I'm looking for a simple function to rotate a sprite (or just one sprite tile) by 90 degrees to reduce sprite-sheet usage. We can already easily flip a sprite, so surely a 90-degree rotation isn't that far off, given we can already do it with the R key in the sprite editor.
I've spent a lot of time searching and seen lots of examples of free rotation to any angle you want. They all look very nice, but they use a lot of code and CPU time. All I want is the ability to rotate by 90 degrees, which I imagine would be a lot simpler and take a lot less CPU time and code, since there's no angle calculations or trigonometry, just a simple rows/columns operation.
Before I try to get my head around it, I was wondering if anyone else already has an elegant solution?



This should work for any sprite size
-- mode 0: clockwise 90 -- mode 1: clockwise 270 -- mode 2: mirror + clockwise 90 -- mode 3: mirror + clockwise 270 -- dx,dy: screen position -- w,h: sprite width and height (1 if not specified) function rotate(sprite,mode,dx,dy,w,h) local sx=sprite%16*8 local sy=flr(sprite/16)*8 w,h=w or 1,h or 1 w,h=w*8-1,h*8-1 local ya,yb,xa,xb=0,1,0,1 if mode==0 then ya,yb=h,-1 elseif mode==1 then xa,xb=w,-1 elseif mode==2 then ya,yb,xa,xb=h,-1,w,-1 end for y=0,h do for x=0,w do pset((y-ya)*yb+dx,(x-xa)*xb+dy,sget(x+sx,y+sy)) end end end |





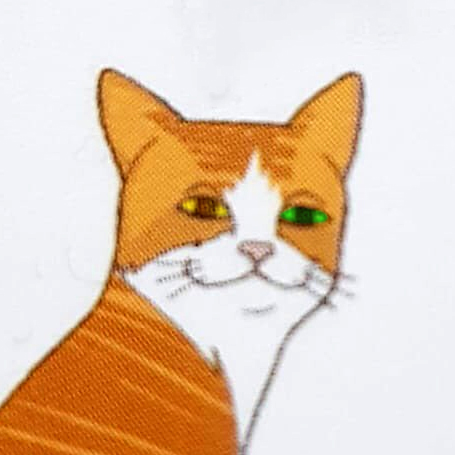
@freds72 tline()! I forgot tline() was a thing now XD
While I'm already using the entire map space, it's a map tile that I want to rotate anyway, so I could rotate the map tile in place using tline() as you said! Thanks :D



For anyone who might be stuck with the tline() method I wrote a function that can rotate the sprite 90 or 270 degrees
function sprot(dx,dy,h,w,mx,my,rot) --dx the x position of the sprite --dy the y position of the sprite --h the height of the sprite from the map --w the width of the sprite from the map --rot = 1 rotate 90 degrees anticlockwise --rot = -1 rotate 90 degrees clockwise rot = rot or 1 if rot!=1 then dx+=8 end for i=0,w-1 do local nx=dx+(i*rot) tline(nx,dy,nx,dy+h,mx,my+i/8) end end |
[Please log in to post a comment]