Sometimes you just can't get what you want by plotting sprites cause they may not be there or you've put a bunch of binary code on top of 'em. Sometimes you just MUST pixel.
Well, that's all good and well, and there's a few ways to go about it.
[1] Record every pixel in the area ahead of time and later plot it all out again.
[2] As above but only plot select pixels. In this case, do not plot black pixels.
[3] Unique method caching pixels. Explained later below.
[4] NEW ! Shoxidizer's Serializer.
[5] NEW ! Using PEEK and POKE.
Try this program I wrote:
Use LEFT and RIGHT arrow keys to change drawing method.
Lots of moving and colorful sprites. It starts out in MODE 0, which is the 1st above.
. . .
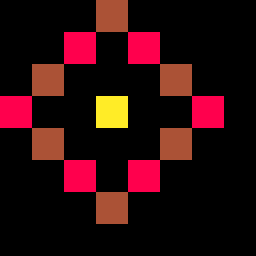
In mode zero you can see above it records every pixel in the sprite and then plots back every single pixel without caring what color they are. For moving 100 sprites, this uses up 63% of your CPU.
. . .
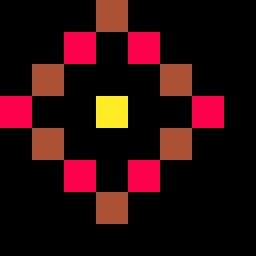
Mode 1 is better and more intelligent. It like above records every pixel in the sprite area but when it comes back to plotting, it only plots pixels that are not color zero (black). This gives you a slight speed increase. Uses 52% of your CPU.
. . .
So what's going on with the cache method which is the fastest using only 32% of your CPU ? Let me see if I can explain.
Instead of using an array that is 8x8 in size to house every pixel, it creates a new single dimensional array that has 3-separate elements in it.
The first is the X-position in the pixel, the next is the Y-position, lastly the color.
So how is this different from the two above ?
Instead of going through 64-different pixels to see which is lit and which is not, it records the X/Y coordinates for each pixel plus the color and saves THAT as a single item in the array.
I'll let you think about that for a minute before giving an answer. Scroll down to see how it's done. No, I don't know how to hide text in Lex.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
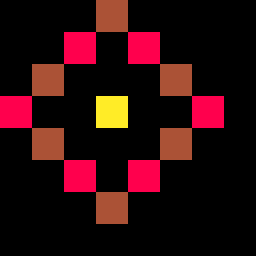
So is this what you were expecting ? If so, congratulations, you got it right ! :D
Yes, THIS method of not only recording each pixel you want plotted but their exact X+Y coordinates runs quite a bit faster than the standard 8x8 sweeping method.
So there you have it.
HOPE THIS HELPS !
NOW DO YOU know of a faster way to plot individual pixels from a table or sprite sheet ?



I managed to make another method that is usually worse, but shaves off a couple of percentage points under certain circumstances. It takes the serialized sprite and breaks it into a multiple rows based on color. To draw, it goes through each row, only having to load each color once.
If the sprites are just 1 or 2 colors and not too small, this comes out slightly faster. Here it is modded in as mode 3 with sprites that it can handle faster.


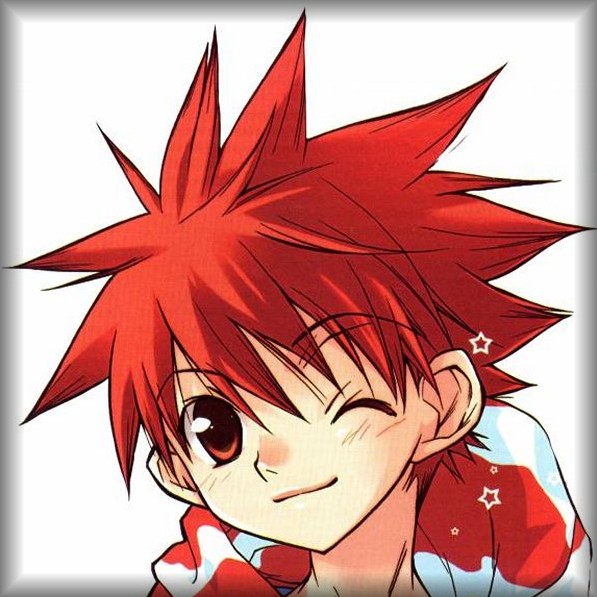
Hmm !
Going to have to set down and see what cleverness you did here, @Shoxidizer.
... ???
Oh, I see what you did here. Hmm ... Yes, if you use a lot of colors that would be slower on your method.
If we don't mind peeks and pokes, I think I can get it even faster. Now understand the pixels are here because you don't want to interfere with your sprite set. Therefore it is possible to save "sprites" via string or arrays.

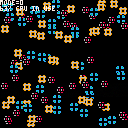
This method may be a bit of a cheat, Shoxidizer, as it uses PEEK4() and POKE4() and SPR() but it does work well, and should work for any image of any number of pixels across and down. If it's to be bigger than 8x8, record that area and then use SSPR() to temporarily render it before recovering the memory you overwrote in the sprite sheet to display it.
This is not the first time I have seen people use the Sprite Sheet as a temporary storage to do some amazing things.
I should be able to use this method for the Book Reader without interfering with the book data (stored in sprites) and then it will really run quickly.
... so I think what I learned from this experience, you CAN use sprites even if you don't want to touch them. Just temporarily save what you're going to overwrite in the sprite sheet, temporarily POKE what you need in the sprite sheet, draw it, then recover the memory you overwrote. Fastest method of all.
[Please log in to post a comment]