Purpose: calculating a line-line intersection point
I've been trying to learn physics coding, which also means re-learning math I have long forgotten. Recent studies in vectors, sin/cos, etc. were difficult but successful, so this task seemed like it would be pretty simple. And yet....
I cannot see what I'm doing wrong here.
I've implemented line-line intersection algorithms as transcribed in various places all over the internet, and the resulting {x,y} is always the same. So the answers are consistent, but do not match expectations whatsoever.
Note: I understand this algorithm does not catch all cases, but rather I'm trying to show the simplest form of the problem I'm struggling with. It should be working for the case illustrated, as far as I understand. The line endpoints are chosen specifically to intersect on-screen, but the intersection algorithm will generate negative values in most, but not all, cases (?!?!?)
function line_intersection(line1, line2) local p1, p2 = line1.p1, line1.p2 local p3, p4 = line2.p1, line2.p2 local a1 = p2.y - p1.y local b1 = p1.x - p2.x local c1 = a1 * p1.x + b1 * p1.y local a2 = p4.y - p3.y local b2 = p3.x - p4.x local c2 = a2 * p3.x + b2 * p3.y local denominator = a1 * b2 - a2 * b1 local x = (b2*c1-b1*c2)/denominator local y = (a1*c2-a2*c1)/denominator print(x..","..y,0,120) return {x=x,y=y} end --l1 = {p1={x=10, y=25}, p2={x=25, y=10}} --THIS WORKS l1 = {p1={x=10, y=26}, p2={x=26, y=10}} --THIS DOESN'T l2 = {p1={x=0, y=0}, p2={x=60, y=60}} function _draw() cls() line(l1.p1.x, l1.p1.y, l1.p2.x, l1.p2.y, 7) line(l2.p1.x, l2.p1.y, l2.p2.x, l2.p2.y, 7) local intersect = line_intersection(l1, l2) circ(intersect.x, intersect.y, 2, 8) end |
Is there a trick to doing this in Pico-8 that I'm not understanding? Other attempts to translate algorithms (say from Wikipedia or Stack Overflow) were successful, so I thought I had a handle on things. It's frustrating being stumped by something that seems so simple. If anyone can point me in the right direction, I'd appreciate the assist.



The bane of the pico8 mathematician: numeric overflow :/
In the 'not working' case, these 2 values:
(b2*c1-b1*c2) (a1*c2-a2*c1) |
are overflowing (e.g. > 256*256), resulting in a (wrong) negative value.
Pro tip: use virtual coordinates (say, the whole screen is [0..1] and multiply by 128 for display)
Example:


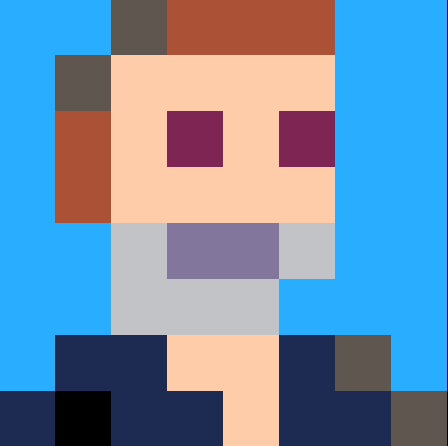
Gah!!!! My brain didn't even go down that route of thinking... I'd never encountered it before! Thank you very much, I've learned a valuable lesson with this.
[Please log in to post a comment]