sprite flag problems
I've been brute-forcing sprite flag numbers for a while and I gave up after like 2000..
What's the number for flag 6+7?
I know that 6 alone is 64 and 7 alone is 128, but combined I have no idea..
Please send help, thanks


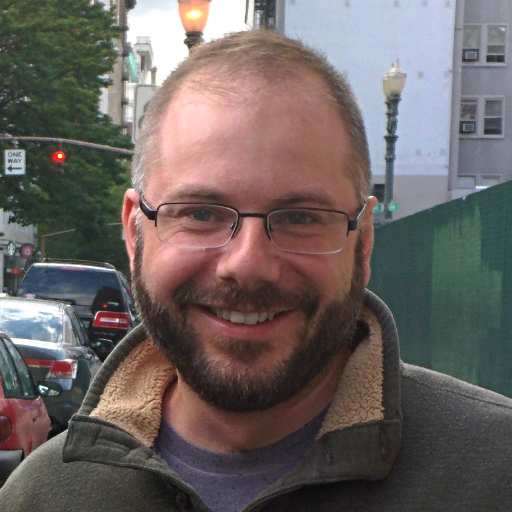
To understand sprite flags, it's easiest to learn how binary numbers work. Each place value in binary is double the place value before it. Like this:
1 = 00000001 2 = 00000010 4 = 00000100 8 = 00001000 16 = 00010000 32 = 00100000 64 = 01000000 128 = 10000000 |
So just like the number 437 means 400 + 30 + 7, the binary number 00001011 means 8 + 2 + 1. Or 11000000 would mean 128 + 64. And 10110001 would mean 128 + 32 + 16 + 1.
The sprite flags in the sprite editor are basically just binary numbers. If a sprite flag is turned on, it's like a 1, and if it's turned off, it's like a 0. So if you had the sprite flags ON ON ON OFF OFF OFF OFF OFF, you could think of it like 11100000. BUT, the tricky part is that sprite flags are like binary numbers in reverse. So sprite flags 11100000 are actually the binary number 00000111. And if you look at that number, it's 4 + 2 + 1. So the sprite flags 11100000 would be the number 7.
TL;DR The first sprite flag is 1, the second is 2, the third is 4, the fourth is 8, the fifth is 16, the sixth is 32, the seventh is 64, the eighth is 128. Look at which ones are turned on and add them together. So OFF ON ON OFF OFF OFF ON ON would be 2 + 4 + 64 + 128, or 198.


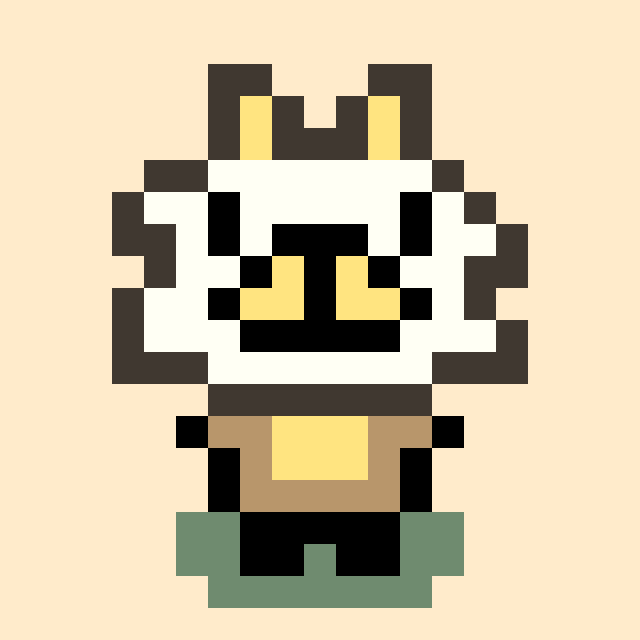
I guess im just being a idot? I thought you could test for multiple flags at ounce by saying fget()==the sum of your flags. but am I thinking of how you can draw the map useing map() with the sum of your flags? I thought I could of saved some tokens by testing both so if ethier was right I would work.. Am I doing it wrong or you can't test for muliple flags with fget()?


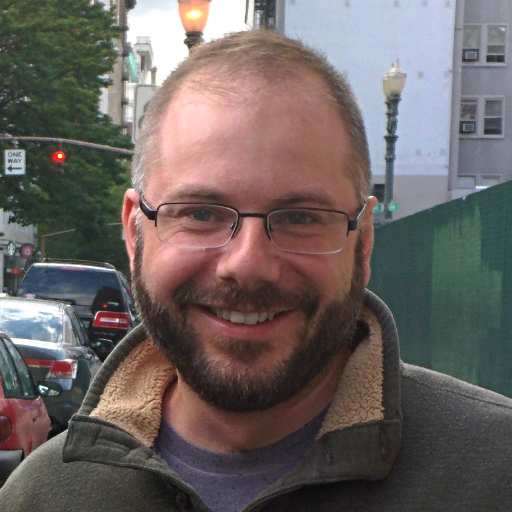
The method above is how you test for multiple flags at once. For example, if you wanted to test if the 1st, 3rd, and 8th flags were on, you see if your fget(sprite_num)==(1+4+128). (I expanded it out, but could just write 135 instead, of course.)
However, you can use fget() to test one specific flag at a time using numbers 0 through 7. For example you could write fget(sprite_num, 0) to test and see if the first sprite flag was on or not, or you could write fget(sprite_num, 4) to test if the fifth sprite flag was turned on or not. When used this way, fget() returns true or false instead of a number.


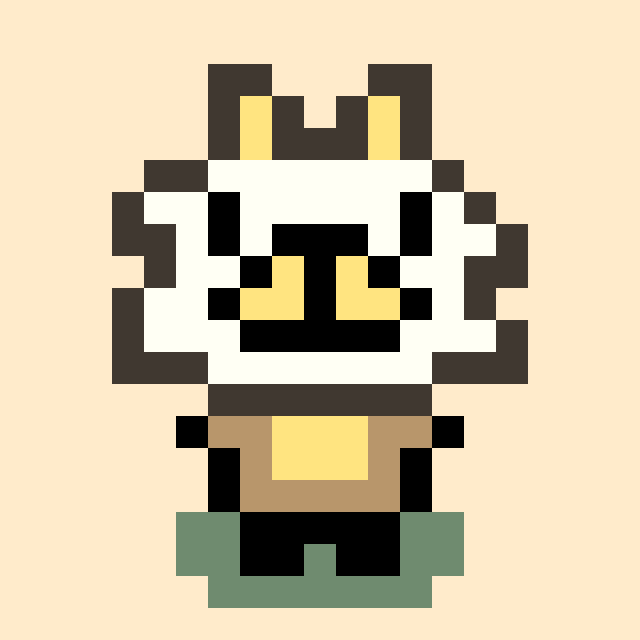
I just realized that I was being a Derp.
I had a if statment:
function drawtilelip() for i=0,23 do for i2=0,23 do if fget(mget(i,i2+1))==192 and fget(mget(i,i2))==1 then line(i*8,i2*8+7,i*8+7,i2*8+7,1) end end end end |
and I had map tiles some with flag 7 on, and some with flag 6 on. And I was testing if both of the flags were on even though that would never be the case..
Like I said before I was thinking about how map() automaticly will print it if it has any of the flags aslong as it's the right sum it becomes a or statement (I think if I remebred hopefully.) but here I was using it for a if statement and of course that won't work :/
thought I could save some tokens not having to use a or. sigh...
I never thought about just testing it as a boolein even though that's what im doing every time I test with fget, once again thanks for the help.
Im making a game where there's shading on the bottom of tiles and it needs to add it in if there's flag 7, or flag 6 beneath a tile.
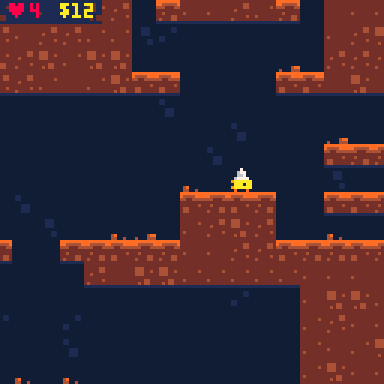


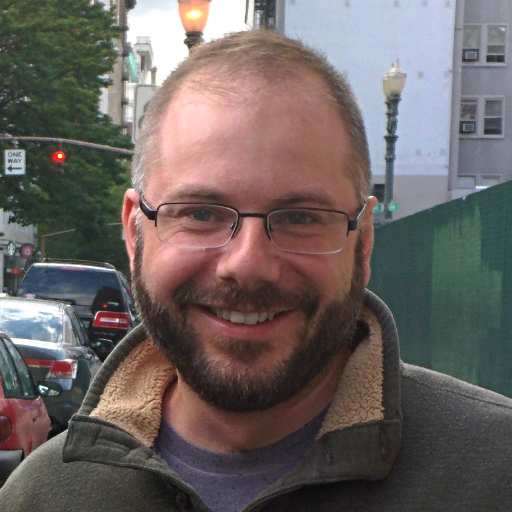
That makes sense what you're using it for. Glad you figured it out!
Also, looking at your sample code, one thing you might notice that a lot of people do is when they have a for loop inside another for loop, they'll often use i for the first counter variable and then j for the second one. Like this:
for i=0,15 do for j=0,15 do --blah blah end end |
Of course what you're doing works perfectly well, so don't change it if it's doing the job for you! :D I only mention it because you will see other programmers using that style a lot and doing it yourself means other programmers can quickly scan your code and that style will be familiar and very readable to them.



You can also use band(), bitwise AND, operator to determine if more than one needed flag is flipped.
Working off of the binary representation @MBoffin provided lets work up an example:
You are looking to see if flags 6 and 7 are set. The value of flags 6 and 7 being set is, 192 (128 + 64).
What we do with band() is apply a logical AND to two numbers.
To see if a sprite has those two flags set you use band() with the flag value you are looking for and your sprite's flags value. You will get your desired value back if the flag(s) you are looking for are set.
flg67 =192 sprflgs = fget(16) --no flag value provided with fget() returns the --value for all of the flags for that sprite result = band(flg67, sprflgs) --see if the result is your desired flags (6 and 7 which is 192) if result == flg67 then -- has flags 6 and 7 set else -- flag 6 and/or 7 not set end |
This is especially useful for when you are using many flags on your sprints and need to check a subset of them being set. 1 flag is easy, it's built in to fget(), but 2 or more is a bit more of a pain.
--It seems inline code formatting isn't working in the bbs


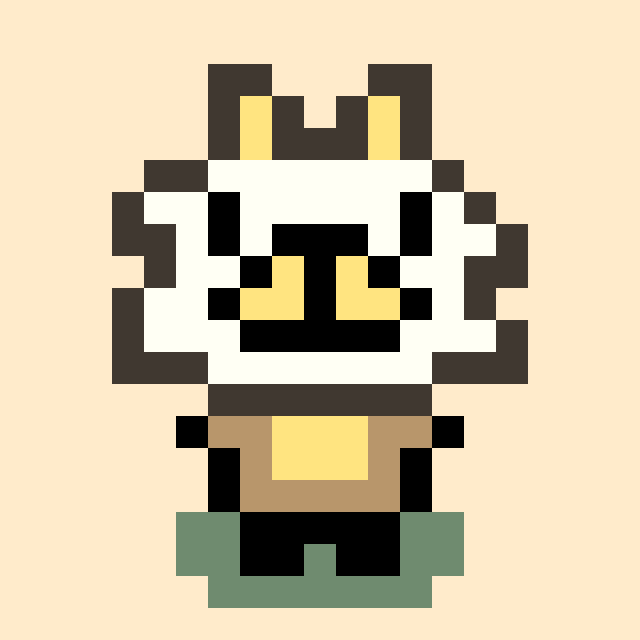
Thanks guys, Im just trying to compress my game as much as possible because I'm going to need every token I can get
[Please log in to post a comment]