Hi, I'm practicing how to do object oriented programming in PICO-8 but struggling a bit to map things in my head between OO design and actual game code. My practice project is doing MBoffin's top-down game tutorial with an OO approach but my long term goal is to do either a top-down adventure/rpg (=Zelda) or turn-based strategy (=UFO X-COM).
The reason I want to do OOP is my Java dev background and also personally I don't like procedural code, it feels so messy and cluttered. I'd like to have the behaviour and presentation of an entity in its class instead of spread around the application but that's the difficult part for me at the moment.
Bunch of questions:
- Is there any point of doing a class for a singleton entity, eg. player actor? As my player1 object will be the only instance I could as well have that and not bother with the prototype. Now I have something like this:
-- prototype player = {} function player:new(o) local o = o or {} setmetatable(o, self) self.__index = self return o end function player:draw() spr(self.sprite, self.x*8, self.y*8) end function player:move() if (btnp(⬅️)) newx-=1 if (btnp(➡️)) newx+=1 if (btnp(⬆️)) newy-=1 if (btnp(⬇️)) newy+=1 ... end function _init() -- actual object player1 = player:new( { x = 10, y = 10, sprite = 2 } ) ... end |
-
A wider question based on above: What entities should I have as classes (or "prototypes" in Lua OOP)? Player actor, NPC characters/enemies, world map, world objects (text signs, anything interactive), ...?
-
What patterns/frameworks/templates you use for game projects? State machines, OOP, functional programming, ...?
- Any pointers to any non-minified game source code to study? Something with longer that 1-char variable names and comments? Anything good in GitHub?


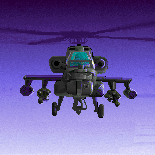
Oh. ECS! So, I answered most of my questions by reading more of the BBS and learning about Entity-Component-Systems architecture. Time to pivot :)
If someone else comes along the same trail, here's my notes from the last hour or two:


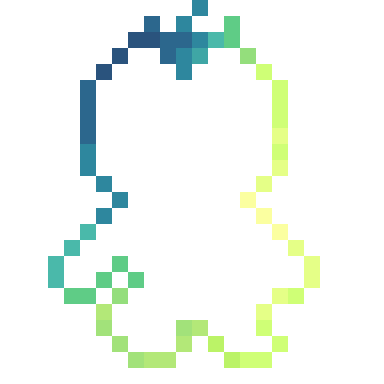
Bookmarked this thread for future reading, thanks for sharing your research! I'm still fairly new to programming and this is my first time hearing about this kind of programming. What exactly is it useful for?


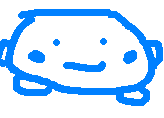
Lua is object-oriented, PICO-8 gives us functions to do things rather than objects with methods, and games can be written with multiple styles (imperative, functional, OOP, ECS…)
Concepts from Java don’t always translate well, as its version of OOP has many constraints and boilerplate. It is also class-based OOP, whereas Lua is based on prototypes (like JavaScript). In short, rather than having classes (define behaviour) and objects (instance of a class), you have tables (defines data and behaviour) that can get some stuff from another table. Here is a thread about prototypes and metatables, with links to other threads and Lua documentation: https://www.lexaloffle.com/bbs/?tid=38095
About ECS:
> Entity Component Systems are design patterns where entities are generic collections of components and logic
> is written for specific component types. It's popular for game design as we often have many 'things' that
> don't always fall within clear cut types.
From https://www.lexaloffle.com/bbs/?tid=30039
Links for more advanced readers:
Great read about OOP: https://eev.ee/blog/2013/03/03/the-controller-pattern-is-awful-and-other-oo-heresy/
More about prototypes: https://gameprogrammingpatterns.com/prototype.html


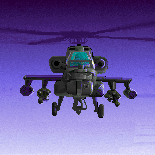
@le dook I'll continue my dabbling with ECS pattern in this blog thread: https://www.lexaloffle.com/bbs/?tid=39315


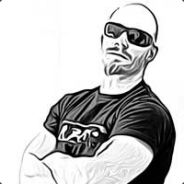
Some short ideas with regards to OOP:
- organize your classes by their software features, not by real world objects
- if you're having problems mapping your objects, the reason might be that you want to OO for OO's sake instead of the actual need/ benefit of OOP
So, what aspect of the code do you want to improveby making it OO?
[Please log in to post a comment]