What up fishes!
So, I'm new to Pico (mostly a Unity/C# person) and I've been getting my bearings this last week. I was looking into ways to get more game content out of fewer sprites. The most obvious way seemed to be using the same sprites but changing the colour palette. I did some searching and found a few posts where this came up but the only answer I found was to use pal() which unless I'm missing something, changes the screen palette, which affects every instance of that colour onscreen.
So, below is the solution I landed on. Is there a better way to do this? If so, pls halp.
(I've changed some variable names for clarity, I don't normally label things like this)
--Define a 2D array of colour indexes. C1 is the colour we're swapping, C2 is what we're --swapping it to. SecondarySpritePalette={ {c1=14,c2=11}, {c1=2,c2=3}, {c1=10,c2=1}, {c1=4,c2=5}, {c1=15,c2=4} } --Draw sprite on screen using custom sprite drawing function that takes in the above array. DrawSprite(SpriteID, XPos, YPos, PaletteArray) function DrawSprite(n, x, y, c) --Get position of sprite within the spritesheet. local ycell=(flr(n/16)) local xcell=(n-(16*ycell)) --Loop through each pixel in the sprite. for lx=0,7 do for ly=0,7 do --Get the current pixel colour. col=sget((xcell*8)+lx,(ycell*8)+ly) --Loop through each colour in the palette array for i in pairs(c) do --If the current pixel matches C1, swap it for C2 and break --out of this loop. if col==c[i].c1 then col=c[i].c2 break end end --Only draw the pixel to screen if it is not 0 (or whatever colour we --want to be transparent. if not(col==0)then pset(x+lx,y+ly,col) end end end end |
The above code works (see below) but feels a bit... messy.
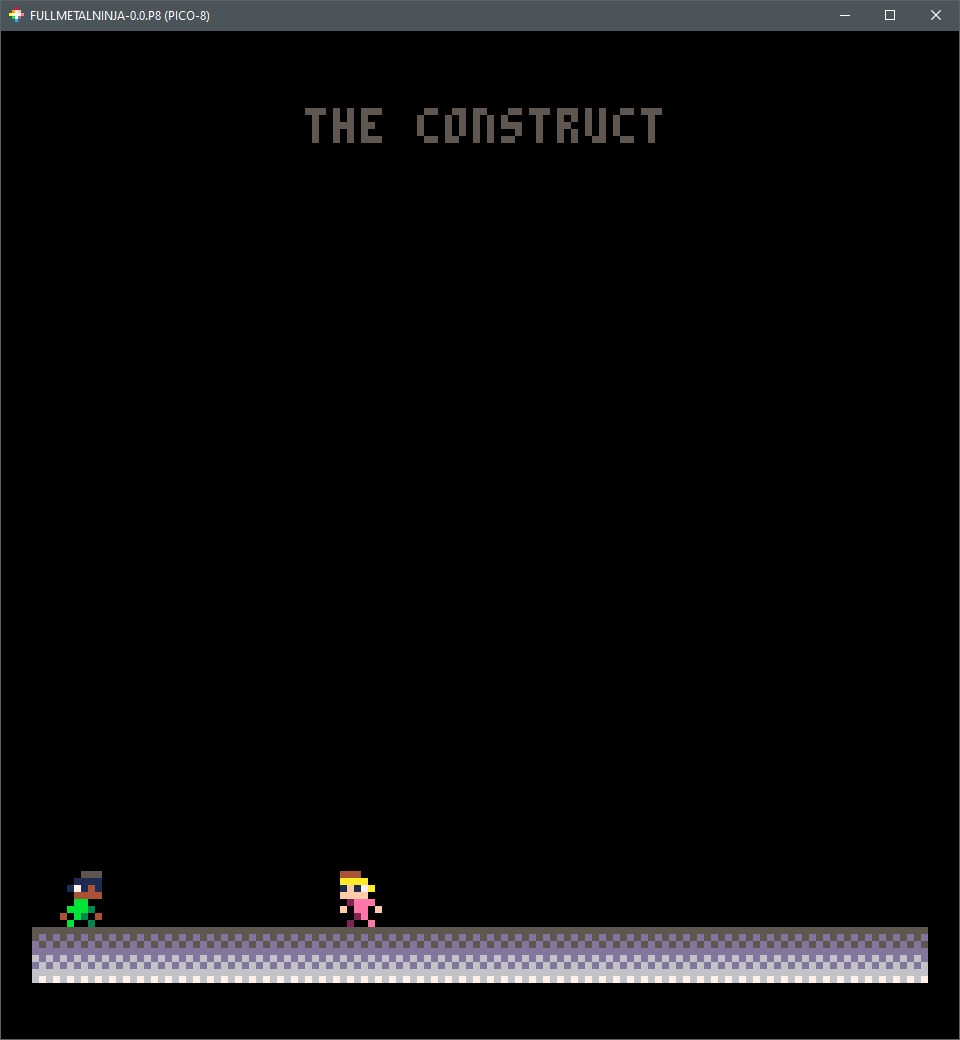


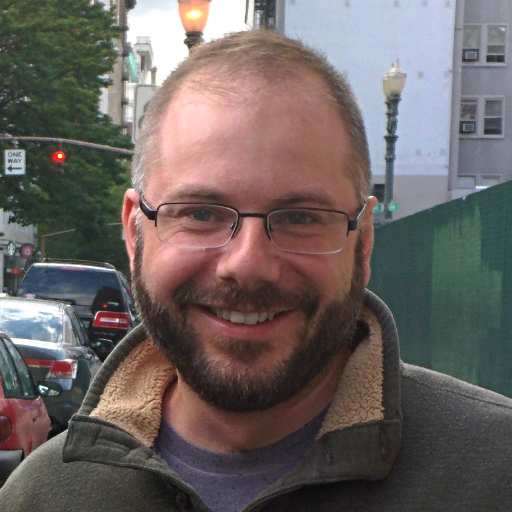
You can use the pal() function to swap colors just fine. I think the thing you were probably missing was using pal() (with no arguments) after you use it to reset colors back to normal before you drew the next thing. Also, if you don't do pal() (no args) before the next _draw() happens, it will continue to use the altered palette. See this cart:


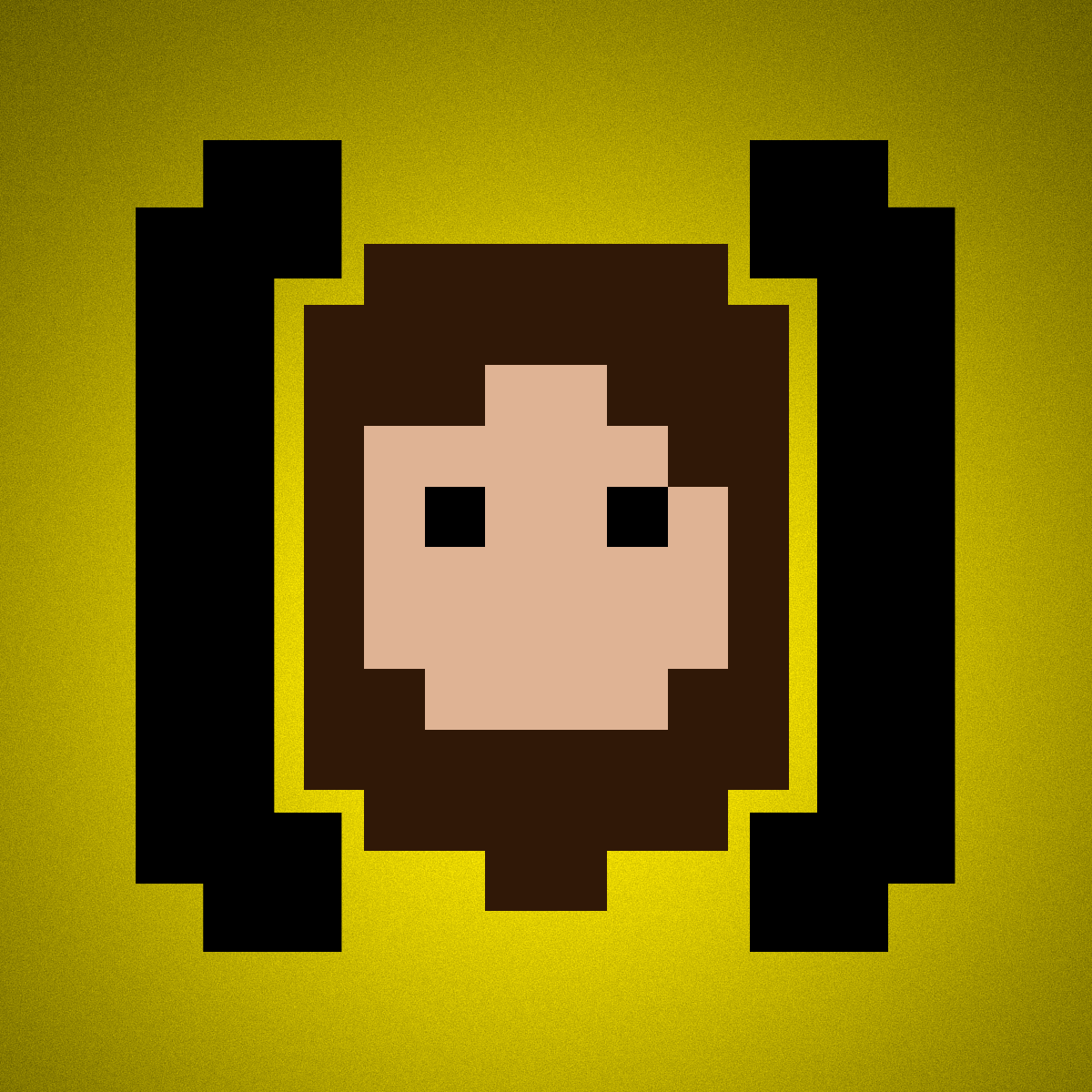
Thank you!
You're right, about the only thing I didn't try was calling pal with no arguments. This makes everything a lot neater. You're a star.
[Please log in to post a comment]