Yep, one more shot, this time Winnie ther Pooh. I definitely like this method and will most certainly use it for any carts I share as a neat way of logo and instructions.
Okay, getting back to working on other projects.


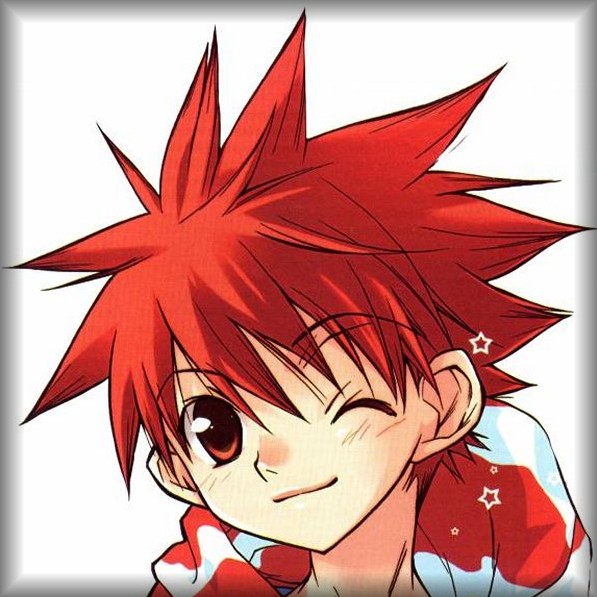
Thanks, Afburgess ! I'm already working on a neat cart that'll make use of this - and perhaps the start of a new game company - will see.
Oh, and it's really not this slow. I put a delay in there so you could see it. PICO is so fast, it just blinks and it's done.



I really like this technique. I think that actually these are 1-bit images (2-color), though, not 2-bit images (4-color). Anyway, I made a couple carts that expand on this.
The first one is an expanded demo, just for fun. It stores four images that you can cycle through (left/right) and change the color of (up/down). I took the extra images from some other carts that have been posted:
tetsuo from pomppo's 1-bit sprites
frog from enno's PNG converter demo
I made a couple changes to the algorithm, which are mostly a matter of personal preference. It does flip the order that it reads blocks of pixels, so your original data won't render correctly (I converted your images for this cart).
- Instead of using >= to check the value of c and then subtracting that amount, you can use a binary AND to check whether a particular bit is set. That way you don't have to modify c at all and you don't need to care which direction your l is going.
if band(c,2^l)>0 then print("bit "..l.." is set") end |
Although, incidentally, you can loop in reverse by adding a step parameter of -1 to your loop:
for l=7,0,-1 do print(l) end |
-
I combined the i/j loops to a single loop through the entire block of data. The pixels are consecutive data, so you can just go through them sequentially instead of keeping track of the x and y coordinates. Of course, then you have to calculate the x/y coordinates when you draw to the screen, but this is my preference.
- I wrapped the whole thing in a function that takes two parameters: the memory address to start reading from and the color to draw with. Here's the final result:
function draw(mstart,col) cls() mstart = mstart or 0x0 col = col or 7 for i=0,0x0800 do c=peek(mstart+i) for l=0,7 do if band(c,2^l)>0 then pset((i%16)*8+l,i/16,col) end end end end |
The second cart I made is an image compactor. (You will need to download this one and run it locally to use the compacted image of course.) It reads the entire contents of the sprite sheet, compacts it down to be read by this algorithm, then saves it back to the sprite sheet (overwriting the original!) and stores the data back to the cart file. Then you can open the file in your text editor, copy the gfx memory section and paste it into your real cart. Again, this follows my slightly adjusted algorithm, so the output won't display exactly right with your original draw algorithm.
Note that this compactor treats all non-black colors as white.


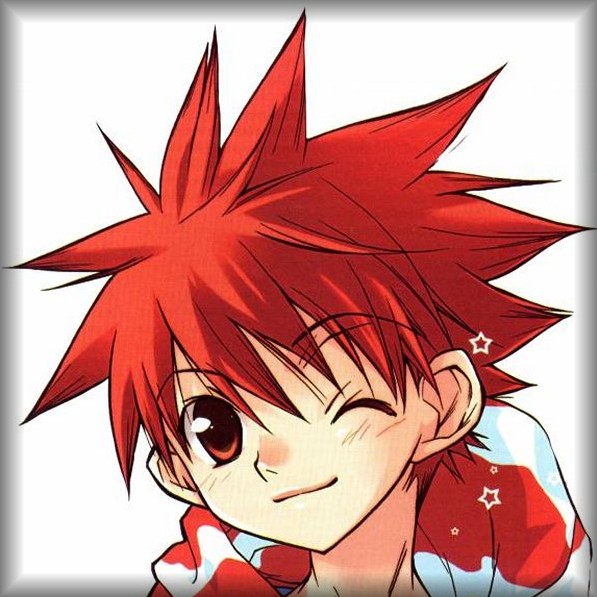
You're right, LRP, it's not 2-bit but 1-bit. Yeah well, the memory is first to go, right ? :) 2-color I meant.
Looking at your mods, yep, I figured someone would find an easier way to implement it. It's like me driving a Ford Pinto all my life and then someone has a Lamborghini.
I'm so old school there's a good chance I had a hand in building the school that was so old. Grin
I noticed that PICO didn't have any bitwise comparison functions like BTSET or BTCLR so I used the ^2. I'll have to look up that BAND command.
I see a lot of incredible 3D games and toys in here too. That is just something I cannot wrap my head around - but maybe I can still bring some of my coding ideas and images and music in PICO and they will still be of use and entertainment.
Your method to compress is a bit busy - I'll go to post the EXE of the compressor I wrote - provided I can settle down on a quick and fast method of storage.
You got me thinking ... Looking at your code, that it should be possible to make this all in one loop. Will experiment.
Thanks for your interest !


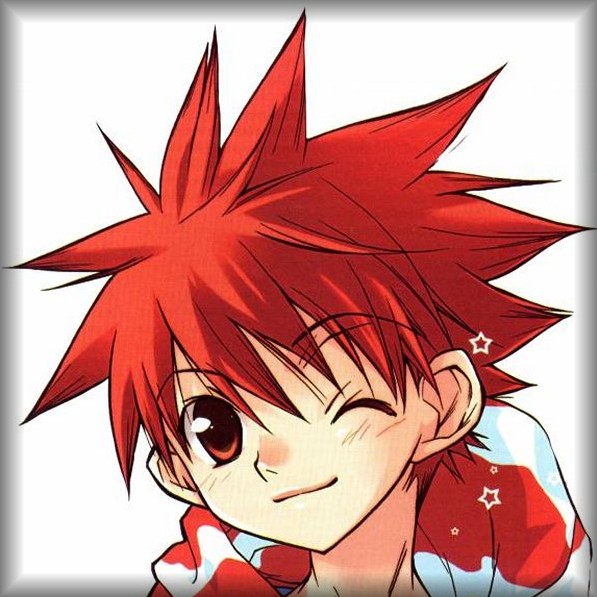
Try this. It's smaller and a bit more sleek, LRP:
r=0 -- necessary to define for i=0,16383 do if band(peek(i/8+6144),2^(i%8))>0 then r+=7+105*(i%2) end poke(24576+i/2,r) if i%2>0 then r=0 end -- ^ rem this line for psychedelic monkey ! end |
Interesting that BAND function, thanks for showing me. I don't have anything like that in BlitzMAX.
Oh, and here is the compressor I wrote in Blitz:
https://www.dropbox.com/s/esds7hwurh0tpc5/Compress%20128x128%20to%20Pico%201-bit%20Image.zip?dl=0
I'm curious about this "write sprite sheet to cart."
What does this do and - is it a way of storing more than 256-bytes of data that can be recalled as well as for Online play ?



You can mess with the map, or sprites, or music during runtime without messing up the game. (When you reboot, everything is reset.) The demo cart jelpi does this with game entities so that pickups and enemy deaths persist offscreen.
If you want to, running cstore() can overwrite everything, or save them to a new file. (which is exactly what you want to do for a development tool like this)


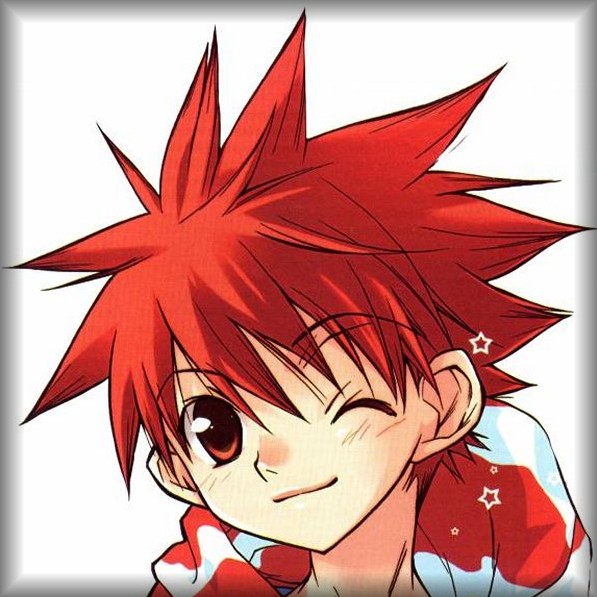
So CSTORE just saves binary data, Tyroney. Could you save and retrieve, oh, I don't know, let's say 100 or more individual files for one game, just for instance - and still be able to post it here Online ?
(( I'm smelling a RPG Maker here and I absolutely live for those )) :D
http://creatools.gameclassification.com/EN/creatools/481-Scenario-RPGMaker/index.html



Good point about the modified loop, dw817. I still prefer pset() to poke() for writing the pixels to screen, though. It saves several tokens because the math is a lot simpler. Here's my revised function.
function draw(mstart,col) cls() mstart = mstart or 0x0 col = col or 7 for i=0,0x3fff do if band(peek(mstart+i/8),2^(i%8))>0 then pset(i%128,i/128,col) end end end |
I also updated the demo cart so that you can switch between the raw data and the extracted image by pressing o/x. (Up/down now switches images instead of left/right, which is more intuitive when in raw mode.)
tyroney: Thanks for pointing out that cstore() can write to a different file. I had forgotten that. It makes a lot more sense than overwriting the source in this case. I'll have to update that cart, too, when I get a chance.


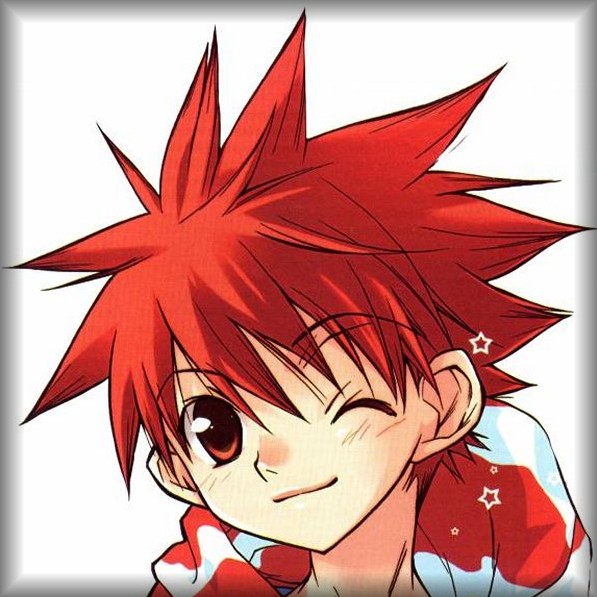
Tyroney, LRP:
Is it possible to import a separate .PNG file (say the one that is the 128x128 pixel B&W, 24-bits per pixel)
And then inside PICO, compress and then SAVE it to to a NEW PNG file ? (One that is actually 2-bit data ?)
As for the smaller code above, LRP. I was just curious to see if I could write an extractor in one loop.



Not simply binary data - cstore() saves .p8 files. (and .p8.png ? The only files you can deal with are carts.) So I believe any data would have to be converted and stored in sprite or map space or such.


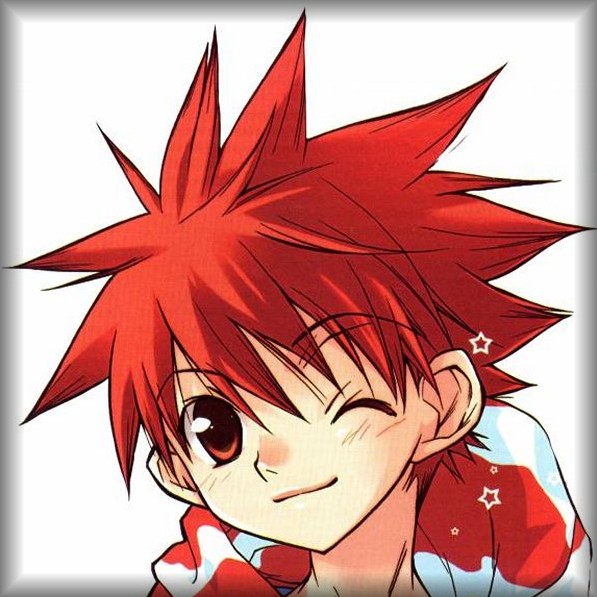
I think that answers it. Thanks, Tyroney. Heh - I've got so many pet projects I'm working on ATM. BlitzMAX, B4GL, and Pico.
And right now for all 3-of 'em, I'm in the tricky part of the coding.
[Please log in to post a comment]