Hi all!
I would like to create a counter that goes from 0 seconds to 1 and vice versa, forever.
I know that I can use time() and indeed I can successfully count from 0 to 1 but then.
How can I decrease and increase again?
I cannot picture a solution...
Thanks!


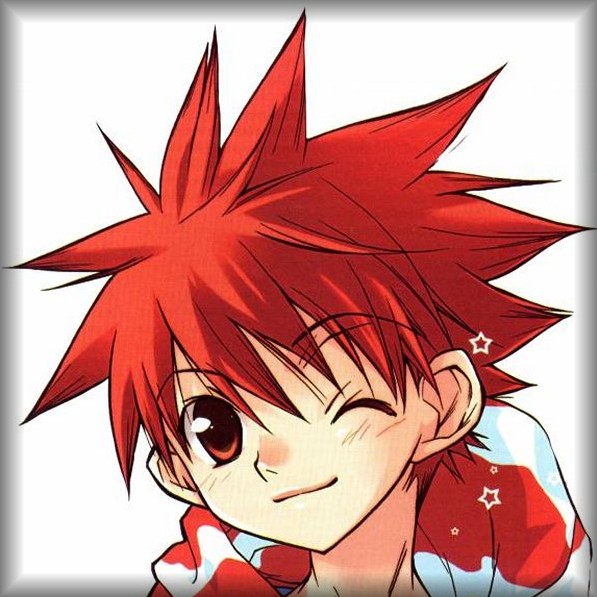



Try this: flr(t()%2)
t()
returns the number of seconds since the start of the program, as a fraction of sections. %
is the modulo operator, the remainder of a division. By modding the value with 2, you get all of the values up to 2, then it restarts to 0 (because 2/2 = 1 with remainder 0, 2.2/2 = 1 with remainder 0.2, etc.).
flr()
removes the fractional portion, if you just want this to flip between integers 0 and 1. Omit flr()
if you need those fractional portions. Note that the fractional portion will always go up. If you want the fractional portion to bounce between 0 and 1, you'll need to process it further. I assume for now you just want the integers.


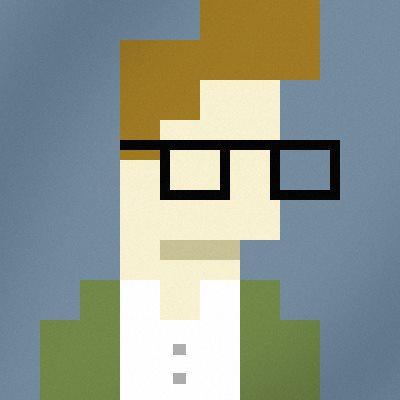
Thanks dan, this is mind blowing!
I want to make a super easy basketball game for 3 points shoots.
Basically the timer should move from 0.0 and go to 1.0 and back.



Here's what that looks like as a single expression:
(t()-t()\1)*(1-(t()%2\1<<1))+(t()%2\1)
It would be better, of course, not to use t() directly as that means the code calling a function every time and also no way to slow down the timer so that it's useful. Instead, assigning t() to a variable and dividing it would be better.
To explain the expression, it's using the method dddaaannn showed (but with /1
instead of flr()
since they're equivalent as far as I know) to instead switch between the fractional portion of t() and 1 minus the fractional portion of t(). Said fractional portion is extracted with t() - t()\1
. The way to switch between something being positive and being negative based on either a value of 0 or 1 is to do 1 - 2 * value
.



I think this does what you're looking for?
function _init() timer=0 dir=1 end function tick() local new_t = t()%2 timer = dir*new_t if timer > 1 then timer = 1 dir = -1 elseif timer <= -1.95 then timer = 0 dir = 1 elseif timer < 0 then timer += 2 end end function _update() tick() end function _draw() cls() print(timer) end |
The dir variable keeps track of which way the counter is counting (1 for forwards, -1 for backwards) and then the tick function calculates the time based on the system time and dir and swaps the direction when necessary.
If you don't need it tied to system time then it's even easier:
function _init() counter = 0 step = 0.01 dir = 1 end function tick() counter += dir * step if counter > 1 then counter = 1 dir = -1 elseif counter < 0 then counter = 0 dir = 1 end end function _update() tick() end function _draw() cls() print(counter) end |
You can set the step size to whatever you want to speed up or slow down the counter and just swap direction any time you go outside your bounds.


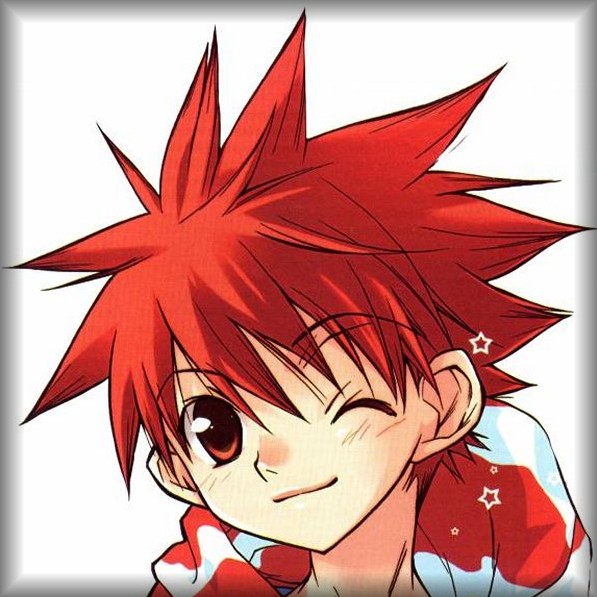
[Please log in to post a comment]