

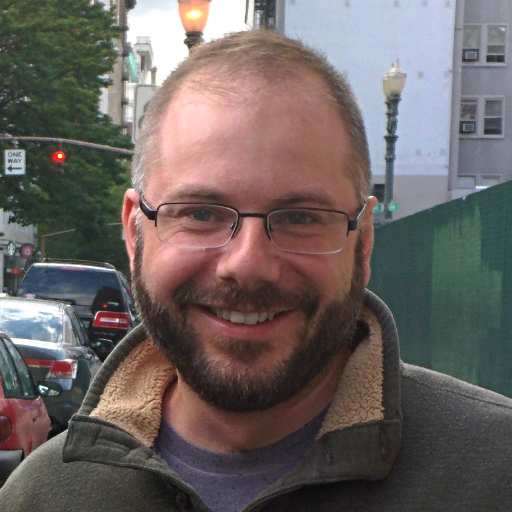
If you don't need to check for "", then you could just do:
function sum(a,b) if (not a or not b) return --blah blah code end |
Otherwise, you'd probably have to do
function sum(a,b) if (not a or not b or a=="" or b=="") return --blah blah code end |


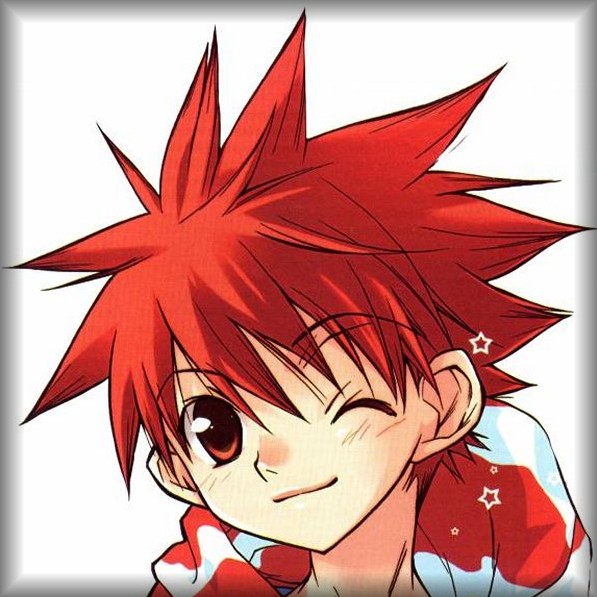
Yep, I need to check for any of those. Sposers I could do a _n=nil global to save me a few keystrokes later. I like what you did there with the (not a or not b or a=="" or b=="") thing.
Sweet.
Welp, I'm working on a calculator right now - or rather the function to calculate. Got that Sprite-flag thingy done, going back to work on the gaming calculator, which does use parentheses amongst other things.
Wanted to separate the code first that does the actual calculation afores I put it in the main cart.
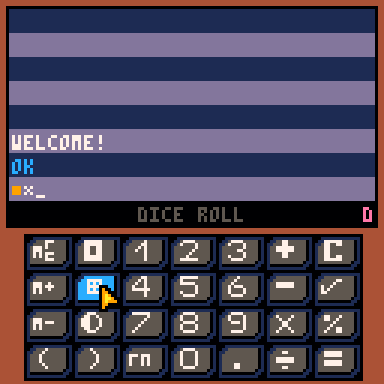
Thanks for the help, MBoffin !


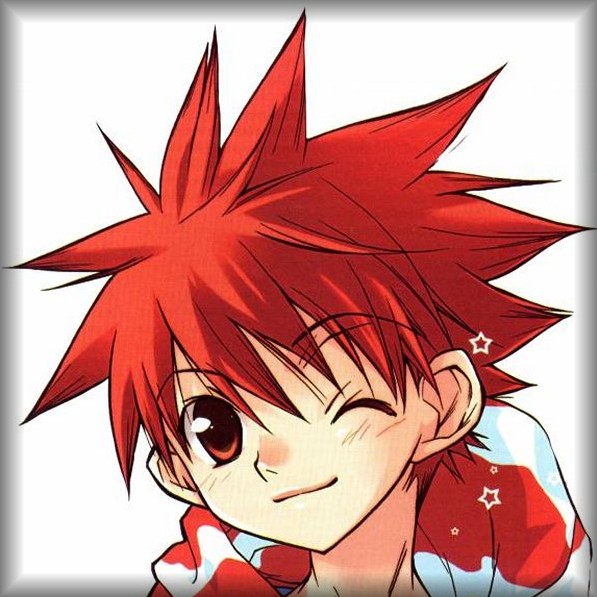
You got my brain spinning now that I see NOT is a possible operand. This also works:
if not(a and b) then print"at least one is nil" end |


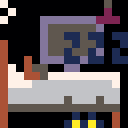
If your question isn't just "how do I make this as short as possible" but is "in terms of characters how do I make this as short as possible in all the functions I use it in", then - presuming each calculator function needs to perform the same checks - you could send it out to another function.
If that was obvious, or wasn't the question, please disregard. (Really I feel like I'm answering something other than the question, and shouldn't click submit.)
function sum(a,b) if (n(a,b)) return --code end function n(a,b) return a==nil or b==nil or a=="" or b=="" end |
(code untested)


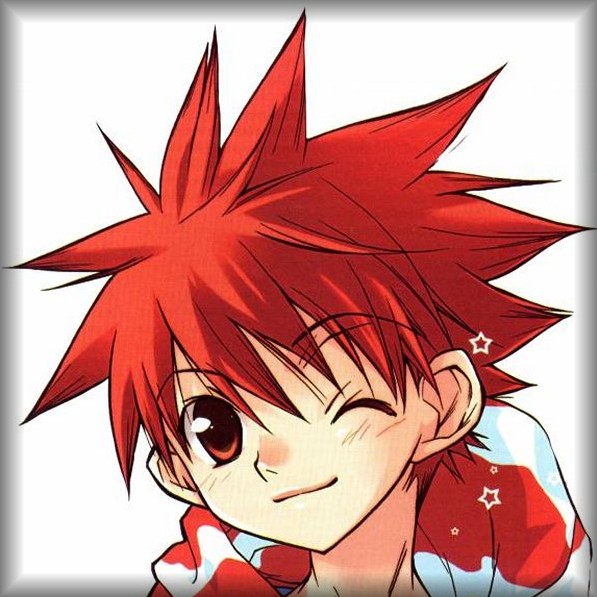
Already there, REM.
-- return true if nil or "" function nnil(a) if a==_n or a=="" then return _1 end return _0 end--nnil(.) |
Your code is tighter though and well written. A definite improvement over mine. Nicely done !
While I've got you on the horn, is there a way to go through a number of arguments ?
IE:
function countargs() for i=1,#args(countargs) do print(i.." "..arg(countargs,i)) end end |
You could call it for instance with this:
countargs(3,4)
results would be:
1 3 2 4 |


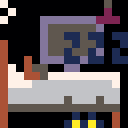
Presumably as I've been offline for about 12 hours you've had a chance to look into this.
But anyway, because it's interesting for me to know these things I researched. Standard Lua would use ... for the parameters and reference them with arg.
printResult = "" function print (...) for i,v in ipairs(arg) do printResult = printResult .. tostring(v) .. "\t" end printResult = printResult .. "\n" end |
Source: lua.org
(I can't get that snippet + a few lines to test it to run at the Lua demo. They tell me my argument is nil. It's early here; perhaps it's me.)
In pico-8 even changing ipairs to pairs won't get that to work - there doesn't seem to be the name "arg" to work with. (Did I miss something?)
So you'd need to pass all your arguments as a table or string instead. Passing them as a table is anyway what Lua does:
"The three dots (...) in the parameter list indicate that the function has a variable number of arguments. When this function is called, all its arguments are collected in a single table, which the function accesses as a hidden parameter named arg. Besides those arguments, the arg table has an extra field, n, with the actual number of arguments collected."
Source as above.
With a table:
function countargs(arg) for i,v in pairs(arg) do print(i.." "..v) end end countargs({0,1,2,3,"a","b","c"}) |
I won't provide a snippet of anything similar with a string because my instinct is that you'd be better off making a table of your separated tokens as soon as possible - preferably at the moment when the calculator button is pressed - then working with the table. (Besides which pico-8 doesn't provide functions for a lot of string processing.)
(As a disclaimer, pico-8 and Lua are relatively new to me.)


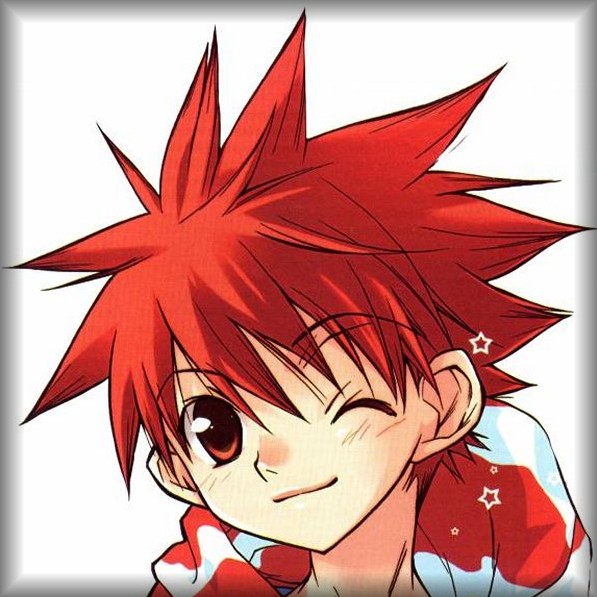
Yeah, was trying to avoid sending an array. Maybe this is something ZEP can come up with.
I know I wouldn't be the only one who would benefit from the ARG() and ARGS() function.
Thanks for your research and workaround though, remcode.
[Please log in to post a comment]