I'm working on my first PICO-8 game which is also the first time I really got into programming. Now I almost hit the token count but I don't really know how to shorten the code. I also admit I got really careless when writing the code and was mostly just happy whenever it worked. In at least three instances I'm 100% certain there needs to be a much more efficient way to code, any experienced programmer will probably cringe so bear with me.
But I'd really appreciate any help!
The first code is a function that adds text to the screen with each sentence delayed.
oprint2() is just a function I copied from Sophie Houldens talkthrough of Curse of Greed which adds an outline to the text.
Right now there are six texts but I want this function to work with an even greater number of texts and to able to make changes without so much hussle!
The text content and the if-statements to trigger them are always different.
The text style/colors are always the same. Right now I have individual timer variables are for each text which I realize is horrible. If it helps, I'd have no problem with all texts having the same speed.
function intro() camera() controls() if ending_init==0 then if help==1 then if game_start_t <= 320 then oprint2(" move\n\n ⬅️ ⬆️ ⬇️ ➡️",8,24,0,15) end if game_start_t >= 180 and game_start_t <= 460 then oprint2("\n\n search for hints\n\n ❎",8,44,0,15) end if game_start_t >= 370 and game_start_t <= 530 then oprint2("\n\nsearch through our belongings\n\n ❎",8,64,0,15) end if game_start_t >= 500 and game_start_t <= 700 then oprint2("\n\n surrender\n\n 🅾️",8,84,0,15) end if game_start_t <= 710 then game_start_t += 1 end end if p.keys >= 1 and dget(2)!=1 then if key_timer <= 240 then oprint2(" a key",8,24,0,15) end if key_timer >= 50 and key_timer <= 270 then oprint2("\n\n opens either a chest\n\n or a door",8,34,0,15) end if key_timer >= 120 and key_timer <= 300 then oprint2(" and might only fit once.",8,82,0,15) end if key_timer <= 310 then key_timer+=1 else dset(2,1) end end if steponfv >= 1 and dget(3)!=1 then if stepon_timer <= 240 then oprint2("every small decision",8,24,0,15) end if stepon_timer >= 50 and stepon_timer <= 270 then oprint2("\n\n will\n\n influence",8,34,0,15) end if stepon_timer >= 120 and stepon_timer <= 300 then oprint2(" our fate.",8,82,0,15) end if stepon_timer <= 310 then stepon_timer+=1 else dset(3,1) end end if musx == 7 and musy == 3 then if restart_timer >= 350 and restart_timer <= 600 then oprint2("the heart of the desert",8,24,0,15) end if restart_timer >= 700 and restart_timer <= 1000 then oprint2("\n\nwhere everything ends\n\n and everything begins ...",8,34,0,15) end if restart_timer >= 1100 and restart_timer <= 1400 then oprint2("we came so far this time",8,54,0,15) end if restart_timer >= 1500 and restart_timer <= 1800 then oprint2("are you ready\n\n to unlearn everything\n\n again ...?",8,74,0,15) end restart_timer+=1 if restart_timer >= 1980 then poke(rnd(23800),rnd(0x100)) end end if musx == 1 and musy == 0 and hiddendoorv == 0 and greedv == 0 and steponv == 0 and steponfv == 0 and textreadv==0 and ending_i==0 then if east_timer >= 50 and east_timer <= 250 then oprint2("this is not the empty room ...",8,24,0,15) end if east_timer >= 200 and east_timer <= 300 then oprint2("we shall head east\n\n and ascend!",8,44,0,15) end if east_timer <= 310 then east_timer+=1 end end if musx == 4 and musy == 0 and hiddendoorv != 0 and textreadv !=0 then if empty_timer >= 30 and empty_timer <= 120 then oprint2("my mind is restless",8,24,0,15) end if empty_timer >= 140 and empty_timer <= 220 then oprint2("the noise is unbearing",8,44,0,15) end if empty_timer <= 230 then empty_timer+=1 end end end end |
The second code teleports the player from certain map tiles to others when stepped on.
Sometimes it works two ways but then it changes to one tile in either x or y to prevent a loop.
My problem is again that the if-statements are always different so I don't know how to group them together and sometimes there are multiple if-statements or they require different variables than p.x and p.y.
function desert_teleport() if ending_init== 0 then if greedv >= greed_one then if p.x == 1 and p.y == 1 then p.x = 49 p.y = 1 end elseif hiddendoorv >= hiddendoor_one then if p.x == 4 and p.y == 6 then p.x = 88 p.y = 54 end elseif textreadv >= textread_one then if p.x == 79 and p.y == 60 then p.x = 121 p.y = 25 end end if (p.x >= 112 and p.x <= 127 and p.y == 47) or (p.y >= 16 and p.y <= 47 and p.x == 127) then p.x = flr(rnd(14)) + 97 p.y = flr(rnd(14)) + 34 elseif (p.y >= 16 and p.y <= 32 and p.x == 112) or (p.x >= 96 and p.x <= 111 and p.y == 32) then p.x = flr(rnd(14)) + 113 p.y = flr(rnd(14)) + 34 elseif (p.y >= 32 and p.y <= 63 and p.x == 96) or (p.x >= 96 and p.x <= 111 and p.y == 63) then p.x = flr(rnd(14)) + 113 p.y = flr(rnd(14)) + 17 elseif (p.y >= 2 and p.y <= 13 and p.x == 31) then p.x = flr(rnd(12)) + 65 p.y = flr(rnd(12)) + 1 elseif p.x == 79 and p.y == 60 then p.x = 17 p.y = 37 elseif p.x == 16 and p.y == 37 then p.x = 78 p.y = 60 elseif p.x == 35 and p.y == 15 then p.x = 89 p.y = 49 elseif p.x == 89 and p.y == 48 then p.x = 35 p.y = 14 elseif p.x == 40 and p.y == 0 then p.x = 99 p.y = 30 elseif p.x == 99 and p.y == 31 then p.x = 40 p.y = 1 elseif p.x == 6 and p.y == 63 then p.x = 107 p.y = 17 elseif p.x == 107 and p.y == 16 then p.x = 6 p.y = 62 elseif p.x == 92 and p.y == 31 then p.x = 19 p.y = 30 elseif p.x == 19 and p.y == 31 then p.x = 92 p.y = 32 elseif p.x == 0 and p.y == 10 then p.x = 110 p.y = 22 elseif p.x == 111 and p.y == 22 then p.x = 1 p.y = 10 elseif p.x == 16 and p.y == 28 then p.x = 110 p.y = 25 elseif p.x == 111 and p.y == 25 then p.x = 17 p.y = 28 elseif p.x == 56 and p.y == 0 then p.x = 40 p.y = 42 elseif p.x == 79 and p.y == 41 then p.x = 1 p.y = 38 elseif p.x == 0 and p.y == 38 then p.x = 78 p.y = 41 elseif p.x == 47 and p.y == 17 then p.x = 1 p.y = 56 elseif p.x == 0 and p.y == 56 then p.x = 46 p.y = 17 elseif p.x == 41 and p.y == 31 then p.x = 39 p.y = 49 elseif p.x == 39 and p.y == 31 then p.x = 88 p.y = 1 elseif p.x == 38 and p.y == 48 then p.x = 40 p.y = 30 elseif p.x == 40 and p.y == 48 then p.x = 69 p.y = 46 elseif p.x == 89 and p.y == 63 then p.x = 118 p.y = 57 elseif (p.x >= 16 and p.x <= 31 and p.y == 0) or (p.x >= 97 and p.x <= 110 and p.y == 0) then p.x = 88 p.y = 10 end end if ending_init==1 then if p.x == 0 or p.x == 127 or p.y == 0 or p.y >= 63 then p.x = flr(rnd(125)) + 2 p.y = flr(rnd(61)) + 2 end end end |
The last function switches out certain maptiles with others if some conditions are met. I have a lot of different tables and functions of this kind but for brevity I'll just post two.
Compared to the first two I think they are less awful but I still feel they could be optimized.
trap_table ={109,109,109,79,79,79,79,79,49,50,24,24,25,25,40,40,41,41,96,96,112,112,75,76,91,115,117,117,100,101} function place_traps() for a=0,127 do for b=0,63 do if mget(a, b) == 109 then mset(a, b, trap_table [ [size=16][color=#ffaabb] [ Continue Reading.. ] [/color][/size] ](/bbs/?pid=78580#p) |



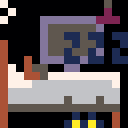