I'm having a problem scrolling a repeating tile. Here's how I setup my tiles:
for i=1,500 do add(bgtiles,{ alive=false, x=0, y=0, vel=0.52 }) end local y=-8 for i=1,17 do bg_spawn(0,y) y+=8 end function bg_spawn(x,y) for _,t in pairs(bgtiles) do if not t.alive then t.alive=true t.x=x t.y=y return end end end |
And here's how I update:
for _,t in pairs(bgtiles) do if t.alive then t.y+=t.vel if(t.y>128) t.y=-8 end end |
But during the scroll I get this:

Here's the tile:

It doesn't matter if vel is an int or a float, I still get the misalignment. I'm guessing this is a common noob mistake! Can anyone help?


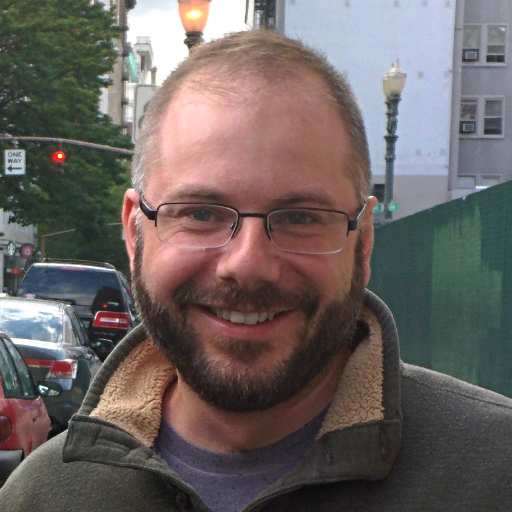
If you make vel=1, but then change the conditional at the end to t.y>127 instead of t.y>128, the weird offset stops. The last pixel on the screen is 127, not 128. (Pixel number 127 is the 128th pixel, because of the 0th pixel.)


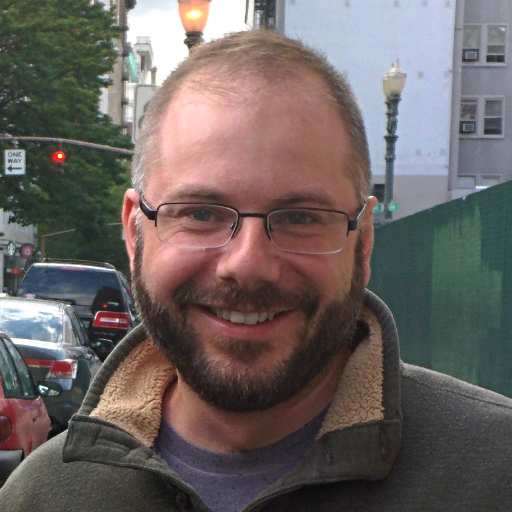
Lastly, if you're trying to loop a number, it's much cleaner to use the modulus operator to do that. In your case, you would use
t.y=((t.y+t.vel+136)%136)-8 |
instead of
t.y+=t.vel if(t.y>128) t.y=-8 |
This will ensure it loops correctly back to -8 once it gets off the screen.


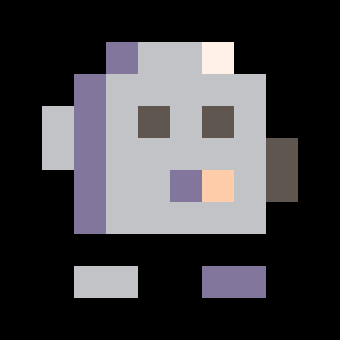
Thanks, those are the fixes I was looking for. I also noticed that you need to use the modulus method if vel isn't an int, otherwise you still end up with misalignment. Why is that?


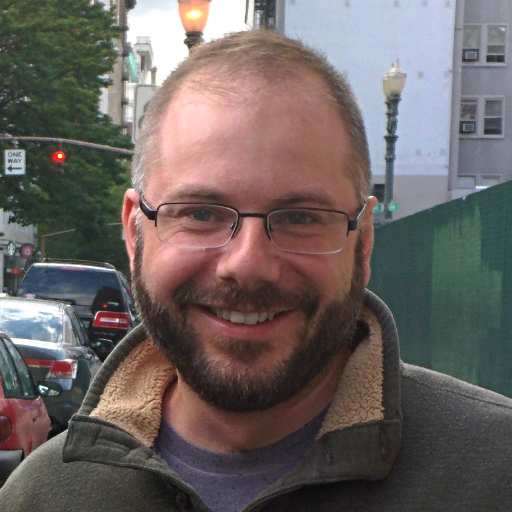
Because modulus loops the number, rather than just resetting it back to a starting number.
For example, if your tile gets to 132, and you use modulus, it loops back to 4, since it's 4 units past 128, so it should loop back to 4. Whereas if you just reset it, if it's at 132, you're just hard-setting it to 0.
Think of modulus like a looped piece of string. If something was traveling around the string and went past the loop point, you wouldn't want to pull it back to the loop point.
Hope that makes sense.
[Please log in to post a comment]