Despite how that sounds, no, it's not a schizophrenic having an argument with himself, although I have seen this before. :)
No, it is the unique ability of a FUNCTION in PICO to change the values of arguments as they are entered. I.E.:
FUNCTION ADDUP(VAR A,B,C) A=3 B=4 C=5 RETURN A+B+C END |
In this, while the return value is A+B+C, the argument entered for A will CHANGE, just like a local variable upon exiting the function.
X=7 Y=8 Z=9 ADDUP(X,Y,Z) PRINT(X) |
The result will be "3" since X was directly modified.
This is useful for calling functions with an input that is changed by the ending result.
While this can be done in other programming languages, can it be done in PICO-8 ? And if so, how ?



[b]Tables,[/b] functions, threads, and (full) userdata values are objects: variables do not actually contain these values, only [i]references to them[/i]. Assignment, parameter passing, and [i]function returns always manipulate references to such values[/i];" |
Either use a global, (i.e. don't pass it. I know, that's not what you want) or make it a part of a table, and pass the table in. (and since tables are always references, any changes made to them effect the original)


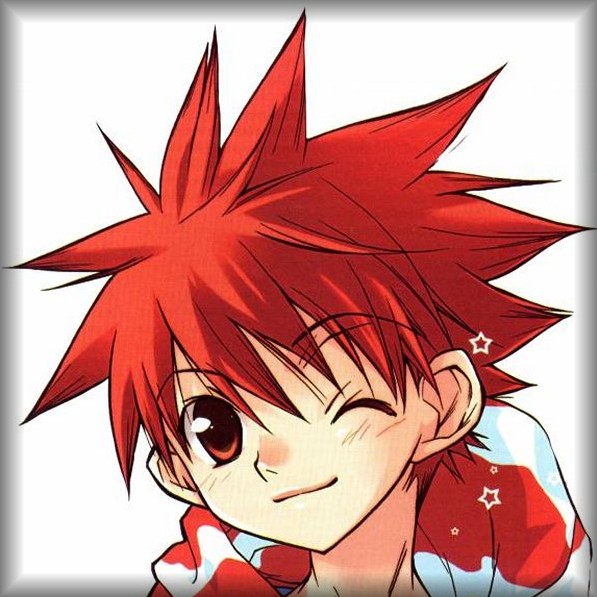
Yeah ... trying to avoid tables just for 2-arguments in a function.
I'm already using a global. Nasty. Really should be an option to modify an argument in a returning function.
Thanks, Tyrone. Just confirming what can't be easily done.
-- yank comma delimited text function yank(t) local r if sub(t,#t,#t)!="," then t=t.."," end r=sub(t,1,instr(t,",")-1) yanked=sub(t,instr(t,",")+1) return r end |
Where YANKED is the global.



I'm not 100% sure what you're trying to do, but it looks like multiple assignment could help you.
function f(a,b) a=a+1 return a,a+b end a=3 a,x=f(a,10) |
The above should set the parameter a to 4 and also set x to 14.
I would actually rewrite the function to this to keep it clear what's going on.
function f(c,b) c=c+1 return c,c+b end |



Oh, and the most proper way is to use "return" more robustly.
function yank(t) if sub(t,#t,#t)!="," then t=t.."," end return sub(t,1,instr(t,",")-1), sub(t,instr(t,",")+1) end |


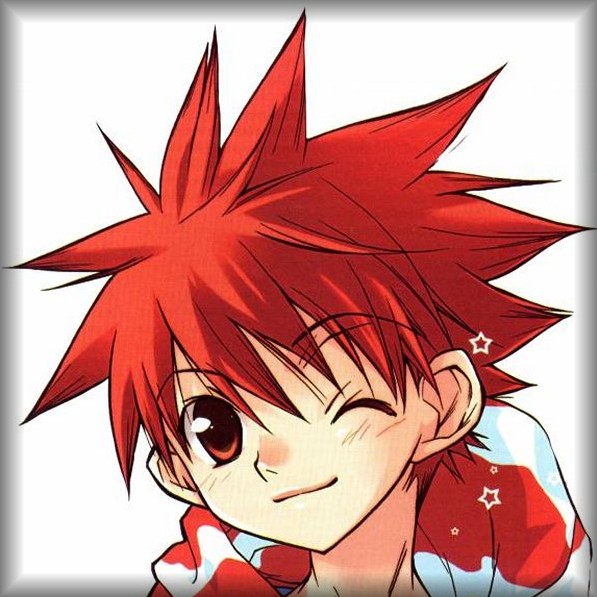
That returns 2-arguments though, right ? That's not what the code needs.
And yes, I can cram it all in one line:
pset(x+sgn(i%2*2-1)*r,y+sgn((flr(i/2)%2)*2-1)*s,c) |
But that's not a very good programming practice as it becomes unreadable to others and unmanageable for yourself.
Instead:
a=x+sgn(i%2*2-1)*r b=y+sgn((flr(i/2)%2)*2-1)*s if t==0 then pset(a,b,c) else line(a,b,x,b,c) end |
So no, Rytrasmi. I disagree. my YANK routine is fine where it's at.
You can work smarter, not harder - but if no-one can read your smart work, then what's the point ?
It's better to work harder and make your code more legible and easily understood by others - including yourself when down the line you can't remember how a complex routine works that you wrote months earlier.
By padding out the calculations and formula, it is that much easier to read and manage. :)



If you want to change a passed variable from within a function, (as well as set whatever other value like you're already doing,) then it sounds like multiple returns is exactly what the code needs.
edit: if you don't know when you call whether you'll be changing the variable, just "a,b=func(stuff)" and inside the function you can can do a test and return the unchanged second value.
function tworeturns(a,b,stuff) if (stuff) and GLOBALFLAG then return a, b+1 else return a,b end end |
If you know ahead of time and you don't need to change it, then you can just leave off the second variable and ignore the extra return values. Below is perfectly fine.
function tworeturns(a,b,stuff) return a+1,b+1 end x,y=10,10 x=tworeturns("blahblahblah") |



Weren't you just bragging about having 5 functions on 5 lines? Plus, your (personal) issue with readability has nothing to do with multiple returns.
Why do you even ask for help???


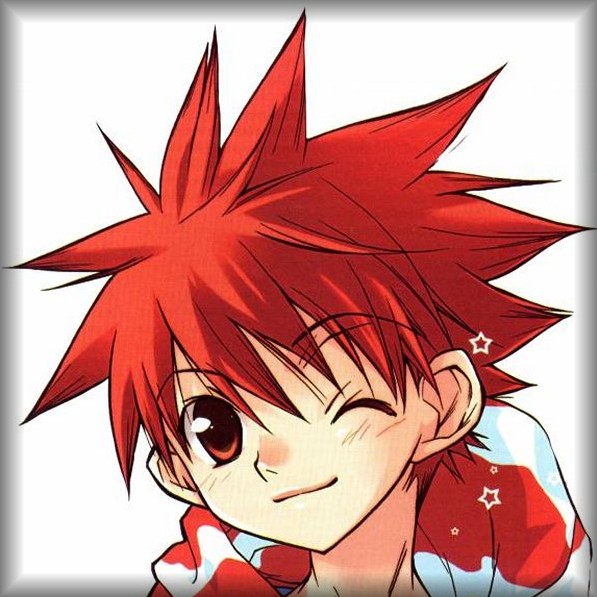
Rytrasmi, that is code I don't plan to use, and it's for everyone else.
If it were in my code from a tool or something I would use myself, I would pad it out.
Now, if it's someone ELSE's code, they are more than welcome to compress and cram it. It's software that I'm using, especially if it's a little tricky for me to understand THAT I WROTE, then I need it padded out.
Please don't take such a negative attitude. I think we're all trying to learn coding here and that doesn't help.
And no, I don't brag - well ... except maybe for that Fireball routine I did recently. I rather like that. :)



@rystrami To keep his name in the LP position on every single thread in the forum, and make the bbs revolve around him. Barring moderation, which I doubt is ever going to happen, I think disengagement is a partial solution.


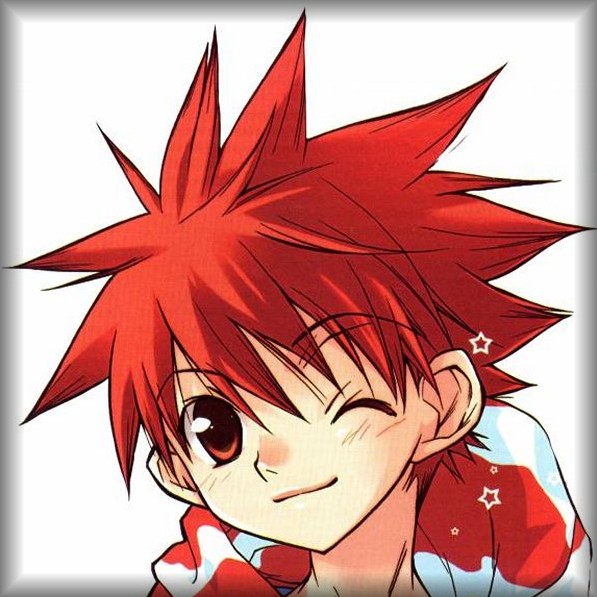
Tyroney, I'm trying to understand what you're writing as it looks like an interesting way of returning extra values from a function.
function tworeturns(a,b,stuff) return a+1,b+1 end x,y=10,10 x=tworeturns("blahblahblah") |
I'm getting back an error from this code you wrote though:
Line 2: Attempt to perform arithmetic on local 'A' (a string value)
Fweez, I type 135wpm and am retired, meaning I can be here every day if I so choose. If I see a post of interest, I will add to it. If not, I don't.
And I agree, disengagement is a good solution for you.



I messed up the call. The point of the example was it's fine to ignore return values and not use them.
function tworeturns(a,b,stuff) return a+1,b+1 end x,y=10,10 x=tworeturns(x,y,"blahblahblah")--leaves y untouched |
edit: glad the lightbulb came on. Here's hoping some kind of PM system gets added to this board sooner rather than later.


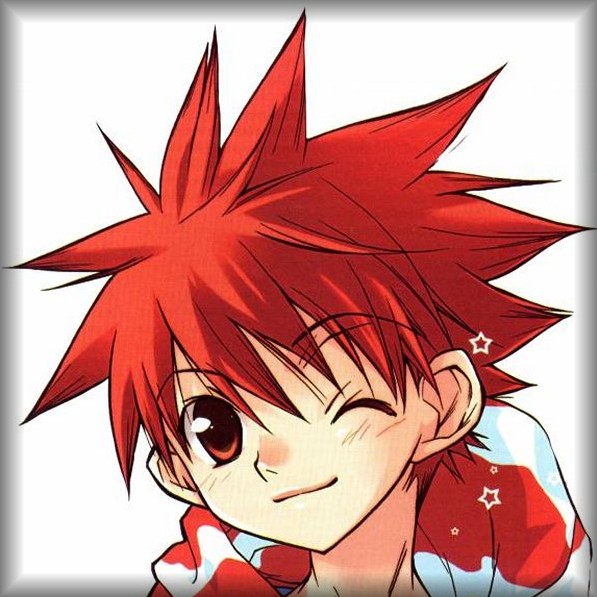
Well now wait just a minute, I think I might understand this. Let's see ...
function fruitme(t) return "["..t,"]"..t end cls() a,b=fruitme("apple") print(a) print(b) |
[apple
apple]
Wholly smokes that's useful, Tyroney ! Wow, I have never seen a programming language do that before ! Thank you so much !
I know you tease me about it, but once again this is something I need to wrap my head around. A turban maybe ? :)



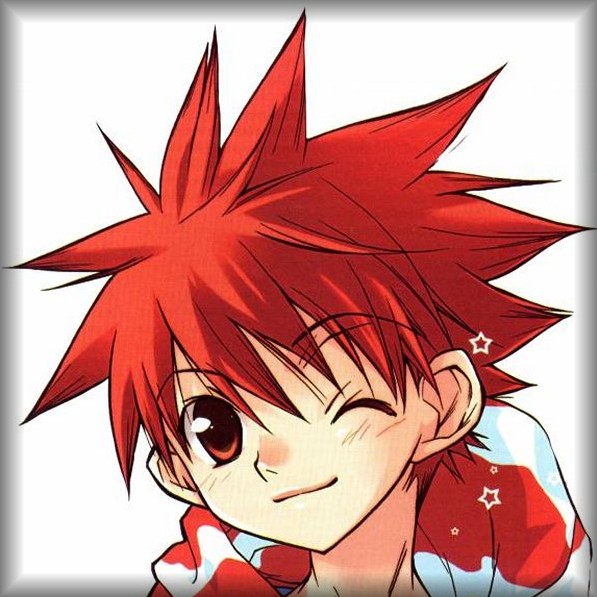
Rytrasmi, there is a famous saying I rather like. See where you fit in.
Great minds talk about ideas.
Normal minds take about events.
Smaller minds talk about people.
Me ? I learned a great idea today, to send and retrieve 2-arguments to a function, thanks to the inestimable and incomparable Tyroney.
And what are you two talking about ? :)
I hope spending time here was just as helpful as mine was.


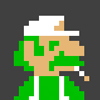
dw817, honestly, there's a really conspicuous sucking-the-air-out-of-the-room phenomenon at play here, whether you want to acknowledge it or not. You are posting so much on the site -- and frequently in a sort of uncool and pushy "let me tell you why your idea is wrong and you should do it my way instead" sort of way -- that it's impacting a lot of other people's ability to use the forum without annoyance.
And that annoyance is manifesting as people starting to get short with you, which in an unmoderated forum is the only tool that exists to give you feedback that your behavior is a problem.
The thing is: it's great that you're digging PICO-8, and that you're enthusiastic. It's great that you have spare time to dig in on it. Those aren't bad things, they're totally welcome. But you have to choose between a couple of ways to express that in terms of your interactions on the site here:
-
Moderating your own volume of output to a reasonable low level, or
- Being seen increasingly as a nuisance by everyone else.
I don't think you're trying to be a nuisance, but until you actually recognize the outsized footprint your participation is having on the site it's what is going to continue to happen. It'll only get worse over time, too, as more people lose their patience with your hyperactive posting.
My suggestion, and I make this in good faith and with genuine encouragement, is to find another channel by which to communicate or collate the bulk of your thoughts and ideas about this stuff. Start up a simple blog and write daily about your PICO-8 thoughts and ideas, maybe; that'd be a good sort of journaling-centric approach.
And if you do that instead of posting a dozen or two dozen times across a whole bunch of threads, you can make instead two or three comments over here on a given day about the stuff that's most useful for other folks. Splitting the difference like that will allow you to integrate into the community here in a much more positive way.
As it's going right now, that's fundamentally not what's happening. You can ignore it or refuse to acknowledge it, but that's just going to lead to further acrimony and unhappiness all around.


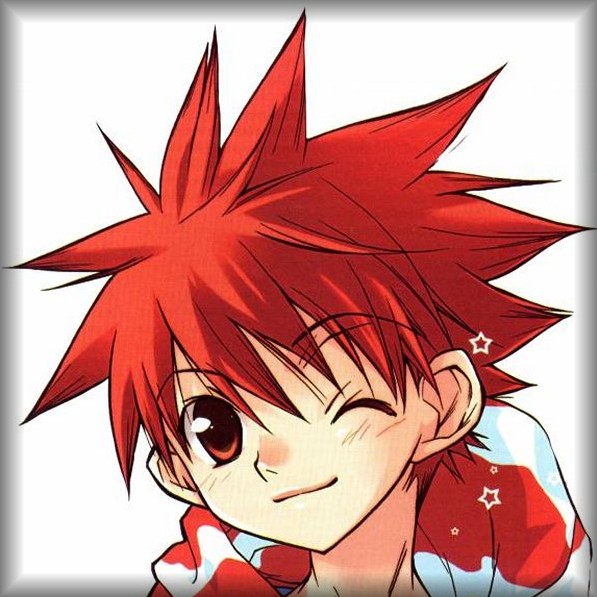
This is really not the time or place to be talking about users - but so be it.
I am an active member. No doubt. I think you will see that in many ways I give compliments and encouragement to others - this must be ignored I suppose.
I have had good brainstorms with others and solved many problems and written many interesting codes. This too must be ignored I suppose.
Josh Millard. There are no problems until someone states there is. You are stating a problem - and so now there is.
The question now becomes, how do I choose to handle it.
Clearly direct confrontation to attacks were not expected from me in reply. I should've ignored them entirely.
By not doing so, it becomes clear that I am a few things, magnanimous, and obnoxious. Two things that really don't go too well together.
I will consider your words, but this, "find another channel by which to communicate" is rather harsh I believe.
You are essentially telling me to leave where "I cannot be found."
And I will tell you, I'm not leaving. Not with the interesting and sometimes acerbic conversations I've had thus far.
Clearly I've knocked someone down a few notches in my arrival - I'm not going to point fingers.
But let me assure you. No-one, absolutely no-one wants to be thought of greater than any other person. To do so defeats the entire purpose of their existence.
I will monitor the "unhappy" posts, but if they continuously only appear from "the select crew" then your words today were empty and meaningless as I am harped on from a specific 2-3 personages in here in ANY posts or replies I make.
If you want to talk to me, I listened. Put that down as a checkmark.
Now the real question is, did you have anything to say to those who encouraged me to defend myself in here ?
No ? Or Yes ?
And be assured, I am active ALL over the Internet - not just here.
I cover a little over 8,000 websites, from art, to music, to writing stories, poems, and entire books, to philosophy, discussion of conspiracy theories, practices and belief in different religions, and many MANY other programming languages outside PICO, including some languages I developed myself for which I still have active members.
Don't ever believe I am here and here only. Don't ever attempt to credit yourself with that.
... in return, I will refrain from making any suggestions for other games I would like to see as you deem this, and quite likely, obnoxious behavior - unless someone asks me for ideas and suggestions.



This is going to sound rude and borderline insulting, but I'm not sure if I care enough to try to soften the blow.
I just wanted to chime in and say that I'm glad that other members are speaking out against dw817's constant and forced presence on this forum, and I'm certain there are several others who have taken issue with it but haven't had the courage or opportunity to speak out.
As joshmillard puts it, you are sucking the air out of the room. You are the kid in the classroom who is constantly raising your hand to be the first one to answer every question the teacher throws at you. This is not inherently "wrong" or against the rules or whatever, but it makes the space revolve around you and makes the people around you grow apathetic, because "what's the point in engaging when they are going to make it about them anyway?". You are not integrating into the community, you are making YOU the community. You are an annoyance.
Your way of constantly forcing yourself into Every. Single. Thread. has effectively killed most of the sense of enjoyment I had in participating in this otherwise amazing and supportive community. Very few of your replies feel genuine, rather, they seem like elaborately plotted ways for you to make yet another thread about yourself. Every time I load up the BBS index and see that the last posts in the most recent threads are all signed by you I die a little inside.
I'm not sure if this narcissistic "pushing yourself into everything" thing is something you are actively trying to accomplish or if it's just a socially clumsy mishap and that you mean no harm, but in my own uneducated opinion the way you structure your posts and your way of humblebragging about various achievements like past programming endeavors or absurd statements like "I cover a little over 8,000 websites" like you did just above makes my suspicions lean strongly towards the former.
As far as it affects me, there are two resolutions to this - either you take what I and several other people in this thread have already said to heart and try to change your ways and all will be well, or I decide that my continued engagement with this forum is not worth the hassle of having to deal with your antics and I try to find alternative channels for me to discover, share and talk about Pico-8 and other amateur gamedev projects.
Peace.


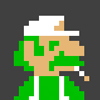
> this must be ignored I suppose
In fact, I made an effort to explicitly acknowledge and laud your genuine enthusiasm. The point is not that you bring nothing to the site, but that the way in which you currently participate is making the site worse for others. I'm suggesting you modify that approach to participating, not that you shove off.
You can take the feedback you're getting -- from multiple people, out of the larger pool of folks who have been so far too polite to say anything -- in stride and try to meet folks halfway, and that'll lead to a good outcome all around. That's what I'm hoping for!
Or you can insist that the problem is other people, that having good intentions means you can't possibly create bad outcomes, and as a result continue to create an unpleasant atmosphere for other people.
I know criticism is difficult to hear and easy to take a defensive stance in response to. I sympathize, on a person-to-person level. But this is really, genuinely, an issue where you are having a significant negative impact on a community larger than yourself. I'm not suggesting you leave, I'm just suggesting you take some feedback and work on reducing that negative impact while otherwise continuing to participate in and enjoy this place.


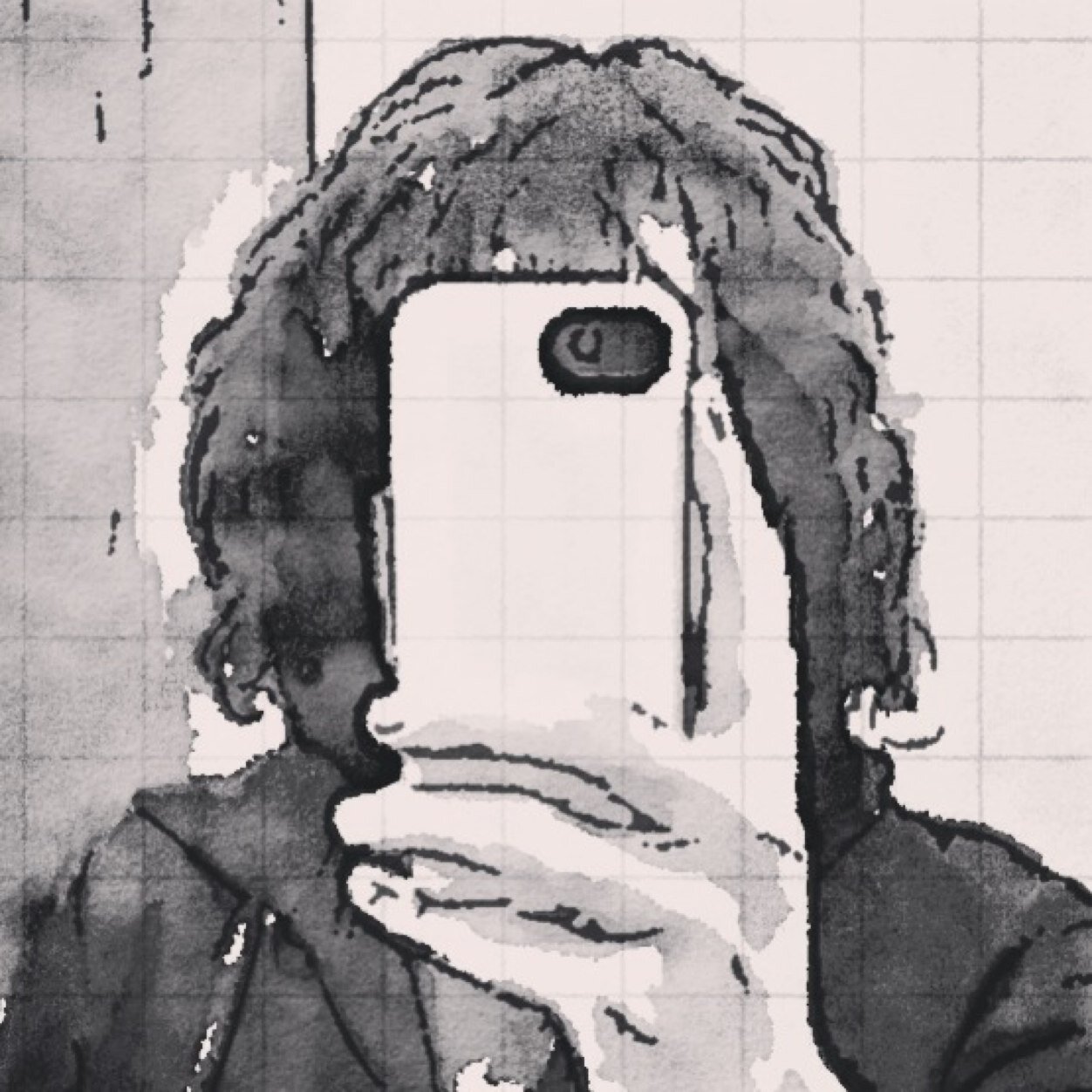
Hey dw817—I just wanted to chime in and thank you for your contributions to the forum, including the byte-to-string routine which was a life-saver for me.
Nobody wants you to leave—but this is a small community, and injecting yourself into every single conversation can be as off-putting in a virtual forum as would be in a real party. The content of your most frequent posts—quick musings, jokes, asides, etc.—would be better suited to an outside blog, as joshmillard suggests.
Posts to the Pico-8 forum should be brief, focused, and infrequent. That's both representative of the culture here, and good etiquette in general.


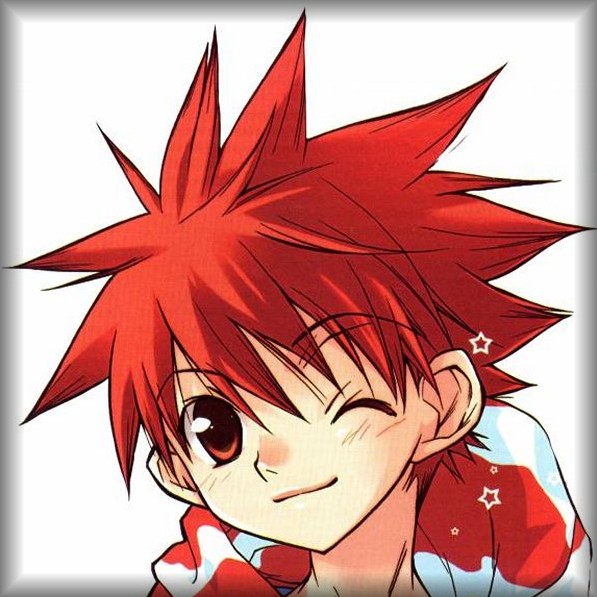
I think you all will be pleased to note that it has been the month of October that I committed myself to focus on PICO-8.
I have already received PMs from Writer's Cafe, one such place I inhabit, when am I coming back to work on my writings ?
With a little over 43,000 readers on my site alone, having written 4-books, science fiction and biographical, and one 2/3 of the way complete, top that with a a popular series I started nearly 5-years ago and was committed to writing in it every week.
Why am I such a busy person and obsessively active in all areas ?
Well for one, I am bipolar, I have been in and out of mental wards a good portion of my life, am classified schizoaffective, and take meds to handle auditory and visual hallucinations. Seroquel if you really want to know - and that is the strongest anti-psychotic out there on the market today.
And that's no secret. I publicly mention that in the beginning of my biography.
I am also alone. Aside from my sister and my Dad dying 8-years ago - I have no other family, period. Thus I try to engage people in active community and on common ground - in this case, coding and learning about PICO-8.
Curiously for ALL the posts I did at Writer's Cafe, there is not one person - not one complained about my writing. Not for all the years I had been there, neither Xanga when it was up and active.
It DID, however, attract cyberbullies though, very serious and very angry that I was in the spotlight.
It also earned one of them a very solid police record by going out of bounds from friendly Internet chatter and actively stalking me at my home. Police don't take kindly to stalkers.
However, to keep this friendly. It is during this month of October I wanted to work primarily in PICO. It is why I have been here, open, forward, honest, and engaged.
Some of you can't stand that. As one said, "see the last posts in the most recent threads are all signed by you I die a little inside."
I am very terribly sorry for that. But I have good news for you that will return life to your body. Read on.
The good news is I will not be here for this type of dedicated activity next month (6-days from now).
I will be working on and in other sites, and especially on my books and writing.
It has been educational being here, not just code, but to see who the "guards" are (or think they are).
Who it is that speaks up against others, and those who just like to join in and kick a dog when he is down, happy to attack someone, anyone, just to get it off their chest.
Couched of course in friendly "advice" and "helpful criticism."
It was not a total loss to see who engaged in this parlay. Touche.
Likely this combined negativity towards a hated member will earn you great respect, admiration, and many friends in the days to follow - and I wish you the best in that. We all need a whipping post I suppose. Hitler had Jews.
My friend Chris once told me that my existence is because of Maslow's Law Of The Hammer. He said I was a 6-pointed divot, hammers into a 7-point the day I was born, and ever since then, I've tried to conform.
There simply MUST be a bad guy in every scenario. In my case.
To answer your other question. Yes, I did raise my hand often in class, I did talk with every teacher after class, I did always offer to do extra homework, to volunteer in a variety of community activities for many years, and yes from youth to adult I did even talk to my preacher in his vestibule every Sunday with questions about his sermon.
I was a curious fellow. Insatiably curious about everything in the world. I could taste ideas and drink concepts. And I was always hungry.
And I always had questions, and sometimes good advice - because of listening, patience, and dedication to finding the answers on things. And yes, bullies followed me in huge DROVES.
If you ever get around to reading my biographical work you will see I was bullied all my life for being so engaged in activities and inquisitive, and being favored by my teachers, the perfect teacher's pet - many years before the Internet. Years in fact before BBSs.
Curiously, how I will handle this will be completely contrary to the way ANY of you would were the terms reversed.
I will not get angry nor point out this is a type of indirect cyberbullying. Because many of you will say it is not - especially since it is not you that is being criticized here.
No.
I will politely nod, "Say thank you for your concern, I will struggle to modify my behavior," and, "I appreciate you for bringing it to my attention." and if I'm especially game say, "I will talk it over with my doctor." which I do see every week.
And I will TRY to read where the positive is in the statements (try very hard with some of you).
It's the best I can do under the circumstances, and I think that will warrant some type of victory for some of you - you are welcome to it.
"Yeah ! That idiot finally got it ! God ! Just who the hell does he think he is !? Get the F out of here already !"
Bottom line. You won, but sadly, you never played. I had already queued at the end of the month to be elsewhere - so you can go back to Pico-8, quite likely without me.
I may return occasionally to post a cart and you are more than welcome to seethe angrily over the fact I dared show up again. I wouldn't expect anything less.
Nonetheless.
Muscura and you other folk who seem to believe I helped and contributed, I thank you. But I am not going to stay in this red target zone painted for me, not after 6-days.
For they will find it exceptionally hard to "constructively" criticize me when I am not even there.
So - if any of you want to join the bandwagon and get in your kicks on a complete stranger. Go ahead. I'm open. You have 6-days to do so.
...
You know what's really hilarious ? Tomorrow, I'll likely feel completely the opposite here through the miraculous power of bipolarism.
I'll actually believe what was written against me, and be so teary-eyed and hating myself and suicidal I would leave PICO and never return. It's quite amusing actually - you just have to have the right mindset to see the irony and humor in it.
Still ... a lot can happen in 6 days. 6 days, 144 hours. Busy busy busy. No time for sleep.
Bring plenty of popcorn because I suspect I'm not going to be the last person to write in here.

Then again ...
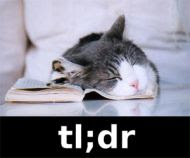


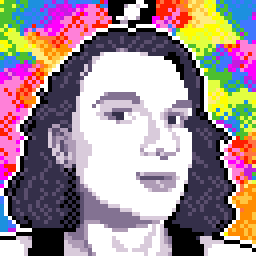
Let me be super clear, after reading this, the first words that come to mind are words I prefer to keep for myself.
First off, Joshmillard has been in the pico-8 community for longer than most of the other active users on here, me included. He knows the community super well as he saw it evolved. Myself I think I know the community well enough, having been here for more than a year now, to say that he speaks for a lot of people, and actually including people that don't write on here at all! Believe it or not, a huge part of our community is silent and/or extremely shy. And someone as loud as you annoys them.
Second, I don't know where you've heard that no problem exist until someone states it does but that's just so very wrong. There's lots of problems everywhere and most of them are unspoken and that doesn't make them less existant. It just let them keep on existing.
Keeping it short, musurca made a great point saying that BBS input should be clear, concise, straight to the point, no weird wise-ass quotes or sayings and infrequent. If you're here to learn about Pico-8, please boot up you Pico-8 and make stuff. Sure, come here writing about your stuff, suggesting things for other people's project, etc but only from time to time, while most of your time is actually spent on Pico-8. You learn a lot more about it that way, believe me.
Nobody's bullying you. Bullying and complaining are very different things. Your medical disorders are a sad thing but please do not use them as a shield. And by all means, STOP WITH THE PASSIVE AGGRESSIVENESS. Stop it. It's despicable. And please stop writing like you're above everyone else. Just get straight to your point, that's really all we ask. No intro, no development, just the conclusion. Efficiency, wah.
edit: Just saw your message on Pixel Session's thread, saying you bought the games. I know this was very bad timing but I hope you understand this has absolutely nothing to do with it and I sincerely hope you do have fun with your purchase.


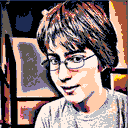
I just want to raise some points for everyone—and especially @dw817—to read:
Yes, dw817 has been posting a lot, perhaps to the point of annoyance of some who are used to, and (fairly) expect the pico-8 forums to generally be sparse and to the point.
At the same time, I think I can sympathize; not all talkativeness stems from attention-seeking, but sometimes from wanting to fit in, or being able to add something meaningful to a conversation, etc. And I have definitely had experience and problems with over-talking as well (being autistic and somewhat bad at sociability), both on some places online, and IRL.
And although I at first shouted out in joy at @joshmillard's post, not because I "hate dw817" or "want to see them go" (sorry to prove you wrong dw817 :), but along the lines of
"finally someone is letting them know that everyone would appreciate it if they toned their amount of posts some".
That is, a post about how one should act appropriately in the pico-8 forums.
But then as it went back and forth, and more people started weighing in, it morphed from constructive critique pointed towards dw817, into almost dog-piling on top of them. And I do not think dog-piling is the appropriate to let someone know they've made a mistake or caused strife.
Yes, some of the things dw817 have said have been rather passive-aggressive, and I think they could've been/can be rephrased more politely, and appropriately for the forums.
But they deserve some credit, and definitely do not deserve to be dog-piled—because I've seen some of the fantastic things they've made; and I've seen how they've tried to help newbies out with code; and I adamantly believe that we can all move forward past this and grow.
As people say; "peace" :)



To follow up, I'll say this:
dw817, I can't speak for any of your past experiences with bullying, but as far as this community is concerned, no-one is bullying you. The fact that you are suggesting that people have arbitrarily painted some sort of target on you and that criticizing your actions will "elevate" our standings in the community is completely fucking ridiculous.
Bullying implies actual abuse and intimidation. This is not what is going on here. People are, in varying degrees of politeness, informing you a) what they have come to expect from the community and b) how your behavior is clashing with those expectations to the extent where it affects people negatively. The only reason I decided to post in this thread and join in on the "dog-piling" (which I think is a stupid word thanks to its constant misuse, but I'll save that rant for later) was because you made it clear that you didn't trust josh in that he was speaking on behalf of the community.
As a person, I can only say that I am sorry and that I feel sympathy for your situation and difficulties. I really, honestly mean it. But as a series of anonymous strings of sentences on the internet, that context in form of an underlying human individual doesn't exist (until made clear), so people are instead going to use the second best thing and judge you by your actions and exactly what is said and done. And it's a matter of fact that what has been said and done by you has affected people negatively, as several members have already stated in this thread. It's only fair, for both you and for us, that we let you know.


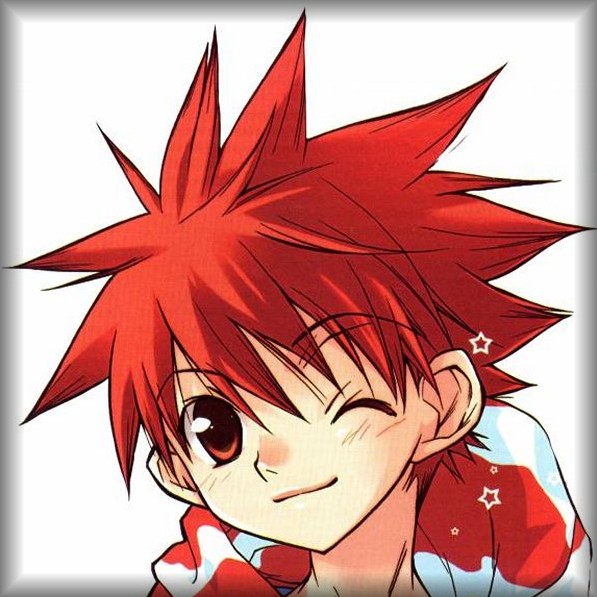
I've given myself off some days to think about all that has happened here. I do admit what I did was wrong - and it's not the first time.
I get excited, like a kid in a candy store, and start replying to everything in sight (and site) on websites that interest me.
And I can be rather obnoxious in that respect.
I apologize for my earlier words. I have received no complaints at all for my massive posts in Writer's Cafe - yet, I think they thoroughly encourage dominating writings in there is why.
I like what Jeremy said, not trying to get attention so much as trying to fit in. I never seem to and there's no point in trying it seems.
And yes, I do feel like I got "piled" on when everyone jumped in here to jump on me. The lack of being able to send any private messages I suspect is why.
I'm certain were private messages available in here, this whole thing would not be aired out as it is - and instead, one person would've written me, quite possible with the same serious tone.
And not feeling so threatened as it was a single private post, quieter and more productive words I suspect would've followed.
In any case, changes are going to take place. I promise I won't be a pest.
Today, I'm going to answer people who wrote me.
Tomorrow I will post the final of Haunted House. It should be a good and enjoyable game.
The day after ... until some of the good updates are made to PICO that ZEP is promising, I will vanish for a-while. I've done all I can in PICO for the moment until the system is updated and upgraded.
You also seem to be doing quite well without me and I don't want to break that peaceful cycle.
Thank you all who wrote me to bring my behavior to attention.
[Please log in to post a comment]