Thought you guys might like it. I needed it to programmatically randomize the look of sprites in my map.
With peek you can read a 32-bit value, while a pixel color is 16 bits. One address therefore holds two pixels. A bit of arithmatic does the trick.
Long version:
-- set sprite pixel -- s: sprite number -- x: x position of pixel in sprite [0..7] -- y: y position of pixel in sprite [0..7] -- c: new color function ssp(s,x,y,c) addr=flr(s/16)*8*64+(s%16*4)+64*y+flr(x/2) -- current decimal address curr=peek(addr) -- current 2-pixel value if(x%2==0) then -- need to change pixel on the right lcol=flr(curr/16) rcol=c else -- need to change pixel on the left lcol=c rcol=curr%16 end ncol=16*lcol + rcol -- new decimal memory value poke(addr,ncol) end |
Shortened version:
function ssp(s,x,y,c) a=flr(s/16)*512+(s%16*4)+64*y+flr(x/2) -- 2-pixel address v=peek(a) if(x%2==0) then poke(a,16*flr(v/16) + c) else poke(a,16*c+v%16) end end |



I don't know if he was aware that we have sset and pal...


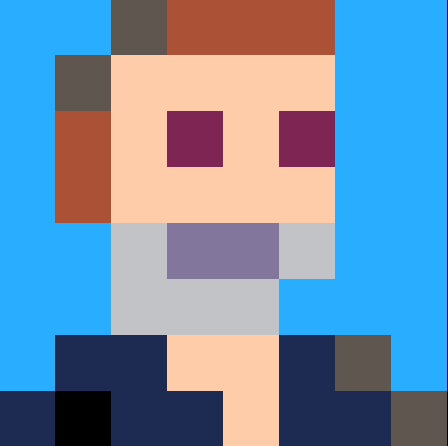
I believe peek() only reads 8 bits, and peek4() reads 32. Pixel colors are definitely only 4-bits (hence 16 color palette).


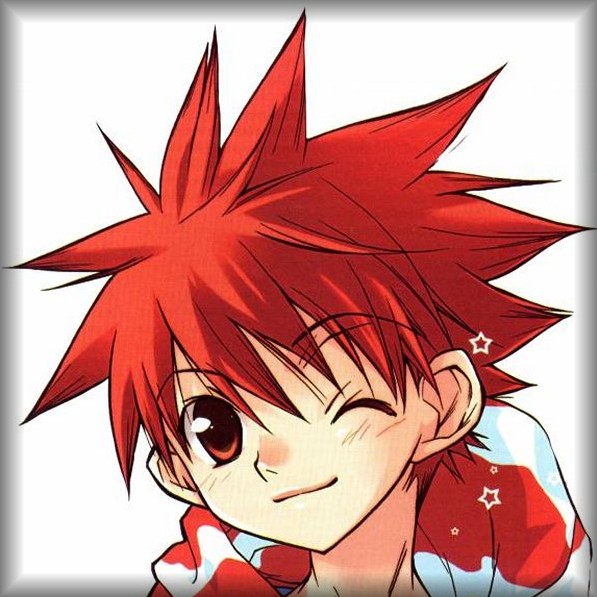
Here's a shorter version. Multi-colors a single sprite:
11-lines of code.
cls() x=48 y=52 spr(1,x,y) pal(5,1) pal(6,13) pal(7,12) spr(1,x+8,y) pal(5,3) pal(6,11) pal(7,10) spr(1,x+16,y) pal(5,2) pal(6,8) pal(7,14) spr(1,x+24,y) pal() -- reset plotting palette print("rupees!",50,y+12) |
NOTE, it is important to know that this does not change the SCREEN palette but the PLOTTING colors. So any other items already on the screen will not be affected by the palette change.
A marvelous command when used properly.



By the way I have adapted your function to this:
function ssp(s,x,y) a=flr(s/16)*512+(s%16*4)+64*y+flr(x/2) -- 2-pixel address v=peek(a) return v%16 end |
This just returns the color of a certain pixel. Useful for me for another project.
[Please log in to post a comment]