by jesstelford

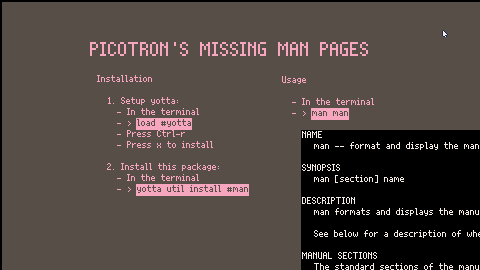
Documentation at your fingertips!
This cart installs the man
terminal utility for reading documentation within picotron itself.
Something not documented? man
will intelligently search the Fandom Wiki! 😱
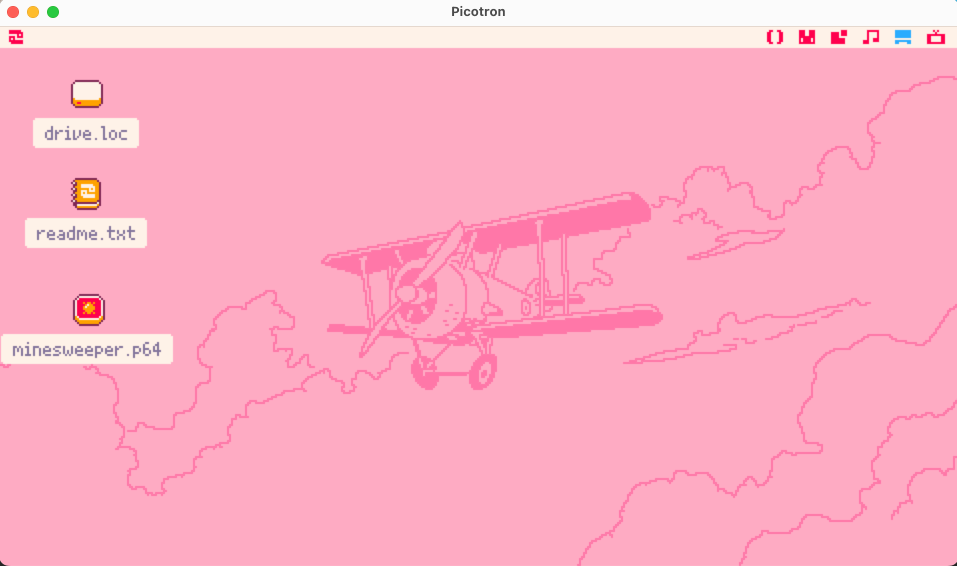
Installation
-
Setup yotta:
- In the terminal
load #yotta
- Press Ctrl-r
- Press x to install
- Install this package:
- In the terminal
yotta util install #man
This will install the following files for you:
appdata └── system ├── lib │ └── man.lua # The `man()` function for library usage [ [size=16][color=#ffaabb] [ Continue Reading.. ] [/color][/size] ](/bbs/?pid=143718#p) |
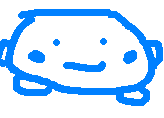
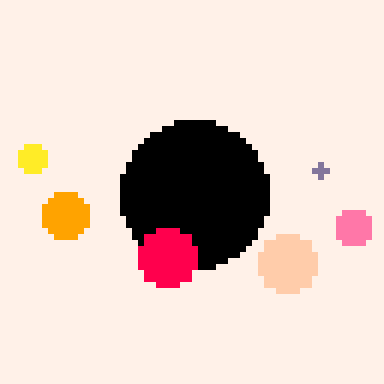
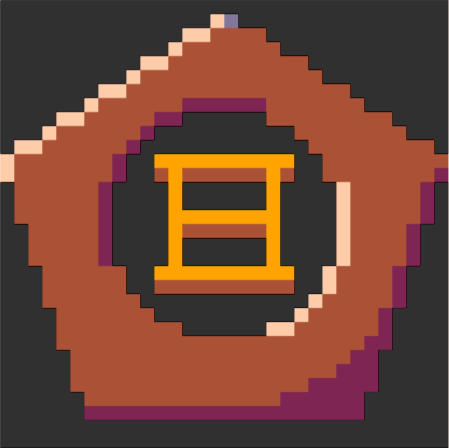

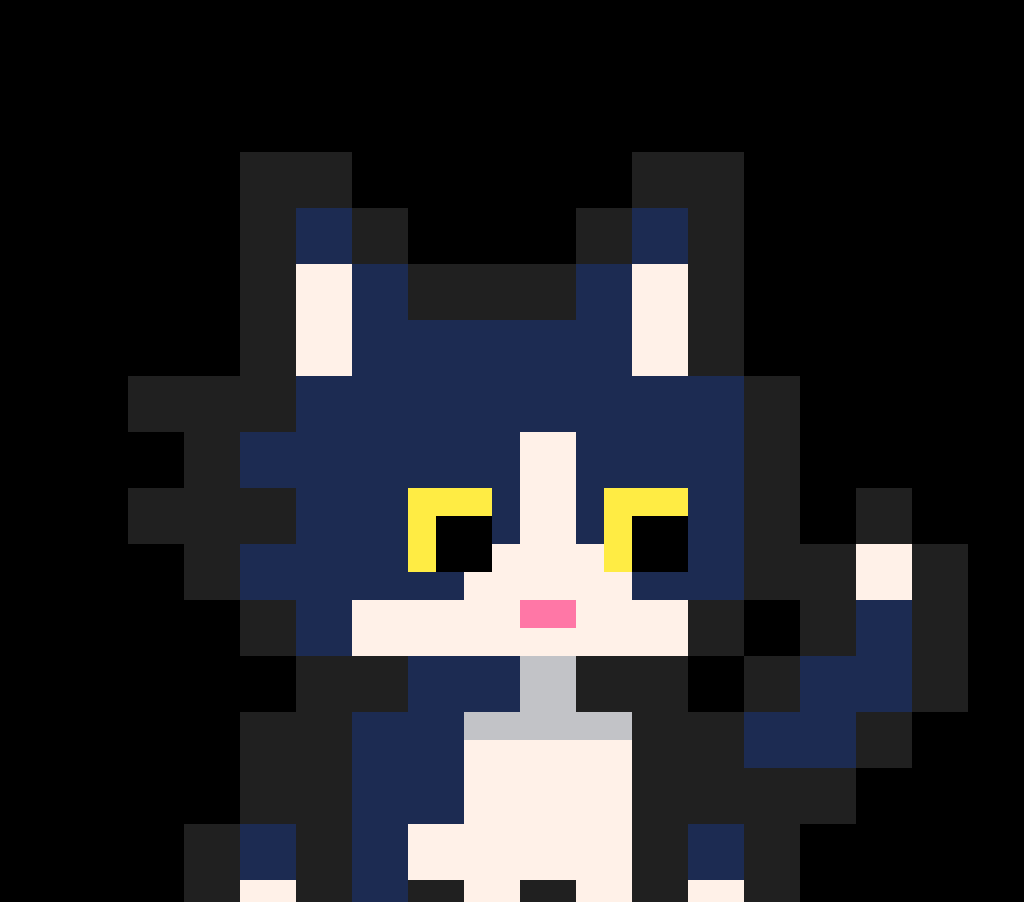
Introducing PECS (PICO-8 Entity Component System)
✅ Small API
🏃♀️ Efficient even with lots of Entities
😀 Fun to say
🎈 Has a "lite" version for the token-conscious
Based on the fantastic Tiny ECS Framework by @KatrinaKitten 🙏
Full code & docs on GitHub: github.com/jesstelford/pecs
Here's a demo cart showing off some Particle Emitters using PECS v2.0.0:
Update 20210316: I have added a Camera Follow/Window example also:

Pseudo motion blur using the extended palette + dithering with fillp
.
The crux of making this happen is:
local defaultFills = { 0b0000000000000000, -- solid 0b0000000000000001.1, -- single pixel missing 0b0000010100000101.1, -- 4 pixels missing 0b0101101001011010.1, -- half pixels missing 0b1111101011111010.1, -- 4 pixels rendered 0b1111111111111110.1, -- 1 pixel rendered } -- Derived from https://stackoverflow.com/a/10086034/473961 function resizeAndFill(input, outputLength) assert(outputLength >= 2, "behaviour not defined for n<2") local step = (#input-1)/(outputLength-1) local result = {} for x=1,outputLength do result [ [size=16][color=#ffaabb] [ Continue Reading.. ] [/color][/size] ](/bbs/?pid=80976#p) |