I have been experimenting with player movement for a top-down 2D game. At the moment, I have "fixed" diagonal movement to be 0.7x the speed of straight-line movement, and also added friction so the character sprite slows down gradually.
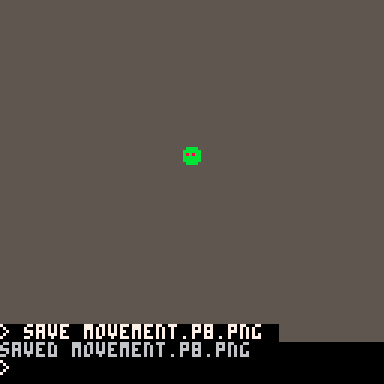
The next step however is acceleration.
I'm wondering how a slow ramp into movement (acceleration) might be achieved using the lerping algorithm as introduced here: https://demoman.net/?a=animation-code-part-1
Does anyone have tips or a tutorial on how I could achieve this acceleration/deceleration??
Code for movement:
if (btn(➡️) and btn(⬆️)) then p.dx=p.speed*.707 p.dy=0-(p.speed*.707) elseif (btn(➡️) and btn(⬇️)) then p.dx=p.speed*.707 p.dy=p.speed*.707 elseif (btn(⬅️) and btn(⬇️)) then p.dx=0-(p.speed*.707) p.dy=p.speed*.707 elseif (btn(⬅️) and btn(⬆️)) then p.dx=0-(p.speed*.707) p.dy=0-(p.speed*.707) elseif (btn(⬆️)) then p.dy=0-p.speed elseif (btn(➡️)) then p.dx=p.speed elseif (btn(⬇️)) then p.dy=p.speed elseif (btn(⬅️)) then p.dx=0-p.speed end if not btnp(⬆️) and not btnp(⬇️) then p.dy*=friction if p.dy<0.01 and p.dy>-0.01 then p.dy=0 end end if not btnp(➡️) and not btnp(⬅️) then p.dx*=friction if p.dx<0.01 and p.dx>-0.01 then p.dx=0 end end p.x+=p.dx p.y+=p.dy p.x=mid(0,p.x,124) p.y=mid(0,p.y,124) |


Hello,
you were actually most of the way there, all you need to change in your current code to have acceleration is to add or subtract a value to the speed instead of reassigning it each frame. I wrote up some example code that accomplishes that while streamlining things, and also resets the speed to zero if you hit a screen edge. You'll probably need to tweak some values like friction, as I don't know the values you're using at present.
pdx=0 pdy=0 b=btn()%16 --outputs bit values for directional buttons(5,6,9,or 10 means diagonals) accel=.1 friction=.94 if (b==5 or b==6 or b==9 or b==10) accel=.0707 if (btn(⬅️)) pdx-=accel if (btn(➡️)) pdx+=accel if (btn(⬆️)) pdy-=accel if (btn(⬇️)) pdy+=accel if (not btn(⬅️) and not btn(➡️)) pdx*=friction if (not btn(⬆️) and not btn(⬇️)) pdy*=friction if (abs(pdx)<.07) pdx=0 if (abs(pdy)<.07) pdy=0 px+=pdx py+=pdy if (px>122) px=122 pdx=0 if (px<0) px=0 pdx=0 if (py>122) py=122 pdy=0 if (py<0) py=0 pdy=0 |


Thanks for replying! I really appreciate it.
b=btn()%16 --outputs bit values for directional buttons(5,6,9,or 10 means diagonals) accel=.1 friction=.94 if (b==5 or b==6 or b==9 or b==10) accel=.0707
Oh my goodness what is this magic?!?! So many tokens saved <3 Thank you for showing me this!
I have changed my code and it works!
Next: I'll try and implement a "quick turn" style movement so the player can turn back quickly (like smash bros when you let go of the stick before moving in the opposite direction 😉 )
Again, I'm so very grateful or you help here. 🙇
James/Cheapshot


Glad I could help. I learned about the btn() feature and how it outputs button bit values if no arguments are entered from reading about optimization strategies for Tweetcarts. There are definitely some cool optimization strategies available if you dig into the system memory a little bit instead of always going with the standard functions.
Oh, one other thing I thought of, if you're noticing the character gets moving too fast, you might want to limit its maximum speed, using something like this.
max=3 if (abs(pdx)>max) pdx=mid(-max,pdx,max) if (abs(pdy)>max) pdy=mid(-max,pdy,max) |
James/JadeLombax =)
[Please log in to post a comment]