Juicy Animation Engine
Hey guys, I'm posting a small animation engine that I'm using for puzzle games and such. It's only about 500 tokens even in its non-minified state, and can be less.
At its core, it is a system that allows an object to self-modify its properties (such as its screen coordinates) over time, subject to an easing function. This allows the creation of really nice looking animations with a minimum of code.
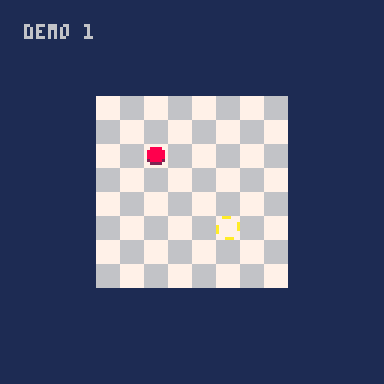
Features
- Dead simple to use, 'fire and forget' method.
- Controls for how many times to repeat the animation, reversing after it's done, etc.
- Choose and select only the easing functions you need to save code
- Can be used for cycling through sprite frames (linear interpolation of sprite numbers!)
- Control for object depth (which sprites are drawn over others)
- Object code can be modified and extended easily depending on the game
Setup
All you need are these three things:
o = {} -- list that holds all objects to animate, put in _init() update_objects() -- put in update draw_objects() -- put in draw |
To create an object, just call the object() function, which creates an object and appends it to the global object
list. Delete the object when not needed.
obj = object(spr, x, y, [z]) -- spr (number) sprite number for the object -- x, y (number) current screen pixel coordinates of sprite -- z (number) sprite "depth"; lower numbered objects drawn first. If z not provided, it's assigned to 1+ the highest z depth in the object list |
To set an animation for the object, simply call this:
anim(obj, p, to, d, [f], [rep], [rev]) -- obj (obj) an object, as defined above -- p (string) parameter to change ("x", "y", or "spr"; can be extended if needed) -- to (number) the object's parameter will be changed to this value... -- d (number) ... over d frames ... -- f (function) ... controlled by this interpolation function, default linear -- rep (number, -1 or >=1) the animation will be repeated rep times, default 1. If -1, animation will loop endlessly -- rev (1 or 0) whether the animation will reverse, default 0 |
So for example, if you have an object called 'piece' at (36, 36), and use the following two functions:
anim(piece, "x", 72, 15, out_quad) anim(piece, "y", 72, 15, in_back) |
This means:
- change the x coordinate of piece to 72, over 15 frames, using an out_quad function (faster at the start, slows at the end)
- same for the y coordinate, but use an in_back function ("pulls" a bit backward like a rubber band, before shooting to the target)
That makes this cute little hop. The animation wasn't set to repeat, so it deletes and garbage-collects itself after it's done.
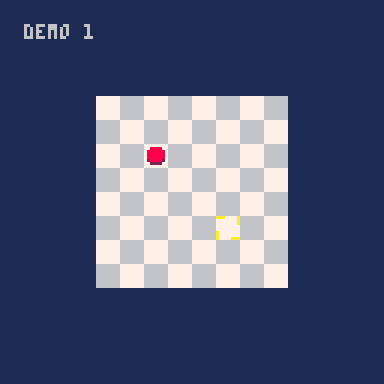
The cursor above is also animated with the following code:
cursor = object(2, 72, 72) -- create an object called cursor with sprite index 2 at (72,72) anim(cursor, "spr", 9, 30, linear, -1) -- change the sprite index of cursor to 8 over 30 seconds (2, 3, 4... 8), linearly, and repeat forever" |
Stuff to do / limitations
- This is mostly used for puzzle games, so I didn't build a way to use sspr with it. Very easy to modify though
- Playing the reverse of the easing function just uses the same thing. The animations should switch - an out_quad should be replaced with an in_quad, for example.
- Currently uses _update60().
Please feel free to message for any concerns or questions!


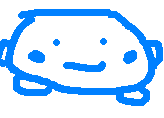
This is really great! (and it wouldn’t be hard to extend it to support width/height params for spr)


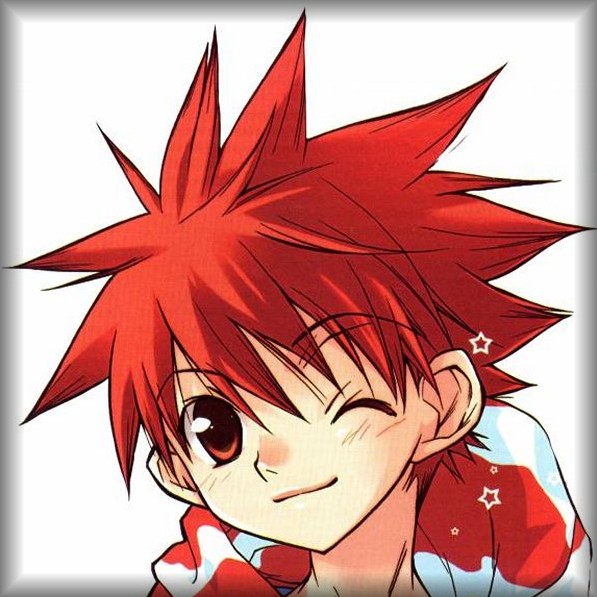
Interesting, @efofecks. Vertical movement though slides and does not jump in the air as does horizontal movements. You can confirm this by not seeing the shadow.
[Please log in to post a comment]