I could easily find a tutorial on how to make a bounding box collision function online, but I could not easily find an example of iterating through a table to test for collisions, so I made it myself in hopes to help new programmers.
-- collision example -- andy c function _init() -- create player object named block, each object in this example will need an x and a y block = {} block.x = 64 block.y = 64 -- create 6 walls walla = {} walla.x = ceil(rnd(120)) walla.y = ceil(rnd(120)) wallb = {} wallb.x = ceil(rnd(120)) wallb.y = ceil(rnd(120)) wallc = {} wallc.x = ceil(rnd(120)) wallc.y = ceil(rnd(120)) walld = {} walld.x = ceil(rnd(120)) walld.y = ceil(rnd(120)) walle = {} walle.x = ceil(rnd(120)) walle.y = ceil(rnd(120)) wallf = {} wallf.x = ceil(rnd(120)) wallf.y = ceil(rnd(120)) -- add the walls to the wall table walls = {walla, wallb, wallc, walld, walle, wallf} -- here's an inspirational quote: "wall-e" - wall-e end function collision(wall) -- basically if it overlaps on two axis, it's a collision if(block.x < wall.x + 8 and block.x + 8 > wall.x and block.y < wall.y + 8 and block.y + 8 > wall.y)then return true end end function _update() -- basically, move the coordinate, and test a collision for every object in the table. -- if any of them return true, move back the coordinate to where it was -- left if (btn(0) and block.x > 0) then block.x -= 1 for i in all(walls) do if (collision(i)) then block.x += 1 end end end -- right if (btn(1) and block.x < 120) then block.x += 1 for i in all(walls) do if (collision(i)) then block.x -= 1 end end end -- up if (btn(2) and block.y > 0) then block.y -= 1 for i in all(walls) do if (collision(i)) then block.y += 1 end end end -- down if (btn(3) and block.y < 120) then block.y += 1 for i in all(walls) do if (collision(i)) then block.y -= 1 end end end end function _draw() -- iterate through the table and draw a blue square for each block. draw a red square for the player cls() spr(1,block.x,block.y) for i in all(walls) do spr(2,i.x,i.y) end end |


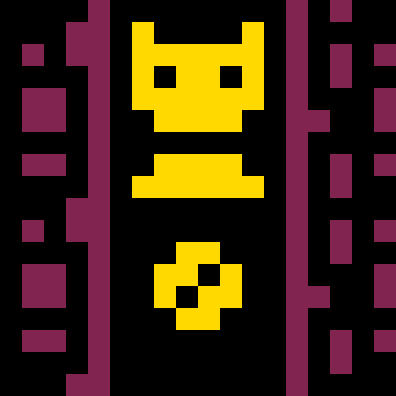
Tysm! This is exactly what I was looking for. Very simple explanation too! You're a lifesaver. <3



suggest to look at the demos/collide sample for a better example (using tables, proper function parameters, variable player width...)
[Please log in to post a comment]