So I made a function to search for a key value in a table and delete that index three times, but the problem is del() deletes the first instance of the value I want to delete and not the index of said value. I put my function below, please help.
function delindex(val) for i=1,#table/3 do if table[i*3-2]==val then del(table, table[i*3-2]) del(table, table[i*3-2]) del(table, table[i*3-2]) end end end |



Before I offer my answer, I would suggest using the step value on the for loop, rather than dividing by 3 and then multiplying by 3. It will simplify all of the math and indexing:
function delindex(val) for i=1,#table,3 do if table[i]==val then del(table, table[i]) del(table, table[i]) del(table, table[i]) end end end |
Also, I am assuming you only mean delindex() to delete one triplet, in which case you would want to return immediately after the deletion:
function delindex(val) for i=1,#table,3 do if table[i]==val then del(table, table[i]) del(table, table[i]) del(table, table[i]) return end end end |
Now, moving on, you're correct about how del() works, so you'll need another for loop that manually copies the contents of the table back three slots:
function delindex(val) for i=1,#table,3 do if table[i]==val then for j=i,#table do table[j]=table[j+3] end return end end end |
Note that we end up copying from past the end of the table. This conveniently sets the last 3 elements to nil, and when you set table[#table] to nil, Lua will automatically decrement #table until table[#table] != nil or #table == 0, effectively shortening the array/sequence.
And, finally, I personally would break that functionality into its own function, because it's likely to be useful elsewhere:
function delrng(t, i, n) for j=i,#t do t[j]=t[j+n] end end function delindex(val) for i=1,#table,3 do if table[i]==val then delrng(table, i, 3) return end end end |
I hope this helps. :)
PS: In case any of this was overly obvious to you, please be aware that I always write my answers for everyone reading, not just the person I'm responding to, so please don't feel like I'm insulting your intelligence if it seems like I'm acting like you're a neophyte. :)


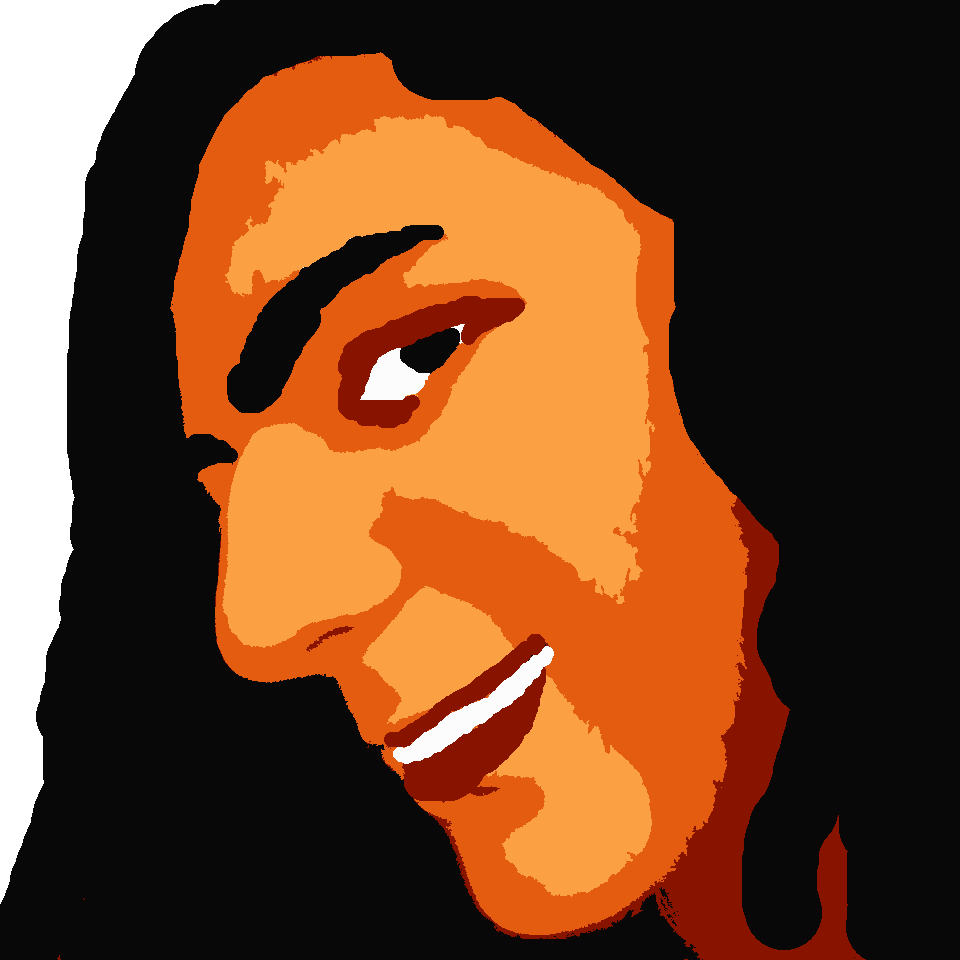
Thank you, but I ended up thinking of a simpler solution, I just need to move the triplet I want to delete to the top of the table, which I can do fairly easily. I do appreciate the help though.



I'd like to add that if you're always deleting in groups of three, it might be worth putting those triplets into their own mini-tables, and then storing those at each index in the main table instead. That way you can delete a single index with del() or Felice's method (if preserving order is important), or even just replace it with the last entry:
table = {{1, 2, 3},{1, 2, 3},{1, 2, 3},{1, 2, 3}} function delbyfirst(val): for ix=1,#table do if table[ix][1] == val then table[ix] = table[#table] table[#table] = nil return end end end |


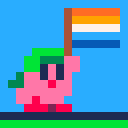
I think you should use deli() to delete an index of a table.
[Please log in to post a comment]