So I'm trying to make a idle game and im using a timer that resets to 0 every 30 cycles to make a 1 second timer, my goal is to make the total number increase by 0.2 every second but after a wile it increases by 0.1999 instead and I have no idea why
heres my code:
function _init()
timer=0
counter=0
end
function _update()
if timer==30 then timer=0 end
if timer==0 then counter+=0.2 end
timer+=1
end
function _draw()
cls()
print("timer:",0,0)
print(timer,24,0)
print("counter:",0,7)
print(counter,32,7)
end


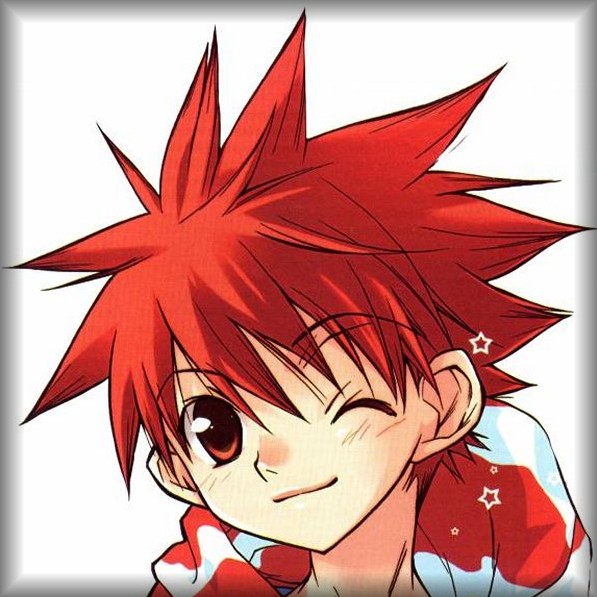
Hi @Tecnology_Wizz:
I was told some years back that PRINT for displaying non-integers is not 100% accurate. You may need to fudge the display. For instance, try out this code:
cls() for i=0,1,.1 do print(i) end |
The results you get back are:
0 0.1 0.2 0.3 0.4 0.5 0.5999 0.6999 0.7999 0.8999 1 |
To fix this particular incident, you can add a small amount:
cls() for i=0,1,.1 do print(i+.0001/2) end |
The results are now correct:
0 0.1 0.2 0.3 0.4 0.5 0.6 0.7 0.8 0.9 1 |



That's a really weird bug, even if I take the addition out of the loop and just manually add 0.2 a number of times to counter, eventually I get 3.3999.
You could always store the counter as a whole number counter+=2
and where you need it as a decimal divide it by 10 counter\10
, thus avoiding any weirdness with adding decimal numbers.


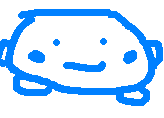
pico-8 numbers are fixed point, not floating points or decimal.
this was chosen to recreate the situation of old home computers, and because it makes us learn fun code tricks (like shifting bits instead of using division or multiplication by 2)
there is very little info in the docs:
https://www.lexaloffle.com/dl/docs/pico-8_manual.html#Types_and_assignment
https://www.lexaloffle.com/dl/docs/pico-8_manual.html#TOSTR
(more about bits: https://www.lexaloffle.com/bbs/?tid=38338)
the smallest value you can use to increment a number is 0x.0001
but for your use case here, you could simply add 1 and reset after 30 or 60 ticks (depending on your using 30 or 60fps)
[Please log in to post a comment]