime = 60/bpm -- time per beat in seconds subdivs = 4 -- subdivisions of a beat fps = stat(7) -- framerate ftime = 1/fps -- time per frame bframes = round_mult( btime / ftime, subdivs) -- the actual bpm to be used, but in frames instead of seconds curframe = 1 -- frame count starts at 1 end function _update() if curframe == bframes then sfx(1) -- this is the end of a beat curframe = 1 elseif (curframe % (bframes / subdivs)) == 0 then sfx(0) -- this is a subdivision of a beat curframe = curframe + 1 else curframe = curframe + 1 end end function _draw() cls() print(tostr(curframe)) end |


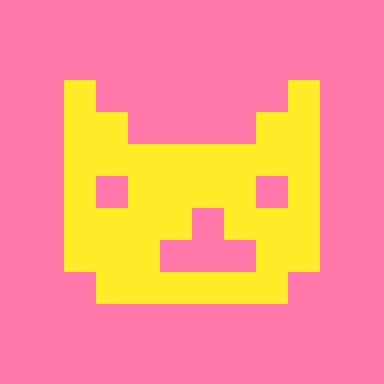