
Hello, I'm trying to develop a game in which there will be some enemies chasing the player on a map, and on this map there will be some obstacles.
I know how to code a simple AI in order to have enemies follow the player, by using math trigonometry functions like sine, cosine and arctangent; but I ACTUALLY HAVE NO IDEA about how to code an enemy AI in order to make it avoiding some obstacles on the screen.
Basically I'd like to code a simple AI that could chase the player on the map, but at the right time when it's about to collide with an obstacle could forget for a moment that chase and dodge the obstacle.
Do you have an idea about what I should try to implement ?
At the moment I've tried something very simple that tries to rotate around an obstacles while the trajectory between the enemy and the obstacle and the trajectory between the enemy and the player is equal or greater than 90 degrees. It's a really simple trick but it doesn't work 100% of the time.
This is my code:
function _init() -- initialize -- enemy e = {} e.f = 1 e.x = 0 e.y = 0 -- player p = {} p.found = false p.distance_b = 0 p.f = 2 p.x = 118 p.y = 118 -- columns bb = {} b1 = {} b1.f = 3 b1.x = 40 b1.y = 40 b2 = {} b2.f = 3 b2.x = 80 b2.y = 80 add(bb, b1) add(bb, b2) end function object_collide_with(x1, y1, x2, y2, gx, gy) -- checks if an object has collided -- with another object, then calculated -- the angle between the ball and the other object -- gx = gravity horizontal center -- gy = gravity vertical center if (gx == nil) gx = 4 -- 8x8 --> 8/2 if (gy == nil) gy = 4 --local bump = c1 and c2 and c3 and c4 local distance = sqrt(((x2+gx)-(x1+gx))^2 + ((y2+gy)-(y1+gy))^2) local angle = atan2((x1 + gx) - (x2 + gx), (y1 + gy) - (y2 + gy)) local direction = flr(angle * 8) return distance, angle, direction end function player_collide_with(x, y, w, h) -- checks if the player has collided -- with another object, then calculated -- the angle between the ball and the other object return object_collide_with(p.x, p.y, x, y, w, h) end function distance_between(x1, y1, x2, y2) -- calculates the distance between two points return sqrt((x2-x1)^2 + (y2-y1)^2) end function check_player() if (btn(0)) p.x -= 1 if (btn(1)) p.x += 1 if (btn(2)) p.y -= 1 if (btn(3)) p.y += 1 end function _update() check_player() p.found = false local distance, angle, direction -- check the distance from the target distance, angle, direction = player_collide_with(e.x, e.y) for i, b in pairs(bb) do local distance_b, angle_b, direction_b = object_collide_with(b.x, b.y, e.x, e.y) if distance_b <= 10 and abs(angle-angle_b) < 1/6 then angle_b += 3.1415/240 e.x = b.x - cos(angle_b) * distance_b e.y = b.y - sin(angle_b) * distance_b p.found = true break end end if not p.found then e.x += cos(angle) e.y += sin(angle) end if distance_between(e.x, e.y, p.x, p.y) < 8 then -- reset if the enemy touches the player e.x = 0 e.y = 0 end end function _draw() cls(0) for i, b in pairs(bb) do spr(b.f, b.x, b.y) end spr(p.f, p.x, p.y) spr(e.f, e.x, e.y) end |


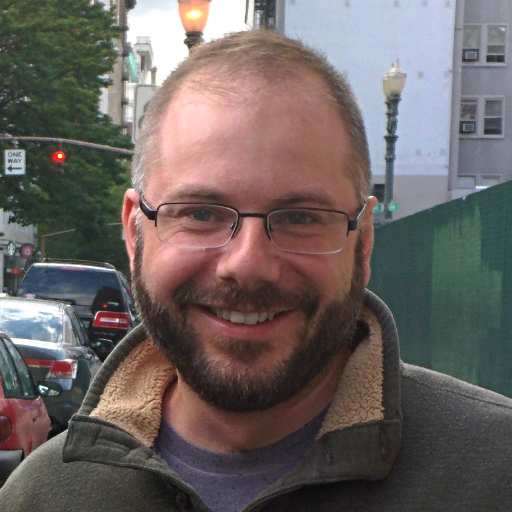
The nice thing about using pathfinding is that it only looks like the enemy "forgot" about the player. In fact, the enemy is still following the player, but it's just following a clear path to the player instead. Fanzine #4 has a tutorial for implementing pathfinding in PICO-8.



You could also look into steering behaviours. (You've already written most of what you need for the basic behaviours, FWIW)
Here's a good set of tutorials to start with - https://gamedevelopment.tutsplus.com/series/understanding-steering-behaviors--gamedev-12732
[Please log in to post a comment]