Hi all,
First time poster and overall Lua and Pico-8 noob. I have played several of the games and watched a few videos on making games and now I'm playing around with creating basic functionality. However, I'm getting stuck with a syntax error that doesn't make any sense to me. Here is my source code:
room={} room.x1=0 --x1 coordinate (coord) room.y1=0 --y1 coord room.x2=127 --x2 coord room.y2=127 --y2 coord room.w=119 --room width room.h=119 --room height p={} --person object p.x=63 --start x coord p.y=63 --start y coord p.sprite=0 --start sprite function _init() end function _update() update_movement() end function update_movement() if btn(0) and not_border() then move_left() end if btn(1) and not_border() then move_right() end if btn(2) and not_border() then move_up() end if btn(3) and not_border() then move_down() end end function not_border() (p.x>1 and p.x<119) or (p.y>1 and p.y<119) end function move_left() p.x-=1 end function move_right() p.x+=1 end function move_up() p.y-=1 end function move_down() p.y+=1 end function _draw() cls() -- draw room boarder rect(room.x1, room.y1, room.x2, room.y2, 10) -- draw sprite spr(0,p.x,p.y) end |
The problem I am having is with my not_border() function. It says there is a <EOF> syntax error near 'or'
, but looking at my code it appears that I have my and
and or
statements written out correctly. Can someone help me understand what is wrong with this specific function and why I am getting the syntax error I'm getting? Thanks!


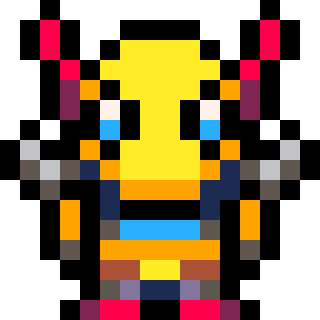
Since you want not_border() to return a True or False, you want to do this instead:
function not_border() return (p.x>1 and p.x<119) or (p.y>1 and p.y<119) end |


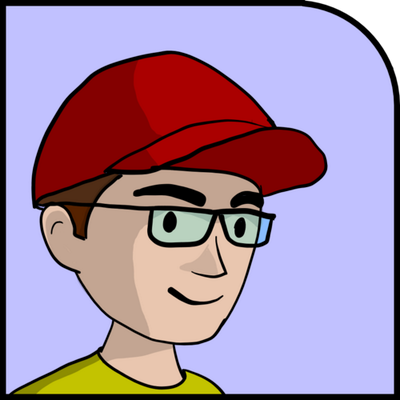
I believe not_border() wants to return a value?
Does it work better like this?
function not_border() return ( (p.x>1 and p.x<119) or (p.y>1 and p.y<119) ) end |
Edit : Yeah, what he said.



Bah,
Thank you. I grew up on Ruby, which doesn't require explicit returns. I saw that Lua was a scripting language and immediately assumed that it functioned with implicit returns. Now I know. Many thanks!



I've never ruby'ed. A non-assigning statement at the end implies the return value?
That's either neat or really messed up. I can't decide. :)



Sort of correct. In Ruby, whatever the final statement evaluated in a function is, that is the return value, even if it is an assigning statement. For example:
def my_foobar_method do my_var = "Hello World" end def my_other_foobar_method do "Hello World" end |
Both would return the string "Hello World". Some hate it. Some love it. I don't know that I either love or hate it. It is just what I started with and so it often becomes my default mindset when programming.



Hm, interesting. As most programmers end up doing, I fiddled with designing my own language. I made it so that what is effectively my function's 'end' statement (which is actually a loc/lang-independent symbol in my case) doubles as the return keyword. Similar. So I kind of approve. :)
[Please log in to post a comment]