Hi,
I've made two games in pico-8 until now and because pico-8 is meant to be both a fantasy game system and a development environnement, I wanted to make sure that my code could be read inside the pico-8 editor.
The problem I came across is that lua is highly verbose. That means that writing a simple if else statement takes almost all of the screen width. Since pico-8 already include some shorthands such as += or -=, I though we could have some more to reduce the number of characters used in code. This could be useful for future twitter jam eventually.
so I've read a bit of the lua doc to know which keywords and which tokens are already used:
--used keywords and break do else elseif end false for function goto if in local nil not or repeat return then true until while --used tokens + - * / % ^ # & ~ | << >> // == ~= <= >= < > = ( ) { } [ ] :: ; : , . .. ... --token added by pico-8 += -= *= /= %= != ? |
Since they are already used, we cannot use them for an other purpose or else the lua interpreter might be confused.
So then I've made a list of tokens that do not exists neither in pico-8 or regular lua:
--not used tokens ! $ \ @ +> -> *> /> %> ^> #> &> ~> |> => <> ~~ && || :> .> |
And from that list I've picked token that could replace current keywords:
--example of shorthands ! : not @ : local \ : end $ : self => : function -> : then <> : elseif *> : return |> : break 0? : false 1? : true |
So here an example of code to see the result:
(the lines represent the width of the pico-8 screen)
--current pico-8 code -------------------------------- function xor(a,b) if a and not b then return true elseif b and not a then return true else return false end end -------------------------------- function l_v_add(l1,l2) for i=0,#l1 do if l1[i] == nil then break end l1[i]:add(l2[i]) end return l1,l2 end -------------------------------- function vec:add(v) if v != nil then self.x += v.x self.y += v.y end return self end -------------------------------- --code with additional shorthands -------------------------------- => xor(a,b) if a and !b -> *> 1? <> b and !a -> *> 1? else *> 0? \ \ -------------------------------- => l_v_add(l1,l2) for i=0,#l1 do if l1[i] == nil -> |> \ l1[i]:add(l2[i]) \ *> l1,l2 \ -------------------------------- => vec:add(v) if v != nil -> $.x += v.x $.y += v.y \ *> $ \ -------------------------------- |
One thing to note is that the second code is more cryptic.
So I've made some screenshot to see how it would look like in the editor
and here it seems easier to read actually.
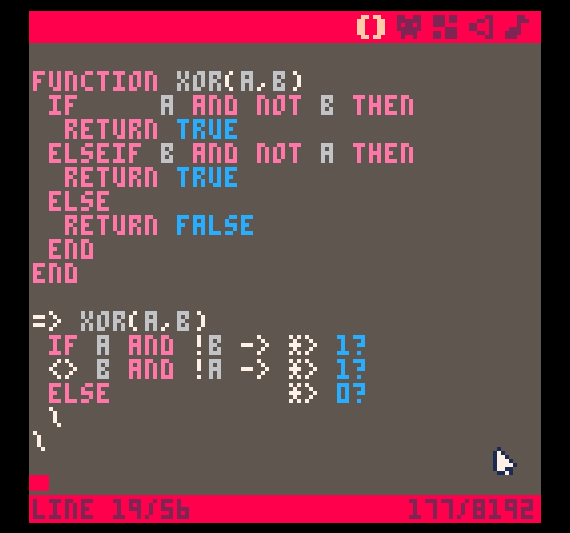
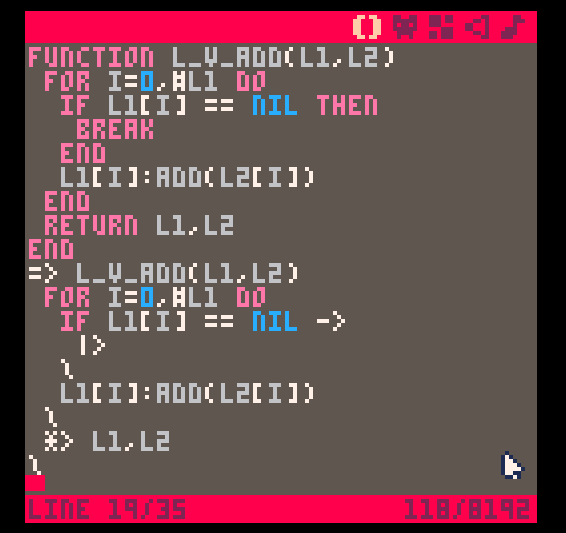
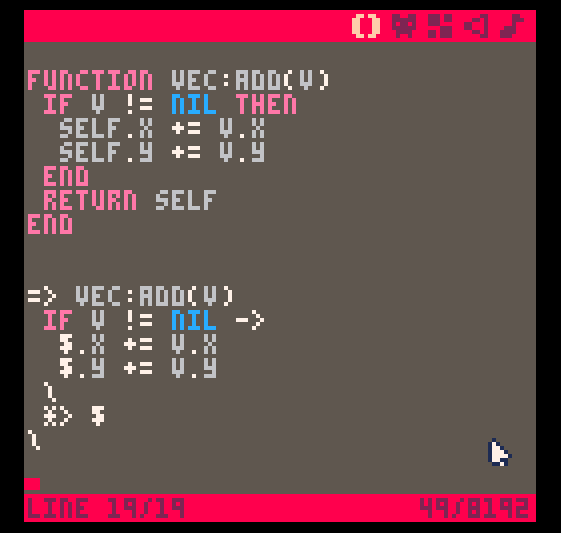
To avoid too cryptic code, maybe it would be better to define shorthand keywords instead.
(instead of "then" or -> why not "do", instead of "elseif" or <> why not "ef")
Also we could have alternative way to write "and" and "or" like in other programming language: && and ||
--alternative code -------------------------------- => xor(a,b) if a && !b do *> 1? ef b && !a do *> 1? else *> 0? \ \ -------------------------------- => l_v_add(l1,l2) for i=0,#l1 do if l1[i] == nil do |> \ l1[i]:add(l2[i]) \ *> l1,l2 \ -------------------------------- => vec:add(v) if v != nil do $.x += v.x $.y += v.y \ *> $ \ -------------------------------- |
So what do you think about that ?
Is it feasible ?
Is the code too cryptic to be useful ?
Is this whole thread pointless ?


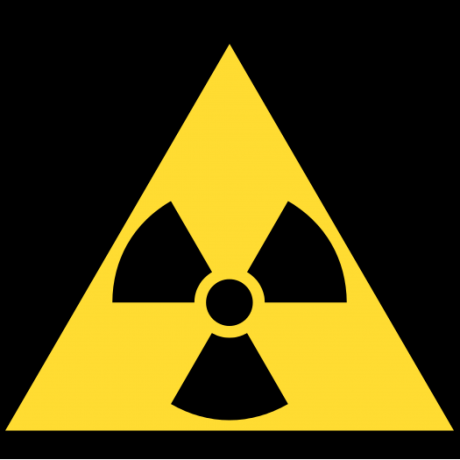
While the more verbose code is harder to fit on screen, keeping "standard" Lua makes the PICO developer environment much more approachable to beginner programmers, who may or may not have coded before, as well as making it easier to look up help and read documentation, because their code will look much more like examples.



My point is not to completely remove the standard lua syntax from pico-8 but to extend the superset which is the pico-8 language.
If I want to code with regular lua keywords I should still be able to do so otherwise the pico-8 language wouldn't be based on lua anymore.
Adding more shorthands doesn't mean we get rid of the standard Lua.
It's the same thing with typescript and javascript for example:
you can code in regular javascript in a typescript environnement because typescript is only a superset of javascript.


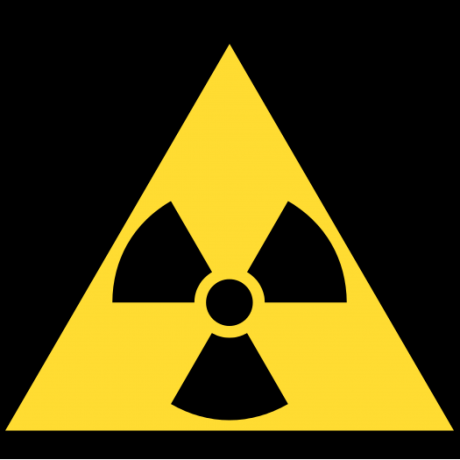
Indeed. However, one of the selling points for the PICO is that you can take other peoples games and open them up to find out how they work.
The use of shorthands would impede beginners learning from your code.



Well when I learnt how to code, the hardest part wasn't to understand what was the effect of a shorthand but rather what was the purpose of the overall function. And the easiest way to understand how a function work is to be able to see it completely.
For example, I wanted to know how some people make 3D renders in pico-8
But I was quite disappointed when I saw that all of the instructions took more than one screen in length. So I have to copy the whole code from the pico-8 editor to Visual code to be able to read it. What's the point of having a text editor in pico-8 in the first place then ? :/
I don't think adding more shorthands for some keywords would be that detrimental neither for pro developers nor beginners.



I don't think the problem comes from the verbosity of lua. I usually tend to write code with long function and variable names, and adding shorthands would be of little help in that matter.
I think the main issue is that it's only possible to navigate horizontally with the text cursor, which is really cumbersome. Adding a shortcut like ctrl+wheel to scroll horizontally would help solve that problem.



But a shortcut to scroll horizontally doesn't change the fact that most of the time, the screen is full of pink keywords and you can't have the full function on the screen at once. An option to reduce the size of the text would be even more useful.
I personally prefer variable names with only one character, to keep code within the screen. I've managed to do that until now but the result is that the code is hard to read because it is really compact and full of keywords.



Another modest style change is to exchange width for height using more newlines, for example:
function funcname( arg1, arg2) if arg1 < arg2 then ... elseif arg2 == nil then ... end end |
Not sure if this is my favorite option and I'd have to try it with some real code to judge an improvement.
Adding new syntax to a language needs a pretty high bar and I don't think this justifies it, but I appreciate the hypothetical. I agree that the narrow screen width interferes with studying real-world code, as well as with editing to a lesser extent. My personal preference would be to improve this experience out-of-band, such as by adding a code browser to the BBS cart view. But I appreciate suggestions to make the in-band editor a better experience.
picotool could be the basis of a third-party cart code browser, and its formatter could be extended to normalize formatting, convert .p8.png to .p8 automatically for text editors, or add newlines to improve in-band browsing.



Yes I had to spread some if else and function call statement on multiple line to keep my code in the 32 characters' limit. The problem with that is that I loose vertical space in order to save horizontal space.
Also I think it is a shame to rely on third party tools to be able to simply code efficiently in pico-8.
However I agree that there should be a good reason to extend the syntax of a language and that screen size is not really a good reason to do so. On the other hand it seems to me that lua because of its verbosity wasn't the best choice for a dev environnement with so much restrictions.
Maybe I put too much shorthands in my first post and people though that I wanted all of them to be included in the pico-8 syntax. If I had to keep only a few of them it would be those:
! : not do : then ef : elseif @ : local \ : end |
in that order.
We should be able to call "!a" instead of "not a" especially when you want to convert a value into boolean,
You would get "!!a" rather than "not not a"
Also why do we have to use "then" rather than "do" for "if" statements, "for" and "while" loop use "do" already and it's a reserved keyword, so it wouldn't cause any ambiguity.
I still think that "elseif" needs a shorthand, "ef" is the same length as "if" so comparaison chains would look better (with the use of the "do" keywords)
A shorthand for "local" would be nice, it would allow to have the variable declaration and its affectation on one line but I agree it's not that important since we can declare and affect multiple variables at once by using commas.
And a shorthand for "end" would reduce the amount of pink characters on the screen, it is not really important I agree.
So yeah overall, ! for "not" and "do" as a synonyme for "then" should be part of the pico-8 syntax.
If we also add the "ef" keyword, then my first function could look like that:
_f=false _t=true -------------------------------- function xor(a,b) if a and !b do return _t ef b and !a do return _t else return _f end end -------------------------------- |
Now that I wrote this, I realize that shorthands for true and false are useless since I can create variables to store those values


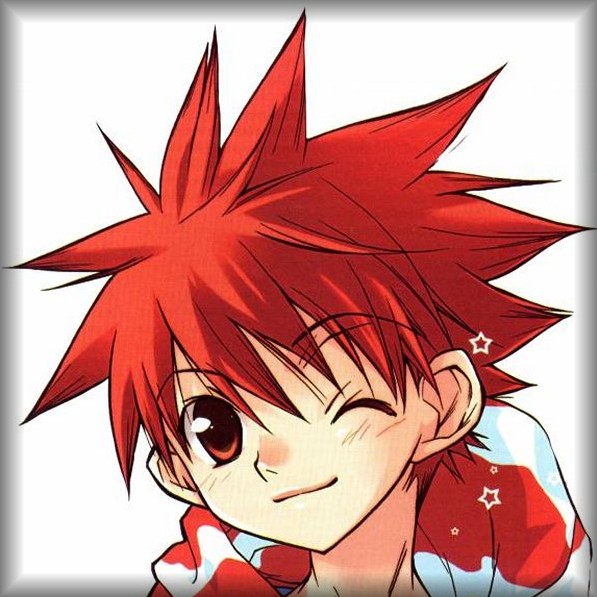
This would indeed be useful for TWEETS, @harraps, which must count the number of characters in their code.
You get my star for your thoroughness.
[Please log in to post a comment]