Hi everybody!
It's been some time since I posted around here, and worked with Pico8 in general. Was a tough summer. But I come back with a second wind, partly because of the arrival of my PocketC.H.I.P.
Last Friday I started a project while I was on a train, to test out the device and how comfortable was to actually code with it. And I decided to post here some updates about the development of said project. So, without further ado:
--------------------------------------------------------------------------------------------------------------------------------
Behavior Trees
Most of you probably know about them, and have worked with them. For those which do not, I'll provide a quick explanation. Please correct me if I'm wrong, as I'm by no means an expert in this matter.
The Behavior Tree is a model widely used in the videogame industry to create goal-oriented plans using small, self-contained, behaviors. They came out in a conference about Halo 2 AI systems, and since then they've been used in lots of different projects, even outside of the domain of videogames (robotics, etc).
A behavior tree(BT) works by going through its nodes, from the root towards the leaves, computing these nodes according to certain rules.
The possible outcome of a node Tick() function is either Success, Failure or Running (usually). Each node computes its Tick() function analyzing the outcome of its own children.
e.g: The Inverter node's Tick() will return Success if its unique child returns Failure, and Failure if it returns Success.
So, in each Tick() (usually inside an Update() function), the tree will be computed and only some behaviors will activate, creating a composed, more complex, behavior.
If you're interested in reading more about them, here are a few nice links to articles and posts:
Gamasutra Article

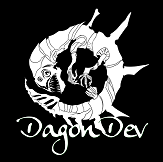
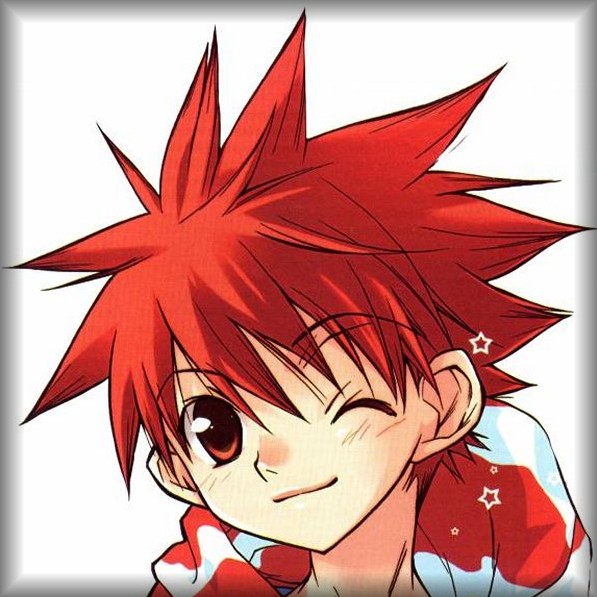
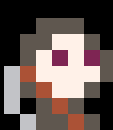
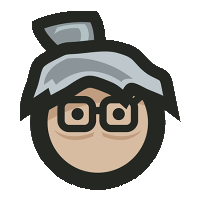
I'm back! It turns out my game had heavy slowdowns after this several Pico8 updates, but on the other hand I got a bunch of tokens! I optimized the game quite a bit, added a new enemy and more. Still not finished, but I hope I'll be able to play this on a PocketCHIP soon :D
I hope you like it!
DESCRIPTION
-----------------
You're the son of Death, he is out for holidays, and left you in charge of the whole soul reaping business. But for some reason, the undead got out of their graves and are wreaking havoc.
Your objective is to find all the scattered souls in the graveyard, and to find the source of this madness.
Everytime you die, you will lose a Soul. If you lose all of them, you die for real. Souls are also your score, so the more souls you have when you reach the ending, the better player you are :D
Right now there's no ending, no highscore screen, nothing. But you can still get a bunch of those souls placed on the map.
There are also two Great Souls, hidden in difficult to reach areas. Those are upgrades, that will allow ReaperJr to Double Jump or Charge his scythe and release a special attack.
[b]CONTROLS


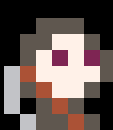





