NOTE: This is a guide to my menu system that I have used in the example cartridge however contains mostly general tips that you can adapt to your own needs.
This is a simple guide for my Menu System that also shows off how to do simple 2-colour palette swapping. Anyone is free to build upon and implement this system into their own games they make.
If there are any questions or queries, feel free to leave a comment and I'll try to reply as soon as possible. Here are some guides suitable for beginners to help explain how to use it.
Guides
How To Add More Options:
How To Select Options:
How To Change Palettes:
Thanks for checking out my guide! I may end up making some more in the future but we'll see how this one turns out...
Happy Coding!
![]() |
[0x0] |


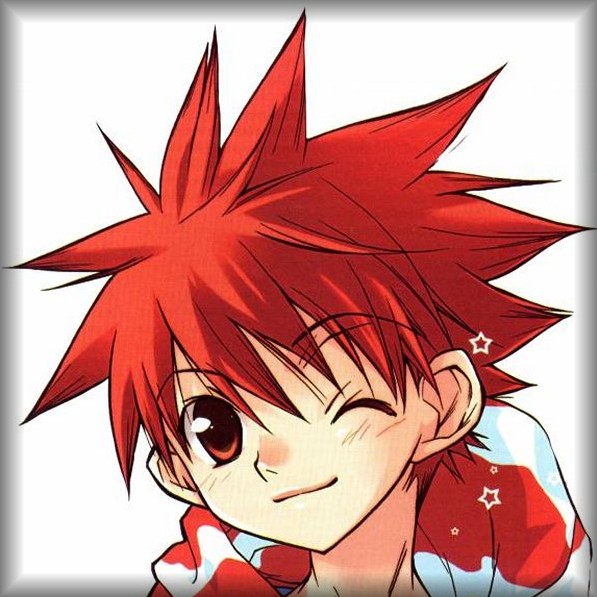
Regarding the menu options, PixelCod. Is there a way to make the menu appear inside your running program when you are using the main EXE interface and execution ?
I've tried [ESC]. The program stops.
I've tried [CR]. It is ignored.
?



@dw817 I'm not quite sure what you mean here...
Do you mean having the menu open while your main game is running? If you can provide a screenshot that would be great.



You filled in m.amt twice in the code. (init_menu and init_options) edit: I finally noticed why. but the below still applies
Also, if you want to save about 30 tokens, remove m.amt altogether and just refer to #m.options
Hi dw! If you want this menu to happen, you have to have the pals{} and palnum filled in and call init_menu() ahead of time, and then when you want a menu it needs update_menu() and draw_menu() both called each frame.
If you want a menu item to do something, it gets more involved, adding more sub-ifs under the btnp(4) in update_menu().


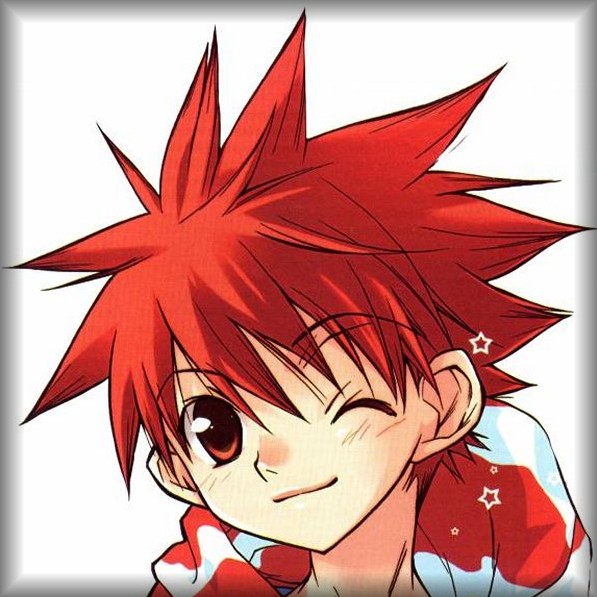
Sure thing. Here are three screens.
The code is called, "My Thing." It's pretty small and you can find it HERE:
Now booting up PICO from Windows, here are the screens.
Having loaded it, I am prepared to run it.
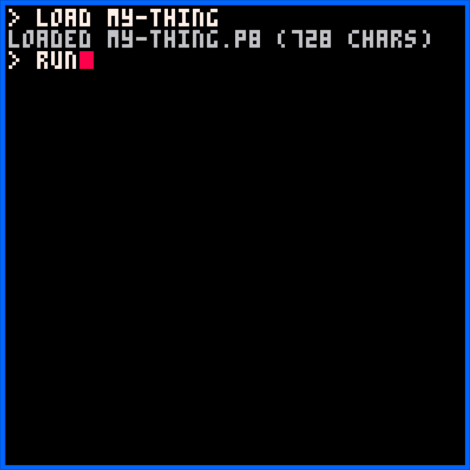
Here is what it looks like when it is running.
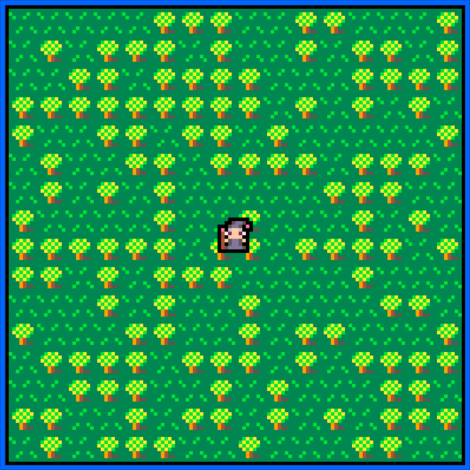
Okay, now I am pressing [ENTER]. There is no change.
Now I am pressing [ENTER] from the NUMBER KEYPAD. There is no change.
I press [ESC] and the program exits immediately.
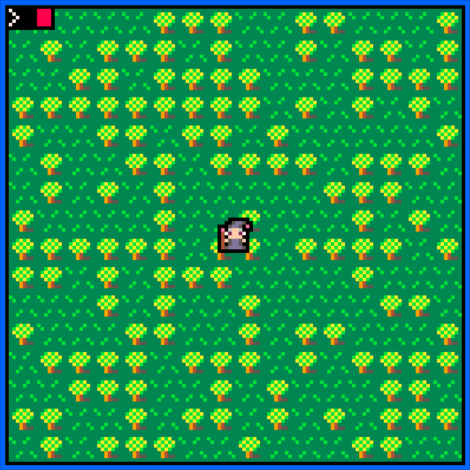
So how do I bring the menu up from the normal PICO editor ?
Tyroney, could you please post a complete and small working example source of this menu ability ?



Thanks for the reply @tyroney! I declared that variable twice as I originally had more options in the settings than the main menu and I forgot to remove it, oops. After checking the API I think i could use count() instead of # to count how many items there are but I'll try both.



@dw817 Elaborating on what tyroney said, you'll need to map a key (e.g. 'x' which is key 5) to make update_menu() and draw_menu() run each frame.
You could do this using a state machine that switches the players state from 'can walk around and play' to 'operating a menu'. In the playing state the arrow keys can make your player around and then when you're in the menu state the arrow keys can make the cursor move up and down.
I recommend reading pages 41 onwards in the Picozine 2 as it walks you through how to make a state machine.



here's some pseudo code to try:
FUNCTION _INIT() STATE=0 END FUNCTION _UPDATE() IF STATE==0 THEN UPDATE_GAME() ELSEIF STATE==1 THEN UPDATE_MENU() END END FUNCTION _DRAW() DRAW_GAME() IF STATE==1 THEN DRAW_MENU() END END |
What this should do is only update the game stuff (e.g. player) if STATE equals 0 and only update the menu if STATE equals 1 but always draws the game and only draws the menu if STATE equals 1.


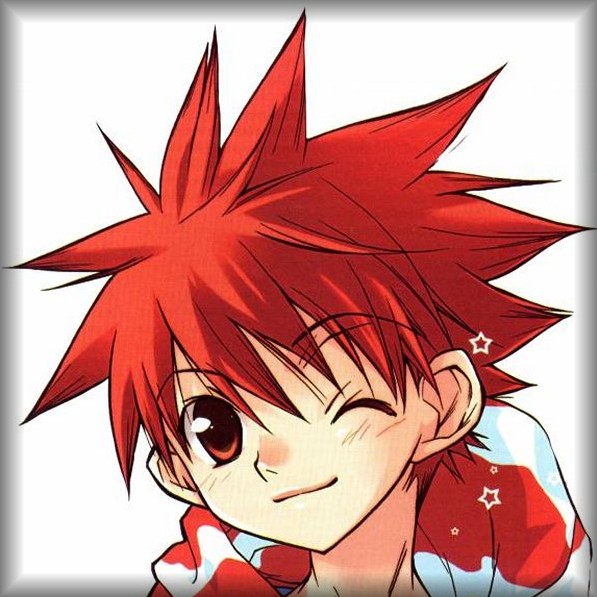
Hi PixelCod. I tried running your code and got an error message.
LINE 7: ATTEMPT TO CALL GLOBAL 'UPDATE_GAME' (A NIL VALUE)
Is your example a complete one ? Just a simple program that demonstrates how to invoke PICO's own menu system.



No, sorry. It was just pseudo code (mock-up code basically). I'll try to post a simple example tomorrow for you :) .



Here's an example cart I came up with that shows how to use the menu in a game to pause and resume. The bouncing ball is here just to show that the game actually pauses. I highly recommend that you download this and read through the code; I've left some comments in it to try and explain what's going on.
Controls:
-Arrow Keys to move
-'Z' Key to pause + interact with menu
NOTE: To be able to use the menu in your own games you will need to have copy and pasted all of the menu code into your program.


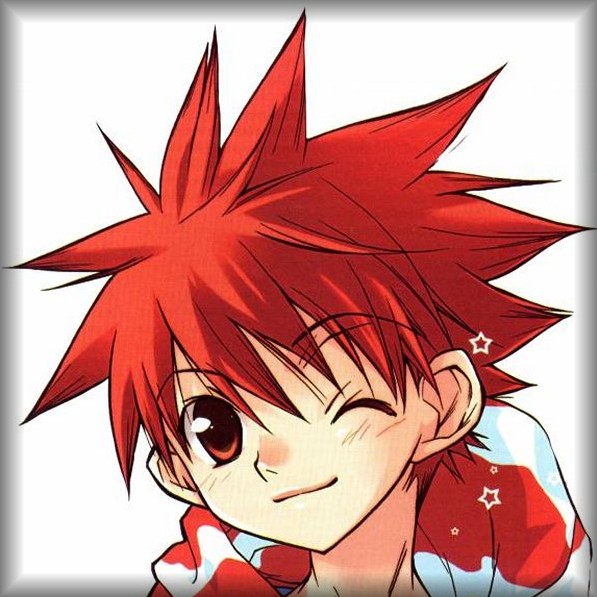
Hi PixelCod. This is great - ahh - not what I had in mind.
No, I wanted to know how to add a MENU item to the default PICO system.
You know, where you try a cart online and press ENTER. It comes up with a menu. And you have an option of adding to these menus.
I tried and couldn't get it to work.
I like your idea for a completely custom menu though. Really professional looking !



Taken from the PICO-8 0.1.8 Changelog
It is now also possible to add your own items to the pause menu to trigger things like 'RESTART PUZZLE' or 'EXIT TO OVERWORLD'. Here's an example program:
col=12 function _draw() cls() rectfill(0,20,127,107,col) end function changecol() col = (col+1) end menuitem(1, "change colour", changecol) |
The first parameter is the position (1-5) in the menu to insert the item, the second is the item's label, and the 3rd is a function to be called when the item is selected. I opted to keep this simple and quite rigid, so there's no way to have extra stuff going on in the background while the cart is paused, or to re-appropriate the pause button as an in-game button.
PixelCod: Is this what you were looking for?


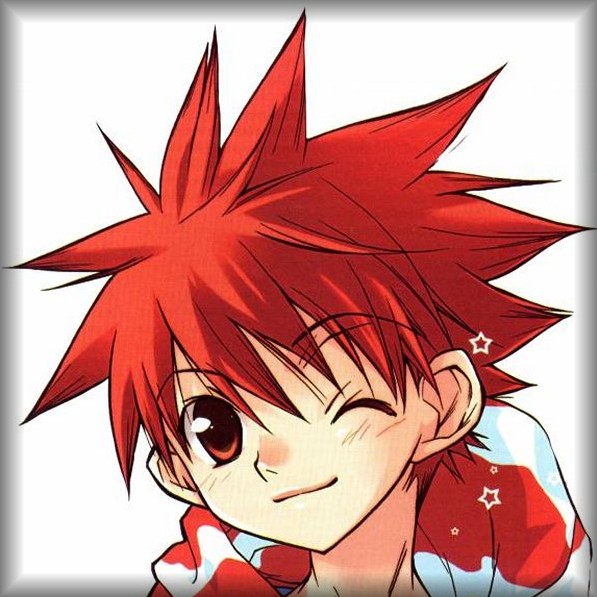
Maybe. If it works. Here is the code I am having problems with:
function main() menuitem(1,"test",doit) getkey("check menu now") end function doit() print"confirmed !" end -- simple get and wait key [a] function getkey(t) if t!=null then print"" print(t.." - press [a]") end repeat flip() until btn(4)==false repeat flip() until btn(4) end--getkey main() -- nifty put at top function _update() end |
What I am wanting is the menu to be active at any time, for instance, in this case, if it is inside a loop.
Thus when you press [ESC] from SPLORE, or [ENTER] from your browser, by selecting the TEST menu item, it will return the text:
"Confirmed !"
Tyroney was trying to help on this too. But so far the menu item only works when it's not in a loop.
Is there a way to make an interrupt so the menu will always work no matter where or what the code is running ?



The built in PICO-8 menu is always available during a program, is it not? Whenever you press 'ENTER' in any program it should pause what's happening on the screen and display the menu options until you press 'ENTER' again. You may be over complicating this for yourself but I'm not sure.
Try just setting all of the menu options in the _init() function at the start and see if it works.


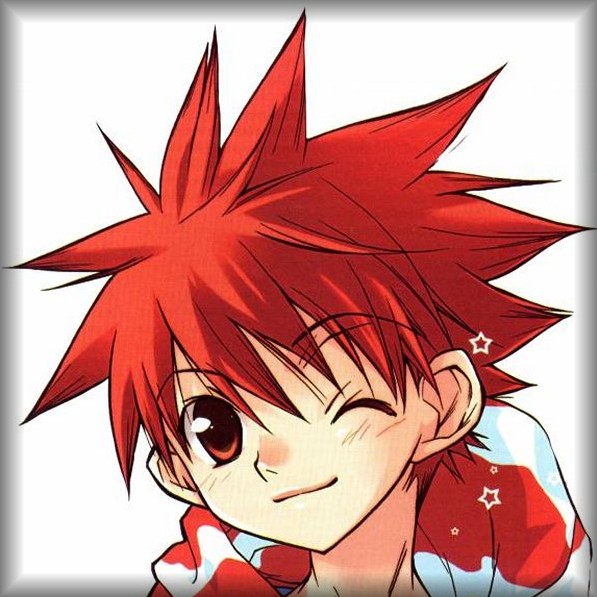
Okay, I added a new function and put it there.
function _init() menuitem(1,"test",doit) end |
When I run the code from SPLORE, the menu option doesn't appear at all now ...



Have you considered trying to use a menu system like the one I'm showing here? Feel free to play around with my code from the example cart.


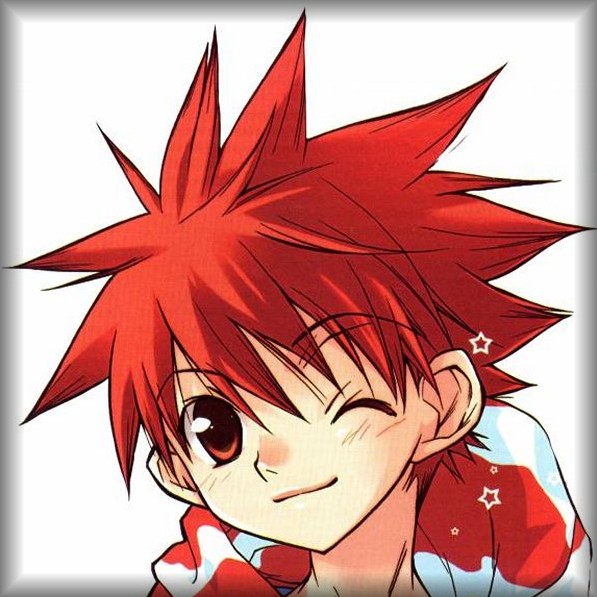
I'm good. I wanted a sliding ICON driven menu, and - that's exactly what I'm programming in my PICO Notepad right now.
Thanks, though. For anyone else (besides me cause I'm a little odd) I think your menu is going to be a big hit and a definite use.


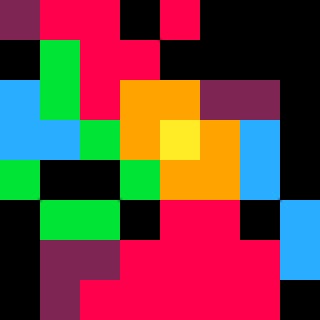
IIRC, "btn(7)" is the start button, in case that helps. But yeah. I look forward to playing with your thing sometime. lol



@TonyTheTGR oh okay, thanks!
@geistvonpa Thanks for the feedback! I think I will make some tutorials that cover more basic principles and game concepts next.


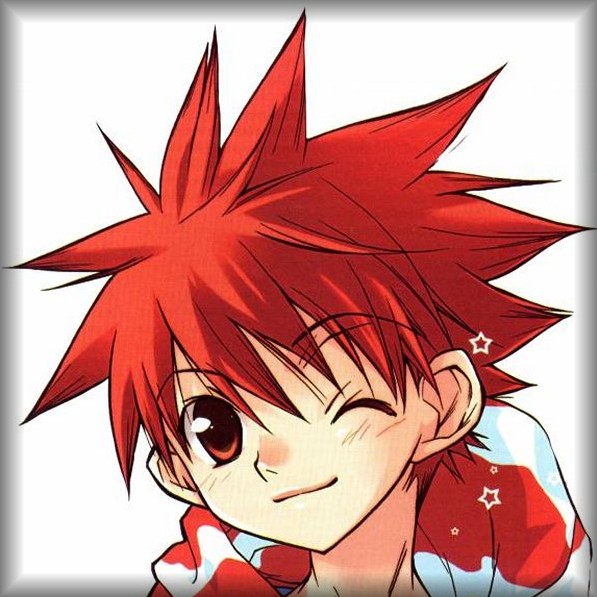
Hi PixelCOD. Any chance of your menu showing two frames ? That is, you click one item, it goes mute in the background, and another menu item appears slightly to the right and below ?
I like your highlight method. Shows me something I need to add to TRX - but later. So many other projects are on hand ! :D



@dw817 Ahh I see what you mean, like in an RPG game! That's an interesting idea, I'll look into it ;)


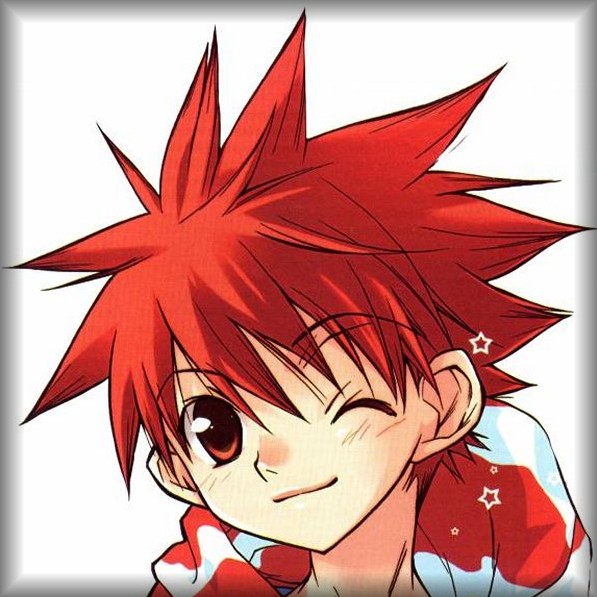
Absolutely, PC ! Once I finish this infernal keyboard thingie, I'll try to finish up Tilemaster. It definitely makes use of this menu approach and you'll be able to see it clearly in there. :)
[Please log in to post a comment]